Class to drive a M24LR64 EEPROM with the STM32746G_DISCOVERY board. This EEPROM can be found on the additional ANT7-M24LR-A daughter board.
Dependents: DISCO-F746NG_Monttory_Base DISCO-F746NG_Monttory_Base
EEPROM_DISCO_F746NG.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __EEPROM_DISCO_F746NG_H 00020 #define __EEPROM_DISCO_F746NG_H 00021 00022 #ifdef TARGET_DISCO_F746NG 00023 00024 #include "mbed.h" 00025 #include "stm32746g_discovery_eeprom.h" 00026 00027 /* 00028 Class to drive a M24LR64 EEPROM. 00029 00030 =================================================================== 00031 Note: 00032 The I2C EEPROM memory (M24LR64) is available on a separate ANT7-M24LR-A 00033 daughter board (not provided with the STM32746G_DISCOVERY board). 00034 This daughter board must be connected on the CN2 connector. 00035 =================================================================== 00036 00037 Usage: 00038 00039 #include "mbed.h" 00040 #include "EEPROM_DISCO_F746NG.h" 00041 00042 EEPROM_DISCO_F746NG eep; 00043 00044 #define BUFFER_SIZE ((uint32_t)32) 00045 #define WRITE_READ_ADDR ((uint32_t)0x0000) 00046 00047 int main() 00048 { 00049 // 12345678901234567890123456789012 00050 uint8_t WriteBuffer[BUFFER_SIZE+1] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ012345"; 00051 uint8_t ReadBuffer[BUFFER_SIZE+1]; 00052 uint16_t bytes_rd; 00053 00054 // Check initialization 00055 if (eep.Init() != EEPROM_OK) 00056 { 00057 error("Initialization FAILED\n"); 00058 } 00059 00060 // Write buffer 00061 if (eep.WriteBuffer(WriteBuffer, WRITE_READ_ADDR, BUFFER_SIZE) != EEPROM_OK) 00062 { 00063 error("Write buffer FAILED\n"); 00064 } 00065 00066 // Read buffer 00067 bytes_rd = BUFFER_SIZE; 00068 if (eep.ReadBuffer(ReadBuffer, WRITE_READ_ADDR, &bytes_rd) != EEPROM_OK) 00069 { 00070 error("Read buffer FAILED\n"); 00071 } 00072 else 00073 { 00074 ReadBuffer[BUFFER_SIZE] = '\0'; 00075 printf("Read buffer PASSED\n"); 00076 printf("Buffer read = [%s]\n", ReadBuffer); 00077 printf("Bytes read = %d\n", bytes_rd); 00078 } 00079 00080 while(1) { 00081 } 00082 } 00083 00084 */ 00085 class EEPROM_DISCO_F746NG 00086 { 00087 00088 public: 00089 //! Constructor 00090 EEPROM_DISCO_F746NG(); 00091 00092 //! Destructor 00093 ~EEPROM_DISCO_F746NG(); 00094 00095 /** 00096 * @brief Initializes peripherals used by the I2C EEPROM driver. 00097 * 00098 * @note There are 2 different versions of M24LR64 (A01 & A02);. 00099 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01); 00100 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02); 00101 * @retval EEPROM_OK (0); if operation is correctly performed, else return value 00102 * different from EEPROM_OK (0); 00103 */ 00104 uint32_t Init(void); 00105 00106 /** 00107 * @brief DeInitializes the EEPROM. 00108 * @retval EEPROM state 00109 */ 00110 uint8_t DeInit(void); 00111 00112 /** 00113 * @brief Reads a block of data from the EEPROM. 00114 * @param pBuffer: pointer to the buffer that receives the data read from 00115 * the EEPROM. 00116 * @param ReadAddr: EEPROM's internal address to start reading from. 00117 * @param NumByteToRead: pointer to the variable holding number of bytes to 00118 * be read from the EEPROM. 00119 * 00120 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00121 * data are read from the EEPROM. Application should monitor this 00122 * variable in order know when the transfer is complete. 00123 * 00124 * @retval EEPROM_OK (0); if operation is correctly performed, else return value 00125 * different from EEPROM_OK (0); or the timeout user callback. 00126 */ 00127 uint32_t ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint16_t* NumByteToRead); 00128 00129 /** 00130 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00131 * 00132 * @note The number of bytes (combined to write start address); must not 00133 * cross the EEPROM page boundary. This function can only write into 00134 * the boundaries of an EEPROM page. 00135 * This function doesn't check on boundaries condition (in this driver 00136 * the function WriteBuffer(); which calls BSP_EEPROM_WritePage() is 00137 * responsible of checking on Page boundaries);. 00138 * 00139 * @param pBuffer: pointer to the buffer containing the data to be written to 00140 * the EEPROM. 00141 * @param WriteAddr: EEPROM's internal address to write to. 00142 * @param NumByteToWrite: pointer to the variable holding number of bytes to 00143 * be written into the EEPROM. 00144 * 00145 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00146 * data are written to the EEPROM. Application should monitor this 00147 * variable in order know when the transfer is complete. 00148 * 00149 * @note This function just configure the communication and enable the DMA 00150 * channel to transfer data. Meanwhile, the user application may perform 00151 * other tasks in parallel. 00152 * 00153 * @retval EEPROM_OK (0); if operation is correctly performed, else return value 00154 * different from EEPROM_OK (0); or the timeout user callback. 00155 */ 00156 uint32_t WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint8_t* NumByteToWrite); 00157 00158 /** 00159 * @brief Writes buffer of data to the I2C EEPROM. 00160 * @param pBuffer: pointer to the buffer containing the data to be written 00161 * to the EEPROM. 00162 * @param WriteAddr: EEPROM's internal address to write to. 00163 * @param NumByteToWrite: number of bytes to write to the EEPROM. 00164 * @retval EEPROM_OK (0); if operation is correctly performed, else return value 00165 * different from EEPROM_OK (0); or the timeout user callback. 00166 */ 00167 uint32_t WriteBuffer(uint8_t *pBuffer, uint16_t WriteAddr, uint16_t NumByteToWrite); 00168 00169 /** 00170 * @brief Wait for EEPROM Standby state. 00171 * 00172 * @note This function allows to wait and check that EEPROM has finished the 00173 * last operation. It is mostly used after Write operation: after receiving 00174 * the buffer to be written, the EEPROM may need additional time to actually 00175 * perform the write operation. During this time, it doesn't answer to 00176 * I2C packets addressed to it. Once the write operation is complete 00177 * the EEPROM responds to its address. 00178 * 00179 * @retval EEPROM_OK (0); if operation is correctly performed, else return value 00180 * different from EEPROM_OK (0); or the timeout user callback. 00181 */ 00182 uint32_t WaitEepromStandbyState(void); 00183 00184 private: 00185 00186 }; 00187 00188 #else 00189 #error "This class must be used with DISCO_F746NG board only." 00190 #endif // TARGET_DISCO_F746NG 00191 00192 #endif
Generated on Thu Jul 21 2022 01:47:05 by
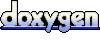