
Basic example showing how to use a M24LR64 EEPROM with the STM32746G_DISCOVERY board. This EEPROM can be found on the additional ANT7-M24LR-A daughter board.
Dependencies: BSP_DISCO_F746NG
main.cpp
00001 #include "mbed.h" 00002 #include "stm32746g_discovery_eeprom.h" 00003 00004 00005 #define BUFFER_SIZE ((uint32_t)32) 00006 #define WRITE_READ_ADDR ((uint32_t)0x0000) 00007 00008 int main() 00009 { 00010 // 12345678901234567890123456789012 00011 uint8_t WriteBuffer[BUFFER_SIZE + 1] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ012345"; 00012 uint8_t ReadBuffer[BUFFER_SIZE + 1]; 00013 uint16_t bytes_rd; 00014 00015 printf("\n\nEEPROM demo started\n"); 00016 printf("NB: you have to connect the RF EEPROM ANT7-M24LR-A to CN1 connector of STM32746G-Discovery board\n"); 00017 00018 // Check initialization 00019 if (BSP_EEPROM_Init() != EEPROM_OK) { 00020 error("Initialization FAILED\n"); 00021 } else { 00022 printf("Initialization PASSED\n"); 00023 } 00024 00025 // Write buffer 00026 if (BSP_EEPROM_WriteBuffer(WriteBuffer, WRITE_READ_ADDR, BUFFER_SIZE) != EEPROM_OK) { 00027 error("Write buffer FAILED\n"); 00028 } else { 00029 printf("Write buffer PASSED\n"); 00030 } 00031 00032 // Read buffer 00033 bytes_rd = BUFFER_SIZE; 00034 if (BSP_EEPROM_ReadBuffer(ReadBuffer, WRITE_READ_ADDR, &bytes_rd) != EEPROM_OK) { 00035 error("Read buffer FAILED\n"); 00036 } else { 00037 ReadBuffer[BUFFER_SIZE] = '\0'; 00038 printf("Read buffer PASSED\n"); 00039 printf("Buffer read = [%s]\n", ReadBuffer); 00040 printf("Bytes read = %d\n", bytes_rd); 00041 } 00042 }
Generated on Mon Jul 18 2022 04:26:37 by
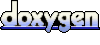