3-axis MEMS ultra low power accelerometer
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: X_NUCLEO_IKS01A3 X_NUCLEO_IKS01A3
lis2dw12_reg.c
00001 /** 00002 ****************************************************************************** 00003 * @file lis2dw12_reg.c 00004 * @author Sensors Software Solution Team 00005 * @brief LIS2DW12 driver file 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2018 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without 00012 * modification, are permitted provided that the following conditions 00013 * are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright 00017 * notice, this list of conditions and the following disclaimer in the 00018 * documentation and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its 00020 * contributors may be used to endorse or promote products derived from 00021 * this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00026 * ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE 00027 * LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00028 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00029 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00030 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00031 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00032 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00033 * POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #include "lis2dw12_reg.h" 00039 00040 /** 00041 * @defgroup LIS2DW12 00042 * @brief This file provides a set of functions needed to drive the 00043 * lis2dw12 enhanced inertial module. 00044 * @{ 00045 * 00046 */ 00047 00048 /** 00049 * @defgroup LIS2DW12_Interfaces_Functions 00050 * @brief This section provide a set of functions used to read and 00051 * write a generic register of the device. 00052 * MANDATORY: return 0 -> no Error. 00053 * @{ 00054 * 00055 */ 00056 00057 /** 00058 * @brief Read generic device register 00059 * 00060 * @param ctx read / write interface definitions(ptr) 00061 * @param reg register to read 00062 * @param data pointer to buffer that store the data read(ptr) 00063 * @param len number of consecutive register to read 00064 * @retval interface status (MANDATORY: return 0 -> no Error) 00065 * 00066 */ 00067 int32_t lis2dw12_read_reg(lis2dw12_ctx_t *ctx, uint8_t reg, uint8_t *data, 00068 uint16_t len) 00069 { 00070 int32_t ret; 00071 ret = ctx->read_reg(ctx->handle, reg, data, len); 00072 return ret; 00073 } 00074 00075 /** 00076 * @brief Write generic device register 00077 * 00078 * @param ctx read / write interface definitions(ptr) 00079 * @param reg register to write 00080 * @param data pointer to data to write in register reg(ptr) 00081 * @param len number of consecutive register to write 00082 * @retval interface status (MANDATORY: return 0 -> no Error) 00083 * 00084 */ 00085 int32_t lis2dw12_write_reg(lis2dw12_ctx_t *ctx, uint8_t reg, uint8_t *data, 00086 uint16_t len) 00087 { 00088 int32_t ret; 00089 ret = ctx->write_reg(ctx->handle, reg, data, len); 00090 return ret; 00091 } 00092 00093 /** 00094 * @} 00095 * 00096 */ 00097 00098 /** 00099 * @defgroup LIS2DW12_Sensitivity 00100 * @brief These functions convert raw-data into engineering units. 00101 * @{ 00102 * 00103 */ 00104 00105 float lis2dw12_from_fs2_to_mg(int16_t lsb) 00106 { 00107 return ((float)lsb) * 0.061f; 00108 } 00109 00110 float lis2dw12_from_fs4_to_mg(int16_t lsb) 00111 { 00112 return ((float)lsb) * 0.122f; 00113 } 00114 00115 float lis2dw12_from_fs8_to_mg(int16_t lsb) 00116 { 00117 return ((float)lsb) * 0.244f; 00118 } 00119 00120 float lis2dw12_from_fs16_to_mg(int16_t lsb) 00121 { 00122 return ((float)lsb) * 0.488f; 00123 } 00124 00125 float lis2dw12_from_fs2_lp1_to_mg(int16_t lsb) 00126 { 00127 return ((float)lsb) * 0.061f; 00128 } 00129 00130 float lis2dw12_from_fs4_lp1_to_mg(int16_t lsb) 00131 { 00132 return ((float)lsb) * 0.122f; 00133 } 00134 00135 float lis2dw12_from_fs8_lp1_to_mg(int16_t lsb) 00136 { 00137 return ((float)lsb) * 0.244f; 00138 } 00139 00140 float lis2dw12_from_fs16_lp1_to_mg(int16_t lsb) 00141 { 00142 return ((float)lsb) * 0.488f; 00143 } 00144 00145 float lis2dw12_from_lsb_to_celsius(int16_t lsb) 00146 { 00147 return (((float)lsb / 16.0f) + 25.0f); 00148 } 00149 00150 /** 00151 * @} 00152 * 00153 */ 00154 00155 /** 00156 * @defgroup LIS2DW12_Data_Generation 00157 * @brief This section groups all the functions concerning 00158 * data generation 00159 * @{ 00160 * 00161 */ 00162 00163 /** 00164 * @brief Select accelerometer operating modes.[set] 00165 * 00166 * @param ctx read / write interface definitions 00167 * @param val change the values of mode / lp_mode in reg CTRL1 00168 * and low_noise in reg CTRL6 00169 * @retval interface status (MANDATORY: return 0 -> no Error) 00170 * 00171 */ 00172 int32_t lis2dw12_power_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_mode_t val) 00173 { 00174 lis2dw12_ctrl1_t ctrl1; 00175 lis2dw12_ctrl6_t ctrl6; 00176 int32_t ret; 00177 00178 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00179 if (ret == 0) { 00180 ctrl1.mode = ((uint8_t) val & 0x0CU) >> 2; 00181 ctrl1.lp_mode = (uint8_t) val & 0x03U ; 00182 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00183 } 00184 if (ret == 0) { 00185 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 00186 } 00187 if (ret == 0) { 00188 ctrl6.low_noise = ((uint8_t) val & 0x10U) >> 4; 00189 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 00190 } 00191 return ret; 00192 } 00193 00194 /** 00195 * @brief Select accelerometer operating modes.[get] 00196 * 00197 * @param ctx read / write interface definitions 00198 * @param val change the values of mode / lp_mode in reg CTRL1 00199 * and low_noise in reg CTRL6 00200 * @retval interface status (MANDATORY: return 0 -> no Error) 00201 * 00202 */ 00203 int32_t lis2dw12_power_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_mode_t *val) 00204 { 00205 lis2dw12_ctrl1_t ctrl1; 00206 lis2dw12_ctrl6_t ctrl6; 00207 int32_t ret; 00208 00209 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00210 if (ret == 0) { 00211 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 00212 00213 switch (((ctrl6.low_noise << 4) + (ctrl1.mode << 2) + 00214 ctrl1.lp_mode)) { 00215 case LIS2DW12_HIGH_PERFORMANCE: 00216 *val = LIS2DW12_HIGH_PERFORMANCE; 00217 break; 00218 case LIS2DW12_CONT_LOW_PWR_4: 00219 *val = LIS2DW12_CONT_LOW_PWR_4; 00220 break; 00221 case LIS2DW12_CONT_LOW_PWR_3: 00222 *val = LIS2DW12_CONT_LOW_PWR_3; 00223 break; 00224 case LIS2DW12_CONT_LOW_PWR_2: 00225 *val = LIS2DW12_CONT_LOW_PWR_2; 00226 break; 00227 case LIS2DW12_CONT_LOW_PWR_12bit: 00228 *val = LIS2DW12_CONT_LOW_PWR_12bit; 00229 break; 00230 case LIS2DW12_SINGLE_LOW_PWR_4: 00231 *val = LIS2DW12_SINGLE_LOW_PWR_4; 00232 break; 00233 case LIS2DW12_SINGLE_LOW_PWR_3: 00234 *val = LIS2DW12_SINGLE_LOW_PWR_3; 00235 break; 00236 case LIS2DW12_SINGLE_LOW_PWR_2: 00237 *val = LIS2DW12_SINGLE_LOW_PWR_2; 00238 break; 00239 case LIS2DW12_SINGLE_LOW_PWR_12bit: 00240 *val = LIS2DW12_SINGLE_LOW_PWR_12bit; 00241 break; 00242 case LIS2DW12_HIGH_PERFORMANCE_LOW_NOISE: 00243 *val = LIS2DW12_HIGH_PERFORMANCE_LOW_NOISE; 00244 break; 00245 case LIS2DW12_CONT_LOW_PWR_LOW_NOISE_4: 00246 *val = LIS2DW12_CONT_LOW_PWR_LOW_NOISE_4; 00247 break; 00248 case LIS2DW12_CONT_LOW_PWR_LOW_NOISE_3: 00249 *val = LIS2DW12_CONT_LOW_PWR_LOW_NOISE_3; 00250 break; 00251 case LIS2DW12_CONT_LOW_PWR_LOW_NOISE_2: 00252 *val = LIS2DW12_CONT_LOW_PWR_LOW_NOISE_2; 00253 break; 00254 case LIS2DW12_CONT_LOW_PWR_LOW_NOISE_12bit: 00255 *val = LIS2DW12_CONT_LOW_PWR_LOW_NOISE_12bit; 00256 break; 00257 case LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_4: 00258 *val = LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_4; 00259 break; 00260 case LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_3: 00261 *val = LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_3; 00262 break; 00263 case LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_2: 00264 *val = LIS2DW12_SINGLE_LOW_PWR_LOW_NOISE_2; 00265 break; 00266 case LIS2DW12_SINGLE_LOW_LOW_NOISE_PWR_12bit: 00267 *val = LIS2DW12_SINGLE_LOW_LOW_NOISE_PWR_12bit; 00268 break; 00269 default: 00270 *val = LIS2DW12_HIGH_PERFORMANCE; 00271 break; 00272 } 00273 } 00274 return ret; 00275 } 00276 00277 /** 00278 * @brief Accelerometer data rate selection.[set] 00279 * 00280 * @param ctx read / write interface definitions 00281 * @param val change the values of odr in reg CTRL1 00282 * @retval interface status (MANDATORY: return 0 -> no Error) 00283 * 00284 */ 00285 int32_t lis2dw12_data_rate_set(lis2dw12_ctx_t *ctx, lis2dw12_odr_t val) 00286 { 00287 lis2dw12_ctrl1_t ctrl1; 00288 lis2dw12_ctrl3_t ctrl3; 00289 int32_t ret; 00290 00291 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00292 if (ret == 0) { 00293 ctrl1.odr = (uint8_t) val; 00294 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00295 } 00296 if (ret == 0) { 00297 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) &ctrl3, 1); 00298 } 00299 if (ret == 0) { 00300 ctrl3.slp_mode = ((uint8_t) val & 0x30U) >> 4; 00301 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) &ctrl3, 1); 00302 } 00303 return ret; 00304 } 00305 00306 /** 00307 * @brief Accelerometer data rate selection.[get] 00308 * 00309 * @param ctx read / write interface definitions 00310 * @param val Get the values of odr in reg CTRL1 00311 * @retval interface status (MANDATORY: return 0 -> no Error) 00312 * 00313 */ 00314 int32_t lis2dw12_data_rate_get(lis2dw12_ctx_t *ctx, lis2dw12_odr_t *val) 00315 { 00316 lis2dw12_ctrl1_t ctrl1; 00317 lis2dw12_ctrl3_t ctrl3; 00318 int32_t ret; 00319 00320 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL1, (uint8_t *) &ctrl1, 1); 00321 if (ret == 0) { 00322 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) &ctrl3, 1); 00323 00324 switch ((ctrl3.slp_mode << 4) + ctrl1.odr) { 00325 case LIS2DW12_XL_ODR_OFF: 00326 *val = LIS2DW12_XL_ODR_OFF; 00327 break; 00328 case LIS2DW12_XL_ODR_1Hz6_LP_ONLY: 00329 *val = LIS2DW12_XL_ODR_1Hz6_LP_ONLY; 00330 break; 00331 case LIS2DW12_XL_ODR_12Hz5: 00332 *val = LIS2DW12_XL_ODR_12Hz5; 00333 break; 00334 case LIS2DW12_XL_ODR_25Hz: 00335 *val = LIS2DW12_XL_ODR_25Hz; 00336 break; 00337 case LIS2DW12_XL_ODR_50Hz: 00338 *val = LIS2DW12_XL_ODR_50Hz; 00339 break; 00340 case LIS2DW12_XL_ODR_100Hz: 00341 *val = LIS2DW12_XL_ODR_100Hz; 00342 break; 00343 case LIS2DW12_XL_ODR_200Hz: 00344 *val = LIS2DW12_XL_ODR_200Hz; 00345 break; 00346 case LIS2DW12_XL_ODR_400Hz: 00347 *val = LIS2DW12_XL_ODR_400Hz; 00348 break; 00349 case LIS2DW12_XL_ODR_800Hz: 00350 *val = LIS2DW12_XL_ODR_800Hz; 00351 break; 00352 case LIS2DW12_XL_ODR_1k6Hz: 00353 *val = LIS2DW12_XL_ODR_1k6Hz; 00354 break; 00355 case LIS2DW12_XL_SET_SW_TRIG: 00356 *val = LIS2DW12_XL_SET_SW_TRIG; 00357 break; 00358 case LIS2DW12_XL_SET_PIN_TRIG: 00359 *val = LIS2DW12_XL_SET_PIN_TRIG; 00360 break; 00361 default: 00362 *val = LIS2DW12_XL_ODR_OFF; 00363 break; 00364 } 00365 } 00366 return ret; 00367 } 00368 00369 /** 00370 * @brief Block data update.[set] 00371 * 00372 * @param ctx read / write interface definitions 00373 * @param val change the values of bdu in reg CTRL2 00374 * @retval interface status (MANDATORY: return 0 -> no Error) 00375 * 00376 */ 00377 int32_t lis2dw12_block_data_update_set(lis2dw12_ctx_t *ctx, uint8_t val) 00378 { 00379 lis2dw12_ctrl2_t reg; 00380 int32_t ret; 00381 00382 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00383 if (ret == 0) { 00384 reg.bdu = val; 00385 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00386 } 00387 return ret; 00388 } 00389 00390 /** 00391 * @brief Block data update.[get] 00392 * 00393 * @param ctx read / write interface definitions 00394 * @param val change the values of bdu in reg CTRL2 00395 * @retval interface status (MANDATORY: return 0 -> no Error) 00396 * 00397 */ 00398 int32_t lis2dw12_block_data_update_get(lis2dw12_ctx_t *ctx, uint8_t *val) 00399 { 00400 lis2dw12_ctrl2_t reg; 00401 int32_t ret; 00402 00403 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00404 *val = reg.bdu; 00405 00406 return ret; 00407 } 00408 00409 /** 00410 * @brief Accelerometer full-scale selection.[set] 00411 * 00412 * @param ctx read / write interface definitions 00413 * @param val change the values of fs in reg CTRL6 00414 * @retval interface status (MANDATORY: return 0 -> no Error) 00415 * 00416 */ 00417 int32_t lis2dw12_full_scale_set(lis2dw12_ctx_t *ctx, lis2dw12_fs_t val) 00418 { 00419 lis2dw12_ctrl6_t reg; 00420 int32_t ret; 00421 00422 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 00423 if (ret == 0) { 00424 reg.fs = (uint8_t) val; 00425 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 00426 } 00427 return ret; 00428 } 00429 00430 /** 00431 * @brief Accelerometer full-scale selection.[get] 00432 * 00433 * @param ctx read / write interface definitions 00434 * @param val Get the values of fs in reg CTRL6 00435 * @retval interface status (MANDATORY: return 0 -> no Error) 00436 * 00437 */ 00438 int32_t lis2dw12_full_scale_get(lis2dw12_ctx_t *ctx, lis2dw12_fs_t *val) 00439 { 00440 lis2dw12_ctrl6_t reg; 00441 int32_t ret; 00442 00443 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 00444 00445 switch (reg.fs) { 00446 case LIS2DW12_2g: 00447 *val = LIS2DW12_2g; 00448 break; 00449 case LIS2DW12_4g: 00450 *val = LIS2DW12_4g; 00451 break; 00452 case LIS2DW12_8g: 00453 *val = LIS2DW12_8g; 00454 break; 00455 case LIS2DW12_16g: 00456 *val = LIS2DW12_16g; 00457 break; 00458 default: 00459 *val = LIS2DW12_2g; 00460 break; 00461 } 00462 return ret; 00463 } 00464 00465 /** 00466 * @brief The STATUS_REG register of the device.[get] 00467 * 00468 * @param ctx read / write interface definitions 00469 * @param val union of registers from STATUS to 00470 * @retval interface status (MANDATORY: return 0 -> no Error) 00471 * 00472 */ 00473 int32_t lis2dw12_status_reg_get(lis2dw12_ctx_t *ctx, lis2dw12_status_t *val) 00474 { 00475 int32_t ret; 00476 ret = lis2dw12_read_reg(ctx, LIS2DW12_STATUS, (uint8_t *) val, 1); 00477 return ret; 00478 } 00479 00480 /** 00481 * @brief Accelerometer new data available.[get] 00482 * 00483 * @param ctx read / write interface definitions 00484 * @param val change the values of drdy in reg STATUS 00485 * @retval interface status (MANDATORY: return 0 -> no Error) 00486 * 00487 */ 00488 int32_t lis2dw12_flag_data_ready_get(lis2dw12_ctx_t *ctx, uint8_t *val) 00489 { 00490 lis2dw12_status_t reg; 00491 int32_t ret; 00492 00493 ret = lis2dw12_read_reg(ctx, LIS2DW12_STATUS, (uint8_t *) ®, 1); 00494 *val = reg.drdy; 00495 00496 return ret; 00497 } 00498 /** 00499 * @brief Read all the interrupt/status flag of the device.[get] 00500 * 00501 * @param ctx read / write interface definitions 00502 * @param val registers STATUS_DUP, WAKE_UP_SRC, 00503 * TAP_SRC, SIXD_SRC, ALL_INT_SRC 00504 * @retval interface status (MANDATORY: return 0 -> no Error) 00505 * 00506 */ 00507 int32_t lis2dw12_all_sources_get(lis2dw12_ctx_t *ctx, 00508 lis2dw12_all_sources_t *val) 00509 { 00510 int32_t ret; 00511 ret = lis2dw12_read_reg(ctx, LIS2DW12_STATUS_DUP, (uint8_t *) val, 5); 00512 return ret; 00513 } 00514 00515 /** 00516 * @brief Accelerometer X-axis user offset correction expressed in two’s 00517 * complement, weight depends on bit USR_OFF_W. The value must be 00518 * in the range [-127 127].[set] 00519 * 00520 * @param ctx read / write interface definitions 00521 * @param buff buffer that contains data to write 00522 * @retval interface status (MANDATORY: return 0 -> no Error) 00523 * 00524 */ 00525 int32_t lis2dw12_usr_offset_x_set(lis2dw12_ctx_t *ctx, uint8_t *buff) 00526 { 00527 int32_t ret; 00528 ret = lis2dw12_write_reg(ctx, LIS2DW12_X_OFS_USR, buff, 1); 00529 return ret; 00530 } 00531 00532 /** 00533 * @brief Accelerometer X-axis user offset correction expressed in two’s 00534 * complement, weight depends on bit USR_OFF_W. The value must be 00535 * in the range [-127 127].[get] 00536 * 00537 * @param ctx read / write interface definitions 00538 * @param buff buffer that stores data read 00539 * @retval interface status (MANDATORY: return 0 -> no Error) 00540 * 00541 */ 00542 int32_t lis2dw12_usr_offset_x_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00543 { 00544 int32_t ret; 00545 ret = lis2dw12_read_reg(ctx, LIS2DW12_X_OFS_USR, buff, 1); 00546 return ret; 00547 } 00548 00549 /** 00550 * @brief Accelerometer Y-axis user offset correction expressed in two’s 00551 * complement, weight depends on bit USR_OFF_W. The value must be 00552 * in the range [-127 127].[set] 00553 * 00554 * @param ctx read / write interface definitions 00555 * @param buff buffer that contains data to write 00556 * @retval interface status (MANDATORY: return 0 -> no Error) 00557 * 00558 */ 00559 int32_t lis2dw12_usr_offset_y_set(lis2dw12_ctx_t *ctx, uint8_t *buff) 00560 { 00561 int32_t ret; 00562 ret = lis2dw12_write_reg(ctx, LIS2DW12_Y_OFS_USR, buff, 1); 00563 return ret; 00564 } 00565 00566 /** 00567 * @brief Accelerometer Y-axis user offset correction expressed in two’s 00568 * complement, weight depends on bit USR_OFF_W. The value must be 00569 * in the range [-127 127].[get] 00570 * 00571 * @param ctx read / write interface definitions 00572 * @param buff buffer that stores data read 00573 * @retval interface status (MANDATORY: return 0 -> no Error) 00574 * 00575 */ 00576 int32_t lis2dw12_usr_offset_y_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00577 { 00578 int32_t ret; 00579 ret = lis2dw12_read_reg(ctx, LIS2DW12_Y_OFS_USR, buff, 1); 00580 return ret; 00581 } 00582 00583 /** 00584 * @brief Accelerometer Z-axis user offset correction expressed in two’s 00585 * complement, weight depends on bit USR_OFF_W. The value must be 00586 * in the range [-127 127].[set] 00587 * 00588 * @param ctx read / write interface definitions 00589 * @param buff buffer that contains data to write 00590 * @retval interface status (MANDATORY: return 0 -> no Error) 00591 * 00592 */ 00593 int32_t lis2dw12_usr_offset_z_set(lis2dw12_ctx_t *ctx, uint8_t *buff) 00594 { 00595 int32_t ret; 00596 ret = lis2dw12_write_reg(ctx, LIS2DW12_Z_OFS_USR, buff, 1); 00597 return ret; 00598 } 00599 00600 /** 00601 * @brief Accelerometer Z-axis user offset correction expressed in two’s 00602 * complement, weight depends on bit USR_OFF_W. The value must be 00603 * in the range [-127 127].[get] 00604 * 00605 * @param ctx read / write interface definitions 00606 * @param buff buffer that stores data read 00607 * @retval interface status (MANDATORY: return 0 -> no Error) 00608 * 00609 */ 00610 int32_t lis2dw12_usr_offset_z_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00611 { 00612 int32_t ret; 00613 ret = lis2dw12_read_reg(ctx, LIS2DW12_Z_OFS_USR, buff, 1); 00614 return ret; 00615 } 00616 00617 /** 00618 * @brief Weight of XL user offset bits of registers X_OFS_USR, 00619 * Y_OFS_USR, Z_OFS_USR.[set] 00620 * 00621 * @param ctx read / write interface definitions 00622 * @param val change the values of usr_off_w in 00623 * reg CTRL_REG7 00624 * @retval interface status (MANDATORY: return 0 -> no Error) 00625 * 00626 */ 00627 int32_t lis2dw12_offset_weight_set(lis2dw12_ctx_t *ctx, 00628 lis2dw12_usr_off_w_t val) 00629 { 00630 lis2dw12_ctrl_reg7_t reg; 00631 int32_t ret; 00632 00633 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00634 if (ret == 0) { 00635 reg.usr_off_w = (uint8_t) val; 00636 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00637 } 00638 return ret; 00639 } 00640 00641 /** 00642 * @brief Weight of XL user offset bits of registers X_OFS_USR, 00643 * Y_OFS_USR, Z_OFS_USR.[get] 00644 * 00645 * @param ctx read / write interface definitions 00646 * @param val Get the values of usr_off_w in reg CTRL_REG7 00647 * @retval interface status (MANDATORY: return 0 -> no Error) 00648 * 00649 */ 00650 int32_t lis2dw12_offset_weight_get(lis2dw12_ctx_t *ctx, 00651 lis2dw12_usr_off_w_t *val) 00652 { 00653 lis2dw12_ctrl_reg7_t reg; 00654 int32_t ret; 00655 00656 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00657 switch (reg.usr_off_w) { 00658 case LIS2DW12_LSb_977ug: 00659 *val = LIS2DW12_LSb_977ug; 00660 break; 00661 case LIS2DW12_LSb_15mg6: 00662 *val = LIS2DW12_LSb_15mg6; 00663 break; 00664 default: 00665 *val = LIS2DW12_LSb_977ug; 00666 break; 00667 } 00668 return ret; 00669 } 00670 00671 /** 00672 * @} 00673 * 00674 */ 00675 00676 /** 00677 * @defgroup LIS2DW12_Data_Output 00678 * @brief This section groups all the data output functions. 00679 * @{ 00680 * 00681 */ 00682 00683 /** 00684 * @brief Temperature data output register (r). L and H registers 00685 * together express a 16-bit word in two’s complement.[get] 00686 * 00687 * @param ctx read / write interface definitions 00688 * @param buff buffer that stores data read 00689 * @retval interface status (MANDATORY: return 0 -> no Error) 00690 * 00691 */ 00692 int32_t lis2dw12_temperature_raw_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00693 { 00694 int32_t ret; 00695 ret = lis2dw12_read_reg(ctx, LIS2DW12_OUT_T_L, buff, 2); 00696 return ret; 00697 } 00698 00699 /** 00700 * @brief Linear acceleration output register. The value is expressed as 00701 * a 16-bit word in two’s complement.[get] 00702 * 00703 * @param ctx read / write interface definitions 00704 * @param buff buffer that stores data read 00705 * @retval interface status (MANDATORY: return 0 -> no Error) 00706 * 00707 */ 00708 int32_t lis2dw12_acceleration_raw_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00709 { 00710 int32_t ret; 00711 ret = lis2dw12_read_reg(ctx, LIS2DW12_OUT_X_L, buff, 6); 00712 return ret; 00713 } 00714 00715 /** 00716 * @} 00717 * 00718 */ 00719 00720 /** 00721 * @defgroup LIS2DW12_Common 00722 * @brief This section groups common useful functions. 00723 * @{ 00724 * 00725 */ 00726 00727 /** 00728 * @brief Device Who am I.[get] 00729 * 00730 * @param ctx read / write interface definitions 00731 * @param buff buffer that stores data read 00732 * @retval interface status (MANDATORY: return 0 -> no Error) 00733 * 00734 */ 00735 int32_t lis2dw12_device_id_get(lis2dw12_ctx_t *ctx, uint8_t *buff) 00736 { 00737 int32_t ret; 00738 ret = lis2dw12_read_reg(ctx, LIS2DW12_WHO_AM_I, buff, 1); 00739 return ret; 00740 } 00741 00742 /** 00743 * @brief Register address automatically incremented during multiple byte 00744 * access with a serial interface.[set] 00745 * 00746 * @param ctx read / write interface definitions 00747 * @param val change the values of if_add_inc in reg CTRL2 00748 * @retval interface status (MANDATORY: return 0 -> no Error) 00749 * 00750 */ 00751 int32_t lis2dw12_auto_increment_set(lis2dw12_ctx_t *ctx, uint8_t val) 00752 { 00753 lis2dw12_ctrl2_t reg; 00754 int32_t ret; 00755 00756 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00757 if (ret == 0) { 00758 reg.if_add_inc = val; 00759 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00760 } 00761 return ret; 00762 } 00763 00764 /** 00765 * @brief Register address automatically incremented during multiple 00766 * byte access with a serial interface.[get] 00767 * 00768 * @param ctx read / write interface definitions 00769 * @param val change the values of if_add_inc in reg CTRL2 00770 * @retval interface status (MANDATORY: return 0 -> no Error) 00771 * 00772 */ 00773 int32_t lis2dw12_auto_increment_get(lis2dw12_ctx_t *ctx, uint8_t *val) 00774 { 00775 lis2dw12_ctrl2_t reg; 00776 int32_t ret; 00777 00778 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00779 *val = reg.if_add_inc; 00780 00781 return ret; 00782 } 00783 00784 /** 00785 * @brief Software reset. Restore the default values in user registers.[set] 00786 * 00787 * @param ctx read / write interface definitions 00788 * @param val change the values of soft_reset in reg CTRL2 00789 * @retval interface status (MANDATORY: return 0 -> no Error) 00790 * 00791 */ 00792 int32_t lis2dw12_reset_set(lis2dw12_ctx_t *ctx, uint8_t val) 00793 { 00794 lis2dw12_ctrl2_t reg; 00795 int32_t ret; 00796 00797 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00798 if (ret == 0) { 00799 reg.soft_reset = val; 00800 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00801 } 00802 00803 return ret; 00804 } 00805 00806 /** 00807 * @brief Software reset. Restore the default values in user registers.[get] 00808 * 00809 * @param ctx read / write interface definitions 00810 * @param val change the values of soft_reset in reg CTRL2 00811 * @retval interface status (MANDATORY: return 0 -> no Error) 00812 * 00813 */ 00814 int32_t lis2dw12_reset_get(lis2dw12_ctx_t *ctx, uint8_t *val) 00815 { 00816 lis2dw12_ctrl2_t reg; 00817 int32_t ret; 00818 00819 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00820 *val = reg.soft_reset; 00821 00822 return ret; 00823 } 00824 00825 /** 00826 * @brief Reboot memory content. Reload the calibration parameters.[set] 00827 * 00828 * @param ctx read / write interface definitions 00829 * @param val change the values of boot in reg CTRL2 00830 * @retval interface status (MANDATORY: return 0 -> no Error) 00831 * 00832 */ 00833 int32_t lis2dw12_boot_set(lis2dw12_ctx_t *ctx, uint8_t val) 00834 { 00835 lis2dw12_ctrl2_t reg; 00836 int32_t ret; 00837 00838 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00839 if (ret == 0) { 00840 reg.boot = val; 00841 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00842 } 00843 return ret; 00844 } 00845 00846 /** 00847 * @brief Reboot memory content. Reload the calibration parameters.[get] 00848 * 00849 * @param ctx read / write interface definitions 00850 * @param val change the values of boot in reg CTRL2 00851 * @retval interface status (MANDATORY: return 0 -> no Error) 00852 * 00853 */ 00854 int32_t lis2dw12_boot_get(lis2dw12_ctx_t *ctx, uint8_t *val) 00855 { 00856 lis2dw12_ctrl2_t reg; 00857 int32_t ret; 00858 00859 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 00860 *val = reg.boot; 00861 00862 return ret; 00863 } 00864 00865 /** 00866 * @brief Sensor self-test enable.[set] 00867 * 00868 * @param ctx read / write interface definitions 00869 * @param val change the values of st in reg CTRL3 00870 * @retval interface status (MANDATORY: return 0 -> no Error) 00871 * 00872 */ 00873 int32_t lis2dw12_self_test_set(lis2dw12_ctx_t *ctx, lis2dw12_st_t val) 00874 { 00875 lis2dw12_ctrl3_t reg; 00876 int32_t ret; 00877 00878 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 00879 if (ret == 0) { 00880 reg.st = (uint8_t) val; 00881 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 00882 } 00883 00884 return ret; 00885 } 00886 00887 /** 00888 * @brief Sensor self-test enable.[get] 00889 * 00890 * @param ctx read / write interface definitions 00891 * @param val Get the values of st in reg CTRL3 00892 * @retval interface status (MANDATORY: return 0 -> no Error) 00893 * 00894 */ 00895 int32_t lis2dw12_self_test_get(lis2dw12_ctx_t *ctx, lis2dw12_st_t *val) 00896 { 00897 lis2dw12_ctrl3_t reg; 00898 int32_t ret; 00899 00900 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 00901 00902 switch (reg.st) { 00903 case LIS2DW12_XL_ST_DISABLE: 00904 *val = LIS2DW12_XL_ST_DISABLE; 00905 break; 00906 case LIS2DW12_XL_ST_POSITIVE: 00907 *val = LIS2DW12_XL_ST_POSITIVE; 00908 break; 00909 case LIS2DW12_XL_ST_NEGATIVE: 00910 *val = LIS2DW12_XL_ST_NEGATIVE; 00911 break; 00912 default: 00913 *val = LIS2DW12_XL_ST_DISABLE; 00914 break; 00915 } 00916 return ret; 00917 } 00918 00919 /** 00920 * @brief Data-ready pulsed / letched mode.[set] 00921 * 00922 * @param ctx read / write interface definitions 00923 * @param val change the values of drdy_pulsed in reg CTRL_REG7 00924 * @retval interface status (MANDATORY: return 0 -> no Error) 00925 * 00926 */ 00927 int32_t lis2dw12_data_ready_mode_set(lis2dw12_ctx_t *ctx, 00928 lis2dw12_drdy_pulsed_t val) 00929 { 00930 lis2dw12_ctrl_reg7_t reg; 00931 int32_t ret; 00932 00933 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00934 if (ret == 0) { 00935 reg.drdy_pulsed = (uint8_t) val; 00936 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00937 } 00938 00939 return ret; 00940 } 00941 00942 /** 00943 * @brief Data-ready pulsed / letched mode.[get] 00944 * 00945 * @param ctx read / write interface definitions 00946 * @param val Get the values of drdy_pulsed in reg CTRL_REG7 00947 * @retval interface status (MANDATORY: return 0 -> no Error) 00948 * 00949 */ 00950 int32_t lis2dw12_data_ready_mode_get(lis2dw12_ctx_t *ctx, 00951 lis2dw12_drdy_pulsed_t *val) 00952 { 00953 lis2dw12_ctrl_reg7_t reg; 00954 int32_t ret; 00955 00956 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 00957 00958 switch (reg.drdy_pulsed) { 00959 case LIS2DW12_DRDY_LATCHED: 00960 *val = LIS2DW12_DRDY_LATCHED; 00961 break; 00962 case LIS2DW12_DRDY_PULSED: 00963 *val = LIS2DW12_DRDY_PULSED; 00964 break; 00965 default: 00966 *val = LIS2DW12_DRDY_LATCHED; 00967 break; 00968 } 00969 return ret; 00970 } 00971 00972 /** 00973 * @} 00974 * 00975 */ 00976 00977 /** 00978 * @defgroup LIS2DW12_Filters 00979 * @brief This section group all the functions concerning the filters 00980 * configuration. 00981 * @{ 00982 * 00983 */ 00984 00985 /** 00986 * @brief Accelerometer filtering path for outputs.[set] 00987 * 00988 * @param ctx read / write interface definitions 00989 * @param val change the values of fds in reg CTRL6 00990 * @retval interface status (MANDATORY: return 0 -> no Error) 00991 * 00992 */ 00993 int32_t lis2dw12_filter_path_set(lis2dw12_ctx_t *ctx, lis2dw12_fds_t val) 00994 { 00995 lis2dw12_ctrl6_t ctrl6; 00996 lis2dw12_ctrl_reg7_t ctrl_reg7; 00997 int32_t ret; 00998 00999 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 01000 if (ret == 0) { 01001 ctrl6.fds = ((uint8_t) val & 0x10U) >> 4; 01002 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 01003 } 01004 if (ret == 0) { 01005 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) &ctrl_reg7, 1); 01006 } 01007 if (ret == 0) { 01008 ctrl_reg7.usr_off_on_out = (uint8_t) val & 0x01U; 01009 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) &ctrl_reg7, 1); 01010 } 01011 01012 return ret; 01013 } 01014 01015 /** 01016 * @brief Accelerometer filtering path for outputs.[get] 01017 * 01018 * @param ctx read / write interface definitions 01019 * @param val Get the values of fds in reg CTRL6 01020 * @retval interface status (MANDATORY: return 0 -> no Error) 01021 * 01022 */ 01023 int32_t lis2dw12_filter_path_get(lis2dw12_ctx_t *ctx, lis2dw12_fds_t *val) 01024 { 01025 lis2dw12_ctrl6_t ctrl6; 01026 lis2dw12_ctrl_reg7_t ctrl_reg7; 01027 int32_t ret; 01028 01029 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) &ctrl6, 1); 01030 if (ret == 0) { 01031 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) &ctrl_reg7, 1); 01032 01033 switch ((ctrl6.fds << 4) + ctrl_reg7.usr_off_on_out) { 01034 case LIS2DW12_LPF_ON_OUT: 01035 *val = LIS2DW12_LPF_ON_OUT; 01036 break; 01037 case LIS2DW12_USER_OFFSET_ON_OUT: 01038 *val = LIS2DW12_USER_OFFSET_ON_OUT; 01039 break; 01040 case LIS2DW12_HIGH_PASS_ON_OUT: 01041 *val = LIS2DW12_HIGH_PASS_ON_OUT; 01042 break; 01043 default: 01044 *val = LIS2DW12_LPF_ON_OUT; 01045 break; 01046 } 01047 } 01048 return ret; 01049 } 01050 01051 /** 01052 * @brief Accelerometer cutoff filter frequency. Valid for low and high 01053 * pass filter.[set] 01054 * 01055 * @param ctx read / write interface definitions 01056 * @param val change the values of bw_filt in reg CTRL6 01057 * @retval interface status (MANDATORY: return 0 -> no Error) 01058 * 01059 */ 01060 int32_t lis2dw12_filter_bandwidth_set(lis2dw12_ctx_t *ctx, 01061 lis2dw12_bw_filt_t val) 01062 { 01063 lis2dw12_ctrl6_t reg; 01064 int32_t ret; 01065 01066 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 01067 if (ret == 0) { 01068 reg.bw_filt = (uint8_t) val; 01069 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 01070 } 01071 01072 return ret; 01073 } 01074 01075 /** 01076 * @brief Accelerometer cutoff filter frequency. Valid for low and 01077 * high pass filter.[get] 01078 * 01079 * @param ctx read / write interface definitions 01080 * @param val Get the values of bw_filt in reg CTRL6 01081 * @retval interface status (MANDATORY: return 0 -> no Error) 01082 * 01083 */ 01084 int32_t lis2dw12_filter_bandwidth_get(lis2dw12_ctx_t *ctx, 01085 lis2dw12_bw_filt_t *val) 01086 { 01087 lis2dw12_ctrl6_t reg; 01088 int32_t ret; 01089 01090 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL6, (uint8_t *) ®, 1); 01091 01092 switch (reg.bw_filt) { 01093 case LIS2DW12_ODR_DIV_2: 01094 *val = LIS2DW12_ODR_DIV_2; 01095 break; 01096 case LIS2DW12_ODR_DIV_4: 01097 *val = LIS2DW12_ODR_DIV_4; 01098 break; 01099 case LIS2DW12_ODR_DIV_10: 01100 *val = LIS2DW12_ODR_DIV_10; 01101 break; 01102 case LIS2DW12_ODR_DIV_20: 01103 *val = LIS2DW12_ODR_DIV_20; 01104 break; 01105 default: 01106 *val = LIS2DW12_ODR_DIV_2; 01107 break; 01108 } 01109 return ret; 01110 } 01111 01112 /** 01113 * @brief Enable HP filter reference mode.[set] 01114 * 01115 * @param ctx read / write interface definitions 01116 * @param val change the values of hp_ref_mode in reg CTRL_REG7 01117 * @retval interface status (MANDATORY: return 0 -> no Error) 01118 * 01119 */ 01120 int32_t lis2dw12_reference_mode_set(lis2dw12_ctx_t *ctx, uint8_t val) 01121 { 01122 lis2dw12_ctrl_reg7_t reg; 01123 int32_t ret; 01124 01125 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01126 if (ret == 0) { 01127 reg.hp_ref_mode = val; 01128 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01129 } 01130 return ret; 01131 } 01132 01133 /** 01134 * @brief Enable HP filter reference mode.[get] 01135 * 01136 * @param ctx read / write interface definitions 01137 * @param val change the values of hp_ref_mode in reg CTRL_REG7 01138 * @retval interface status (MANDATORY: return 0 -> no Error) 01139 * 01140 */ 01141 int32_t lis2dw12_reference_mode_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01142 { 01143 lis2dw12_ctrl_reg7_t reg; 01144 int32_t ret; 01145 01146 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01147 *val = reg.hp_ref_mode; 01148 01149 return ret; 01150 } 01151 01152 /** 01153 * @} 01154 * 01155 */ 01156 01157 /** 01158 * @defgroup LIS2DW12_Serial_Interface 01159 * @brief This section groups all the functions concerning main serial 01160 * interface management (not auxiliary) 01161 * @{ 01162 * 01163 */ 01164 01165 /** 01166 * @brief SPI Serial Interface Mode selection.[set] 01167 * 01168 * @param ctx read / write interface definitions 01169 * @param val change the values of sim in reg CTRL2 01170 * @retval interface status (MANDATORY: return 0 -> no Error) 01171 * 01172 */ 01173 int32_t lis2dw12_spi_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_sim_t val) 01174 { 01175 lis2dw12_ctrl2_t reg; 01176 int32_t ret; 01177 01178 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01179 if (ret == 0) { 01180 reg.sim = (uint8_t) val; 01181 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01182 } 01183 return ret; 01184 } 01185 01186 /** 01187 * @brief SPI Serial Interface Mode selection.[get] 01188 * 01189 * @param ctx read / write interface definitions 01190 * @param val Get the values of sim in reg CTRL2 01191 * @retval interface status (MANDATORY: return 0 -> no Error) 01192 * 01193 */ 01194 int32_t lis2dw12_spi_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_sim_t *val) 01195 { 01196 lis2dw12_ctrl2_t reg; 01197 int32_t ret; 01198 01199 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01200 01201 switch (reg.sim) { 01202 case LIS2DW12_SPI_4_WIRE: 01203 *val = LIS2DW12_SPI_4_WIRE; 01204 break; 01205 case LIS2DW12_SPI_3_WIRE: 01206 *val = LIS2DW12_SPI_3_WIRE; 01207 break; 01208 default: 01209 *val = LIS2DW12_SPI_4_WIRE; 01210 break; 01211 } 01212 return ret; 01213 } 01214 01215 /** 01216 * @brief Disable / Enable I2C interface.[set] 01217 * 01218 * @param ctx read / write interface definitions 01219 * @param val change the values of i2c_disable in 01220 * reg CTRL2 01221 * @retval interface status (MANDATORY: return 0 -> no Error) 01222 * 01223 */ 01224 int32_t lis2dw12_i2c_interface_set(lis2dw12_ctx_t *ctx, 01225 lis2dw12_i2c_disable_t val) 01226 { 01227 lis2dw12_ctrl2_t reg; 01228 int32_t ret; 01229 01230 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01231 if (ret == 0) { 01232 reg.i2c_disable = (uint8_t) val; 01233 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01234 } 01235 return ret; 01236 } 01237 01238 /** 01239 * @brief Disable / Enable I2C interface.[get] 01240 * 01241 * @param ctx read / write interface definitions 01242 * @param val Get the values of i2c_disable in reg CTRL2 01243 * @retval interface status (MANDATORY: return 0 -> no Error) 01244 * 01245 */ 01246 int32_t lis2dw12_i2c_interface_get(lis2dw12_ctx_t *ctx, 01247 lis2dw12_i2c_disable_t *val) 01248 { 01249 lis2dw12_ctrl2_t reg; 01250 int32_t ret; 01251 01252 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01253 01254 switch (reg.i2c_disable) { 01255 case LIS2DW12_I2C_ENABLE: 01256 *val = LIS2DW12_I2C_ENABLE; 01257 break; 01258 case LIS2DW12_I2C_DISABLE: 01259 *val = LIS2DW12_I2C_DISABLE; 01260 break; 01261 default: 01262 *val = LIS2DW12_I2C_ENABLE; 01263 break; 01264 } 01265 return ret; 01266 } 01267 01268 /** 01269 * @brief Disconnect CS pull-up.[set] 01270 * 01271 * @param ctx read / write interface definitions 01272 * @param val change the values of cs_pu_disc in reg CTRL2 01273 * @retval interface status (MANDATORY: return 0 -> no Error) 01274 * 01275 */ 01276 int32_t lis2dw12_cs_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_cs_pu_disc_t val) 01277 { 01278 lis2dw12_ctrl2_t reg; 01279 int32_t ret; 01280 01281 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01282 if (ret == 0) { 01283 reg.cs_pu_disc = (uint8_t) val; 01284 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01285 } 01286 return ret; 01287 } 01288 01289 /** 01290 * @brief Disconnect CS pull-up.[get] 01291 * 01292 * @param ctx read / write interface definitions 01293 * @param val Get the values of cs_pu_disc in reg CTRL2 01294 * @retval interface status (MANDATORY: return 0 -> no Error) 01295 * 01296 */ 01297 int32_t lis2dw12_cs_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_cs_pu_disc_t *val) 01298 { 01299 lis2dw12_ctrl2_t reg; 01300 int32_t ret; 01301 01302 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL2, (uint8_t *) ®, 1); 01303 01304 switch (reg.cs_pu_disc) { 01305 case LIS2DW12_PULL_UP_CONNECT: 01306 *val = LIS2DW12_PULL_UP_CONNECT; 01307 break; 01308 case LIS2DW12_PULL_UP_DISCONNECT: 01309 *val = LIS2DW12_PULL_UP_DISCONNECT; 01310 break; 01311 default: 01312 *val = LIS2DW12_PULL_UP_CONNECT; 01313 break; 01314 } 01315 return ret; 01316 } 01317 01318 /** 01319 * @} 01320 * 01321 */ 01322 01323 /** 01324 * @defgroup LIS2DW12_Interrupt_Pins 01325 * @brief This section groups all the functions that manage interrupt pins 01326 * @{ 01327 * 01328 */ 01329 01330 /** 01331 * @brief Interrupt active-high/low.[set] 01332 * 01333 * @param ctx read / write interface definitions 01334 * @param val change the values of h_lactive in reg CTRL3 01335 * @retval interface status (MANDATORY: return 0 -> no Error) 01336 * 01337 */ 01338 int32_t lis2dw12_pin_polarity_set(lis2dw12_ctx_t *ctx, 01339 lis2dw12_h_lactive_t val) 01340 { 01341 lis2dw12_ctrl3_t reg; 01342 int32_t ret; 01343 01344 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01345 if (ret == 0) { 01346 reg.h_lactive = (uint8_t) val; 01347 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01348 } 01349 return ret; 01350 } 01351 01352 /** 01353 * @brief Interrupt active-high/low.[get] 01354 * 01355 * @param ctx read / write interface definitions 01356 * @param val Get the values of h_lactive in reg CTRL3 01357 * @retval interface status (MANDATORY: return 0 -> no Error) 01358 * 01359 */ 01360 int32_t lis2dw12_pin_polarity_get(lis2dw12_ctx_t *ctx, 01361 lis2dw12_h_lactive_t *val) 01362 { 01363 lis2dw12_ctrl3_t reg; 01364 int32_t ret; 01365 01366 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01367 01368 switch (reg.h_lactive) { 01369 case LIS2DW12_ACTIVE_HIGH: 01370 *val = LIS2DW12_ACTIVE_HIGH; 01371 break; 01372 case LIS2DW12_ACTIVE_LOW: 01373 *val = LIS2DW12_ACTIVE_LOW; 01374 break; 01375 default: 01376 *val = LIS2DW12_ACTIVE_HIGH; 01377 break; 01378 } 01379 return ret; 01380 } 01381 01382 /** 01383 * @brief Latched/pulsed interrupt.[set] 01384 * 01385 * @param ctx read / write interface definitions 01386 * @param val change the values of lir in reg CTRL3 01387 * @retval interface status (MANDATORY: return 0 -> no Error) 01388 * 01389 */ 01390 int32_t lis2dw12_int_notification_set(lis2dw12_ctx_t *ctx, 01391 lis2dw12_lir_t val) 01392 { 01393 lis2dw12_ctrl3_t reg; 01394 int32_t ret; 01395 01396 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01397 if (ret == 0) { 01398 reg.lir = (uint8_t) val; 01399 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01400 } 01401 return ret; 01402 } 01403 01404 /** 01405 * @brief Latched/pulsed interrupt.[get] 01406 * 01407 * @param ctx read / write interface definitions 01408 * @param val Get the values of lir in reg CTRL3 01409 * @retval interface status (MANDATORY: return 0 -> no Error) 01410 * 01411 */ 01412 int32_t lis2dw12_int_notification_get(lis2dw12_ctx_t *ctx, 01413 lis2dw12_lir_t *val) 01414 { 01415 lis2dw12_ctrl3_t reg; 01416 int32_t ret; 01417 01418 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01419 01420 switch (reg.lir) { 01421 case LIS2DW12_INT_PULSED: 01422 *val = LIS2DW12_INT_PULSED; 01423 break; 01424 case LIS2DW12_INT_LATCHED: 01425 *val = LIS2DW12_INT_LATCHED; 01426 break; 01427 default: 01428 *val = LIS2DW12_INT_PULSED; 01429 break; 01430 } 01431 return ret; 01432 } 01433 01434 /** 01435 * @brief Push-pull/open drain selection on interrupt pads.[set] 01436 * 01437 * @param ctx read / write interface definitions 01438 * @param val change the values of pp_od in reg CTRL3 01439 * @retval interface status (MANDATORY: return 0 -> no Error) 01440 * 01441 */ 01442 int32_t lis2dw12_pin_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_pp_od_t val) 01443 { 01444 lis2dw12_ctrl3_t reg; 01445 int32_t ret; 01446 01447 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01448 if (ret == 0) { 01449 reg.pp_od = (uint8_t) val; 01450 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01451 } 01452 return ret; 01453 } 01454 01455 /** 01456 * @brief Push-pull/open drain selection on interrupt pads.[get] 01457 * 01458 * @param ctx read / write interface definitions 01459 * @param val Get the values of pp_od in reg CTRL3 01460 * @retval interface status (MANDATORY: return 0 -> no Error) 01461 * 01462 */ 01463 int32_t lis2dw12_pin_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_pp_od_t *val) 01464 { 01465 lis2dw12_ctrl3_t reg; 01466 int32_t ret; 01467 01468 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL3, (uint8_t *) ®, 1); 01469 01470 switch (reg.pp_od) { 01471 case LIS2DW12_PUSH_PULL: 01472 *val = LIS2DW12_PUSH_PULL; 01473 break; 01474 case LIS2DW12_OPEN_DRAIN: 01475 *val = LIS2DW12_OPEN_DRAIN; 01476 break; 01477 default: 01478 *val = LIS2DW12_PUSH_PULL; 01479 break; 01480 } 01481 return ret; 01482 } 01483 01484 /** 01485 * @brief Select the signal that need to route on int1 pad.[set] 01486 * 01487 * @param ctx read / write interface definitions 01488 * @param val register CTRL4_INT1_PAD_CTRL. 01489 * @retval interface status (MANDATORY: return 0 -> no Error) 01490 * 01491 */ 01492 int32_t lis2dw12_pin_int1_route_set(lis2dw12_ctx_t *ctx, 01493 lis2dw12_ctrl4_int1_pad_ctrl_t *val) 01494 { 01495 lis2dw12_ctrl_reg7_t reg; 01496 int32_t ret; 01497 01498 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01499 if (ret == 0) { 01500 if ((val->int1_tap | val->int1_ff | val->int1_wu | val->int1_single_tap | 01501 val->int1_6d) != PROPERTY_DISABLE) { 01502 reg.interrupts_enable = PROPERTY_ENABLE; 01503 } else { 01504 reg.interrupts_enable = PROPERTY_DISABLE; 01505 } 01506 01507 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL4_INT1_PAD_CTRL, 01508 (uint8_t *) val, 1); 01509 } 01510 if (ret == 0) { 01511 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01512 } 01513 return ret; 01514 } 01515 01516 /** 01517 * @brief Select the signal that need to route on int1 pad.[get] 01518 * 01519 * @param ctx read / write interface definitions 01520 * @param val register CTRL4_INT1_PAD_CTRL. 01521 * @retval interface status (MANDATORY: return 0 -> no Error) 01522 * 01523 */ 01524 int32_t lis2dw12_pin_int1_route_get(lis2dw12_ctx_t *ctx, 01525 lis2dw12_ctrl4_int1_pad_ctrl_t *val) 01526 { 01527 int32_t ret; 01528 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL4_INT1_PAD_CTRL, 01529 (uint8_t *) val, 1); 01530 return ret; 01531 } 01532 01533 /** 01534 * @brief Select the signal that need to route on int2 pad.[set] 01535 * 01536 * @param ctx read / write interface definitions 01537 * @param val register CTRL5_INT2_PAD_CTRL. 01538 * @retval interface status (MANDATORY: return 0 -> no Error) 01539 * 01540 */ 01541 int32_t lis2dw12_pin_int2_route_set(lis2dw12_ctx_t *ctx, 01542 lis2dw12_ctrl5_int2_pad_ctrl_t *val) 01543 { 01544 lis2dw12_ctrl_reg7_t reg; 01545 int32_t ret; 01546 01547 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01548 if (ret == 0) { 01549 if ((val->int2_sleep_state | val->int2_sleep_chg) != PROPERTY_DISABLE) { 01550 reg.interrupts_enable = PROPERTY_ENABLE; 01551 } else { 01552 reg.interrupts_enable = PROPERTY_DISABLE; 01553 } 01554 01555 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL5_INT2_PAD_CTRL, 01556 (uint8_t *) val, 1); 01557 } 01558 if (ret == 0) { 01559 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01560 } 01561 01562 return ret; 01563 } 01564 01565 /** 01566 * @brief Select the signal that need to route on int2 pad.[get] 01567 * 01568 * @param ctx read / write interface definitions 01569 * @param val register CTRL5_INT2_PAD_CTRL 01570 * @retval interface status (MANDATORY: return 0 -> no Error) 01571 * 01572 */ 01573 int32_t lis2dw12_pin_int2_route_get(lis2dw12_ctx_t *ctx, 01574 lis2dw12_ctrl5_int2_pad_ctrl_t *val) 01575 { 01576 int32_t ret; 01577 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL5_INT2_PAD_CTRL, 01578 (uint8_t *) val, 1); 01579 return ret; 01580 } 01581 /** 01582 * @brief All interrupt signals become available on INT1 pin.[set] 01583 * 01584 * @param ctx read / write interface definitions 01585 * @param val change the values of int2_on_int1 in reg CTRL_REG7 01586 * @retval interface status (MANDATORY: return 0 -> no Error) 01587 * 01588 */ 01589 int32_t lis2dw12_all_on_int1_set(lis2dw12_ctx_t *ctx, uint8_t val) 01590 { 01591 lis2dw12_ctrl_reg7_t reg; 01592 int32_t ret; 01593 01594 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01595 if (ret == 0) { 01596 reg.int2_on_int1 = val; 01597 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01598 } 01599 return ret; 01600 } 01601 01602 /** 01603 * @brief All interrupt signals become available on INT1 pin.[get] 01604 * 01605 * @param ctx read / write interface definitions 01606 * @param val change the values of int2_on_int1 in reg CTRL_REG7 01607 * @retval interface status (MANDATORY: return 0 -> no Error) 01608 * 01609 */ 01610 int32_t lis2dw12_all_on_int1_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01611 { 01612 lis2dw12_ctrl_reg7_t reg; 01613 int32_t ret; 01614 01615 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01616 *val = reg.int2_on_int1; 01617 01618 return ret; 01619 } 01620 01621 /** 01622 * @} 01623 * 01624 */ 01625 01626 /** 01627 * @defgroup LIS2DW12_Wake_Up_Event 01628 * @brief This section groups all the functions that manage the Wake 01629 * Up event generation. 01630 * @{ 01631 * 01632 */ 01633 01634 /** 01635 * @brief Threshold for wakeup.1 LSB = FS_XL / 64.[set] 01636 * 01637 * @param ctx read / write interface definitions 01638 * @param val change the values of wk_ths in reg WAKE_UP_THS 01639 * @retval interface status (MANDATORY: return 0 -> no Error) 01640 * 01641 */ 01642 int32_t lis2dw12_wkup_threshold_set(lis2dw12_ctx_t *ctx, uint8_t val) 01643 { 01644 lis2dw12_wake_up_ths_t reg; 01645 int32_t ret; 01646 01647 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 01648 if (ret == 0) { 01649 reg.wk_ths = val; 01650 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 01651 } 01652 return ret; 01653 } 01654 01655 /** 01656 * @brief Threshold for wakeup.1 LSB = FS_XL / 64.[get] 01657 * 01658 * @param ctx read / write interface definitions 01659 * @param val change the values of wk_ths in reg WAKE_UP_THS 01660 * @retval interface status (MANDATORY: return 0 -> no Error) 01661 * 01662 */ 01663 int32_t lis2dw12_wkup_threshold_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01664 { 01665 lis2dw12_wake_up_ths_t reg; 01666 int32_t ret; 01667 01668 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 01669 *val = reg.wk_ths; 01670 01671 return ret; 01672 } 01673 01674 /** 01675 * @brief Wake up duration event.1LSb = 1 / ODR.[set] 01676 * 01677 * @param ctx read / write interface definitions 01678 * @param val change the values of wake_dur in reg WAKE_UP_DUR 01679 * @retval interface status (MANDATORY: return 0 -> no Error) 01680 * 01681 */ 01682 int32_t lis2dw12_wkup_dur_set(lis2dw12_ctx_t *ctx, uint8_t val) 01683 { 01684 lis2dw12_wake_up_dur_t reg; 01685 int32_t ret; 01686 01687 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01688 if (ret == 0) { 01689 reg.wake_dur = val; 01690 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01691 } 01692 return ret; 01693 } 01694 01695 /** 01696 * @brief Wake up duration event.1LSb = 1 / ODR.[get] 01697 * 01698 * @param ctx read / write interface definitions 01699 * @param val change the values of wake_dur in reg WAKE_UP_DUR 01700 * @retval interface status (MANDATORY: return 0 -> no Error) 01701 * 01702 */ 01703 int32_t lis2dw12_wkup_dur_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01704 { 01705 lis2dw12_wake_up_dur_t reg; 01706 int32_t ret; 01707 01708 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01709 *val = reg.wake_dur; 01710 01711 return ret; 01712 } 01713 01714 /** 01715 * @brief Data sent to wake-up interrupt function.[set] 01716 * 01717 * @param ctx read / write interface definitions 01718 * @param val change the values of usr_off_on_wu in reg CTRL_REG7 01719 * @retval interface status (MANDATORY: return 0 -> no Error) 01720 * 01721 */ 01722 int32_t lis2dw12_wkup_feed_data_set(lis2dw12_ctx_t *ctx, 01723 lis2dw12_usr_off_on_wu_t val) 01724 { 01725 lis2dw12_ctrl_reg7_t reg; 01726 int32_t ret; 01727 01728 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01729 if (ret == 0) { 01730 reg.usr_off_on_wu = (uint8_t) val; 01731 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01732 } 01733 return ret; 01734 } 01735 01736 /** 01737 * @brief Data sent to wake-up interrupt function.[get] 01738 * 01739 * @param ctx read / write interface definitions 01740 * @param val Get the values of usr_off_on_wu in reg CTRL_REG7 01741 * @retval interface status (MANDATORY: return 0 -> no Error) 01742 * 01743 */ 01744 int32_t lis2dw12_wkup_feed_data_get(lis2dw12_ctx_t *ctx, 01745 lis2dw12_usr_off_on_wu_t *val) 01746 { 01747 lis2dw12_ctrl_reg7_t reg; 01748 int32_t ret; 01749 01750 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 01751 01752 switch (reg.usr_off_on_wu) { 01753 case LIS2DW12_HP_FEED: 01754 *val = LIS2DW12_HP_FEED; 01755 break; 01756 case LIS2DW12_USER_OFFSET_FEED: 01757 *val = LIS2DW12_USER_OFFSET_FEED; 01758 break; 01759 default: 01760 *val = LIS2DW12_HP_FEED; 01761 break; 01762 } 01763 return ret; 01764 } 01765 01766 /** 01767 * @} 01768 * 01769 */ 01770 01771 /** 01772 * @defgroup LIS2DW12_Activity/Inactivity_Detection 01773 * @brief This section groups all the functions concerning 01774 * activity/inactivity detection. 01775 * @{ 01776 * 01777 */ 01778 01779 /** 01780 * @brief Config activity / inactivity or 01781 * stationary / motion detection.[set] 01782 * 01783 * @param ctx read / write interface definitions 01784 * @param val change the values of sleep_on / stationary in 01785 * reg WAKE_UP_THS / WAKE_UP_DUR 01786 * @retval interface status (MANDATORY: return 0 -> no Error) 01787 * 01788 */ 01789 int32_t lis2dw12_act_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_sleep_on_t val) 01790 { 01791 lis2dw12_wake_up_ths_t wake_up_ths; 01792 lis2dw12_wake_up_dur_t wake_up_dur; 01793 int32_t ret; 01794 01795 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) &wake_up_ths, 1); 01796 if (ret == 0) { 01797 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 01798 } 01799 if (ret == 0) { 01800 wake_up_ths.sleep_on = (uint8_t) val & 0x01U; 01801 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) &wake_up_ths, 1); 01802 } 01803 if (ret == 0) { 01804 wake_up_dur.stationary = ((uint8_t)val & 0x02U) >> 1; 01805 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 01806 } 01807 01808 return ret; 01809 } 01810 01811 /** 01812 * @brief Config activity / inactivity or 01813 * stationary / motion detection. [get] 01814 * 01815 * @param ctx read / write interface definitions 01816 * @param val Get the values of sleep_on in reg WAKE_UP_THS 01817 * @retval interface status (MANDATORY: return 0 -> no Error) 01818 * 01819 */ 01820 int32_t lis2dw12_act_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_sleep_on_t *val) 01821 { 01822 lis2dw12_wake_up_ths_t wake_up_ths; 01823 lis2dw12_wake_up_dur_t wake_up_dur;; 01824 int32_t ret; 01825 01826 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) &wake_up_ths, 1); 01827 if (ret == 0) { 01828 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 01829 01830 switch ((wake_up_dur.stationary << 1) + wake_up_ths.sleep_on) { 01831 case LIS2DW12_NO_DETECTION: 01832 *val = LIS2DW12_NO_DETECTION; 01833 break; 01834 case LIS2DW12_DETECT_ACT_INACT: 01835 *val = LIS2DW12_DETECT_ACT_INACT; 01836 break; 01837 case LIS2DW12_DETECT_STAT_MOTION: 01838 *val = LIS2DW12_DETECT_STAT_MOTION; 01839 break; 01840 default: 01841 *val = LIS2DW12_NO_DETECTION; 01842 break; 01843 } 01844 } 01845 return ret; 01846 } 01847 01848 /** 01849 * @brief Duration to go in sleep mode (1 LSb = 512 / ODR).[set] 01850 * 01851 * @param ctx read / write interface definitions 01852 * @param val change the values of sleep_dur in reg WAKE_UP_DUR 01853 * @retval interface status (MANDATORY: return 0 -> no Error) 01854 * 01855 */ 01856 int32_t lis2dw12_act_sleep_dur_set(lis2dw12_ctx_t *ctx, uint8_t val) 01857 { 01858 lis2dw12_wake_up_dur_t reg; 01859 int32_t ret; 01860 01861 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01862 if (ret == 0) { 01863 reg.sleep_dur = val; 01864 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01865 } 01866 return ret; 01867 } 01868 01869 /** 01870 * @brief Duration to go in sleep mode (1 LSb = 512 / ODR).[get] 01871 * 01872 * @param ctx read / write interface definitions 01873 * @param val change the values of sleep_dur in reg WAKE_UP_DUR 01874 * @retval interface status (MANDATORY: return 0 -> no Error) 01875 * 01876 */ 01877 int32_t lis2dw12_act_sleep_dur_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01878 { 01879 lis2dw12_wake_up_dur_t reg; 01880 int32_t ret; 01881 01882 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) ®, 1); 01883 *val = reg.sleep_dur; 01884 01885 return ret; 01886 } 01887 01888 /** 01889 * @} 01890 * 01891 */ 01892 01893 /** 01894 * @defgroup LIS2DW12_Tap_Generator 01895 * @brief This section groups all the functions that manage the tap 01896 * and double tap event generation. 01897 * @{ 01898 * 01899 */ 01900 01901 /** 01902 * @brief Threshold for tap recognition.[set] 01903 * 01904 * @param ctx read / write interface definitions 01905 * @param val change the values of tap_thsx in reg TAP_THS_X 01906 * @retval interface status (MANDATORY: return 0 -> no Error) 01907 * 01908 */ 01909 int32_t lis2dw12_tap_threshold_x_set(lis2dw12_ctx_t *ctx, uint8_t val) 01910 { 01911 lis2dw12_tap_ths_x_t reg; 01912 int32_t ret; 01913 01914 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 01915 if (ret == 0) { 01916 reg.tap_thsx = val; 01917 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 01918 } 01919 return ret; 01920 } 01921 01922 /** 01923 * @brief Threshold for tap recognition.[get] 01924 * 01925 * @param ctx read / write interface definitions 01926 * @param val change the values of tap_thsx in reg TAP_THS_X 01927 * @retval interface status (MANDATORY: return 0 -> no Error) 01928 * 01929 */ 01930 int32_t lis2dw12_tap_threshold_x_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01931 { 01932 lis2dw12_tap_ths_x_t reg; 01933 int32_t ret; 01934 01935 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 01936 *val = reg.tap_thsx; 01937 01938 return ret; 01939 } 01940 01941 /** 01942 * @brief Threshold for tap recognition.[set] 01943 * 01944 * @param ctx read / write interface definitions 01945 * @param val change the values of tap_thsy in reg TAP_THS_Y 01946 * @retval interface status (MANDATORY: return 0 -> no Error) 01947 * 01948 */ 01949 int32_t lis2dw12_tap_threshold_y_set(lis2dw12_ctx_t *ctx, uint8_t val) 01950 { 01951 lis2dw12_tap_ths_y_t reg; 01952 int32_t ret; 01953 01954 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 01955 if (ret == 0) { 01956 reg.tap_thsy = val; 01957 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 01958 } 01959 return ret; 01960 } 01961 01962 /** 01963 * @brief Threshold for tap recognition.[get] 01964 * 01965 * @param ctx read / write interface definitions 01966 * @param val change the values of tap_thsy in reg TAP_THS_Y 01967 * @retval interface status (MANDATORY: return 0 -> no Error) 01968 * 01969 */ 01970 int32_t lis2dw12_tap_threshold_y_get(lis2dw12_ctx_t *ctx, uint8_t *val) 01971 { 01972 lis2dw12_tap_ths_y_t reg; 01973 int32_t ret; 01974 01975 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 01976 *val = reg.tap_thsy; 01977 01978 return ret; 01979 } 01980 01981 /** 01982 * @brief Selection of axis priority for TAP detection.[set] 01983 * 01984 * @param ctx read / write interface definitions 01985 * @param val change the values of tap_prior in reg TAP_THS_Y 01986 * @retval interface status (MANDATORY: return 0 -> no Error) 01987 * 01988 */ 01989 int32_t lis2dw12_tap_axis_priority_set(lis2dw12_ctx_t *ctx, 01990 lis2dw12_tap_prior_t val) 01991 { 01992 lis2dw12_tap_ths_y_t reg; 01993 int32_t ret; 01994 01995 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 01996 if (ret == 0) { 01997 reg.tap_prior = (uint8_t) val; 01998 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 01999 } 02000 return ret; 02001 } 02002 02003 /** 02004 * @brief Selection of axis priority for TAP detection.[get] 02005 * 02006 * @param ctx read / write interface definitions 02007 * @param val Get the values of tap_prior in reg TAP_THS_Y 02008 * @retval interface status (MANDATORY: return 0 -> no Error) 02009 * 02010 */ 02011 int32_t lis2dw12_tap_axis_priority_get(lis2dw12_ctx_t *ctx, 02012 lis2dw12_tap_prior_t *val) 02013 { 02014 lis2dw12_tap_ths_y_t reg; 02015 int32_t ret; 02016 02017 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Y, (uint8_t *) ®, 1); 02018 02019 switch (reg.tap_prior) { 02020 case LIS2DW12_XYZ: 02021 *val = LIS2DW12_XYZ; 02022 break; 02023 case LIS2DW12_YXZ: 02024 *val = LIS2DW12_YXZ; 02025 break; 02026 case LIS2DW12_XZY: 02027 *val = LIS2DW12_XZY; 02028 break; 02029 case LIS2DW12_ZYX: 02030 *val = LIS2DW12_ZYX; 02031 break; 02032 case LIS2DW12_YZX: 02033 *val = LIS2DW12_YZX; 02034 break; 02035 case LIS2DW12_ZXY: 02036 *val = LIS2DW12_ZXY; 02037 break; 02038 default: 02039 *val = LIS2DW12_XYZ; 02040 break; 02041 } 02042 return ret; 02043 } 02044 02045 /** 02046 * @brief Threshold for tap recognition.[set] 02047 * 02048 * @param ctx read / write interface definitions 02049 * @param val change the values of tap_thsz in reg TAP_THS_Z 02050 * @retval interface status (MANDATORY: return 0 -> no Error) 02051 * 02052 */ 02053 int32_t lis2dw12_tap_threshold_z_set(lis2dw12_ctx_t *ctx, uint8_t val) 02054 { 02055 lis2dw12_tap_ths_z_t reg; 02056 int32_t ret; 02057 02058 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02059 if (ret == 0) { 02060 reg.tap_thsz = val; 02061 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02062 } 02063 02064 return ret; 02065 } 02066 02067 /** 02068 * @brief Threshold for tap recognition.[get] 02069 * 02070 * @param ctx read / write interface definitions 02071 * @param val change the values of tap_thsz in reg TAP_THS_Z 02072 * @retval interface status (MANDATORY: return 0 -> no Error) 02073 * 02074 */ 02075 int32_t lis2dw12_tap_threshold_z_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02076 { 02077 lis2dw12_tap_ths_z_t reg; 02078 int32_t ret; 02079 02080 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02081 *val = reg.tap_thsz; 02082 02083 return ret; 02084 } 02085 02086 /** 02087 * @brief Enable Z direction in tap recognition.[set] 02088 * 02089 * @param ctx read / write interface definitions 02090 * @param val change the values of tap_z_en in reg TAP_THS_Z 02091 * @retval interface status (MANDATORY: return 0 -> no Error) 02092 * 02093 */ 02094 int32_t lis2dw12_tap_detection_on_z_set(lis2dw12_ctx_t *ctx, uint8_t val) 02095 { 02096 lis2dw12_tap_ths_z_t reg; 02097 int32_t ret; 02098 02099 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02100 if (ret == 0) { 02101 reg.tap_z_en = val; 02102 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02103 } 02104 return ret; 02105 } 02106 02107 /** 02108 * @brief Enable Z direction in tap recognition.[get] 02109 * 02110 * @param ctx read / write interface definitions 02111 * @param val change the values of tap_z_en in reg TAP_THS_Z 02112 * @retval interface status (MANDATORY: return 0 -> no Error) 02113 * 02114 */ 02115 int32_t lis2dw12_tap_detection_on_z_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02116 { 02117 lis2dw12_tap_ths_z_t reg; 02118 int32_t ret; 02119 02120 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02121 *val = reg.tap_z_en; 02122 02123 return ret; 02124 } 02125 02126 /** 02127 * @brief Enable Y direction in tap recognition.[set] 02128 * 02129 * @param ctx read / write interface definitions 02130 * @param val change the values of tap_y_en in reg TAP_THS_Z 02131 * @retval interface status (MANDATORY: return 0 -> no Error) 02132 * 02133 */ 02134 int32_t lis2dw12_tap_detection_on_y_set(lis2dw12_ctx_t *ctx, uint8_t val) 02135 { 02136 lis2dw12_tap_ths_z_t reg; 02137 int32_t ret; 02138 02139 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02140 if (ret == 0) { 02141 reg.tap_y_en = val; 02142 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02143 } 02144 return ret; 02145 } 02146 02147 /** 02148 * @brief Enable Y direction in tap recognition.[get] 02149 * 02150 * @param ctx read / write interface definitions 02151 * @param val change the values of tap_y_en in reg TAP_THS_Z 02152 * @retval interface status (MANDATORY: return 0 -> no Error) 02153 * 02154 */ 02155 int32_t lis2dw12_tap_detection_on_y_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02156 { 02157 lis2dw12_tap_ths_z_t reg; 02158 int32_t ret; 02159 02160 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02161 *val = reg.tap_y_en; 02162 02163 return ret; 02164 } 02165 02166 /** 02167 * @brief Enable X direction in tap recognition.[set] 02168 * 02169 * @param ctx read / write interface definitions 02170 * @param val change the values of tap_x_en in reg TAP_THS_Z 02171 * @retval interface status (MANDATORY: return 0 -> no Error) 02172 * 02173 */ 02174 int32_t lis2dw12_tap_detection_on_x_set(lis2dw12_ctx_t *ctx, uint8_t val) 02175 { 02176 lis2dw12_tap_ths_z_t reg; 02177 int32_t ret; 02178 02179 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02180 if (ret == 0) { 02181 reg.tap_x_en = val; 02182 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02183 } 02184 return ret; 02185 } 02186 02187 /** 02188 * @brief Enable X direction in tap recognition.[get] 02189 * 02190 * @param ctx read / write interface definitions 02191 * @param val change the values of tap_x_en in reg TAP_THS_Z 02192 * @retval interface status (MANDATORY: return 0 -> no Error) 02193 * 02194 */ 02195 int32_t lis2dw12_tap_detection_on_x_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02196 { 02197 lis2dw12_tap_ths_z_t reg; 02198 int32_t ret; 02199 02200 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_Z, (uint8_t *) ®, 1); 02201 *val = reg.tap_x_en; 02202 02203 return ret; 02204 } 02205 02206 /** 02207 * @brief Maximum duration is the maximum time of an overthreshold signal 02208 * detection to be recognized as a tap event. The default value 02209 * of these bits is 00b which corresponds to 4*ODR_XL time. 02210 * If the SHOCK[1:0] bits are set to a different value, 1LSB 02211 * corresponds to 8*ODR_XL time.[set] 02212 * 02213 * @param ctx read / write interface definitions 02214 * @param val change the values of shock in reg INT_DUR 02215 * @retval interface status (MANDATORY: return 0 -> no Error) 02216 * 02217 */ 02218 int32_t lis2dw12_tap_shock_set(lis2dw12_ctx_t *ctx, uint8_t val) 02219 { 02220 lis2dw12_int_dur_t reg; 02221 int32_t ret; 02222 02223 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02224 if (ret == 0) { 02225 reg.shock = val; 02226 ret = lis2dw12_write_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02227 } 02228 02229 return ret; 02230 } 02231 02232 /** 02233 * @brief Maximum duration is the maximum time of an overthreshold signal 02234 * detection to be recognized as a tap event. The default value 02235 * of these bits is 00b which corresponds to 4*ODR_XL time. 02236 * If the SHOCK[1:0] bits are set to a different value, 1LSB 02237 * corresponds to 8*ODR_XL time.[get] 02238 * 02239 * @param ctx read / write interface definitions 02240 * @param val change the values of shock in reg INT_DUR 02241 * @retval interface status (MANDATORY: return 0 -> no Error) 02242 * 02243 */ 02244 int32_t lis2dw12_tap_shock_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02245 { 02246 lis2dw12_int_dur_t reg; 02247 int32_t ret; 02248 02249 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02250 *val = reg.shock; 02251 02252 return ret; 02253 } 02254 02255 /** 02256 * @brief Quiet time is the time after the first detected tap in which 02257 * there must not be any overthreshold event. 02258 * The default value of these bits is 00b which corresponds 02259 * to 2*ODR_XL time. If the QUIET[1:0] bits are set to a different 02260 * value, 1LSB corresponds to 4*ODR_XL time.[set] 02261 * 02262 * @param ctx read / write interface definitions 02263 * @param val change the values of quiet in reg INT_DUR 02264 * @retval interface status (MANDATORY: return 0 -> no Error) 02265 * 02266 */ 02267 int32_t lis2dw12_tap_quiet_set(lis2dw12_ctx_t *ctx, uint8_t val) 02268 { 02269 lis2dw12_int_dur_t reg; 02270 int32_t ret; 02271 02272 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02273 if (ret == 0) { 02274 reg.quiet = val; 02275 ret = lis2dw12_write_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02276 } 02277 return ret; 02278 } 02279 02280 /** 02281 * @brief Quiet time is the time after the first detected tap in which 02282 * there must not be any overthreshold event. 02283 * The default value of these bits is 00b which corresponds 02284 * to 2*ODR_XL time. If the QUIET[1:0] bits are set to a different 02285 * value, 1LSB corresponds to 4*ODR_XL time.[get] 02286 * 02287 * @param ctx read / write interface definitions 02288 * @param val change the values of quiet in reg INT_DUR 02289 * @retval interface status (MANDATORY: return 0 -> no Error) 02290 * 02291 */ 02292 int32_t lis2dw12_tap_quiet_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02293 { 02294 lis2dw12_int_dur_t reg; 02295 int32_t ret; 02296 02297 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02298 *val = reg.quiet; 02299 02300 return ret; 02301 } 02302 02303 /** 02304 * @brief When double tap recognition is enabled, this register expresses 02305 * the maximum time between two consecutive detected taps to 02306 * determine a double tap event. 02307 * The default value of these bits is 0000b which corresponds 02308 * to 16*ODR_XL time. If the DUR[3:0] bits are set to a different 02309 * value, 1LSB corresponds to 32*ODR_XL time.[set] 02310 * 02311 * @param ctx read / write interface definitions 02312 * @param val change the values of latency in reg INT_DUR 02313 * @retval interface status (MANDATORY: return 0 -> no Error) 02314 * 02315 */ 02316 int32_t lis2dw12_tap_dur_set(lis2dw12_ctx_t *ctx, uint8_t val) 02317 { 02318 lis2dw12_int_dur_t reg; 02319 int32_t ret; 02320 02321 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02322 if (ret == 0) { 02323 reg.latency = val; 02324 ret = lis2dw12_write_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02325 } 02326 return ret; 02327 } 02328 02329 /** 02330 * @brief When double tap recognition is enabled, this register expresses 02331 * the maximum time between two consecutive detected taps to 02332 * determine a double tap event. 02333 * The default value of these bits is 0000b which corresponds 02334 * to 16*ODR_XL time. If the DUR[3:0] bits are set to a different 02335 * value, 1LSB corresponds to 32*ODR_XL time.[get] 02336 * 02337 * @param ctx read / write interface definitions 02338 * @param val change the values of latency in reg INT_DUR 02339 * @retval interface status (MANDATORY: return 0 -> no Error) 02340 * 02341 */ 02342 int32_t lis2dw12_tap_dur_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02343 { 02344 lis2dw12_int_dur_t reg; 02345 int32_t ret; 02346 02347 ret = lis2dw12_read_reg(ctx, LIS2DW12_INT_DUR, (uint8_t *) ®, 1); 02348 *val = reg.latency; 02349 02350 return ret; 02351 } 02352 02353 /** 02354 * @brief Single/double-tap event enable.[set] 02355 * 02356 * @param ctx read / write interface definitions 02357 * @param val change the values of single_double_tap in reg WAKE_UP_THS 02358 * @retval interface status (MANDATORY: return 0 -> no Error) 02359 * 02360 */ 02361 int32_t lis2dw12_tap_mode_set(lis2dw12_ctx_t *ctx, 02362 lis2dw12_single_double_tap_t val) 02363 { 02364 lis2dw12_wake_up_ths_t reg; 02365 int32_t ret; 02366 02367 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 02368 if (ret == 0) { 02369 reg.single_double_tap = (uint8_t) val; 02370 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 02371 } 02372 return ret; 02373 } 02374 02375 /** 02376 * @brief Single/double-tap event enable.[get] 02377 * 02378 * @param ctx read / write interface definitions 02379 * @param val Get the values of single_double_tap in reg WAKE_UP_THS 02380 * @retval interface status (MANDATORY: return 0 -> no Error) 02381 * 02382 */ 02383 int32_t lis2dw12_tap_mode_get(lis2dw12_ctx_t *ctx, 02384 lis2dw12_single_double_tap_t *val) 02385 { 02386 lis2dw12_wake_up_ths_t reg; 02387 int32_t ret; 02388 02389 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_THS, (uint8_t *) ®, 1); 02390 02391 switch (reg.single_double_tap) { 02392 case LIS2DW12_ONLY_SINGLE: 02393 *val = LIS2DW12_ONLY_SINGLE; 02394 break; 02395 case LIS2DW12_BOTH_SINGLE_DOUBLE: 02396 *val = LIS2DW12_BOTH_SINGLE_DOUBLE; 02397 break; 02398 default: 02399 *val = LIS2DW12_ONLY_SINGLE; 02400 break; 02401 } 02402 02403 return ret; 02404 } 02405 02406 /** 02407 * @brief Read the tap / double tap source register.[get] 02408 * 02409 * @param ctx read / write interface definitions 02410 * @param lis2dw12_tap_src: union of registers from TAP_SRC to 02411 * @retval interface status (MANDATORY: return 0 -> no Error) 02412 * 02413 */ 02414 int32_t lis2dw12_tap_src_get(lis2dw12_ctx_t *ctx, lis2dw12_tap_src_t *val) 02415 { 02416 int32_t ret; 02417 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_SRC, (uint8_t *) val, 1); 02418 return ret; 02419 } 02420 02421 /** 02422 * @} 02423 * 02424 */ 02425 02426 /** 02427 * @defgroup LIS2DW12_Six_Position_Detection(6D/4D) 02428 * @brief This section groups all the functions concerning six 02429 * position detection (6D). 02430 * @{ 02431 * 02432 */ 02433 02434 /** 02435 * @brief Threshold for 4D/6D function.[set] 02436 * 02437 * @param ctx read / write interface definitions 02438 * @param val change the values of 6d_ths in reg TAP_THS_X 02439 * @retval interface status (MANDATORY: return 0 -> no Error) 02440 * 02441 */ 02442 int32_t lis2dw12_6d_threshold_set(lis2dw12_ctx_t *ctx, uint8_t val) 02443 { 02444 lis2dw12_tap_ths_x_t reg; 02445 int32_t ret; 02446 02447 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02448 if (ret == 0) { 02449 reg._6d_ths = val; 02450 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02451 } 02452 return ret; 02453 } 02454 02455 /** 02456 * @brief Threshold for 4D/6D function.[get] 02457 * 02458 * @param ctx read / write interface definitions 02459 * @param val change the values of 6d_ths in reg TAP_THS_X 02460 * @retval interface status (MANDATORY: return 0 -> no Error) 02461 * 02462 */ 02463 int32_t lis2dw12_6d_threshold_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02464 { 02465 lis2dw12_tap_ths_x_t reg; 02466 int32_t ret; 02467 02468 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02469 *val = reg._6d_ths; 02470 02471 return ret; 02472 } 02473 02474 /** 02475 * @brief 4D orientation detection enable.[set] 02476 * 02477 * @param ctx read / write interface definitions 02478 * @param val change the values of 4d_en in reg TAP_THS_X 02479 * @retval interface status (MANDATORY: return 0 -> no Error) 02480 * 02481 */ 02482 int32_t lis2dw12_4d_mode_set(lis2dw12_ctx_t *ctx, uint8_t val) 02483 { 02484 lis2dw12_tap_ths_x_t reg; 02485 int32_t ret; 02486 02487 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02488 if (ret == 0) { 02489 reg._4d_en = val; 02490 ret = lis2dw12_write_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02491 } 02492 02493 return ret; 02494 } 02495 02496 /** 02497 * @brief 4D orientation detection enable.[get] 02498 * 02499 * @param ctx read / write interface definitions 02500 * @param val change the values of 4d_en in reg TAP_THS_X 02501 * @retval interface status (MANDATORY: return 0 -> no Error) 02502 * 02503 */ 02504 int32_t lis2dw12_4d_mode_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02505 { 02506 lis2dw12_tap_ths_x_t reg; 02507 int32_t ret; 02508 02509 ret = lis2dw12_read_reg(ctx, LIS2DW12_TAP_THS_X, (uint8_t *) ®, 1); 02510 *val = reg._4d_en; 02511 02512 return ret; 02513 } 02514 02515 /** 02516 * @brief Read the 6D tap source register.[get] 02517 * 02518 * @param ctx read / write interface definitions 02519 * @param val union of registers from SIXD_SRC 02520 * @retval interface status (MANDATORY: return 0 -> no Error) 02521 * 02522 */ 02523 int32_t lis2dw12_6d_src_get(lis2dw12_ctx_t *ctx, lis2dw12_sixd_src_t *val) 02524 { 02525 int32_t ret; 02526 ret = lis2dw12_read_reg(ctx, LIS2DW12_SIXD_SRC, (uint8_t *) val, 1); 02527 return ret; 02528 } 02529 /** 02530 * @brief Data sent to 6D interrupt function.[set] 02531 * 02532 * @param ctx read / write interface definitions 02533 * @param val change the values of lpass_on6d in reg CTRL_REG7 02534 * @retval interface status (MANDATORY: return 0 -> no Error) 02535 * 02536 */ 02537 int32_t lis2dw12_6d_feed_data_set(lis2dw12_ctx_t *ctx, 02538 lis2dw12_lpass_on6d_t val) 02539 { 02540 lis2dw12_ctrl_reg7_t reg; 02541 int32_t ret; 02542 02543 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 02544 if (ret == 0) { 02545 reg.lpass_on6d = (uint8_t) val; 02546 ret = lis2dw12_write_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 02547 } 02548 return ret; 02549 } 02550 02551 /** 02552 * @brief Data sent to 6D interrupt function.[get] 02553 * 02554 * @param ctx read / write interface definitions 02555 * @param val Get the values of lpass_on6d in reg CTRL_REG7 02556 * @retval interface status (MANDATORY: return 0 -> no Error) 02557 * 02558 */ 02559 int32_t lis2dw12_6d_feed_data_get(lis2dw12_ctx_t *ctx, 02560 lis2dw12_lpass_on6d_t *val) 02561 { 02562 lis2dw12_ctrl_reg7_t reg; 02563 int32_t ret; 02564 02565 ret = lis2dw12_read_reg(ctx, LIS2DW12_CTRL_REG7, (uint8_t *) ®, 1); 02566 02567 switch (reg.lpass_on6d) { 02568 case LIS2DW12_ODR_DIV_2_FEED: 02569 *val = LIS2DW12_ODR_DIV_2_FEED; 02570 break; 02571 case LIS2DW12_LPF2_FEED: 02572 *val = LIS2DW12_LPF2_FEED; 02573 break; 02574 default: 02575 *val = LIS2DW12_ODR_DIV_2_FEED; 02576 break; 02577 } 02578 return ret; 02579 } 02580 02581 /** 02582 * @} 02583 * 02584 */ 02585 02586 /** 02587 * @defgroup LIS2DW12_Free_Fall 02588 * @brief This section group all the functions concerning 02589 * the free fall detection. 02590 * @{ 02591 * 02592 */ 02593 02594 /** 02595 * @brief Wake up duration event(1LSb = 1 / ODR).[set] 02596 * 02597 * @param ctx read / write interface definitions 02598 * @param val change the values of ff_dur in reg 02599 * WAKE_UP_DUR /F REE_FALL 02600 * @retval interface status (MANDATORY: return 0 -> no Error) 02601 * 02602 */ 02603 int32_t lis2dw12_ff_dur_set(lis2dw12_ctx_t *ctx, uint8_t val) 02604 { 02605 lis2dw12_wake_up_dur_t wake_up_dur; 02606 lis2dw12_free_fall_t free_fall; 02607 int32_t ret; 02608 02609 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 02610 if (ret == 0) { 02611 ret = lis2dw12_read_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) &free_fall, 1); 02612 } 02613 if (ret == 0) { 02614 wake_up_dur.ff_dur = ((uint8_t) val & 0x20U) >> 5; 02615 free_fall.ff_dur = (uint8_t) val & 0x1FU; 02616 ret = lis2dw12_write_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 02617 } 02618 if (ret == 0) { 02619 ret = lis2dw12_write_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) &free_fall, 1); 02620 } 02621 02622 return ret; 02623 } 02624 02625 /** 02626 * @brief Wake up duration event(1LSb = 1 / ODR).[get] 02627 * 02628 * @param ctx read / write interface definitions 02629 * @param val change the values of ff_dur in 02630 * reg WAKE_UP_DUR /F REE_FALL 02631 * @retval interface status (MANDATORY: return 0 -> no Error) 02632 * 02633 */ 02634 int32_t lis2dw12_ff_dur_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02635 { 02636 lis2dw12_wake_up_dur_t wake_up_dur; 02637 lis2dw12_free_fall_t free_fall; 02638 int32_t ret; 02639 02640 ret = lis2dw12_read_reg(ctx, LIS2DW12_WAKE_UP_DUR, (uint8_t *) &wake_up_dur, 1); 02641 if (ret == 0) { 02642 ret = lis2dw12_read_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) &free_fall, 1); 02643 *val = (wake_up_dur.ff_dur << 5) + free_fall.ff_dur; 02644 } 02645 return ret; 02646 } 02647 02648 /** 02649 * @brief Free fall threshold setting.[set] 02650 * 02651 * @param ctx read / write interface definitions 02652 * @param val change the values of ff_ths in reg FREE_FALL 02653 * @retval interface status (MANDATORY: return 0 -> no Error) 02654 * 02655 */ 02656 int32_t lis2dw12_ff_threshold_set(lis2dw12_ctx_t *ctx, lis2dw12_ff_ths_t val) 02657 { 02658 lis2dw12_free_fall_t reg; 02659 int32_t ret; 02660 02661 ret = lis2dw12_read_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) ®, 1); 02662 if (ret == 0) { 02663 reg.ff_ths = (uint8_t) val; 02664 ret = lis2dw12_write_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) ®, 1); 02665 } 02666 02667 return ret; 02668 } 02669 02670 /** 02671 * @brief Free fall threshold setting.[get] 02672 * 02673 * @param ctx read / write interface definitions 02674 * @param val Get the values of ff_ths in reg FREE_FALL 02675 * @retval interface status (MANDATORY: return 0 -> no Error) 02676 * 02677 */ 02678 int32_t lis2dw12_ff_threshold_get(lis2dw12_ctx_t *ctx, 02679 lis2dw12_ff_ths_t *val) 02680 { 02681 lis2dw12_free_fall_t reg; 02682 int32_t ret; 02683 02684 ret = lis2dw12_read_reg(ctx, LIS2DW12_FREE_FALL, (uint8_t *) ®, 1); 02685 02686 switch (reg.ff_ths) { 02687 case LIS2DW12_FF_TSH_5LSb_FS2g: 02688 *val = LIS2DW12_FF_TSH_5LSb_FS2g; 02689 break; 02690 case LIS2DW12_FF_TSH_7LSb_FS2g: 02691 *val = LIS2DW12_FF_TSH_7LSb_FS2g; 02692 break; 02693 case LIS2DW12_FF_TSH_8LSb_FS2g: 02694 *val = LIS2DW12_FF_TSH_8LSb_FS2g; 02695 break; 02696 case LIS2DW12_FF_TSH_10LSb_FS2g: 02697 *val = LIS2DW12_FF_TSH_10LSb_FS2g; 02698 break; 02699 case LIS2DW12_FF_TSH_11LSb_FS2g: 02700 *val = LIS2DW12_FF_TSH_11LSb_FS2g; 02701 break; 02702 case LIS2DW12_FF_TSH_13LSb_FS2g: 02703 *val = LIS2DW12_FF_TSH_13LSb_FS2g; 02704 break; 02705 case LIS2DW12_FF_TSH_15LSb_FS2g: 02706 *val = LIS2DW12_FF_TSH_15LSb_FS2g; 02707 break; 02708 case LIS2DW12_FF_TSH_16LSb_FS2g: 02709 *val = LIS2DW12_FF_TSH_16LSb_FS2g; 02710 break; 02711 default: 02712 *val = LIS2DW12_FF_TSH_5LSb_FS2g; 02713 break; 02714 } 02715 return ret; 02716 } 02717 02718 /** 02719 * @} 02720 * 02721 */ 02722 02723 /** 02724 * @defgroup LIS2DW12_Fifo 02725 * @brief This section group all the functions concerning the fifo usage 02726 * @{ 02727 * 02728 */ 02729 02730 /** 02731 * @brief FIFO watermark level selection.[set] 02732 * 02733 * @param ctx read / write interface definitions 02734 * @param val change the values of fth in reg FIFO_CTRL 02735 * @retval interface status (MANDATORY: return 0 -> no Error) 02736 * 02737 */ 02738 int32_t lis2dw12_fifo_watermark_set(lis2dw12_ctx_t *ctx, uint8_t val) 02739 { 02740 lis2dw12_fifo_ctrl_t reg; 02741 int32_t ret; 02742 02743 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02744 if (ret == 0) { 02745 reg.fth = val; 02746 ret = lis2dw12_write_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02747 } 02748 02749 return ret; 02750 } 02751 02752 /** 02753 * @brief FIFO watermark level selection.[get] 02754 * 02755 * @param ctx read / write interface definitions 02756 * @param val change the values of fth in reg FIFO_CTRL 02757 * @retval interface status (MANDATORY: return 0 -> no Error) 02758 * 02759 */ 02760 int32_t lis2dw12_fifo_watermark_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02761 { 02762 lis2dw12_fifo_ctrl_t reg; 02763 int32_t ret; 02764 02765 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02766 *val = reg.fth; 02767 02768 return ret; 02769 } 02770 02771 /** 02772 * @brief FIFO mode selection.[set] 02773 * 02774 * @param ctx read / write interface definitions 02775 * @param val change the values of fmode in reg FIFO_CTRL 02776 * @retval interface status (MANDATORY: return 0 -> no Error) 02777 * 02778 */ 02779 int32_t lis2dw12_fifo_mode_set(lis2dw12_ctx_t *ctx, lis2dw12_fmode_t val) 02780 { 02781 lis2dw12_fifo_ctrl_t reg; 02782 int32_t ret; 02783 02784 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02785 if (ret == 0) { 02786 reg.fmode = (uint8_t) val; 02787 ret = lis2dw12_write_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02788 } 02789 return ret; 02790 } 02791 02792 /** 02793 * @brief FIFO mode selection.[get] 02794 * 02795 * @param ctx read / write interface definitions 02796 * @param val Get the values of fmode in reg FIFO_CTRL 02797 * @retval interface status (MANDATORY: return 0 -> no Error) 02798 * 02799 */ 02800 int32_t lis2dw12_fifo_mode_get(lis2dw12_ctx_t *ctx, lis2dw12_fmode_t *val) 02801 { 02802 lis2dw12_fifo_ctrl_t reg; 02803 int32_t ret; 02804 02805 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_CTRL, (uint8_t *) ®, 1); 02806 02807 switch (reg.fmode) { 02808 case LIS2DW12_BYPASS_MODE: 02809 *val = LIS2DW12_BYPASS_MODE; 02810 break; 02811 case LIS2DW12_FIFO_MODE: 02812 *val = LIS2DW12_FIFO_MODE; 02813 break; 02814 case LIS2DW12_STREAM_TO_FIFO_MODE: 02815 *val = LIS2DW12_STREAM_TO_FIFO_MODE; 02816 break; 02817 case LIS2DW12_BYPASS_TO_STREAM_MODE: 02818 *val = LIS2DW12_BYPASS_TO_STREAM_MODE; 02819 break; 02820 case LIS2DW12_STREAM_MODE: 02821 *val = LIS2DW12_STREAM_MODE; 02822 break; 02823 default: 02824 *val = LIS2DW12_BYPASS_MODE; 02825 break; 02826 } 02827 return ret; 02828 } 02829 02830 /** 02831 * @brief Number of unread samples stored in FIFO.[get] 02832 * 02833 * @param ctx read / write interface definitions 02834 * @param val change the values of diff in reg FIFO_SAMPLES 02835 * @retval interface status (MANDATORY: return 0 -> no Error) 02836 * 02837 */ 02838 int32_t lis2dw12_fifo_data_level_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02839 { 02840 lis2dw12_fifo_samples_t reg; 02841 int32_t ret; 02842 02843 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_SAMPLES, (uint8_t *) ®, 1); 02844 *val = reg.diff; 02845 02846 return ret; 02847 } 02848 /** 02849 * @brief FIFO overrun status.[get] 02850 * 02851 * @param ctx read / write interface definitions 02852 * @param val change the values of fifo_ovr in reg FIFO_SAMPLES 02853 * @retval interface status (MANDATORY: return 0 -> no Error) 02854 * 02855 */ 02856 int32_t lis2dw12_fifo_ovr_flag_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02857 { 02858 lis2dw12_fifo_samples_t reg; 02859 int32_t ret; 02860 02861 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_SAMPLES, (uint8_t *) ®, 1); 02862 *val = reg.fifo_ovr; 02863 02864 return ret; 02865 } 02866 /** 02867 * @brief FIFO threshold status flag.[get] 02868 * 02869 * @param ctx read / write interface definitions 02870 * @param val change the values of fifo_fth in reg FIFO_SAMPLES 02871 * @retval interface status (MANDATORY: return 0 -> no Error) 02872 * 02873 */ 02874 int32_t lis2dw12_fifo_wtm_flag_get(lis2dw12_ctx_t *ctx, uint8_t *val) 02875 { 02876 lis2dw12_fifo_samples_t reg; 02877 int32_t ret; 02878 02879 ret = lis2dw12_read_reg(ctx, LIS2DW12_FIFO_SAMPLES, (uint8_t *) ®, 1); 02880 *val = reg.fifo_fth; 02881 02882 return ret; 02883 } 02884 /** 02885 * @} 02886 * 02887 */ 02888 02889 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 14 2022 10:48:26 by
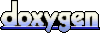