mbed library for STMicroelectronics' X-NUCLEO-IKA01A1 expansion board.
Dependents: HelloWorld_IKA01A1
Fork of X_NUCLEO_IKA01A1 by
TSV734.h
00001 /** 00002 ****************************************************************************** 00003 * @file TSV734.h 00004 * @author Central Labs 00005 * @version 1.0.0 00006 * @date 11-February-2016 00007 * @brief Portable architecture for TSV734 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 /* Generated with STM32CubeTOO -----------------------------------------------*/ 00040 00041 00042 /* Revision ------------------------------------------------------------------*/ 00043 /* 00044 Repository: http://svn.x-nucleodev.codex.cro.st.com/svnroot/X-NucleoDev 00045 Branch/Trunk/Tag: trunk 00046 Based on: X-CUBE-IKA01A1/trunk/Drivers/BSP/Components/tsv734/tsv734.h 00047 Revision: 402 00048 */ 00049 00050 00051 /* Define to prevent recursive inclusion -------------------------------------*/ 00052 00053 #ifndef __TSV734_CLASS_H 00054 #define __TSV734_CLASS_H 00055 00056 00057 /* Includes ------------------------------------------------------------------*/ 00058 00059 /* ACTION 1 ------------------------------------------------------------------* 00060 * Include here platform specific header files. * 00061 *----------------------------------------------------------------------------*/ 00062 #include "mbed.h" 00063 /* ACTION 2 ------------------------------------------------------------------* 00064 * Include here component specific header files. * 00065 *----------------------------------------------------------------------------*/ 00066 #include "TSV734_def.h" 00067 /* ACTION 3 ------------------------------------------------------------------* 00068 * Include here interface specific header files. * 00069 * * 00070 * Example: * 00071 * #include "HumiditySensor.h" * 00072 * #include "TemperatureSensor.h" * 00073 *----------------------------------------------------------------------------*/ 00074 #include "../Interfaces/LedDriver.h" 00075 00076 00077 /* Classes -------------------------------------------------------------------*/ 00078 00079 /** 00080 * @brief Class representing a TSV734 operational amplifier component. 00081 */ 00082 class TSV734 : public LedDriver 00083 { 00084 public: 00085 00086 /*** Constructor and Destructor Methods ***/ 00087 00088 /** 00089 * @brief Constructor. 00090 */ 00091 TSV734(PinName ledDriverPin, double ledPeriod) : LedDriver() 00092 { 00093 led_driver_pin = ledDriverPin; 00094 00095 period_us = ledPeriod; 00096 PwmOut ledOut(led_driver_pin); 00097 ledOut.period_us(period_us); 00098 } 00099 00100 /** 00101 * @brief Destructor. 00102 */ 00103 virtual ~TSV734(void) {} 00104 00105 00106 /*** Public Component Related Methods ***/ 00107 00108 /* ACTION 5 --------------------------------------------------------------* 00109 * Implement here the component's public methods, as wrappers of the C * 00110 * component's functions. * 00111 * They should be: * 00112 * + Methods with the same name of the C component's virtual table's * 00113 * functions (1); * 00114 * + Methods with the same name of the C component's extended virtual * 00115 * table's functions, if any (2). * 00116 * * 00117 * Example: * 00118 * virtual int get_value(float *p_data) //(1) * 00119 * { * 00120 * return COMPONENT_get_value(float *pf_data); * 00121 * } * 00122 * * 00123 * virtual int enable_feature(void) //(2) * 00124 * { * 00125 * return COMPONENT_enable_feature(); * 00126 * } * 00127 *------------------------------------------------------------------------*/ 00128 00129 /** 00130 * @brief Public functions inherited from the Component Class 00131 */ 00132 00133 /** 00134 * @brief initialize the class for TSV734 operational amplifier component 00135 * @retval COMPONENT_OK if initialization is successfull 00136 * @retval suitable error code otherwise 00137 */ 00138 virtual int init(void *init = NULL) 00139 { 00140 return (int) TSV734_Init((void *) init); 00141 } 00142 00143 /** 00144 * @brief obtain component ID for TSV734 perational amplifier 00145 * @retval component ID for TSV734 operational amplifier 00146 */ 00147 virtual int read_id(uint8_t *id = NULL) 00148 { 00149 return (int) TSV734_ReadID((uint8_t *) id); 00150 } 00151 00152 /** 00153 * @brief Public functions inherited from the LedDriver Class 00154 */ 00155 00156 /** 00157 * @brief set the duty cycle of LED in LED driver configuration 00158 * @param dc duty cycle to set 00159 * @retval duty cycle in LED driver configuration 00160 */ 00161 virtual double set_duty_cycle(double dc) 00162 { 00163 PwmOut ledOut(led_driver_pin); 00164 ledOut.pulsewidth_us(period_us*dc); 00165 return (double) dc; 00166 } 00167 00168 00169 /*** Public Interrupt Related Methods ***/ 00170 00171 /* ACTION 6 --------------------------------------------------------------* 00172 * Implement here interrupt related methods, if any. * 00173 * Note that interrupt handling is platform dependent, e.g.: * 00174 * + mbed: * 00175 * InterruptIn feature_irq(pin); //Interrupt object. * 00176 * feature_irq.fall(callback); //Attach a callback. * 00177 * feature_irq.mode(PullNone); //Set interrupt mode. * 00178 * feature_irq.enable_irq(); //Enable interrupt. * 00179 * feature_irq.disable_irq(); //Disable interrupt. * 00180 * + Arduino: * 00181 * attachInterrupt(pin, callback, RISING); //Attach a callback. * 00182 * detachInterrupt(pin); //Detach a callback. * 00183 * * 00184 * Example (mbed): * 00185 * void attach_feature_irq(void (*fptr) (void)) * 00186 * { * 00187 * feature_irq.rise(fptr); * 00188 * } * 00189 * * 00190 * void enable_feature_irq(void) * 00191 * { * 00192 * feature_irq.enable_irq(); * 00193 * } * 00194 * * 00195 * void disable_feature_irq(void) * 00196 * { * 00197 * feature_irq.disable_irq(); * 00198 * } * 00199 *------------------------------------------------------------------------*/ 00200 00201 00202 protected: 00203 00204 /*** Protected Component Related Methods ***/ 00205 00206 /* ACTION 7 --------------------------------------------------------------* 00207 * Declare here the component's specific methods. * 00208 * They should be: * 00209 * + Methods with the same name of the C component's virtual table's * 00210 * functions (1); * 00211 * + Methods with the same name of the C component's extended virtual * 00212 * table's functions, if any (2); * 00213 * + Helper methods, if any, like functions declared in the component's * 00214 * source files but not pointed by the component's virtual table (3). * 00215 * * 00216 * Example: * 00217 * status_t COMPONENT_get_value(float *f); //(1) * 00218 * status_t COMPONENT_enable_feature(void); //(2) * 00219 * status_t COMPONENT_compute_average(void); //(3) * 00220 *------------------------------------------------------------------------*/ 00221 /* TSV734's generic functions. */ 00222 status_t TSV734_Init(void *init); 00223 status_t TSV734_ReadID(void *id); 00224 00225 /* TSV734's interrupts related functions. */ 00226 status_t TSV734_ConfigIT(void* a); 00227 status_t TSV734_SetDutyCycle(float dutyCycle); 00228 double period_us; 00229 PinName led_driver_pin; 00230 00231 00232 /*** Component's I/O Methods ***/ 00233 00234 /*** Component's Instance Variables ***/ 00235 00236 /* ACTION 9 --------------------------------------------------------------* 00237 * Declare here interrupt related variables, if needed. * 00238 * Note that interrupt handling is platform dependent, see * 00239 * "Interrupt Related Methods" above. * 00240 * * 00241 * Example: * 00242 * + mbed: * 00243 * InterruptIn feature_irq; * 00244 *------------------------------------------------------------------------*/ 00245 00246 /* ACTION 10 -------------------------------------------------------------* 00247 * Declare here other pin related variables, if needed. * 00248 * * 00249 * Example: * 00250 * + mbed: * 00251 * DigitalOut standby_reset; * 00252 *------------------------------------------------------------------------*/ 00253 00254 /* ACTION 11 -------------------------------------------------------------* 00255 * Declare here communication related variables, if needed. * 00256 * * 00257 * Example: * 00258 * + mbed: * 00259 * DigitalOut address; * 00260 * DevI2C &dev_i2c; * 00261 *------------------------------------------------------------------------*/ 00262 00263 /* ACTION 12 -------------------------------------------------------------* 00264 * Declare here identity related variables, if needed. * 00265 * Note that there should be only a unique identifier for each component, * 00266 * which should be the "who_am_i" parameter. * 00267 *------------------------------------------------------------------------*/ 00268 /* Identity */ 00269 uint8_t who_am_i; 00270 00271 00272 /* ACTION 13 -------------------------------------------------------------* 00273 * Declare here the component's static and non-static data, one variable * 00274 * per line. * 00275 * * 00276 * Example: * 00277 * float measure; * 00278 * int instance_id; * 00279 * static int number_of_instances; * 00280 *------------------------------------------------------------------------*/ 00281 }; 00282 00283 #endif /* __TSV734_CLASS_H */ 00284 00285 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:01:48 by
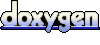