Firmware Library for X-NUCLEO-IKS01A1 (MEMS Inertial & Environmental Sensors) Expansion Board
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: MultiTech_Dragonfly_2015_ATT_Gov_Solutions_Hackathon_Example HelloWorld_IKS01A1 LoRaWAN-test-10secs ServoMotorDemo ... more
Fork of X_NUCLEO_IKS01A1 by
hts221.h
00001 /** 00002 ****************************************************************************** 00003 * @file hts221.h 00004 * @author MEMS Application Team 00005 * @version V1.2.0 00006 * @date 11-February-2015 00007 * @brief This file contains definitions for the hts221.c 00008 * firmware driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __HTS221_H 00042 #define __HTS221_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "hum_temp.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup Components 00056 * @{ 00057 */ 00058 00059 /** @addtogroup HTS221 00060 * @{ 00061 */ 00062 00063 /** @defgroup HTS221_Exported_Defines HTS221_Exported_Defines 00064 * @{ 00065 */ 00066 #ifndef NULL 00067 #define NULL (void *) 0 00068 #endif 00069 00070 /** 00071 * @brief Device Address 00072 */ 00073 #define HTS221_ADDRESS 0xBE 00074 00075 /******************************************************************************/ 00076 /*************************** START REGISTER MAPPING **************************/ 00077 /******************************************************************************/ 00078 00079 00080 /** 00081 * @brief Device identification register. 00082 * \code 00083 * Read 00084 * Default value: 0xBC 00085 * 7:0 This read-only register contains the device identifier that, for HTS221, is set to BCh. 00086 * \endcode 00087 */ 00088 #define HTS221_WHO_AM_I_ADDR 0x0F 00089 00090 00091 /** 00092 * @brief Humidity resolution Register 00093 * \code 00094 * Read/write 00095 * Default value: 0x1B 00096 * 7:6 RFU 00097 * 5:3 AVGT2-AVGT0: Temperature internal average. 00098 * AVGT2 | AVGT1 | AVGT0 | Nr. Internal Average 00099 * ------------------------------------------------------ 00100 * 0 | 0 | 0 | 2 00101 * 0 | 0 | 1 | 4 00102 * 0 | 1 | 0 | 8 00103 * 0 | 1 | 1 | 16 00104 * 1 | 0 | 0 | 32 00105 * 1 | 0 | 1 | 64 00106 * 1 | 1 | 0 | 128 00107 * 1 | 1 | 1 | 256 00108 * 00109 * 2:0 AVGH2-AVGH0: Humidity internal average. 00110 * AVGH2 | AVGH1 | AVGH0 | Nr. Internal Average 00111 * ------------------------------------------------------ 00112 * 0 | 0 | 0 | 4 00113 * 0 | 0 | 1 | 8 00114 * 0 | 1 | 0 | 16 00115 * 0 | 1 | 1 | 32 00116 * 1 | 0 | 0 | 64 00117 * 1 | 0 | 1 | 128 00118 * 1 | 1 | 0 | 256 00119 * 1 | 1 | 1 | 512 00120 * 00121 * \endcode 00122 */ 00123 #define HTS221_RES_CONF_ADDR 0x10 00124 00125 00126 /** 00127 * @brief INFO Register (LSB data) 00128 * \code 00129 * Read/write 00130 * Default value: 0x00 00131 * 7:0 INFO7-INFO0: Lower part of the INFO reference 00132 * used for traceability of the sample. 00133 * \endcode 00134 */ 00135 #define HTS221_INFO_L_ADDR 0x1E 00136 00137 00138 /** 00139 * @brief INFO & Calibration Version Register (LSB data) 00140 * \code 00141 * Read/write 00142 * Default value: 0x00 00143 * 7:6 CALVER1:CALVER0 00144 * 5:0 INFO13-INFO8: Higher part of the INFO reference 00145 * used for traceability of the sample. 00146 * \endcode 00147 */ 00148 #define HTS221_INFO_H_ADDR 0x1F 00149 00150 00151 /** 00152 * @brief Humidity sensor control register 1 00153 * \code 00154 * Read/write 00155 * Default value: 0x00 00156 * 7 PD: power down control. 0 - disable; 1 - enable 00157 * 6:3 RFU 00158 * 2 BDU: block data update. 0 - disable; 1 - enable 00159 * 1:0 RFU 00160 * \endcode 00161 */ 00162 00163 #define HTS221_CTRL_REG1_ADDR 0x20 00164 00165 00166 /** 00167 * @brief Humidity sensor control register 2 00168 * \code 00169 * Read/write 00170 * Default value: 0x00 00171 * 7 BOOT: Reboot memory content. 0: normal mode; 1: reboot memory content 00172 * 6:3 Reserved. 00173 * 2 Reserved. 00174 * 1 Reserved. 00175 * 0 ONE_SHOT: One shot enable. 0: waiting for start of conversion; 1: start for a new dataset 00176 * \endcode 00177 */ 00178 #define HTS221_CTRL_REG2_ADDR 0x21 00179 00180 00181 /** 00182 * @brief Humidity sensor control register 3 00183 * \code 00184 * Read/write 00185 * Default value: 0x00 00186 * [7] DRDY_H_L: Data Ready output signal active high, low (0: active high -default;1: active low) 00187 * [6] PP_OD: Push-pull / Open Drain selection on pin 3 (DRDY) (0: push-pull - default; 1: open drain) 00188 * [5:3] Reserved 00189 * [2] DRDY_EN: Data Ready enable (0: Data Ready disabled - default;1: Data Ready signal available on pin 3) 00190 * [1:0] Reserved 00191 * \endcode 00192 */ 00193 #define HTS221_CTRL_REG3_ADDR 0x22 00194 00195 00196 /** 00197 * @brief Status Register 00198 * \code 00199 * Read 00200 * Default value: 0x00 00201 * 7:2 RFU 00202 * 1 H_DA: Humidity data available. 0: new data for Humidity is not yet available; 1: new data for Humidity is available. 00203 * 0 T_DA: Temperature data available. 0: new data for temperature is not yet available; 1: new data for temperature is available. 00204 * \endcode 00205 */ 00206 #define HTS221_STATUS_REG_ADDR 0x27 00207 00208 00209 /** 00210 * @brief Humidity data (LSB). 00211 * \code 00212 * Read 00213 * Default value: 0x00. 00214 * POUT7 - POUT0: Humidity data LSB (2's complement) => signed 16 bits 00215 * RAW Humidity output data: Hout(%)=(HUMIDITY_OUT_H & HUMIDITY_OUT_L). 00216 * \endcode 00217 */ 00218 #define HTS221_HUMIDITY_OUT_L_ADDR 0x28 00219 00220 00221 /** 00222 * @brief Humidity data (MSB). 00223 * \code 00224 * Read 00225 * Default value: 0x00. 00226 * POUT7 - POUT0: Humidity data LSB (2's complement) => signed 16 bits 00227 * RAW Humidity output data: Hout(%)=(HUMIDITY_OUT_H & HUMIDITY_OUT_L). 00228 * \endcode 00229 */ 00230 #define HTS221_HUMIDITY_OUT_H_ADDR 0x29 00231 00232 00233 /** 00234 * @brief Temperature data (LSB). 00235 * \code 00236 * Read 00237 * Default value: 0x00. 00238 * TOUT7 - TOUT0: temperature data LSB (2's complement) => signed 16 bits 00239 * RAW Temperature output data: Tout (LSB)=(TEMP_OUT_H & TEMP_OUT_L). 00240 * \endcode 00241 */ 00242 #define HTS221_TEMP_OUT_L_ADDR 0x2A 00243 00244 00245 /** 00246 * @brief Temperature data (MSB). 00247 * \code 00248 * Read 00249 * Default value: 0x00. 00250 * TOUT15 - TOUT8: temperature data MSB (2's complement) => signed 16 bits 00251 * RAW Temperature output data: Tout (LSB)=(TEMP_OUT_H & TEMP_OUT_L). 00252 * \endcode 00253 */ 00254 #define HTS221_TEMP_OUT_H_ADDR 0x2B 00255 00256 00257 /** 00258 *@brief Humidity 0 Register in %RH with sensitivity=2 00259 *\code 00260 * Read 00261 * Value: (Unsigned 8 Bit)/2 00262 *\endcode 00263 */ 00264 #define HTS221_H0_RH_X2_ADDR 0x30 00265 00266 00267 /** 00268 *@brief Humidity 1 Register in %RH with sensitivity=2 00269 *\code 00270 * Read 00271 * Value: (Unsigned 8 Bit)/2 00272 *\endcode 00273 */ 00274 #define HTS221_H1_RH_X2_ADDR 0x31 00275 00276 00277 /** 00278 *@brief Temperature 0 Register in deg with sensitivity=8 00279 *\code 00280 * Read 00281 * Value: (Unsigned 16 Bit)/2 00282 *\endcode 00283 */ 00284 #define HTS221_T0_degC_X8_ADDR 0x32 00285 00286 00287 /** 00288 *@brief Temperature 1 Register in deg with sensitivity=8 00289 *\code 00290 * Read 00291 * Value: (Unsigned 16 Bit)/2 00292 *\endcode 00293 */ 00294 #define HTS221_T1_degC_X8_ADDR 0x33 00295 00296 00297 /** 00298 *@brief Temperature 1/0 MSB Register in deg with sensitivity=8 00299 *\code 00300 * Read 00301 * Value: (Unsigned 16 Bit)/2 00302 * 3:2 T1(9):T1(8) MSB T1_degC_X8 bits 00303 * 1:0 T0(9):T0(8) MSB T0_degC_X8 bits 00304 *\endcode 00305 */ 00306 #define HTS221_T1_T0_MSB_X8_ADDR 0x35 00307 00308 00309 /** 00310 *@brief Humidity LOW CALIBRATION Register 00311 *\code 00312 * Read 00313 * Default value: 0x00. 00314 * H0_T0_TOUT7 - H0_T0_TOUT0: HUMIDITY data lSB (2's complement) => signed 16 bits 00315 *\endcode 00316 */ 00317 #define HTS221_H0_T0_OUT_L_ADDR 0x36 00318 00319 00320 /** 00321 *@brief Humidity LOW CALIBRATION Register 00322 *\code 00323 * Read 00324 * Default value: 0x00. 00325 * H0_T0_TOUT15 - H0_T0_TOUT8: HUMIDITY data mSB (2's complement) => signed 16 bits 00326 *\endcode 00327 */ 00328 #define HTS221_H0_T0_OUT_H_ADDR 0x37 00329 00330 00331 /** 00332 *@brief Humidity HIGH CALIBRATION Register 00333 *\code 00334 * Read 00335 * Default value: 0x00. 00336 * H1_T0_TOUT7 - H1_T0_TOUT0: HUMIDITY data lSB (2's complement) => signed 16 bits 00337 *\endcode 00338 */ 00339 #define HTS221_H1_T0_OUT_L_ADDR 0x3A 00340 00341 00342 /** 00343 *@brief Humidity HIGH CALIBRATION Register 00344 *\code 00345 * Read 00346 * Default value: 0x00. 00347 * H1_T0_TOUT15 - H1_T0_TOUT8: HUMIDITY data mSB (2's complement) => signed 16 bits 00348 *\endcode 00349 */ 00350 #define HTS221_H1_T0_OUT_H_ADDR 0x3B 00351 00352 00353 /** 00354 * @brief Low Calibration Temperature Register (LSB). 00355 * \code 00356 * Read 00357 * Default value: 0x00. 00358 * T0_OUT7 - T0_OUT0: temperature data LSB (2's complement) => signed 16 bits 00359 * RAW LOW Calibration data: T0_OUT (LSB)=(T0_OUT_H & T0_OUT_L). 00360 * \endcode 00361 */ 00362 #define HTS221_T0_OUT_L_ADDR 0x3C 00363 00364 00365 /** 00366 * @brief Low Calibration Temperature Register (MSB) 00367 * \code 00368 * Read 00369 * Default value: 0x00. 00370 * T0_OUT15 - T0_OUT8: temperature data MSB (2's complement) => signed 16 bits 00371 * RAW LOW Calibration data: T0_OUT (LSB)=(T0_OUT_H & T0_OUT_L). 00372 * \endcode 00373 */ 00374 #define HTS221_T0_OUT_H_ADDR 0x3D 00375 00376 00377 /** 00378 * @brief Low Calibration Temperature Register (LSB). 00379 * \code 00380 * Read 00381 * Default value: 0x00. 00382 * T1_OUT7 - T1_OUT0: temperature data LSB (2's complement) => signed 16 bits 00383 * RAW LOW Calibration data: T1_OUT (LSB)=(T1_OUT_H & T1_OUT_L). 00384 * \endcode 00385 */ 00386 #define HTS221_T1_OUT_L_ADDR 0x3E 00387 00388 00389 /** 00390 * @brief Low Calibration Temperature Register (MSB) 00391 * \code 00392 * Read 00393 * Default value: 0x00. 00394 * T1_OUT15 - T1_OUT8: temperature data MSB (2's complement) => signed 16 bits 00395 * RAW LOW Calibration data: T1_OUT (LSB)=(T1_OUT_H & T1_OUT_L). 00396 * \endcode 00397 */ 00398 #define HTS221_T1_OUT_H_ADDR 0x3F 00399 00400 00401 /******************************************************************************/ 00402 /**************************** END REGISTER MAPPING ***************************/ 00403 /******************************************************************************/ 00404 00405 /** 00406 * @brief Multiple Byte. Mask for enabling multiple byte read/write command. 00407 */ 00408 #define HTS221_I2C_MULTIPLEBYTE_CMD ((uint8_t)0x80) 00409 00410 /** 00411 * @brief Device Identifier. Default value of the WHO_AM_I register. 00412 */ 00413 #define I_AM_HTS221 ((uint8_t)0xBC) 00414 00415 00416 /** @defgroup HTS221_Power_Mode_Selection_CTRL_REG1 HTS221_Power_Mode_Selection_CTRL_REG1 00417 * @{ 00418 */ 00419 #define HTS221_MODE_POWERDOWN ((uint8_t)0x00) 00420 #define HTS221_MODE_ACTIVE ((uint8_t)0x80) 00421 00422 #define HTS221_MODE_MASK ((uint8_t)0x80) 00423 /** 00424 * @} 00425 */ 00426 00427 00428 /** @defgroup HTS221_Block_Data_Update_Mode_Selection_CTRL_REG1 HTS221_Block_Data_Update_Mode_Selection_CTRL_REG1 00429 * @{ 00430 */ 00431 #define HTS221_BDU_CONTINUOUS ((uint8_t)0x00) 00432 #define HTS221_BDU_NOT_UNTIL_READING ((uint8_t)0x04) 00433 00434 #define HTS221_BDU_MASK ((uint8_t)0x04) 00435 /** 00436 * @} 00437 */ 00438 00439 /** @defgroup HTS221_Output_Data_Rate_Selection_CTRL_REG1 HTS221_Output_Data_Rate_Selection_CTRL_REG1 00440 * @{ 00441 */ 00442 #define HTS221_ODR_ONE_SHOT ((uint8_t)0x00) /*!< Output Data Rate: H - one shot, T - one shot */ 00443 #define HTS221_ODR_1Hz ((uint8_t)0x01) /*!< Output Data Rate: H - 1Hz, T - 1Hz */ 00444 #define HTS221_ODR_7Hz ((uint8_t)0x02) /*!< Output Data Rate: H - 7Hz, T - 7Hz */ 00445 #define HTS221_ODR_12_5Hz ((uint8_t)0x03) /*!< Output Data Rate: H - 12.5Hz, T - 12.5Hz */ 00446 00447 #define HTS221_ODR_MASK ((uint8_t)0x03) 00448 /** 00449 * @} 00450 */ 00451 00452 00453 /** @defgroup HTS221_Boot_Mode_Selection_CTRL_REG2 HTS221_Boot_Mode_Selection_CTRL_REG2 00454 * @{ 00455 */ 00456 #define HTS221_BOOT_NORMALMODE ((uint8_t)0x00) 00457 #define HTS221_BOOT_REBOOTMEMORY ((uint8_t)0x80) 00458 00459 #define HTS221_BOOT_MASK ((uint8_t)0x80) 00460 /** 00461 * @} 00462 */ 00463 00464 00465 /** @defgroup HTS221_One_Shot_Selection_CTRL_REG2 HTS221_One_Shot_Selection_CTRL_REG2 00466 * @{ 00467 */ 00468 #define HTS221_ONE_SHOT_START ((uint8_t)0x01) 00469 00470 #define HTS221_ONE_SHOT_MASK ((uint8_t)0x01) 00471 /** 00472 * @} 00473 */ 00474 00475 /** @defgroup HTS221_PushPull_OpenDrain_Selection_CTRL_REG3 HTS221_PushPull_OpenDrain_Selection_CTRL_REG3 00476 * @{ 00477 */ 00478 #define HTS221_PP_OD_PUSH_PULL ((uint8_t)0x00) 00479 #define HTS221_PP_OD_OPEN_DRAIN ((uint8_t)0x40) 00480 00481 #define HTS221_PP_OD_MASK ((uint8_t)0x40) 00482 /** 00483 * @} 00484 */ 00485 00486 00487 /** @defgroup HTS221_Data_Ready_Selection_CTRL_REG3 HTS221_Data_Ready_Selection_CTRL_REG3 00488 * @{ 00489 */ 00490 #define HTS221_DRDY_DISABLE ((uint8_t)0x00) 00491 #define HTS221_DRDY_AVAILABLE ((uint8_t)0x04) 00492 00493 #define HTS221_DRDY_MASK ((uint8_t)0x04) 00494 /** 00495 * @} 00496 */ 00497 00498 00499 /** @defgroup HTS221_Humidity_Resolution_Selection_RES_CONF HTS221_Humidity_Resolution_Selection_RES_CONF 00500 * @{ 00501 */ 00502 #define HTS221_H_RES_AVG_4 ((uint8_t)0x00) 00503 #define HTS221_H_RES_AVG_8 ((uint8_t)0x01) 00504 #define HTS221_H_RES_AVG_16 ((uint8_t)0x02) 00505 #define HTS221_H_RES_AVG_32 ((uint8_t)0x03) 00506 #define HTS221_H_RES_AVG_64 ((uint8_t)0x04) 00507 #define HTS221_H_RES_AVG_128 ((uint8_t)0x05) 00508 00509 #define HTS221_H_RES_MASK ((uint8_t)0x07) 00510 /** 00511 * @} 00512 */ 00513 00514 00515 /** @defgroup HTS221_Temperature_Resolution_Selection_RES_CONF HTS221_Temperature_Resolution_Selection_RES_CONF 00516 * @{ 00517 */ 00518 #define HTS221_T_RES_AVG_2 ((uint8_t)0x00) 00519 #define HTS221_T_RES_AVG_4 ((uint8_t)0x08) 00520 #define HTS221_T_RES_AVG_8 ((uint8_t)0x10) 00521 #define HTS221_T_RES_AVG_16 ((uint8_t)0x18) 00522 #define HTS221_T_RES_AVG_32 ((uint8_t)0x20) 00523 #define HTS221_T_RES_AVG_64 ((uint8_t)0x28) 00524 00525 #define HTS221_T_RES_MASK ((uint8_t)0x38) 00526 /** 00527 * @} 00528 */ 00529 00530 00531 /** @defgroup HTS221_Temperature_Humidity_Data_Available_STATUS_REG HTS221_Temperature_Humidity_Data_Available_STATUS_REG 00532 * @{ 00533 */ 00534 #define HTS221_H_DATA_AVAILABLE_MASK ((uint8_t)0x02) 00535 #define HTS221_T_DATA_AVAILABLE_MASK ((uint8_t)0x01) 00536 /** 00537 * @} 00538 */ 00539 00540 /* Data resolution */ 00541 #define HUM_DECIMAL_DIGITS (2) 00542 #define TEMP_DECIMAL_DIGITS (2) 00543 00544 /** 00545 * @} 00546 */ 00547 00548 00549 /** @defgroup HTS221_Imported_Functions HTS221_Imported_Functions 00550 * @{ 00551 */ 00552 /* HUM_TEMP sensor IO functions */ 00553 extern HUM_TEMP_StatusTypeDef HTS221_IO_Init(void); 00554 extern HUM_TEMP_StatusTypeDef HTS221_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, 00555 uint16_t NumByteToWrite); 00556 extern HUM_TEMP_StatusTypeDef HTS221_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, 00557 uint16_t NumByteToRead); 00558 extern void HTS221_IO_ITConfig( void ); 00559 00560 /** 00561 * @} 00562 */ 00563 00564 /* ------------------------------------------------------- */ 00565 /* Here you should declare the internal struct of */ 00566 /* extended features of HTS221. See the example of */ 00567 /* LSM6DS3 in lsm6ds3.h */ 00568 /* ------------------------------------------------------- */ 00569 00570 /** @addtogroup HTS221_Exported_Variables HTS221_Exported_Variables 00571 * @{ 00572 */ 00573 /* HUM_TEMP sensor driver structure */ 00574 extern HUM_TEMP_DrvTypeDef Hts221Drv; 00575 extern HUM_TEMP_DrvExtTypeDef Hts221Drv_ext; 00576 /** 00577 * @} 00578 */ 00579 00580 /** 00581 * @} 00582 */ 00583 00584 /** 00585 * @} 00586 */ 00587 00588 /** 00589 * @} 00590 */ 00591 00592 #ifdef __cplusplus 00593 } 00594 #endif 00595 00596 #endif /* __HTS221_H */ 00597 00598 00599 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:19:45 by
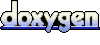