
Library to drive the Zumo shield from pololu.
Dependents: Nucleo_Zumo_BLE_IDB04A1
ZumoShield.h
00001 #include "mbed.h" 00002 00003 /** Zumo Shield Control Class 00004 */ 00005 class ZumoShield { 00006 public: 00007 00008 /** Create a Zumo shield object 00009 * 00010 * @param m1pwm Motor1 pwm pin 00011 * @param m1dir Motor1 direction pin 00012 * @param m2pwm Motor2 pwm pin 00013 * @param m2dir Motor2 direction pin 00014 */ 00015 ZumoShield(PinName m1_pwm_pin, PinName m1_dir_pin, 00016 PinName m2_pwm_pin, PinName m2_dir_pin); 00017 //PinName a0_pin, PinName a1_pin, PinName a2_pin, PinName a3_pin, PinName a4_pin, PinName a5_pin); 00018 00019 /** Switch on the left motor at the given speed. 00020 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00021 */ 00022 void left_motor(float speed); 00023 00024 /** Switch on the right motor at the given speed. 00025 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00026 */ 00027 void right_motor(float speed); 00028 00029 /** Switch on both motors, forwards at the given speed. 00030 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00031 */ 00032 void forward(float speed); 00033 00034 /** Switch on both motors, backwards at the given speed. 00035 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00036 */ 00037 void backward(float speed); 00038 00039 /** Switch on both motors at the given speed, in opposite directions so as to turn left. 00040 * @param speed The speed, from 0.0 to 1.0 at which to spin the motors. 00041 */ 00042 void left(float speed); 00043 00044 /** Switch on both motors at the given speed, in opposite directions so as to turn right. 00045 * @param speed The speed, from 0.0 to 1.0 at which to spin the motors. 00046 */ 00047 void right(float speed); 00048 00049 /** Turns left. 00050 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00051 */ 00052 void turn_left(float speed); 00053 00054 /** Turns right. 00055 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00056 */ 00057 void turn_right(float speed); 00058 00059 /** Stop a chosen motor. 00060 * @param motor Number, either 1 or 2 choosing the motor. 00061 */ 00062 void stop(int motor); 00063 00064 /** Stop left motor. 00065 */ 00066 void stopLeft(); 00067 00068 /** Stop right motor. 00069 */ 00070 void stopRight(); 00071 00072 /** Stop both motors at the same time. Different to disable. 00073 */ 00074 void stopAll(); 00075 00076 /** Gives an indication of the data given by the reflectivity sensors. 00077 */ 00078 //float position(); 00079 00080 private: 00081 PwmOut m1pwm; 00082 PwmOut m2pwm; 00083 DigitalOut m1dir; 00084 DigitalOut m2dir; 00085 /* 00086 AnalogIn a0sens; 00087 AnalogIn a1sens; 00088 AnalogIn a2sens; 00089 AnalogIn a3sens; 00090 AnalogIn a4sens; 00091 AnalogIn a5sens; 00092 */ 00093 };
Generated on Fri Jul 22 2022 05:46:12 by
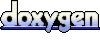