
Sat Chat
Dependencies: 4DGL-uLCD-SE USBDevice max32630fthr
main.cpp
00001 /* BLACK CODE INCLUDE START 00002 External libraries are worrying! 00003 Keep it simple and avoid anything non essential or obscure. 00004 Consider copying functions from libraries into the code so 00005 we have control. */ 00006 #include "mbed.h" 00007 #include "uLCD_4DGL.h" 00008 #include "max32630fthr.h" 00009 #include <stdbool.h> 00010 #include <string> 00011 //#include <Keypad.h> 00012 /* BLACK CODE INCLUDE END */ 00013 00014 /* BLACK CODE DEFINE START */ 00015 #define ONN 0 00016 #define OFFF 1 00017 #define EXIT_SUCCESS 0 00018 #define EXIT_FAILURE 1 00019 /* BLACK CODE DEFINE END */ 00020 00021 /* BLACK CODE GLOBAL VAR START */ 00022 //const int GPS_TIMEOUT=180; //Wait three minutes maximum for GPS. 00023 char gpsfix_last_utc_time[11] = {0}; 00024 char gpsfix_last_utc_date[7] = {0}; 00025 char gpsfix_longtitude[12] = {0}; 00026 char gpsfix_latitude[12] = {0}; 00027 char gpsfix_speed[8] = {0}; //Set but not used 00028 char gpsfix_course[7] = {0}; //Set but not used 00029 char gpsfix_variation[7] = {0}; //Set but not used 00030 char gpsfix_mag_var_ew[1] = {0};//Set but not used 00031 char gpsfix_ns = 0; 00032 char gpsfix_ew = 0; 00033 bool gps_data_present = false; //If this is false we can't even use stale GPS data. 00034 /* BLACK CODE GLOBAL VAR END */ 00035 00036 /* BLACK CODE PERIPHERAL INIT START */ 00037 uLCD_4DGL uLCD(P3_1,P3_0,P5_2); 00038 DigitalOut red_led(LED1,1); 00039 DigitalOut green_led(LED2,1); 00040 DigitalOut gps_led(LED3,1); //Blue 00041 AnalogIn joyX (AIN_0); 00042 AnalogIn joyY (AIN_1); 00043 DigitalIn joyButton(P4_0); 00044 //Buttons 00045 DigitalIn redButton (P5_6,PullUp); 00046 DigitalIn blackButton (P5_5,PullUp); 00047 //Keypad 00048 DigitalIn keypadLine2(P3_2); 00049 DigitalIn keypadLine7(P5_0); 00050 DigitalIn keypadLine6(P6_0); 00051 DigitalIn keypadLine4(P3_4); 00052 DigitalOut keypadLine3(P3_5); 00053 DigitalOut keypadLine1(P3_3); 00054 DigitalOut keypadLine5(P5_1); 00055 Serial pc(USBTX, USBRX); 00056 Serial gps(P5_3, P5_4, 9600); 00057 I2C i2c(P5_7,P6_0); // SDA, SCL 00058 /* BLACK CODE PERIPHERAL INIT END */ 00059 00060 void home(); 00061 void CompareKey(); 00062 void Getmessage(); 00063 void TrackingService(); 00064 void Sos(); 00065 char ChartoAlpha(char num); 00066 void shortcut(); 00067 void ReadMessage(); 00068 void PrintChar(char key); 00069 void tracking_power(bool state); 00070 00071 00072 /*char getkey(void) 00073 { 00074 keypadLine3 = 0; 00075 keypadLine1 = 1; 00076 keypadLine5 = 1; 00077 if (!keypadLine2) { 00078 00079 wait(.5); 00080 return '1'; 00081 } 00082 if (!keypadLine7) { 00083 wait(.5); 00084 return '4'; 00085 } 00086 if (!keypadLine6) { 00087 wait(.5); 00088 return '7'; 00089 } 00090 if (!keypadLine4) { 00091 wait(.5); 00092 return '*'; 00093 } 00094 keypadLine3 = 1; 00095 keypadLine1 = 0; 00096 if (!keypadLine2) { 00097 wait(.5); 00098 return '2'; 00099 00100 } 00101 if (!keypadLine7) { 00102 wait(.5); 00103 return '5'; 00104 } 00105 if (!keypadLine6) { 00106 wait(.5); 00107 return '8'; 00108 } 00109 if (!keypadLine4) { 00110 wait(.5); 00111 return '0'; 00112 } 00113 keypadLine1 = 1; 00114 keypadLine5 = 0; 00115 if (!keypadLine2) { 00116 wait(.5); 00117 return '3'; 00118 } 00119 if (!keypadLine7) { 00120 wait(.5); 00121 return '6'; 00122 } 00123 if (!keypadLine6) { 00124 00125 wait(.5); 00126 return '9'; 00127 00128 } 00129 if (!keypadLine4) { 00130 wait(.5); 00131 return '#'; 00132 } 00133 return NULL; 00134 } 00135 */ 00136 00137 /*int i, k, pwcount=0; 00138 char password[] = "999", entry[10]; 00139 00140 char getkey() 00141 { 00142 00143 keypadLine3 = 0; 00144 keypadLine1 = 1; 00145 keypadLine5 = 1; 00146 if (!keypadLine2) { 00147 entry[pwcount] = '1'; 00148 // pwcount++; 00149 wait(.5); 00150 00151 return '1' ; 00152 } 00153 if (!keypadLine7) { 00154 entry[pwcount] = '4'; 00155 // pwcount++; 00156 wait(.5); 00157 00158 return '4' ; 00159 } 00160 if (!keypadLine6) { 00161 entry[pwcount] = '7'; 00162 // pwcount++; 00163 wait(.5); 00164 return '7'; 00165 } 00166 if (!keypadLine4) { 00167 entry[pwcount] = '*'; 00168 // pwcount++; 00169 wait(.5); 00170 return '*'; 00171 } 00172 keypadLine3 = 1; 00173 keypadLine1 = 0; 00174 if (!keypadLine2) { 00175 entry[pwcount] = '2'; 00176 // pwcount++; 00177 wait(.5); 00178 00179 return '2' ; 00180 } 00181 if (!keypadLine7) { 00182 entry[pwcount] = '5'; 00183 // pwcount++; 00184 wait(.5); 00185 return '5'; 00186 } 00187 if (!keypadLine6) { 00188 entry[pwcount] = '8'; 00189 // pwcount++; 00190 wait(.5); 00191 return '8'; 00192 } 00193 if (!keypadLine4) { 00194 entry[pwcount] = '0'; 00195 // pwcount++; 00196 wait(.5); 00197 return '0'; 00198 } 00199 keypadLine1 = 1; 00200 keypadLine5 = 0; 00201 if (!keypadLine2) { 00202 entry[pwcount] = '3'; 00203 // pwcount++; 00204 wait(.5); 00205 00206 return '3' ; 00207 } 00208 if (!keypadLine7) { 00209 entry[pwcount] = '6'; 00210 // pwcount++; 00211 wait(.5); 00212 return '6'; 00213 } 00214 if (!keypadLine6) { 00215 entry[pwcount] = '9'; 00216 pwcount++; 00217 uLCD.printf("%d",pwcount); 00218 wait(.5); 00219 return '9'; 00220 } 00221 if (!keypadLine4) { 00222 entry[pwcount] = '#'; 00223 pwcount++; 00224 wait(.5); 00225 return '#'; 00226 } 00227 if (blackButton == 0) 00228 { 00229 wait(.5); 00230 pwcount=0; 00231 for(k=0; k<=3; k++){ 00232 if(password[k] != entry[k]){ 00233 k=0; 00234 uLCD.cls(); 00235 uLCD.text_string("Wrong Number", 1, 4, FONT_7X8, GREEN); 00236 uLCD.printf("%d",k); 00237 wait(3); 00238 uLCD.cls(); 00239 Sos(); 00240 break; 00241 } 00242 if(k==2){ 00243 uLCD.cls(); 00244 uLCD.text_string("Calling has\nreached!", 1, 4, FONT_7X8, GREEN); 00245 uLCD.printf("%d",k); 00246 wait(3); 00247 uLCD.cls(); 00248 home(); 00249 break; 00250 } 00251 } 00252 for(k=0; k<10; k++) 00253 entry[k]=0; 00254 } 00255 return NULL; 00256 } 00257 */ 00258 00259 int i, k, pw2 , j,pwcount=0; 00260 char sos[] = "999", entry[10]; 00261 char track2[] = "111", entry2[10]; bool track ; 00262 char getkey() 00263 { 00264 00265 keypadLine3 = 0; 00266 keypadLine1 = 1; 00267 keypadLine5 = 1; 00268 if (!keypadLine2) { 00269 entry2[pw2] = '1'; 00270 pw2++; 00271 wait(.5); 00272 return '1'; 00273 } 00274 if (!keypadLine7) { 00275 entry[pwcount] = '4'; 00276 00277 wait(.5); 00278 00279 return '4' ; 00280 } 00281 if (!keypadLine6) { 00282 entry[pwcount] = '7'; 00283 00284 wait(.5); 00285 return '7'; 00286 } 00287 if (!keypadLine4) { 00288 entry[pwcount] = '*'; 00289 00290 wait(.5); 00291 pw2=0; 00292 for(j=0; j<=3; j++){ 00293 if(track2[j] != entry2[j]){ 00294 uLCD.cls(); 00295 uLCD.text_string("Invalid\nKeypressed\n", 1, 4, FONT_7X8, GREEN); 00296 wait(3); 00297 uLCD.cls(); 00298 for(j=0; j<10; j++) 00299 entry2[j]=0; 00300 TrackingService(); 00301 break; 00302 } 00303 if(j==2){ 00304 uLCD.cls(); 00305 track =! track ; 00306 if ( track == ONN) 00307 { 00308 uLCD.text_string("Tracking has\nswitched On!", 1, 4, FONT_7X8, GREEN); 00309 } 00310 if ( track == OFFF ) 00311 { 00312 uLCD.text_string("Tracking has\nswitched OFF!", 1, 4, FONT_7X8, GREEN); 00313 } 00314 wait(3); 00315 uLCD.cls(); 00316 for(j=0; j<10; j++) 00317 entry2[j]=0; 00318 home(); 00319 break; 00320 } 00321 00322 } 00323 for(j=0; j<10; j++) 00324 entry2[j]=0; 00325 return '*'; 00326 } 00327 keypadLine3 = 1; 00328 keypadLine1 = 0; 00329 if (!keypadLine2) { 00330 entry[pwcount] = '2'; 00331 00332 wait(.5); 00333 00334 return '2' ; 00335 } 00336 if (!keypadLine7) { 00337 entry[pwcount] = '5'; 00338 00339 wait(.5); 00340 return '5'; 00341 } 00342 if (!keypadLine6) { 00343 entry2[pwcount] = '8'; 00344 pw2++; 00345 wait(.5); 00346 return '8'; 00347 } 00348 if (!keypadLine4) { 00349 entry[pwcount] = '0'; 00350 00351 wait(.5); 00352 return '0'; 00353 } 00354 keypadLine1 = 1; 00355 keypadLine5 = 0; 00356 if (!keypadLine2) { 00357 entry[pwcount] = '3'; 00358 00359 wait(.5); 00360 00361 return '3' ; 00362 } 00363 if (!keypadLine7) { 00364 entry[pwcount] = '6'; 00365 00366 wait(.5); 00367 return '6'; 00368 } 00369 if (!keypadLine6) { 00370 entry[pwcount] = '9'; 00371 pwcount++; 00372 00373 wait(.5); 00374 return '9'; 00375 } 00376 if (!keypadLine4) { 00377 entry[pwcount] = '#'; 00378 00379 wait(.5); 00380 00381 00382 return '#'; 00383 } 00384 if (blackButton == 0) 00385 { 00386 wait(.5); 00387 pwcount=0; 00388 for(k=0; k<=3; k++){ 00389 if(sos[k] != entry[k]){ 00390 uLCD.cls(); 00391 uLCD.text_string("Wrong Number", 1, 4, FONT_7X8, GREEN); 00392 wait(3); 00393 uLCD.cls(); 00394 for(k=0; k<10; k++) 00395 entry[k]=0; 00396 Sos(); 00397 break; 00398 } 00399 if(k==2){ 00400 uLCD.cls(); 00401 uLCD.text_string("SOS calling has\nreached!", 1, 4, FONT_7X8, GREEN); 00402 wait(3); 00403 uLCD.cls(); 00404 for(k=0; k<10; k++) 00405 entry[k]=0; 00406 home(); 00407 break; 00408 } 00409 00410 } 00411 for(k=0; k<10; k++) 00412 entry[k]=0; 00413 } 00414 00415 return NULL; 00416 } 00417 00418 00419 void ReadMessage() 00420 { 00421 00422 uLCD.printf("\n1.Hey I have been busy..... "); 00423 uLCD.printf("\n2.Hi Sir,we would like..... "); 00424 uLCD.printf("\n3.Darling,Be safe.C you..... "); 00425 while ( true) 00426 { char cut = getkey(); 00427 switch ( cut ) 00428 { 00429 case '1' : uLCD.cls(); 00430 uLCD.text_string("Hey I have been\nbusy these weeks\nSorry I cannot meet\nwith you.see you\nwhen you'r back!", 1, 4, FONT_7X8, GREEN); 00431 wait (5); 00432 uLCD.cls(); 00433 ReadMessage(); 00434 break; 00435 00436 case '2' : uLCD.cls(); 00437 uLCD.text_string("Hi Sir.\nwe would like\nto confirm for\nyour booking\non 2nd Nov 2017", 1, 4, FONT_7X8, GREEN); 00438 wait (5); 00439 uLCD.cls(); 00440 ReadMessage(); 00441 break; 00442 case '3' : uLCD.cls(); 00443 uLCD.text_string("Darling,\nBe safe.\nWe will join you\non 2nd Nov", 1, 4, FONT_7X8, GREEN); 00444 wait (5); 00445 uLCD.cls(); 00446 ReadMessage(); 00447 break; 00448 00449 00450 case '#' : 00451 uLCD.cls(); 00452 home(); 00453 break; 00454 00455 00456 case '0' : 00457 uLCD.cls(); 00458 Getmessage(); 00459 break; 00460 00461 00462 } 00463 } 00464 } 00465 00466 00467 00468 char ChartoAlpha(char num) 00469 { 00470 00471 if ( num == '2') 00472 { 00473 00474 uLCD.putc('A'); 00475 00476 } 00477 if ( num == '2' && num == '2' ) 00478 { 00479 00480 uLCD.putc('B'); 00481 00482 } 00483 if ( num == '2' && num == '2' && num == '2' ) 00484 { 00485 wait(.5); 00486 uLCD.putc('C'); 00487 00488 } 00489 if ( num == '0' ) 00490 { wait(.2); 00491 uLCD.putc(' '); 00492 } 00493 if ( num == '3' ) 00494 { wait(.2); 00495 uLCD.putc('D'); 00496 } 00497 00498 if ( num == '4' ) 00499 { wait(.2); 00500 uLCD.putc('G'); 00501 } 00502 if ( num == '5' ) 00503 { wait(.2); 00504 uLCD.putc('J'); 00505 } 00506 if ( num == '6' ) 00507 { wait(.2); 00508 uLCD.putc('M'); 00509 } 00510 if ( num == '7' ) 00511 { wait(.2); 00512 uLCD.putc('P'); 00513 } 00514 if ( num == '8' ) 00515 { wait(.2); 00516 uLCD.putc('T'); 00517 } 00518 if ( num == '9' ) 00519 { wait(.2); 00520 uLCD.putc('W'); 00521 } 00522 if (blackButton == 0) 00523 { 00524 uLCD.cls(); 00525 uLCD.text_string("Your message\nhas been\nsucessfully sent!", 1, 4, FONT_7X8, GREEN); 00526 wait(2); 00527 uLCD.cls(); 00528 home(); 00529 } 00530 if ( num == '#') 00531 { 00532 uLCD.cls(); 00533 home(); 00534 } 00535 00536 } 00537 00538 void shortcut() 00539 { 00540 uLCD.printf("\n Press 1 to call help!"); 00541 uLCD.printf("\n Press 2 to say you are safe!"); 00542 uLCD.printf("\n Press 3 to request for your GPS co-ordinates!"); 00543 00544 while ( true) 00545 { char cut = getkey(); 00546 switch ( cut ) 00547 { 00548 case '1' : uLCD.cls(); 00549 uLCD.text_string("Calling Help\nIt will\narrive in 30-45mins!", 1, 4, FONT_7X8, GREEN); 00550 wait (5); 00551 uLCD.cls(); 00552 shortcut(); 00553 break; 00554 00555 case '2' : uLCD.cls(); 00556 uLCD.text_string("Thank you.\nIf you need\nany help,\nPress *999 for \nSOS!", 1, 4, FONT_7X8, GREEN); 00557 wait (5); 00558 uLCD.cls(); 00559 shortcut(); 00560 break; 00561 00562 00563 case '#' : 00564 uLCD.cls(); 00565 home(); 00566 break; 00567 00568 00569 case '0' : 00570 uLCD.cls(); 00571 Getmessage(); 00572 break; 00573 00574 00575 } 00576 } 00577 } 00578 00579 void Getmessage() 00580 { 00581 uLCD.printf("\n1.Type message"); 00582 uLCD.printf("\n2.Short-cut message"); 00583 uLCD.printf("\n3.Read message"); 00584 uLCD.printf("\n4.Delete message"); 00585 00586 char key = getkey(); 00587 00588 uLCD.printf("\n\n Press # to go back to home page"); 00589 while ( true) 00590 { 00591 char keyy = getkey(); 00592 00593 if ( keyy == '#' ) 00594 { uLCD.cls(); 00595 home(); } 00596 00597 if ( keyy == '0' ) 00598 { uLCD.cls(); 00599 Getmessage(); } 00600 00601 if ( keyy == '1' ) 00602 { 00603 uLCD.cls(); 00604 uLCD.printf("\n Type your message and Press Black button to send.\n"); 00605 while (true) 00606 { char num = getkey(); 00607 00608 char alpah = ChartoAlpha(num); 00609 00610 } 00611 } 00612 if ( keyy == '2' ) 00613 { uLCD.cls(); 00614 shortcut(); 00615 } 00616 00617 if ( keyy == '3' ) 00618 { uLCD.cls(); 00619 ReadMessage(); 00620 } 00621 if ( keyy == '4' ) 00622 { uLCD.cls(); 00623 uLCD.printf("\n Select to delete message: "); 00624 } 00625 00626 00627 } 00628 00629 } 00630 00631 00632 00633 00634 void TrackingService() 00635 { 00636 if ( track == ONN ) 00637 { 00638 uLCD.printf("Tracking Service is On!Press 111 and * to turn off!"); 00639 uLCD.printf("\n\n Press # to go back to home page"); 00640 } 00641 if ( track == OFFF ) 00642 { 00643 uLCD.printf("Tracking Service is Off!Press 111 and * to turn on!"); 00644 uLCD.printf("\n\n Press # to go back to home page"); 00645 } 00646 00647 00648 00649 while ( true) 00650 { 00651 char keyy = getkey(); 00652 uLCD.putc(keyy); 00653 if ( keyy == '#' ) 00654 { uLCD.cls(); 00655 home(); } 00656 } 00657 00658 } 00659 00660 void Sos() 00661 { 00662 k = 0 ; 00663 uLCD.printf("\n Press 999 and black button for SOS service! "); 00664 00665 uLCD.printf("\n\n Press # to go back to home page\n\n"); 00666 while (true) 00667 { 00668 char key = getkey(); 00669 uLCD.putc(key); 00670 00671 if ( key == '#' ) 00672 { uLCD.cls(); 00673 home(); } 00674 00675 } 00676 00677 } 00678 00679 void GPS() 00680 { 00681 uLCD.printf("\nYour co-ordinates now is X=%i Y=%i ", int(joyX.read_u16()), int(joyY.read_u16())); 00682 00683 00684 uLCD.printf("\n\n Press # to go back to home page"); 00685 while ( true) 00686 { 00687 char keyy = getkey(); 00688 00689 if ( keyy == '#' ) 00690 { uLCD.cls(); 00691 home(); } 00692 } 00693 } 00694 00695 void Onoff() 00696 { 00697 uLCD.printf("\nPress Red Button to power off device"); 00698 00699 00700 uLCD.printf("\n\n Press # to go back to home page"); 00701 while ( true) 00702 { 00703 char keyy = getkey(); 00704 if (redButton == 0) 00705 { uLCD.cls(); 00706 uLCD.text_string("Shutting\nDown.......", 1, 4, FONT_7X8, GREEN); 00707 wait(3 ); 00708 uLCD.reset(); 00709 00710 } 00711 00712 if ( keyy == '#' ) 00713 { uLCD.cls(); 00714 home(); } 00715 00716 00717 } 00718 } 00719 00720 00721 void home() 00722 { 00723 00724 uLCD.printf("\nHello\nWelcome to SatChat.\n"); //Default Green on black text 00725 uLCD.printf("\n1.Message"); 00726 uLCD.printf("\n2.Call for help(#999)"); 00727 uLCD.printf("\n3.Tracking Service"); 00728 uLCD.printf("\n4.Request GPS co-ordinates"); 00729 uLCD.printf("\n5.Power on/off"); 00730 00731 00732 while(true) 00733 { 00734 00735 gps_led= !gps_led ; 00736 //uLCD.printf("\nJoystick: X=%i Y=%i ", int(joyX.read_u16()), int(joyY.read_u16())); 00737 if (joyButton==0){ 00738 uLCD.printf("Joystick Button pressed"); 00739 } 00740 00741 char keypressed = getkey(); 00742 if (keypressed == '1') 00743 { 00744 uLCD.cls(); 00745 Getmessage(); 00746 } 00747 if (keypressed == '2') 00748 { 00749 uLCD.cls(); 00750 Sos(); 00751 } 00752 if (keypressed == '3') 00753 { 00754 uLCD.cls(); 00755 00756 TrackingService(); 00757 00758 } 00759 00760 if (keypressed == '4') 00761 { 00762 uLCD.cls(); 00763 GPS(); 00764 } 00765 if (keypressed == '5') 00766 { 00767 uLCD.cls(); 00768 Onoff(); 00769 } 00770 if (redButton == 0) 00771 { uLCD.cls(); 00772 uLCD.text_string("Shutting\nDown.......", 1, 4, FONT_7X8, GREEN); 00773 wait(3 ); 00774 uLCD.reset(); 00775 00776 } 00777 if (blackButton == 0){ 00778 uLCD.printf(",Black button pressed"); 00779 uLCD.cls(); 00780 00781 } 00782 } 00783 } 00784 /* 00785 void tracking_power(bool state) 00786 /* BLACK CODE 00787 MAX32630FTHR routine to control the output of the 3v3 line. 00788 This is achieved by issuing commands to the MAX14690 controller. 00789 In this case when the GPS is shutdown we clear any serial 00790 data to avoid issues with mbeds buggy serial code */ 00791 /*{ 00792 /* char data[2]; 00793 data[0] = 0x16; //MAX14690 LDO3cfg register 00794 uLCD.printf("Tracing Service is:"); 00795 if (state == ONN) { 00796 data[1] = 0xE2; //Enable LDO3 00797 i2c.write( 0x50, data, 2 ); 00798 gps_led= ONN; 00799 uLCD.printf("ON\n\r"); 00800 } else { 00801 data[1] = 0xE0; //Disable LDO3 00802 i2c.write( 0x50, data, 2 ); 00803 gps_led=OFFF; 00804 while (gps.readable()) { 00805 char dummy = gps.getc(); //Empty serial buffer because overflows reveal MBED bugs :-( 00806 } 00807 uLCD.printf("OFF\n\r"); 00808 } 00809 } 00810 00811 int get_epoch_from_last_gps_time(void) 00812 /* BLACK CODE 00813 Given appropriate global char arrays of time and date information, 00814 return a unix time epoch. 00815 */ 00816 /*{ 00817 struct tm t; 00818 time_t epoch; 00819 char two_char_str[3] = {0}; 00820 memcpy(two_char_str, gpsfix_last_utc_date+4, 2); 00821 t.tm_year = atoi(two_char_str)+100; //Years since 1900 00822 memcpy(two_char_str, gpsfix_last_utc_date+2, 2); 00823 t.tm_mon = atoi(two_char_str)-1; // Month, 0 - jan gpsfix_last_utc_date 00824 memcpy(two_char_str, gpsfix_last_utc_date, 2); 00825 t.tm_mday = atoi(two_char_str); // Day of the month gpsfix_last_utc_date 00826 memcpy(two_char_str, gpsfix_last_utc_time, 2); 00827 t.tm_hour = atoi(two_char_str); 00828 memcpy(two_char_str, gpsfix_last_utc_time+2, 2); 00829 t.tm_min = atoi(two_char_str); 00830 memcpy(two_char_str, gpsfix_last_utc_time+4, 2); 00831 t.tm_sec = atoi(two_char_str); 00832 t.tm_isdst = 0; // Is DST on? 1 = yes, 0 = no, -1 = unknown 00833 epoch = mktime(&t); 00834 return epoch; 00835 //BLACK CODE 00836 } 00837 00838 /*int gps_update(void) 00839 /* BLACK CODE 00840 gps_update 00841 Reads NMEA data from a serial interface defined as "gps". 00842 The function waits for a valid $GPRMC sentence. It then decodes the sentence and populates the 00843 following global variables which are assumed to exist. 00844 00845 gpsfix_last_utc_time[11] = {0}; char gpsfix_last_utc_date[7] = {0};char gpsfix_longtitude[12] = {0}; 00846 char gpsfix_latitude[12] = {0}; char gpsfix_speed[8] = {0}; char gpsfix_course[7] = {0}; 00847 char gpsfix_variation[7] = {0}; char gpsfix_mag_var_ew[1] = {0}; char gpsfix_ns = 0; char gpsfix_ew = 0; 00848 00849 The following are also assumed to be part of the global declarations. 00850 #define EXIT_SUCCESS 0 00851 #define EXIT_FAILURE 1 00852 const int GPS_TIMEOUT=180; 00853 a gps_power() function that controls power to the GPS unit. 00854 00855 If the function is successful it returns a 0. If a valid fix is not obtined within the GPS_TIMEOUT 00856 period a 1 is returned. 00857 00858 The code has been tested with a uBlox 6M but other GPS units may work. 00859 The code is deliberately blocking as the mbed OS seems to crash on serial interrupts and buffer overflow. 00860 The serial port is continuously read while waiting for a fix. Once a fix is obtained or a timeout occurs 00861 the GPS is powered down and remaining data read out of the buffer. 00862 */ 00863 /*{ 00864 gps_power(ONN); 00865 time_t gps_on_time = time(NULL); //Start time for GPS timeout calculation. 00866 bool wait_for_fix = true; //Set this to false once a fix is obtained. 00867 while (wait_for_fix) { //Keep monitoring the GPS until we get a fix. 00868 if ((time(NULL) - gps_on_time) > GPS_TIMEOUT) { 00869 gps_power(OFFF); 00870 return EXIT_FAILURE; //Return an error if the GPS takes too long for a fix. 00871 } 00872 int checksum = 0; 00873 char nmea_sentence[83] = {0}; //NMEA length max is 82 + 1 terminator. Fill with NULL terminators to save doing it later. 00874 while (gps.getc()!='$'); //wait for start of sentence 00875 int nmea_index = 0; 00876 nmea_sentence[nmea_index] = '$'; //Manually insert the '$' because we don't want it included in the checksum loop 00877 char nmea_char = gps.getc(); //get sentence first char from GPS 00878 while (nmea_char != '*') { //Loop, building sentence and calc'ing CS until a * is seen 00879 checksum ^= nmea_char; //Calc checksum as we read sentence 00880 if ((nmea_sentence[nmea_index] == ',')&&(nmea_char == ',')) { 00881 nmea_sentence[++nmea_index] = ' '; //Pad consecutive comma with a space to make it possible to use strtok with empty values 00882 } 00883 nmea_sentence[++nmea_index] = nmea_char; //build the sentence with the next character 00884 if (nmea_index > 81) { 00885 nmea_index=81; //Don't overflow sentence buffer 00886 } 00887 nmea_char = gps.getc(); //get next char from GPS 00888 } 00889 //Last character was the '*' so next two are CS 00890 char hex_checksum[3] = {0}; 00891 hex_checksum[0] = gps.getc(); 00892 hex_checksum[1] = gps.getc(); 00893 if (checksum == (int)strtol(hex_checksum, NULL, 16) ) { //Compare calc and read checksums. 00894 //Valid sentence so check if it's a GPRMC 00895 const char gprmc[7] = "$GPRMC"; 00896 char *token; 00897 token = strtok(nmea_sentence, ","); 00898 if (strcmp(token,gprmc) == 0) { //GPRMC 00899 pc.printf( " %s\n\r", token ); //Get the time 00900 if (token != NULL) { 00901 token = strtok(NULL, ","); 00902 if (*token != 32) { //If there is a time present (anything but a space), record it. 00903 //pc.printf("Time: %s\n\r",token); 00904 gps_led =! gps_led; //Flash blue LED 00905 memcpy(gpsfix_last_utc_time, token, sizeof gpsfix_last_utc_time - 1); 00906 } 00907 } 00908 if (token != NULL) { 00909 token = strtok(NULL, ","); 00910 if (*token == 'V') { 00911 pc.printf("VOID"); 00912 } 00913 } 00914 if (*token == 'A') { //Is this an 'A'ctive (valid) fix? 00915 pc.printf("Got a fix\n\r"); 00916 gps_power(OFF); //Yes - No need for GPS now 00917 wait_for_fix = false; //Stop looping now we have a fix. 00918 if (token != NULL) { 00919 token = strtok(NULL, ","); 00920 pc.printf("Latitude: %s\n\r",token); 00921 memcpy(gpsfix_latitude, token, sizeof gpsfix_latitude - 1); 00922 } 00923 if (token != NULL) { 00924 token = strtok(NULL, ","); 00925 pc.printf("North/South: %s\n\r",token); 00926 gpsfix_ns = *token; 00927 } 00928 if (token != NULL) { 00929 token = strtok(NULL, ","); 00930 pc.printf("Longitude: %s\n\r",token); 00931 memcpy(gpsfix_longtitude, token, sizeof gpsfix_longtitude - 1); 00932 } 00933 if (token != NULL) { 00934 token = strtok(NULL, ","); 00935 pc.printf("East/West: %s\n\r",token); 00936 gpsfix_ew = *token; 00937 } 00938 if (token != NULL) { 00939 token = strtok(NULL, ","); 00940 //pc.printf("Speed in knots: %s\n\r",token); 00941 } 00942 if (token != NULL) { 00943 token = strtok(NULL, ","); 00944 //pc.printf("True course: %s\n\r",token); 00945 } 00946 if (token != NULL) { 00947 token = strtok(NULL, ","); 00948 pc.printf("Date: %s\n\r",token); 00949 memcpy(gpsfix_last_utc_date, token, sizeof gpsfix_last_utc_date - 1); 00950 } 00951 if (token != NULL) { 00952 token = strtok(NULL, ","); 00953 //pc.printf("Variation: %s\n\r",token); 00954 } 00955 if (token != NULL) { 00956 token = strtok(NULL, ","); 00957 //pc.printf("Variation East/West: %s\n\r",token); 00958 } 00959 } 00960 } 00961 } 00962 } 00963 return EXIT_SUCCESS; 00964 //BLACK CODE 00965 }*/ 00966 00967 main() 00968 { /*Set the power button behaviour. 00969 char data[2]; 00970 data[0] = 0x1A; //MAX14690 BootCfg register 00971 data[1] = 0x30; //Always-On Mode, off state via PWR_OFF_CMD 00972 i2c.write( 0x50, data, 2 ); 00973 */ 00974 00975 char data[2]; 00976 data[0] = 0x17; //MAX14690 LDO3Vset register 00977 data[1] = 0x19; //3.3V 00978 i2c.write( 0x50, data, 2 ); 00979 //tracking_power(OFFF); 00980 00981 00982 uLCD.printf("\nTracking Service is On!"); 00983 uLCD.printf("\n\nThis Unit require your GPS fix at every interval"); 00984 uLCD.printf("\n\nPlesae enter your desirable interval for us to check on you"); 00985 00986 wait(3); 00987 00988 while ( true) 00989 { char mins = getkey(); 00990 int min = mins; 00991 if ( mins ) 00992 { uLCD.cls(); 00993 uLCD.printf("\n You have entered %c mins for the interval",mins); 00994 } 00995 if (redButton == 0) 00996 { uLCD.cls(); 00997 uLCD.text_string("Shutting\nDown.......", 1, 4, FONT_7X8, GREEN); 00998 wait(3 ); 00999 uLCD.reset(); 01000 01001 } 01002 uLCD.cls(); 01003 home(); 01004 } 01005 01006 01007 01008 01009 01010 //wait(5); 01011 //uLCD.cls(); 01012 01013 //uLCD.printf("Tracking Service is On!Press 111 to turn off!"); 01014 01015 // gps_led= !gps_led ; 01016 01017 01018 01019 01020 01021 01022 01023 01024 01025 //Set the voltage to 3v3 for the GPS. 01026 /* char data[2]; 01027 data[0] = 0x17; //MAX14690 LDO3Vset register 01028 data[1] = 0x19; //3.3V 01029 i2c.write( 0x50, data, 2 ); 01030 gps_power(OFF);*/ 01031 01032 /* while (1) { 01033 01034 if (gps_update()==EXIT_SUCCESS) { 01035 gps_data_present = true; 01036 int gps_epoch = get_epoch_from_last_gps_time(); 01037 set_time(gps_epoch); 01038 pc.printf("Got a GPS fix and time.\n\r"); 01039 } else { 01040 pc.printf("GPS timed out and we have no existing fix.\n\r"); 01041 pc.printf("We can send an Iridium packet but coordinates are rough.\n\r"); 01042 } 01043 time_t seconds = time(NULL); 01044 //printf("Time as a basic string = %s", ctime(&seconds)); 01045 wait(60); 01046 seconds = time(NULL); 01047 01048 wait(33); 01049 seconds = time(NULL); 01050 printf("Time as a basic string = %s", ctime(&seconds)); 01051 wait(60); 01052 } */ 01053 } 01054 01055 01056 01057 01058 01059 01060 01061 01062
Generated on Wed Jul 13 2022 23:04:59 by
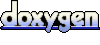