Driver of ST X-NUCLEO-OUT01A1 Industrial Digital output expansion board based on ISO8200BQ component.
Dependents: HelloWorld_OUT01A1
XNucleoOUT01A1.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file XNucleoOUT01A1.cpp 00004 * @author ST CLab 00005 * @version V1.0.0 00006 * @date 1 February 2018 00007 * @brief Implementation of XNucleoOUT01A1 class 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ----------------------------------------------------------------*/ 00039 00040 #include "XNucleoOUT01A1.h" 00041 00042 /* Macros --------------------------------------------------------------------*/ 00043 00044 #define SET_PIN(pin) ((pin != NC) ? new DigitalOut(pin) : NULL) 00045 00046 /* Class Implementation ------------------------------------------------------*/ 00047 00048 /** Constructor 00049 * @param ctl_mode Control mode, direct or sync. 00050 * @param CTL_MODE_PIN Control mode pin, if connected then jumper J4 overrides ctl_mode parameter 00051 * @param OUT_EN Output enable pin. 00052 * @param N_SYNC Input-to-output synchronization signal. Active low. 00053 * @param N_LOAD Load input data signal. Active low. 00054 * @param INx Channel x input pin, NC if not connected 00055 */ 00056 00057 XNucleoOUT01A1::XNucleoOUT01A1(control_mode_t ctl_mode, PinName CTL_MODE_PIN, 00058 PinName OUT_EN, PinName N_SYNC, PinName N_LOAD, PinName IN1, 00059 PinName IN2, PinName IN3, PinName IN4, PinName IN5, PinName IN6, 00060 PinName IN7, PinName IN8) 00061 : iso8200bq(OUT_EN, N_SYNC, N_LOAD) 00062 { 00063 00064 if (CTL_MODE_PIN != NC) { // ctl_mode selected by jumper J4 00065 _ctl_mode_pin = new DigitalIn(CTL_MODE_PIN); 00066 if (*_ctl_mode_pin == 1) { 00067 _mode = mode_sync; 00068 } else { 00069 _mode = mode_direct; 00070 } 00071 } else { // ctl_mode provided by the user 00072 _mode = ctl_mode; 00073 00074 } 00075 00076 if (_mode == mode_direct) { // set the component in direct mode 00077 iso8200bq.direct_mode(); 00078 } 00079 00080 _in_array[0] = SET_PIN(IN1); 00081 _in_array[1] = SET_PIN(IN2); 00082 _in_array[2] = SET_PIN(IN3); 00083 _in_array[3] = SET_PIN(IN4); 00084 _in_array[4] = SET_PIN(IN5); 00085 _in_array[5] = SET_PIN(IN6); 00086 _in_array[6] = SET_PIN(IN7); 00087 _in_array[7] = SET_PIN(IN8); 00088 00089 } 00090 00091 00092 /** 00093 * 00094 * @brief Enable outputs 00095 * 00096 * @param enable Enable outputs when true, disable otherwise. 00097 */ 00098 00099 void XNucleoOUT01A1::enable_outputs(bool enable) { 00100 iso8200bq.enable_outputs(enable); 00101 } 00102 00103 /** 00104 * 00105 * @brief Set input status, also loaded into input buffer when in sync mode 00106 * 00107 * @param input_values Bitmask of input values, bit x represents INx pin's value 00108 */ 00109 void XNucleoOUT01A1::set_inputs(uint8_t input_values) { 00110 00111 if (_mode == mode_sync) { 00112 iso8200bq.sync_mode_load_inputs(); 00113 } 00114 00115 for (int i=0; i<8; i++) { 00116 int value = (input_values >> i) & 0x01; 00117 if (_in_array[i] != NULL) { 00118 *_in_array[i] = value; 00119 } 00120 } 00121 } 00122 00123 /** 00124 * 00125 * @brief Update output status according to input buffer when in sync mode 00126 * 00127 * @param input_values Bitmask of input values, bit x represents INx pin's value 00128 */ 00129 void XNucleoOUT01A1::update_outputs(void) { 00130 00131 if (_mode == mode_sync) { 00132 iso8200bq.sync_mode_update_outputs(); 00133 } 00134 }
Generated on Tue Jul 12 2022 20:17:02 by
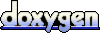