Firmware Library for X-NUCLEO-IKS01A1 (MEMS Inertial & Environmental Sensors) Expansion Board
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: MultiTech_Dragonfly_2015_ATT_Gov_Solutions_Hackathon_Example HelloWorld_IKS01A1 LoRaWAN-test-10secs ServoMotorDemo ... more
Fork of X_NUCLEO_IKS01A1 by
lps25h_class.h
00001 /** 00002 ****************************************************************************** 00003 * @file lps25h_class.h 00004 * @author AST / EST 00005 * @version V0.0.1 00006 * @date 14-April-2015 00007 * @brief Header file for component LPS25H 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __LPS25H_CLASS_H 00039 #define __LPS25H_CLASS_H 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "mbed.h" 00043 #include "DevI2C.h" 00044 #include "lps25h.h" 00045 #include "PressureSensor.h" 00046 #include "TempSensor.h" 00047 00048 /* Classes -------------------------------------------------------------------*/ 00049 /** Class representing a LPS25H sensor component 00050 */ 00051 class LPS25H : public PressureSensor, public TempSensor { 00052 public: 00053 /** Constructor 00054 * @param[in] i2c device I2C to be used for communication 00055 */ 00056 LPS25H(DevI2C &i2c) : PressureSensor(), TempSensor(), dev_i2c(i2c) { 00057 LPS25H_SlaveAddress = LPS25H_ADDRESS_HIGH; 00058 } 00059 00060 /** Destructor 00061 */ 00062 virtual ~LPS25H() {} 00063 00064 /*** Interface Methods ***/ 00065 virtual int init(void *init_struct) { 00066 return LPS25H_Init((PRESSURE_InitTypeDef*)init_struct); 00067 } 00068 00069 /** 00070 * @brief Enter sensor shutdown mode 00071 * @return 0 in case of success, an error code otherwise 00072 */ 00073 virtual int PowerOff(void) { 00074 return LPS25H_PowerOff(); 00075 } 00076 00077 virtual int read_id(uint8_t *p_id) { 00078 return LPS25H_ReadID(p_id); 00079 } 00080 00081 /** 00082 * @brief Reset sensor 00083 * @return 0 in case of success, an error code otherwise 00084 */ 00085 virtual int Reset(void) { 00086 return LPS25H_RebootCmd(); 00087 } 00088 00089 virtual int get_pressure(float *pfData) { 00090 return LPS25H_GetPressure(pfData); 00091 } 00092 00093 virtual int get_temperature(float *pfData) { 00094 return LPS25H_GetTemperature(pfData); 00095 } 00096 00097 void SlaveAddrRemap(uint8_t SA0_Bit_Status) { 00098 LPS25H_SlaveAddrRemap(SA0_Bit_Status); 00099 } 00100 00101 protected: 00102 /*** Methods ***/ 00103 PRESSURE_StatusTypeDef LPS25H_Init(PRESSURE_InitTypeDef *LPS25H_Init); 00104 PRESSURE_StatusTypeDef LPS25H_ReadID(uint8_t *p_id); 00105 PRESSURE_StatusTypeDef LPS25H_RebootCmd(void); 00106 PRESSURE_StatusTypeDef LPS25H_GetPressure(float* pfData); 00107 PRESSURE_StatusTypeDef LPS25H_GetTemperature(float* pfData); 00108 PRESSURE_StatusTypeDef LPS25H_PowerOff(void); 00109 void LPS25H_SlaveAddrRemap(uint8_t SA0_Bit_Status); 00110 00111 PRESSURE_StatusTypeDef LPS25H_PowerOn(void); 00112 PRESSURE_StatusTypeDef LPS25H_I2C_ReadRawPressure(int32_t *raw_press); 00113 PRESSURE_StatusTypeDef LPS25H_I2C_ReadRawTemperature(int16_t *raw_data); 00114 00115 /** 00116 * @brief Configures LPS25H interrupt lines for NUCLEO boards 00117 */ 00118 void LPS25H_IO_ITConfig(void) 00119 { 00120 /* To be implemented */ 00121 } 00122 00123 /** 00124 * @brief Configures LPS25H I2C interface 00125 * @return PRESSURE_OK in case of success, an error code otherwise 00126 */ 00127 PRESSURE_StatusTypeDef LPS25H_IO_Init(void) 00128 { 00129 return PRESSURE_OK; /* done in constructor */ 00130 } 00131 00132 /** 00133 * @brief Utility function to read data from LPS25H 00134 * @param[out] pBuffer pointer to the byte-array to read data in to 00135 * @param[in] RegisterAddr specifies internal address register to read from. 00136 * @param[in] NumByteToRead number of bytes to be read. 00137 * @retval PRESSURE_OK if ok, 00138 * @retval PRESSURE_ERROR if an I2C error has occured 00139 */ 00140 PRESSURE_StatusTypeDef LPS25H_IO_Read(uint8_t* pBuffer, 00141 uint8_t RegisterAddr, uint16_t NumByteToRead) 00142 { 00143 int ret = dev_i2c.i2c_read(pBuffer, 00144 LPS25H_SlaveAddress, 00145 RegisterAddr, 00146 NumByteToRead); 00147 if(ret != 0) { 00148 return PRESSURE_ERROR; 00149 } 00150 return PRESSURE_OK; 00151 } 00152 00153 /** 00154 * @brief Utility function to write data to LPS25H 00155 * @param[in] pBuffer pointer to the byte-array data to send 00156 * @param[in] RegisterAddr specifies internal address register to read from. 00157 * @param[in] NumByteToWrite number of bytes to write. 00158 * @retval PRESSURE_OK if ok, 00159 * @retval PRESSURE_ERROR if an I2C error has occured 00160 */ 00161 PRESSURE_StatusTypeDef LPS25H_IO_Write(uint8_t* pBuffer, 00162 uint8_t RegisterAddr, uint16_t NumByteToWrite) 00163 { 00164 int ret = dev_i2c.i2c_write(pBuffer, 00165 LPS25H_SlaveAddress, 00166 RegisterAddr, 00167 NumByteToWrite); 00168 if(ret != 0) { 00169 return PRESSURE_ERROR; 00170 } 00171 return PRESSURE_OK; 00172 } 00173 00174 /*** Instance Variables ***/ 00175 /* IO Device */ 00176 DevI2C &dev_i2c; 00177 00178 uint8_t LPS25H_SlaveAddress; 00179 }; 00180 00181 #endif // __LPS25H_CLASS_H
Generated on Tue Jul 12 2022 17:19:45 by
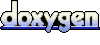