STM32L476G-Discovery board drivers V1.0.0
Dependents: DiscoLogger DISCO_L476VG_GlassLCD DISCO_L476VG_MicrophoneRecorder DISCO_L476VG_UART ... more
stm32l476g_discovery_idd.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_idd.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage the 00006 * Idd measurement driver for STM32L476G-Discovery board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2016 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include "stm32l476g_discovery_idd.h" 00023 00024 /** @addtogroup BSP 00025 * @{ 00026 */ 00027 00028 /** @addtogroup STM32L476G_DISCOVERY 00029 * @{ 00030 */ 00031 00032 /** @defgroup STM32L476G_DISCOVERY_IDD STM32L476G-DISCOVERY IDD 00033 * @brief This file includes the Idd driver for STM32L476G-DISCOVERY board. 00034 * It allows user to measure MCU Idd current on board, especially in 00035 * different low power modes. 00036 * @{ 00037 */ 00038 00039 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Defines Private Defines 00040 * @{ 00041 */ 00042 00043 /** 00044 * @} 00045 */ 00046 00047 00048 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Variables Private Variables 00049 * @{ 00050 */ 00051 static IDD_DrvTypeDef *IddDrv; 00052 00053 /** 00054 * @} 00055 */ 00056 00057 /** @defgroup STM32L476G_DISCOVERY_IDD_Private_Functions Private Functions 00058 * @{ 00059 */ 00060 00061 /** 00062 * @} 00063 */ 00064 00065 /** @defgroup STM32L476G_DISCOVERY_IDD_Exported_Functions Exported Functions 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief Configures IDD measurement component. 00071 * @retval IDD_OK if no problem during initialization 00072 */ 00073 uint8_t BSP_IDD_Init(void) 00074 { 00075 IDD_ConfigTypeDef iddconfig = {0}; 00076 uint8_t mfxstm32l152_id = 0; 00077 uint8_t ret = 0; 00078 00079 /* wake up mfx component in case it went to standby mode */ 00080 mfxstm32l152_idd_drv.WakeUp(IDD_I2C_ADDRESS); 00081 HAL_Delay(5); 00082 00083 /* Read ID and verify if the MFX is ready */ 00084 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00085 00086 if ((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00087 { 00088 /* Initialize the Idd driver structure */ 00089 IddDrv = &mfxstm32l152_idd_drv; 00090 00091 /* Initialize the Idd driver */ 00092 if (IddDrv->Init != NULL) 00093 { 00094 IddDrv->Init(IDD_I2C_ADDRESS); 00095 } 00096 00097 /* Configure Idd component with default values */ 00098 iddconfig.AmpliGain = DISCOVERY_IDD_AMPLI_GAIN; 00099 iddconfig.VddMin = DISCOVERY_IDD_VDD_MIN; 00100 iddconfig.Shunt0Value = DISCOVERY_IDD_SHUNT0_VALUE; 00101 iddconfig.Shunt1Value = DISCOVERY_IDD_SHUNT1_VALUE; 00102 iddconfig.Shunt2Value = DISCOVERY_IDD_SHUNT2_VALUE; 00103 iddconfig.Shunt3Value = 0; 00104 iddconfig.Shunt4Value = DISCOVERY_IDD_SHUNT4_VALUE; 00105 iddconfig.Shunt0StabDelay = DISCOVERY_IDD_SHUNT0_STABDELAY; 00106 iddconfig.Shunt1StabDelay = DISCOVERY_IDD_SHUNT1_STABDELAY; 00107 iddconfig.Shunt2StabDelay = DISCOVERY_IDD_SHUNT2_STABDELAY; 00108 iddconfig.Shunt3StabDelay = 0; 00109 iddconfig.Shunt4StabDelay = DISCOVERY_IDD_SHUNT4_STABDELAY; 00110 iddconfig.ShuntNbOnBoard = MFXSTM32L152_IDD_SHUNT_NB_4; 00111 iddconfig.ShuntNbUsed = MFXSTM32L152_IDD_SHUNT_NB_4; 00112 iddconfig.VrefMeasurement = MFXSTM32L152_IDD_VREF_AUTO_MEASUREMENT_ENABLE; 00113 iddconfig.Calibration = MFXSTM32L152_IDD_AUTO_CALIBRATION_ENABLE; 00114 iddconfig.PreDelayUnit = MFXSTM32L152_IDD_PREDELAY_20_MS; 00115 iddconfig.PreDelayValue = 0x7F; 00116 iddconfig.MeasureNb = 100; 00117 iddconfig.DeltaDelayUnit = MFXSTM32L152_IDD_DELTADELAY_0_5_MS; 00118 iddconfig.DeltaDelayValue = 10; 00119 BSP_IDD_Config(iddconfig); 00120 00121 ret = IDD_OK; 00122 } 00123 else 00124 { 00125 ret = IDD_ERROR; 00126 } 00127 00128 return ret; 00129 } 00130 00131 /** 00132 * @brief Unconfigures IDD measurement component. 00133 * @retval IDD_OK if no problem during deinitialization 00134 */ 00135 void BSP_IDD_DeInit(void) 00136 { 00137 if (IddDrv->DeInit != NULL) 00138 { 00139 IddDrv->DeInit(IDD_I2C_ADDRESS); 00140 } 00141 } 00142 00143 /** 00144 * @brief Reset Idd measurement component. 00145 * @retval None 00146 */ 00147 void BSP_IDD_Reset(void) 00148 { 00149 if (IddDrv->Reset != NULL) 00150 { 00151 IddDrv->Reset(IDD_I2C_ADDRESS); 00152 } 00153 } 00154 00155 /** 00156 * @brief Turn Idd measurement component in low power (standby/sleep) mode 00157 * @retval None 00158 */ 00159 void BSP_IDD_LowPower(void) 00160 { 00161 if (IddDrv->LowPower != NULL) 00162 { 00163 IddDrv->LowPower(IDD_I2C_ADDRESS); 00164 } 00165 } 00166 00167 /** 00168 * @brief Start Measurement campaign 00169 * @retval None 00170 */ 00171 void BSP_IDD_StartMeasure(void) 00172 { 00173 if (IddDrv->Start != NULL) 00174 { 00175 IddDrv->Start(IDD_I2C_ADDRESS); 00176 } 00177 } 00178 00179 /** 00180 * @brief Configure Idd component 00181 * @param IddConfig: structure of idd parameters 00182 * @retval None 00183 */ 00184 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig) 00185 { 00186 if (IddDrv->Config != NULL) 00187 { 00188 IddDrv->Config(IDD_I2C_ADDRESS, IddConfig); 00189 } 00190 } 00191 00192 /** 00193 * @brief Get Idd current value. 00194 * @param IddValue: Pointer on u32 to store Idd. Value unit is 10 nA. 00195 * @retval None 00196 */ 00197 void BSP_IDD_GetValue(uint32_t *IddValue) 00198 { 00199 if (IddDrv->GetValue != NULL) 00200 { 00201 IddDrv->GetValue(IDD_I2C_ADDRESS, IddValue); 00202 } 00203 } 00204 00205 /** 00206 * @brief Enable Idd interrupt that warn end of measurement 00207 * @retval None 00208 */ 00209 void BSP_IDD_EnableIT(void) 00210 { 00211 if (IddDrv->EnableIT != NULL) 00212 { 00213 IddDrv->EnableIT(IDD_I2C_ADDRESS); 00214 } 00215 } 00216 00217 /** 00218 * @brief Clear Idd interrupt that warn end of measurement 00219 * @retval None 00220 */ 00221 void BSP_IDD_ClearIT(void) 00222 { 00223 if (IddDrv->ClearIT != NULL) 00224 { 00225 IddDrv->ClearIT(IDD_I2C_ADDRESS); 00226 } 00227 } 00228 00229 /** 00230 * @brief Get Idd interrupt status 00231 * @retval status 00232 */ 00233 uint8_t BSP_IDD_GetITStatus(void) 00234 { 00235 if (IddDrv->GetITStatus != NULL) 00236 { 00237 return (IddDrv->GetITStatus(IDD_I2C_ADDRESS)); 00238 } 00239 else 00240 { 00241 return IDD_ERROR; 00242 } 00243 } 00244 00245 /** 00246 * @brief Disable Idd interrupt that warn end of measurement 00247 * @retval None 00248 */ 00249 void BSP_IDD_DisableIT(void) 00250 { 00251 if (IddDrv->DisableIT != NULL) 00252 { 00253 IddDrv->DisableIT(IDD_I2C_ADDRESS); 00254 } 00255 } 00256 00257 /** 00258 * @brief Get Error Code . 00259 * @retval Error code or error status 00260 */ 00261 uint8_t BSP_IDD_ErrorGetCode(void) 00262 { 00263 if (IddDrv->ErrorGetSrc != NULL) 00264 { 00265 if ((IddDrv->ErrorGetSrc(IDD_I2C_ADDRESS) & MFXSTM32L152_IDD_ERROR_SRC) != RESET) 00266 { 00267 if (IddDrv->ErrorGetCode != NULL) 00268 { 00269 return IddDrv->ErrorGetCode(IDD_I2C_ADDRESS); 00270 } 00271 else 00272 { 00273 return IDD_ERROR; 00274 } 00275 } 00276 else 00277 { 00278 return IDD_ERROR; 00279 } 00280 } 00281 else 00282 { 00283 return IDD_ERROR; 00284 } 00285 } 00286 00287 00288 /** 00289 * @brief Enable error interrupt that warn end of measurement 00290 * @retval None 00291 */ 00292 void BSP_IDD_ErrorEnableIT(void) 00293 { 00294 if (IddDrv->ErrorEnableIT != NULL) 00295 { 00296 IddDrv->ErrorEnableIT(IDD_I2C_ADDRESS); 00297 } 00298 } 00299 00300 /** 00301 * @brief Clear Error interrupt that warn end of measurement 00302 * @retval None 00303 */ 00304 void BSP_IDD_ErrorClearIT(void) 00305 { 00306 if (IddDrv->ErrorClearIT != NULL) 00307 { 00308 IddDrv->ErrorClearIT(IDD_I2C_ADDRESS); 00309 } 00310 } 00311 00312 /** 00313 * @brief Get Error interrupt status 00314 * @retval Status 00315 */ 00316 uint8_t BSP_IDD_ErrorGetITStatus(void) 00317 { 00318 if (IddDrv->ErrorGetITStatus != NULL) 00319 { 00320 return (IddDrv->ErrorGetITStatus(IDD_I2C_ADDRESS)); 00321 } 00322 else 00323 { 00324 return 0; 00325 } 00326 } 00327 00328 /** 00329 * @brief Disable Error interrupt 00330 * @retval None 00331 */ 00332 void BSP_IDD_ErrorDisableIT(void) 00333 { 00334 if (IddDrv->ErrorDisableIT != NULL) 00335 { 00336 IddDrv->ErrorDisableIT(IDD_I2C_ADDRESS); 00337 } 00338 } 00339 00340 /** 00341 * @brief Wake up Idd measurement component. 00342 * @retval None 00343 */ 00344 void BSP_IDD_WakeUp(void) 00345 { 00346 if (IddDrv->WakeUp != NULL) 00347 { 00348 IddDrv->WakeUp(IDD_I2C_ADDRESS); 00349 } 00350 } 00351 00352 /** 00353 * @} 00354 */ 00355 00356 /** 00357 * @} 00358 */ 00359 00360 /** 00361 * @} 00362 */ 00363 00364 /** 00365 * @} 00366 */ 00367 00368 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00369
Generated on Tue Jul 12 2022 18:37:21 by
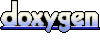