mbed library for STMicroelectronics' X-NUCLEO-IKA01A1 expansion board.
Dependents: HelloWorld_IKA01A1
Fork of X_NUCLEO_IKA01A1 by
XNucleoIKA01A1.h
00001 /** 00002 ****************************************************************************** 00003 * @file XNucleoIKA01A1.h 00004 * @author AST / Software Platforms and Cloud 00005 * @version V1.0 00006 * @date October 1st, 2015 00007 * @brief Class header file for the X_NUCLEO_IKA01A1 expansion board. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 /* Generated with STM32CubeTOO -----------------------------------------------*/ 00040 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 00044 #ifndef __X_NUCLEO_IKA01A1_CLASS_H 00045 #define __X_NUCLEO_IKA01A1_CLASS_H 00046 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 00050 /* ACTION 1 ------------------------------------------------------------------* 00051 * Include here platform specific header files. * 00052 *----------------------------------------------------------------------------*/ 00053 #include "mbed.h" 00054 /* ACTION 2 ------------------------------------------------------------------* 00055 * Include here expansion board configuration's header files. * 00056 *----------------------------------------------------------------------------*/ 00057 #include "XNucleoIKA01A1.h" 00058 /* ACTION 3 ------------------------------------------------------------------* 00059 * Include here expansion board's components' header files. * 00060 * * 00061 * Example: * 00062 * #include "COMPONENT_1.h" * 00063 * #include "COMPONENT_2.h" * 00064 *----------------------------------------------------------------------------*/ 00065 #include "TSZ124.h" 00066 #include "TSU104.h" 00067 #include "TSV734.h" 00068 00069 00070 /* Classes -------------------------------------------------------------------*/ 00071 00072 /** Class XNucleoIKA01A1 represents multifunctional expansion board based on operational amplifiers. 00073 * It provides an easy-to-use and affordable solution for different multifunctional use cases with your 00074 * STM32 Nucleo board. For current sensing configuration and the instrumentation amplifier configuration, 00075 * a highly accurate operational amplifier (TSZ124) is used. The expansion board also contains Nanopower (TSU104) 00076 * and Micropower (TSV734) operational amplifiers for mobile applications. 00077 * It is intentionally implemented as a singleton because only one 00078 * XNucleoIKA01A1 at a time might be deployed in a HW component stack.\n 00079 * In order to get the singleton instance you have to call class method `Instance()`, 00080 * e.g.: 00081 * @code 00082 * // Inertial & Environmental expansion board singleton instance 00083 * static XNucleoIKA01A1 *<TODO>_expansion_board = XNucleoIKA01A1::instance(); 00084 * @endcode 00085 * 00086 */ 00087 class XNucleoIKA01A1 00088 { 00089 public: 00090 00091 /*** Instance, Initialization and Destructor Methods ***/ 00092 00093 /** 00094 * @brief Getting a singleton instance of XNucleoIKA01A1 class. 00095 * @retval a singleton instance of XNucleoIKA01A1 class. 00096 */ 00097 static XNucleoIKA01A1 *instance(PinName instrumentAmpPin, PinName currentSensorPin, PinName photoSensorPin, PinName windCmpSignalPin_1, 00098 PinName windCmpSignalPin_2, PinName ledDriverPin, double ledDriverPeriod_us); 00099 00100 /** 00101 * @brief Initialize the singleton's operational amplifiers to default settings 00102 * @retval true if initialization is successful 00103 * @retval false otherwise. 00104 */ 00105 bool init(void); 00106 00107 /** 00108 * @brief Destructor. 00109 */ 00110 ~XNucleoIKA01A1(void) {} 00111 00112 00113 /*** Public Expansion Board Related Attributes ***/ 00114 00115 /* ACTION 4 --------------------------------------------------------------* 00116 * Declare here a public attribute for each expansion board's component. * 00117 * You will have to call these attributes' public methods within your * 00118 * main program. * 00119 * * 00120 * Example: * 00121 * COMPONENT_1 *component_1; * 00122 * COMPONENT_2 *component_2; * 00123 *------------------------------------------------------------------------*/ 00124 TSZ124 *tsz124; 00125 TSU104 *tsu104; 00126 TSV734 *tsv734; 00127 00128 00129 protected: 00130 00131 /*** Protected Constructor Method ***/ 00132 00133 /** 00134 * @brief Constructor. 00135 */ 00136 XNucleoIKA01A1(PinName instrumentAmpPin, PinName currentSensorPin, PinName photoSensorPin, PinName windCmpSignalPin_1, 00137 PinName windCmpSignalPin_2, PinName ledDriverPin, double ledDriverPeriod_us); 00138 00139 00140 /*** Protected Expansion Board Related Initialization Methods ***/ 00141 00142 /* ACTION 5 --------------------------------------------------------------* 00143 * Declare here a protected initialization method for each expansion * 00144 * board's component. * 00145 * * 00146 * Example: * 00147 * bool init_COMPONENT_1(void); * 00148 * bool init_COMPONENT_2(void); * 00149 *------------------------------------------------------------------------*/ 00150 bool init_TSZ124(void); 00151 bool init_TSU104(void); 00152 bool init_TSV734(void); 00153 00154 00155 /*** Component's Instance Variables ***/ 00156 00157 /* Singleton instance of XNucleoIKA01A1 class. */ 00158 static XNucleoIKA01A1 *_instance; 00159 }; 00160 00161 #endif /* __X_NUCLEO_IKA01A1_CLASS_H */ 00162 00163 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:01:48 by
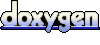