
AUDIO DISCO_F769NI audioloop basic example
Dependencies: BSP_DISCO_F769NI
main.cpp
00001 #include "mbed.h" 00002 #include "stm32f769i_discovery.h" 00003 #include "stm32f769i_discovery_audio.h" 00004 00005 static void CopyBuffer(int16_t *pbuffer1, int16_t *pbuffer2, uint16_t BufferSize); 00006 00007 #define SCRATCH_BUFF_SIZE 1024 00008 #define RECORD_BUFFER_SIZE 4096 00009 00010 typedef enum { 00011 BUFFER_OFFSET_NONE = 0, 00012 BUFFER_OFFSET_HALF = 1, 00013 BUFFER_OFFSET_FULL = 2, 00014 } BUFFER_StateTypeDef; 00015 00016 volatile uint32_t audio_rec_buffer_state = BUFFER_OFFSET_NONE; 00017 int32_t Scratch[SCRATCH_BUFF_SIZE]; 00018 00019 /* Buffer containing the PCM samples coming from the microphone */ 00020 int16_t RecordBuffer[RECORD_BUFFER_SIZE]; 00021 00022 /* Buffer used to stream the recorded PCM samples towards the audio codec. */ 00023 int16_t PlaybackBuffer[RECORD_BUFFER_SIZE]; 00024 00025 int main() 00026 { 00027 uint32_t audio_loop_back_init = RESET ; 00028 00029 printf("\n\nAUDIO LOOPBACK EXAMPLE FOR DISCO-F769NI START:\n"); 00030 00031 /* Initialize Audio Recorder with 4 channels to be used */ 00032 if (BSP_AUDIO_IN_Init(BSP_AUDIO_FREQUENCY_44K, DEFAULT_AUDIO_IN_BIT_RESOLUTION, 2*DEFAULT_AUDIO_IN_CHANNEL_NBR) == AUDIO_ERROR) { 00033 printf("BSP_AUDIO_IN_Init error\n"); 00034 } 00035 00036 /* Allocate scratch buffers */ 00037 if (BSP_AUDIO_IN_AllocScratch (Scratch, SCRATCH_BUFF_SIZE) == AUDIO_ERROR) { 00038 printf("BSP_AUDIO_IN_AllocScratch error\n"); 00039 } 00040 00041 /* Start Recording */ 00042 if (BSP_AUDIO_IN_Record((uint16_t*)&RecordBuffer[0], RECORD_BUFFER_SIZE) == AUDIO_ERROR) { 00043 printf("BSP_AUDIO_IN_Record error\n"); 00044 } 00045 uint8_t ChannelNumber = BSP_AUDIO_IN_GetChannelNumber(); 00046 00047 audio_rec_buffer_state = BUFFER_OFFSET_NONE; 00048 00049 while (1) { 00050 /* 1st or 2nd half of the record buffer ready for being copied to the playbakc buffer */ 00051 if(audio_rec_buffer_state != BUFFER_OFFSET_NONE) { 00052 /* Copy half of the record buffer to the playback buffer */ 00053 if(audio_rec_buffer_state == BUFFER_OFFSET_HALF) { 00054 CopyBuffer(&PlaybackBuffer[0], &RecordBuffer[0], RECORD_BUFFER_SIZE/2); 00055 if (audio_loop_back_init == RESET) { 00056 /* Initialize the audio device*/ 00057 if (BSP_AUDIO_OUT_Init(OUTPUT_DEVICE_HEADPHONE, 70, BSP_AUDIO_FREQUENCY_44K) == AUDIO_ERROR) { 00058 printf("BSP_AUDIO_OUT_Init error\n"); 00059 } 00060 if(ChannelNumber > 2) { 00061 BSP_AUDIO_OUT_SetAudioFrameSlot(CODEC_AUDIOFRAME_SLOT_0123); 00062 } else { 00063 BSP_AUDIO_OUT_SetAudioFrameSlot(CODEC_AUDIOFRAME_SLOT_02); 00064 } 00065 00066 /* Play the recorded buffer */ 00067 if (BSP_AUDIO_OUT_Play((uint16_t *) &PlaybackBuffer[0], 2*RECORD_BUFFER_SIZE) == AUDIO_ERROR) { 00068 printf("BSP_AUDIO_OUT_Play error\n"); 00069 } 00070 /* Audio device is initialized only once */ 00071 audio_loop_back_init = SET; 00072 } 00073 00074 00075 } else { /* if(audio_rec_buffer_state == BUFFER_OFFSET_FULL)*/ 00076 CopyBuffer(&PlaybackBuffer[RECORD_BUFFER_SIZE/2], &RecordBuffer[RECORD_BUFFER_SIZE/2], RECORD_BUFFER_SIZE/2); 00077 } 00078 00079 /* Wait for next data */ 00080 audio_rec_buffer_state = BUFFER_OFFSET_NONE; 00081 } 00082 } 00083 } 00084 00085 /*------------------------------------------------------------------------------------- 00086 Callbacks implementation: 00087 the callbacks API are defined __weak in the stm32f769i_discovery_audio.c file 00088 and their implementation should be done in the user code if they are needed. 00089 Below some examples of callback implementations. 00090 -------------------------------------------------------------------------------------*/ 00091 /** 00092 * @brief Manages the DMA Transfer complete interrupt. 00093 * @param None 00094 * @retval None 00095 */ 00096 void BSP_AUDIO_IN_TransferComplete_CallBack(void) 00097 { 00098 audio_rec_buffer_state = BUFFER_OFFSET_FULL; 00099 } 00100 00101 /** 00102 * @brief Manages the DMA Half Transfer complete interrupt. 00103 * @param None 00104 * @retval None 00105 */ 00106 void BSP_AUDIO_IN_HalfTransfer_CallBack(void) 00107 { 00108 audio_rec_buffer_state = BUFFER_OFFSET_HALF; 00109 } 00110 00111 /** 00112 * @brief Audio IN Error callback function. 00113 * @param None 00114 * @retval None 00115 */ 00116 void BSP_AUDIO_IN_Error_CallBack(void) 00117 { 00118 printf("BSP_AUDIO_IN_Error_CallBack\n"); 00119 } 00120 00121 00122 /** 00123 * @brief Copy content of pbuffer2 to pbuffer1 00124 * @param1 BufferOut 00125 * @param2 BufferIn 00126 * @param3 Size 00127 * @retval None 00128 */ 00129 static void CopyBuffer(int16_t *pbuffer1, int16_t *pbuffer2, uint16_t BufferSize) 00130 { 00131 uint32_t i = 0; 00132 for(i = 0; i < BufferSize; i++) { 00133 pbuffer1[i] = pbuffer2[i]; 00134 } 00135 }
Generated on Fri Jul 15 2022 01:52:54 by
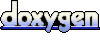