mbed library for STMicroelectronics' X-NUCLEO-IKA01A1 expansion board.
Dependents: HelloWorld_IKA01A1
Fork of X_NUCLEO_IKA01A1 by
XNucleoIKA01A1.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file XNucleoIKA01A1.cpp 00004 * @author AST / Software Platforms and Cloud 00005 * @version V1.0 00006 * @date October 1st, 2015 00007 * @brief Implementation file for the X_NUCLEO_IKA01A1 expansion board. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 /* Generated with STM32CubeTOO -----------------------------------------------*/ 00040 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 00044 /* ACTION 1 ------------------------------------------------------------------* 00045 * Include here platform specific header files. * 00046 *----------------------------------------------------------------------------*/ 00047 #include "mbed.h" 00048 /* ACTION 2 ------------------------------------------------------------------* 00049 * Include here expansion board specific header files. * 00050 *----------------------------------------------------------------------------*/ 00051 #include "XNucleoIKA01A1.h" 00052 00053 00054 /* Variables -----------------------------------------------------------------*/ 00055 00056 /* Singleton instance of XNucleoIKA01A1 class. */ 00057 XNucleoIKA01A1 *XNucleoIKA01A1::_instance; 00058 00059 00060 /* Methods -------------------------------------------------------------------*/ 00061 00062 /** 00063 * @brief Constructor. 00064 */ 00065 XNucleoIKA01A1::XNucleoIKA01A1(PinName instrumentAmpPin, 00066 PinName currentSensorPin, 00067 PinName photoSensorPin, 00068 PinName windCmpSignalPin_1, 00069 PinName windCmpSignalPin_2, 00070 PinName ledDriverPin, 00071 double ledDriverPeriod_us) 00072 { 00073 /* Instantiating the components. */ 00074 /* ACTION 3 --------------------------------------------------------------* 00075 * Instantiate here the expansion board's components. * 00076 * * 00077 * Example: * 00078 * component_1 = new COMPONENT_1(); * 00079 * component_2 = new COMPONENT_2(); * 00080 *------------------------------------------------------------------------*/ 00081 tsz124 = new TSZ124(instrumentAmpPin, currentSensorPin); 00082 tsu104 = new TSU104(photoSensorPin, windCmpSignalPin_1, windCmpSignalPin_2); 00083 tsv734 = new TSV734(ledDriverPin, ledDriverPeriod_us); 00084 } 00085 00086 /** 00087 * @brief Getting a singleton instance of XNucleoIKA01A1 class. 00088 * @retval a singleton instance of XNucleoIKA01A1 class. 00089 */ 00090 XNucleoIKA01A1 *XNucleoIKA01A1::instance(PinName instrumentAmpPin, 00091 PinName currentSensorPin, 00092 PinName photoSensorPin, 00093 PinName windCmpSignalPin_1, 00094 PinName windCmpSignalPin_2, 00095 PinName ledDriverPin, 00096 double ledDriverPeriod_us) 00097 { 00098 if (_instance == NULL) { 00099 /* Instantiating the board. */ 00100 _instance = new XNucleoIKA01A1(instrumentAmpPin, currentSensorPin, photoSensorPin, windCmpSignalPin_1, windCmpSignalPin_2, ledDriverPin, ledDriverPeriod_us); 00101 00102 /* Initializing the components. */ 00103 if (!_instance->init()) { 00104 error("Initialization of the X_NUCLEO_IKA01A1 expansion board failed.\n"); 00105 } 00106 } 00107 00108 return _instance; 00109 } 00110 00111 /** 00112 * @brief Initializing the X_NUCLEO_IKA01A1 board. 00113 * @retval true if initialization is successful 00114 * @retval false otherwise. 00115 */ 00116 bool XNucleoIKA01A1::init(void) 00117 { 00118 /* Initializing the components. */ 00119 /* ACTION 4 --------------------------------------------------------------* 00120 * Initialize here the expansion board's components. * 00121 * * 00122 * Example: * 00123 * return (init_COMPONENT_1() && init_COMPONENT_2()); * 00124 *------------------------------------------------------------------------*/ 00125 //return (init_TSZ124() && init_TSU104() && init_TSV734()); 00126 return true; 00127 } 00128 00129 00130 /** 00131 * @brief Initialize the TSZ124 component. 00132 * @retval true if initialization is successful 00133 * @retval false otherwise 00134 */ 00135 bool XNucleoIKA01A1::init_TSZ124(void) 00136 { 00137 /* Verifying identity. */ 00138 uint8_t id = 0; 00139 int ret = tsz124->read_id(&id); 00140 if ((ret != COMPONENT_OK) || (id != I_AM_TSZ124)) { 00141 delete tsz124; 00142 tsz124 = NULL; 00143 return true; 00144 } 00145 00146 /* Configuration. */ 00147 void* init_structure = NULL; 00148 /* ACTION ----------------------------------------------------------------* 00149 * Configure here the component's initialization structure. * 00150 * * 00151 * Example: * 00152 * init_structure.Property_1 = COMPONENT_PROPERY_1_INIT; * 00153 * init_structure.Property_N = COMPONENT_PROPERY_N_INIT; * 00154 *------------------------------------------------------------------------*/ 00155 00156 /* Initialization. */ 00157 if (tsz124->init(&init_structure) != COMPONENT_OK) { 00158 return false; 00159 } 00160 00161 return true; 00162 } 00163 00164 /** 00165 * @brief Initialize the TSU104 component. 00166 * @retval true if initialization is successful 00167 * @retval false otherwise 00168 */ 00169 bool XNucleoIKA01A1::init_TSU104(void) 00170 { 00171 /* Verifying identity. */ 00172 uint8_t id = 0; 00173 int ret = tsu104->read_id(&id); 00174 if ((ret != COMPONENT_OK) || (id != I_AM_TSU104)) { 00175 delete tsu104; 00176 tsu104 = NULL; 00177 return true; 00178 } 00179 00180 /* Configuration. */ 00181 void* init_structure = NULL; 00182 /* ACTION ----------------------------------------------------------------* 00183 * Configure here the component's initialization structure. * 00184 * * 00185 * Example: * 00186 * init_structure.Property_1 = COMPONENT_PROPERY_1_INIT; * 00187 * init_structure.Property_N = COMPONENT_PROPERY_N_INIT; * 00188 *------------------------------------------------------------------------*/ 00189 00190 /* Initialization. */ 00191 if (tsu104->init(&init_structure) != COMPONENT_OK) { 00192 return false; 00193 } 00194 00195 return true; 00196 } 00197 00198 /** 00199 * @brief Initialize the TSV734 component. 00200 * @retval true if initialization is successful 00201 * @retval false otherwise 00202 */ 00203 bool XNucleoIKA01A1::init_TSV734(void) 00204 { 00205 /* Verifying identity. */ 00206 uint8_t id = 0; 00207 int ret = tsv734->read_id(&id); 00208 if ((ret != COMPONENT_OK) || (id != I_AM_TSV734)) { 00209 delete tsv734; 00210 tsv734 = NULL; 00211 return true; 00212 } 00213 00214 /* Configuration. */ 00215 void* init_structure = NULL; 00216 /* ACTION ----------------------------------------------------------------* 00217 * Configure here the component's initialization structure. * 00218 * * 00219 * Example: * 00220 * init_structure.Property_1 = COMPONENT_PROPERY_1_INIT; * 00221 * init_structure.Property_N = COMPONENT_PROPERY_N_INIT; * 00222 *------------------------------------------------------------------------*/ 00223 00224 /* Initialization. */ 00225 if (tsv734->init(&init_structure) != COMPONENT_OK) { 00226 return false; 00227 } 00228 00229 return true; 00230 } 00231 00232 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:01:48 by
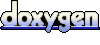