
BLE Thermometer example
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <events/mbed_events.h> 00018 #include "mbed.h" 00019 #include "ble/BLE.h" 00020 #include "ble/services/HealthThermometerService.h" 00021 00022 // Uncomment this line if you want to use the board temperature sensor instead of 00023 // a simulated one. 00024 #define USE_BOARD_TEMP_SENSOR 00025 00026 #ifdef USE_BOARD_TEMP_SENSOR 00027 #include "stm32l475e_iot01_tsensor.h" 00028 #endif 00029 00030 DigitalOut led1(LED1, 1); 00031 00032 const static char DEVICE_NAME[] = "Therm"; 00033 static const uint16_t uuid16_list[] = {GattService::UUID_HEALTH_THERMOMETER_SERVICE}; 00034 00035 static float currentTemperature = 39.6; 00036 static HealthThermometerService *thermometerServicePtr; 00037 00038 static EventQueue eventQueue(/* event count */ 16 * EVENTS_EVENT_SIZE); 00039 00040 /* Restart Advertising on disconnection*/ 00041 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00042 { 00043 BLE::Instance().gap().startAdvertising(); 00044 } 00045 00046 void updateSensorValue(void) { 00047 /* Do blocking calls or whatever is necessary for sensor polling. 00048 In our case, we simply update the Temperature measurement. */ 00049 #ifdef USE_BOARD_TEMP_SENSOR 00050 currentTemperature = BSP_TSENSOR_ReadTemp(); 00051 #else 00052 currentTemperature = (currentTemperature + 0.1 > 43.0) ? 39.6 : currentTemperature + 0.1; 00053 #endif 00054 thermometerServicePtr->updateTemperature(currentTemperature); 00055 } 00056 00057 void periodicCallback(void) 00058 { 00059 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00060 00061 if (BLE::Instance().gap().getState().connected) { 00062 eventQueue.call(updateSensorValue); 00063 } 00064 } 00065 00066 void onBleInitError(BLE &ble, ble_error_t error) 00067 { 00068 /* Initialization error handling should go here */ 00069 } 00070 00071 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00072 { 00073 BLE& ble = params->ble; 00074 ble_error_t error = params->error; 00075 00076 if (error != BLE_ERROR_NONE) { 00077 onBleInitError(ble, error); 00078 return; 00079 } 00080 00081 if (ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00082 return; 00083 } 00084 00085 ble.gap().onDisconnection(disconnectionCallback); 00086 00087 /* Setup primary service. */ 00088 thermometerServicePtr = new HealthThermometerService(ble, currentTemperature, HealthThermometerService::LOCATION_EAR); 00089 00090 /* setup advertising */ 00091 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00092 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00093 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::THERMOMETER_EAR); 00094 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00095 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00096 ble.gap().setAdvertisingInterval(1000); /* 1000ms */ 00097 ble.gap().startAdvertising(); 00098 } 00099 00100 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00101 BLE &ble = BLE::Instance(); 00102 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00103 } 00104 00105 int main() 00106 { 00107 #ifdef USE_BOARD_TEMP_SENSOR 00108 BSP_TSENSOR_Init(); 00109 #endif 00110 00111 eventQueue.call_every(500, periodicCallback); 00112 00113 BLE &ble = BLE::Instance(); 00114 ble.onEventsToProcess(scheduleBleEventsProcessing); 00115 ble.init(bleInitComplete); 00116 00117 eventQueue.dispatch_forever(); 00118 00119 return 0; 00120 }
Generated on Thu Jul 14 2022 23:12:39 by
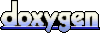