iNEMO inertial module: 3D accelerometer and 3D gyroscope.
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
LSM6DSOXSensor.h
00001 /** 00002 ****************************************************************************** 00003 * @file LSM6DSOXSensor.h 00004 * @author SRA 00005 * @version V1.0.0 00006 * @date February 2019 00007 * @brief Abstract Class of an LSM6DSOX Inertial Measurement Unit (IMU) 6 axes 00008 * sensor. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2019 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Prevent recursive inclusion -----------------------------------------------*/ 00041 00042 #ifndef __LSM6DSOXSensor_H__ 00043 #define __LSM6DSOXSensor_H__ 00044 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "DevI2C.h" 00048 #include "lsm6dsox_reg.h" 00049 #include "MotionSensor.h" 00050 #include "GyroSensor.h" 00051 #include "mbed.h" 00052 #include <assert.h> 00053 00054 /* Defines -------------------------------------------------------------------*/ 00055 00056 #define LSM6DSOX_ACC_SENSITIVITY_FS_2G 0.061f 00057 #define LSM6DSOX_ACC_SENSITIVITY_FS_4G 0.122f 00058 #define LSM6DSOX_ACC_SENSITIVITY_FS_8G 0.244f 00059 #define LSM6DSOX_ACC_SENSITIVITY_FS_16G 0.488f 00060 00061 #define LSM6DSOX_GYRO_SENSITIVITY_FS_125DPS 4.375f 00062 #define LSM6DSOX_GYRO_SENSITIVITY_FS_250DPS 8.750f 00063 #define LSM6DSOX_GYRO_SENSITIVITY_FS_500DPS 17.500f 00064 #define LSM6DSOX_GYRO_SENSITIVITY_FS_1000DPS 35.000f 00065 #define LSM6DSOX_GYRO_SENSITIVITY_FS_2000DPS 70.000f 00066 00067 00068 /* Typedefs ------------------------------------------------------------------*/ 00069 00070 typedef enum 00071 { 00072 LSM6DSOX_INT1_PIN, 00073 LSM6DSOX_INT2_PIN, 00074 } LSM6DSOX_Interrupt_Pin_t; 00075 00076 typedef enum 00077 { 00078 LSM6DSOX_ACC_HIGH_PERFORMANCE_MODE, 00079 LSM6DSOX_ACC_LOW_POWER_NORMAL_MODE, 00080 LSM6DSOX_ACC_ULTRA_LOW_POWER_MODE 00081 } LSM6DSOX_ACC_Operating_Mode_t; 00082 00083 typedef enum 00084 { 00085 LSM6DSOX_GYRO_HIGH_PERFORMANCE_MODE, 00086 LSM6DSOX_GYRO_LOW_POWER_NORMAL_MODE 00087 } LSM6DSOX_GYRO_Operating_Mode_t; 00088 00089 typedef struct 00090 { 00091 unsigned int FreeFallStatus : 1; 00092 unsigned int TapStatus : 1; 00093 unsigned int DoubleTapStatus : 1; 00094 unsigned int WakeUpStatus : 1; 00095 unsigned int StepStatus : 1; 00096 unsigned int TiltStatus : 1; 00097 unsigned int D6DOrientationStatus : 1; 00098 unsigned int SleepStatus : 1; 00099 } LSM6DSOX_Event_Status_t; 00100 00101 typedef struct { 00102 unsigned int is_mlc1 : 1; 00103 unsigned int is_mlc2 : 1; 00104 unsigned int is_mlc3 : 1; 00105 unsigned int is_mlc4 : 1; 00106 unsigned int is_mlc5 : 1; 00107 unsigned int is_mlc6 : 1; 00108 unsigned int is_mlc7 : 1; 00109 unsigned int is_mlc8 : 1; 00110 } LSM6DSOX_MLC_Status_t; 00111 00112 00113 /* Class Declaration ---------------------------------------------------------*/ 00114 00115 /** 00116 * Abstract class of an LSM6DSOX Inertial Measurement Unit (IMU) 6 axes 00117 * sensor. 00118 */ 00119 class LSM6DSOXSensor : public MotionSensor, public GyroSensor 00120 { 00121 public: 00122 enum SPI_type_t {SPI3W, SPI4W}; 00123 LSM6DSOXSensor(SPI *spi, PinName cs_pin, PinName int1_pin=NC, PinName int2_pin=NC, SPI_type_t spi_type=SPI4W); 00124 LSM6DSOXSensor(DevI2C *i2c, uint8_t address=LSM6DSOX_I2C_ADD_H, PinName int1_pin=NC, PinName int2_pin=NC); 00125 virtual int init(void *init); 00126 virtual int read_id(uint8_t *id); 00127 virtual int get_x_axes(int32_t *acceleration); 00128 virtual int get_g_axes(int32_t *angular_rate); 00129 virtual int get_x_sensitivity(float *sensitivity); 00130 virtual int get_g_sensitivity(float *sensitivity); 00131 virtual int get_x_axes_raw(int16_t *value); 00132 virtual int get_g_axes_raw(int16_t *value); 00133 virtual int get_x_odr(float *odr); 00134 virtual int get_g_odr(float *odr); 00135 virtual int set_x_odr(float odr); 00136 virtual int set_x_odr_with_mode(float odr, LSM6DSOX_ACC_Operating_Mode_t mode); 00137 virtual int set_g_odr(float odr); 00138 virtual int set_g_odr_with_mode(float odr, LSM6DSOX_GYRO_Operating_Mode_t mode); 00139 virtual int get_x_fs(float *full_scale); 00140 virtual int get_g_fs(float *full_scale); 00141 virtual int set_x_fs(float full_scale); 00142 virtual int set_g_fs(float full_scale); 00143 int enable_x(void); 00144 int enable_g(void); 00145 int disable_x(void); 00146 int disable_g(void); 00147 int enable_free_fall_detection(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT1_PIN); 00148 int disable_free_fall_detection(void); 00149 int set_free_fall_threshold(uint8_t thr); 00150 int set_free_fall_duration(uint8_t dur); 00151 int enable_pedometer(void); 00152 int disable_pedometer(void); 00153 int get_step_counter(uint16_t *step_count); 00154 int reset_step_counter(void); 00155 int enable_tilt_detection(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT1_PIN); 00156 int disable_tilt_detection(void); 00157 int enable_wake_up_detection(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT2_PIN); 00158 int disable_wake_up_detection(void); 00159 int set_wake_up_threshold(uint8_t thr); 00160 int set_wake_up_duration(uint8_t dur); 00161 int enable_single_tap_detection(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT1_PIN); 00162 int disable_single_tap_detection(void); 00163 int enable_double_tap_detection(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT1_PIN); 00164 int disable_double_tap_detection(void); 00165 int set_tap_threshold(uint8_t thr); 00166 int set_tap_shock_time(uint8_t time); 00167 int set_tap_quiet_time(uint8_t time); 00168 int set_tap_duration_time(uint8_t time); 00169 int enable_6d_orientation(LSM6DSOX_Interrupt_Pin_t pin = LSM6DSOX_INT1_PIN); 00170 int disable_6d_orientation(void); 00171 int set_6d_orientation_threshold(uint8_t thr); 00172 int get_6d_orientation_xl(uint8_t *xl); 00173 int get_6d_orientation_xh(uint8_t *xh); 00174 int get_6d_orientation_yl(uint8_t *yl); 00175 int get_6d_orientation_yh(uint8_t *yh); 00176 int get_6d_orientation_zl(uint8_t *zl); 00177 int get_6d_orientation_zh(uint8_t *zh); 00178 int get_event_status(LSM6DSOX_Event_Status_t *status); 00179 int read_reg(uint8_t reg, uint8_t *data); 00180 int write_reg(uint8_t reg, uint8_t data); 00181 int set_interrupt_latch(uint8_t status); 00182 int get_x_drdy_status(uint8_t *status); 00183 int set_x_self_test(uint8_t status); 00184 int get_g_drdy_status(uint8_t *status); 00185 int set_g_self_test(uint8_t status); 00186 int get_fifo_num_samples(uint16_t *num_samples); 00187 int get_fifo_full_status(uint8_t *status); 00188 int set_fifo_int1_fifo_full(uint8_t status); 00189 int set_fifo_watermark_level(uint16_t watermark); 00190 int set_fifo_stop_on_fth(uint8_t status); 00191 int set_fifo_mode(uint8_t mode); 00192 int get_fifo_tag(uint8_t *tag); 00193 int get_fifo_data(uint8_t *data); 00194 int get_fifo_x_axes(int32_t *acceleration); 00195 int set_fifo_x_bdr(float bdr); 00196 int get_fifo_g_axes(int32_t *angular_velocity); 00197 int set_fifo_g_bdr(float bdr); 00198 int get_mlc_status(LSM6DSOX_MLC_Status_t *status); 00199 int get_mlc_output(uint8_t *output); 00200 00201 /** 00202 * @brief Attaching an interrupt handler to the INT1 interrupt. 00203 * @param fptr An interrupt handler. 00204 * @retval None. 00205 */ 00206 void attach_int1_irq(void (*fptr)(void)) 00207 { 00208 _int1_irq.rise(fptr); 00209 } 00210 00211 /** 00212 * @brief Enabling the INT1 interrupt handling. 00213 * @param None. 00214 * @retval None. 00215 */ 00216 void enable_int1_irq(void) 00217 { 00218 _int1_irq.enable_irq(); 00219 } 00220 00221 /** 00222 * @brief Disabling the INT1 interrupt handling. 00223 * @param None. 00224 * @retval None. 00225 */ 00226 void disable_int1_irq(void) 00227 { 00228 _int1_irq.disable_irq(); 00229 } 00230 00231 /** 00232 * @brief Attaching an interrupt handler to the INT2 interrupt. 00233 * @param fptr An interrupt handler. 00234 * @retval None. 00235 */ 00236 void attach_int2_irq(void (*fptr)(void)) 00237 { 00238 _int2_irq.rise(fptr); 00239 } 00240 00241 /** 00242 * @brief Enabling the INT2 interrupt handling. 00243 * @param None. 00244 * @retval None. 00245 */ 00246 void enable_int2_irq(void) 00247 { 00248 _int2_irq.enable_irq(); 00249 } 00250 00251 /** 00252 * @brief Disabling the INT2 interrupt handling. 00253 * @param None. 00254 * @retval None. 00255 */ 00256 void disable_int2_irq(void) 00257 { 00258 _int2_irq.disable_irq(); 00259 } 00260 00261 /** 00262 * @brief Utility function to read data. 00263 * @param pBuffer: pointer to data to be read. 00264 * @param RegisterAddr: specifies internal address register to be read. 00265 * @param NumByteToRead: number of bytes to be read. 00266 * @retval 0 if ok, an error code otherwise. 00267 */ 00268 uint8_t io_read(uint8_t* pBuffer, uint8_t RegisterAddr, uint16_t NumByteToRead) 00269 { 00270 if (_dev_spi) { 00271 /* Write Reg Address */ 00272 _dev_spi->lock(); 00273 _cs_pin = 0; 00274 if (_spi_type == SPI4W) { 00275 _dev_spi->write(RegisterAddr | 0x80); 00276 for (int i=0; i<NumByteToRead; i++) { 00277 *(pBuffer+i) = _dev_spi->write(0x00); 00278 } 00279 } else if (_spi_type == SPI3W){ 00280 /* Write RD Reg Address with RD bit*/ 00281 uint8_t TxByte = RegisterAddr | 0x80; 00282 _dev_spi->write((char *)&TxByte, 1, (char *)pBuffer, (int) NumByteToRead); 00283 } 00284 _cs_pin = 1; 00285 _dev_spi->unlock(); 00286 return 0; 00287 } 00288 if (_dev_i2c) return (uint8_t) _dev_i2c->i2c_read(pBuffer, _address, RegisterAddr, NumByteToRead); 00289 return 1; 00290 } 00291 00292 /** 00293 * @brief Utility function to write data. 00294 * @param pBuffer: pointer to data to be written. 00295 * @param RegisterAddr: specifies internal address register to be written. 00296 * @param NumByteToWrite: number of bytes to write. 00297 * @retval 0 if ok, an error code otherwise. 00298 */ 00299 uint8_t io_write(uint8_t* pBuffer, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00300 { 00301 if (_dev_spi) { 00302 _dev_spi->lock(); 00303 _cs_pin = 0; 00304 _dev_spi->write(RegisterAddr); 00305 _dev_spi->write((char *)pBuffer, (int) NumByteToWrite, NULL, 0); 00306 _cs_pin = 1; 00307 _dev_spi->unlock(); 00308 return 0; 00309 } 00310 if (_dev_i2c) return (uint8_t) _dev_i2c->i2c_write(pBuffer, _address, RegisterAddr, NumByteToWrite); 00311 return 1; 00312 } 00313 00314 private: 00315 int set_x_odr_when_enabled(float odr); 00316 int set_g_odr_when_enabled(float odr); 00317 int set_x_odr_when_disabled(float odr); 00318 int set_g_odr_when_disabled(float odr); 00319 00320 /* Helper classes. */ 00321 DevI2C *_dev_i2c; 00322 SPI *_dev_spi; 00323 00324 /* Configuration */ 00325 uint8_t _address; 00326 DigitalOut _cs_pin; 00327 InterruptIn _int1_irq; 00328 InterruptIn _int2_irq; 00329 SPI_type_t _spi_type; 00330 00331 uint8_t _x_is_enabled; 00332 lsm6dsox_odr_xl_t _x_last_odr; 00333 uint8_t _g_is_enabled; 00334 lsm6dsox_odr_g_t _g_last_odr; 00335 00336 lsm6dsox_ctx_t _reg_ctx; 00337 }; 00338 00339 #ifdef __cplusplus 00340 extern "C" { 00341 #endif 00342 int32_t LSM6DSOX_io_write( void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite ); 00343 int32_t LSM6DSOX_io_read( void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead ); 00344 #ifdef __cplusplus 00345 } 00346 #endif 00347 00348 #endif
Generated on Tue Jul 12 2022 20:15:23 by
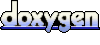