
BLE Button example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <events/mbed_events.h> 00017 00018 #include <mbed.h> 00019 #include "ble/BLE.h" 00020 #include "ble/Gap.h" 00021 #include "ButtonService.h" 00022 00023 DigitalOut led1(LED1, 1); 00024 InterruptIn button(BLE_BUTTON_PIN_NAME); 00025 00026 static EventQueue eventQueue(/* event count */ 10 * EVENTS_EVENT_SIZE); 00027 00028 const static char DEVICE_NAME[] = "Button"; 00029 static const uint16_t uuid16_list[] = {ButtonService::BUTTON_SERVICE_UUID}; 00030 00031 ButtonService *buttonServicePtr; 00032 00033 void buttonPressedCallback(void) 00034 { 00035 eventQueue.call(Callback<void(bool)>(buttonServicePtr, &ButtonService::updateButtonState), true); 00036 } 00037 00038 void buttonReleasedCallback(void) 00039 { 00040 eventQueue.call(Callback<void(bool)>(buttonServicePtr, &ButtonService::updateButtonState), false); 00041 } 00042 00043 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00044 { 00045 BLE::Instance().gap().startAdvertising(); // restart advertising 00046 } 00047 00048 void blinkCallback(void) 00049 { 00050 led1 = !led1; /* Do blinky on LED1 to indicate system aliveness. */ 00051 } 00052 00053 void onBleInitError(BLE &ble, ble_error_t error) 00054 { 00055 /* Initialization error handling should go here */ 00056 } 00057 00058 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00059 { 00060 BLE& ble = params->ble; 00061 ble_error_t error = params->error; 00062 00063 if (error != BLE_ERROR_NONE) { 00064 /* In case of error, forward the error handling to onBleInitError */ 00065 onBleInitError(ble, error); 00066 return; 00067 } 00068 00069 /* Ensure that it is the default instance of BLE */ 00070 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00071 return; 00072 } 00073 00074 ble.gap().onDisconnection(disconnectionCallback); 00075 00076 button.fall(buttonPressedCallback); 00077 button.rise(buttonReleasedCallback); 00078 00079 /* Setup primary service. */ 00080 buttonServicePtr = new ButtonService(ble, false /* initial value for button pressed */); 00081 00082 /* setup advertising */ 00083 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00084 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00085 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00086 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00087 ble.gap().setAdvertisingInterval(1000); /* 1000ms. */ 00088 ble.gap().startAdvertising(); 00089 } 00090 00091 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00092 BLE &ble = BLE::Instance(); 00093 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00094 } 00095 00096 int main() 00097 { 00098 eventQueue.call_every(500, blinkCallback); 00099 00100 BLE &ble = BLE::Instance(); 00101 ble.onEventsToProcess(scheduleBleEventsProcessing); 00102 ble.init(bleInitComplete); 00103 00104 eventQueue.dispatch_forever(); 00105 00106 return 0; 00107 }
Generated on Thu Jul 21 2022 20:49:45 by
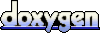