This class drives the LCD display (RK043FN48H-CT672B 4,3" 480x272 pixels device) present on DISCO_F746NG board.
Dependents: DISCO-F746NG_LCDTS_CC3000_NTP DISCO-F746NG_QSPI DISCO-F746NG_ROPE_WIFI F746_SpectralAnalysis_NoPhoto ... more
LCD_DISCO_F746NG.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __LCD_DISCO_F746NG_H 00020 #define __LCD_DISCO_F746NG_H 00021 00022 #ifdef TARGET_DISCO_F746NG 00023 00024 #include "mbed.h" 00025 #include "stm32746g_discovery_lcd.h" 00026 00027 /* 00028 This class drives the LCD display (RK043FN48H-CT672B 4,3" 480x272 pixels device) present on DISCO_F746NG board. 00029 00030 Usage: 00031 00032 #include "mbed.h" 00033 #include "LCD_DISCO_F746NG.h" 00034 00035 LCD_DISCO_F746NG lcd; 00036 00037 int main() 00038 { 00039 lcd.DisplayStringAt(0, LINE(1), (uint8_t *)"MBED EXAMPLE", CENTER_MODE); 00040 wait(1); 00041 lcd.Clear(LCD_COLOR_BLUE); 00042 lcd.SetBackColor(LCD_COLOR_BLUE); 00043 lcd.SetTextColor(LCD_COLOR_WHITE); 00044 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"DISCOVERY STM32F746NG", CENTER_MODE); 00045 while(1) 00046 { 00047 } 00048 } 00049 */ 00050 class LCD_DISCO_F746NG 00051 { 00052 00053 public: 00054 //! Constructor 00055 LCD_DISCO_F746NG(); 00056 00057 //! Destructor 00058 ~LCD_DISCO_F746NG(); 00059 00060 /** 00061 * @brief Initializes the LCD. 00062 * @retval LCD state 00063 */ 00064 uint8_t Init(void); 00065 00066 /** 00067 * @brief DeInitializes the LCD. 00068 * @retval LCD state 00069 */ 00070 uint8_t DeInit(void); 00071 00072 /** 00073 * @brief Gets the LCD X size. 00074 * @retval Used LCD X size 00075 */ 00076 uint32_t GetXSize(void); 00077 00078 /** 00079 * @brief Gets the LCD Y size. 00080 * @retval Used LCD Y size 00081 */ 00082 uint32_t GetYSize(void); 00083 00084 /** 00085 * @brief Set the LCD X size. 00086 * @param imageWidthPixels : image width in pixels unit 00087 * @retval None 00088 */ 00089 void SetXSize(uint32_t imageWidthPixels); 00090 00091 /** 00092 * @brief Set the LCD Y size. 00093 * @param imageHeightPixels : image height in lines unit 00094 * @retval None 00095 */ 00096 void SetYSize(uint32_t imageHeightPixels); 00097 00098 /** 00099 * @brief Initializes the LCD layer in ARGB8888 format (32 bits per pixel);. 00100 * @param LayerIndex: Layer foreground or background 00101 * @param FB_Address: Layer frame buffer 00102 * @retval None 00103 */ 00104 void LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address); 00105 00106 /** 00107 * @brief Initializes the LCD layer in RGB565 format (16 bits per pixel);. 00108 * @param LayerIndex: Layer foreground or background 00109 * @param FB_Address: Layer frame buffer 00110 * @retval None 00111 */ 00112 void LayerRgb565Init(uint16_t LayerIndex, uint32_t FB_Address); 00113 00114 /** 00115 * @brief Selects the LCD Layer. 00116 * @param LayerIndex: Layer foreground or background 00117 * @retval None 00118 */ 00119 void SelectLayer(uint32_t LayerIndex); 00120 00121 /** 00122 * @brief Sets an LCD Layer visible 00123 * @param LayerIndex: Visible Layer 00124 * @param State: New state of the specified layer 00125 * This parameter can be one of the following values: 00126 * @arg ENABLE 00127 * @arg DISABLE 00128 * @retval None 00129 */ 00130 void SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00131 00132 /** 00133 * @brief Configures the transparency. 00134 * @param LayerIndex: Layer foreground or background. 00135 * @param Transparency: Transparency 00136 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00137 * @retval None 00138 */ 00139 void SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00140 00141 /** 00142 * @brief Sets an LCD layer frame buffer address. 00143 * @param LayerIndex: Layer foreground or background 00144 * @param Address: New LCD frame buffer value 00145 * @retval None 00146 */ 00147 void SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00148 00149 /** 00150 * @brief Sets display window. 00151 * @param LayerIndex: Layer index 00152 * @param Xpos: LCD X position 00153 * @param Ypos: LCD Y position 00154 * @param Width: LCD window width 00155 * @param Height: LCD window height 00156 * @retval None 00157 */ 00158 void SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00159 00160 /** 00161 * @brief Configures and sets the color keying. 00162 * @param LayerIndex: Layer foreground or background 00163 * @param RGBValue: Color reference 00164 * @retval None 00165 */ 00166 void SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00167 00168 /** 00169 * @brief Disables the color keying. 00170 * @param LayerIndex: Layer foreground or background 00171 * @retval None 00172 */ 00173 void ResetColorKeying(uint32_t LayerIndex); 00174 00175 /** 00176 * @brief Sets the LCD text color. 00177 * @param Color: Text color code ARGB(8-8-8-8); 00178 * @retval None 00179 */ 00180 void SetTextColor(uint32_t Color); 00181 00182 /** 00183 * @brief Gets the LCD text color. 00184 * @retval Used text color. 00185 */ 00186 uint32_t GetTextColor(void); 00187 00188 /** 00189 * @brief Sets the LCD background color. 00190 * @param Color: Layer background color code ARGB(8-8-8-8); 00191 * @retval None 00192 */ 00193 void SetBackColor(uint32_t Color); 00194 00195 /** 00196 * @brief Gets the LCD background color. 00197 * @retval Used background colour 00198 */ 00199 uint32_t GetBackColor(void); 00200 00201 /** 00202 * @brief Sets the LCD text font. 00203 * @param fonts: Layer font to be used 00204 * @retval None 00205 */ 00206 void SetFont(sFONT *fonts); 00207 00208 /** 00209 * @brief Gets the LCD text font. 00210 * @retval Used layer font 00211 */ 00212 sFONT *GetFont(void); 00213 00214 /** 00215 * @brief Reads an LCD pixel. 00216 * @param Xpos: X position 00217 * @param Ypos: Y position 00218 * @retval RGB pixel color 00219 */ 00220 uint32_t ReadPixel(uint16_t Xpos, uint16_t Ypos); 00221 00222 /** 00223 * @brief Clears the whole LCD. 00224 * @param Color: Color of the background 00225 * @retval None 00226 */ 00227 void Clear(uint32_t Color); 00228 00229 /** 00230 * @brief Clears the selected line. 00231 * @param Line: Line to be cleared 00232 * @retval None 00233 */ 00234 void ClearStringLine(uint32_t Line); 00235 00236 /** 00237 * @brief Displays one character. 00238 * @param Xpos: Start column address 00239 * @param Ypos: Line where to display the character shape. 00240 * @param Ascii: Character ascii code 00241 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00242 * @retval None 00243 */ 00244 void DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00245 00246 /** 00247 * @brief Displays characters on the LCD. 00248 * @param Xpos: X position (in pixel); 00249 * @param Ypos: Y position (in pixel); 00250 * @param Text: Pointer to string to display on LCD 00251 * @param Mode: Display mode 00252 * This parameter can be one of the following values: 00253 * @arg CENTER_MODE 00254 * @arg RIGHT_MODE 00255 * @arg LEFT_MODE 00256 * @retval None 00257 */ 00258 void DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00259 00260 /** 00261 * @brief Displays a maximum of 60 characters on the LCD. 00262 * @param Line: Line where to display the character shape 00263 * @param ptr: Pointer to string to display on LCD 00264 * @retval None 00265 */ 00266 void DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00267 00268 /** 00269 * @brief Draws an horizontal line. 00270 * @param Xpos: X position 00271 * @param Ypos: Y position 00272 * @param Length: Line length 00273 * @retval None 00274 */ 00275 void DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00276 00277 /** 00278 * @brief Draws a vertical line. 00279 * @param Xpos: X position 00280 * @param Ypos: Y position 00281 * @param Length: Line length 00282 * @retval None 00283 */ 00284 void DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00285 00286 /** 00287 * @brief Draws an uni-line (between two points);. 00288 * @param x1: Point 1 X position 00289 * @param y1: Point 1 Y position 00290 * @param x2: Point 2 X position 00291 * @param y2: Point 2 Y position 00292 * @retval None 00293 */ 00294 void DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00295 00296 /** 00297 * @brief Draws a rectangle. 00298 * @param Xpos: X position 00299 * @param Ypos: Y position 00300 * @param Width: Rectangle width 00301 * @param Height: Rectangle height 00302 * @retval None 00303 */ 00304 void DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00305 00306 /** 00307 * @brief Draws a circle. 00308 * @param Xpos: X position 00309 * @param Ypos: Y position 00310 * @param Radius: Circle radius 00311 * @retval None 00312 */ 00313 void DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00314 00315 /** 00316 * @brief Draws an poly-line (between many points);. 00317 * @param Points: Pointer to the points array 00318 * @param PointCount: Number of points 00319 * @retval None 00320 */ 00321 void DrawPolygon(pPoint Points, uint16_t PointCount); 00322 00323 /** 00324 * @brief Draws an ellipse on LCD. 00325 * @param Xpos: X position 00326 * @param Ypos: Y position 00327 * @param XRadius: Ellipse X radius 00328 * @param YRadius: Ellipse Y radius 00329 * @retval None 00330 */ 00331 void DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00332 00333 /** 00334 * @brief Draws a pixel on LCD. 00335 * @param Xpos: X position 00336 * @param Ypos: Y position 00337 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8); 00338 * @retval None 00339 */ 00340 void DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code); 00341 00342 /** 00343 * @brief Draws a bitmap picture loaded in the internal Flash in ARGB888 format (32 bits per pixel);. 00344 * @param Xpos: Bmp X position in the LCD 00345 * @param Ypos: Bmp Y position in the LCD 00346 * @param pbmp: Pointer to Bmp picture address in the internal Flash 00347 * @retval None 00348 */ 00349 void DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00350 00351 /** 00352 * @brief Draws a full rectangle. 00353 * @param Xpos: X position 00354 * @param Ypos: Y position 00355 * @param Width: Rectangle width 00356 * @param Height: Rectangle height 00357 * @retval None 00358 */ 00359 void FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00360 00361 /** 00362 * @brief Draws a full circle. 00363 * @param Xpos: X position 00364 * @param Ypos: Y position 00365 * @param Radius: Circle radius 00366 * @retval None 00367 */ 00368 void FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00369 00370 /** 00371 * @brief Draws a full poly-line (between many points);. 00372 * @param Points: Pointer to the points array 00373 * @param PointCount: Number of points 00374 * @retval None 00375 */ 00376 void FillPolygon(pPoint Points, uint16_t PointCount); 00377 00378 /** 00379 * @brief Draws a full ellipse. 00380 * @param Xpos: X position 00381 * @param Ypos: Y position 00382 * @param XRadius: Ellipse X radius 00383 * @param YRadius: Ellipse Y radius 00384 * @retval None 00385 */ 00386 void FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00387 00388 /** 00389 * @brief Enables the display. 00390 * @retval None 00391 */ 00392 void DisplayOn(void); 00393 00394 /** 00395 * @brief Disables the display. 00396 * @retval None 00397 */ 00398 void DisplayOff(void); 00399 00400 private: 00401 00402 }; 00403 00404 #else 00405 #error "This class must be used with DISCO_F746NG board only." 00406 #endif // TARGET_DISCO_F746NG 00407 00408 #endif
Generated on Wed Jul 13 2022 01:53:09 by
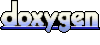