BSP files for STM32H747I-Discovery Copy from ST Cube delivery
Dependents: DISCO_H747I_LCD_demo DISCO_H747I_AUDIO_demo
This file includes the driver for Liquid Crystal Display (LCD) module mounted on STM32H747I_DISCOVERY board. More...
Go to the source code of this file.
Functions | |
static void | DrawChar (uint16_t Xpos, uint16_t Ypos, const uint8_t *c) |
Draws a character on LCD. | |
static void | FillTriangle (uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) |
Fills a triangle (between 3 points). | |
static void | LL_FillBuffer (uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) |
Fills a buffer. | |
static void | LL_ConvertLineToARGB8888 (void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) |
Converts a line to an ARGB8888 pixel format. | |
static uint16_t | LCD_IO_GetID (void) |
Returns the ID of connected screen by checking the HDMI (adv7533 component) ID or LCD DSI (via TS ID) ID. | |
uint8_t | BSP_LCD_Init (void) |
Initializes the DSI LCD. | |
uint8_t | BSP_LCD_InitEx (LCD_OrientationTypeDef orientation) |
Initializes the DSI LCD. | |
uint8_t | BSP_LCD_HDMIInitEx (uint8_t format) |
Initializes the DSI for HDMI monitor. | |
void | BSP_LCD_Reset (void) |
BSP LCD Reset Hw reset the LCD DSI activating its XRES signal (active low for some time) and deactivating it later. | |
uint32_t | BSP_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_LCD_GetYSize (void) |
Gets the LCD Y size. | |
void | BSP_LCD_SetXSize (uint32_t imageWidthPixels) |
Set the LCD X size. | |
void | BSP_LCD_SetYSize (uint32_t imageHeightPixels) |
Set the LCD Y size. | |
void | BSP_LCD_LayerDefaultInit (uint16_t LayerIndex, uint32_t FB_Address) |
Initializes the LCD layers. | |
void | BSP_LCD_SelectLayer (uint32_t LayerIndex) |
Selects the LCD Layer. | |
void | BSP_LCD_SetLayerVisible (uint32_t LayerIndex, FunctionalState State) |
Sets an LCD Layer visible. | |
void | BSP_LCD_SetTransparency (uint32_t LayerIndex, uint8_t Transparency) |
Configures the transparency. | |
void | BSP_LCD_SetLayerAddress (uint32_t LayerIndex, uint32_t Address) |
Sets an LCD layer frame buffer address. | |
void | BSP_LCD_SetLayerWindow (uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets display window. | |
void | BSP_LCD_SetColorKeying (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color keying. | |
void | BSP_LCD_ResetColorKeying (uint32_t LayerIndex) |
Disables the color keying. | |
void | BSP_LCD_SetTextColor (uint32_t Color) |
Sets the LCD text color. | |
uint32_t | BSP_LCD_GetTextColor (void) |
Gets the LCD text color. | |
void | BSP_LCD_SetBackColor (uint32_t Color) |
Sets the LCD background color. | |
uint32_t | BSP_LCD_GetBackColor (void) |
Gets the LCD background color. | |
void | BSP_LCD_SetFont (sFONT *fonts) |
Sets the LCD text font. | |
sFONT * | BSP_LCD_GetFont (void) |
Gets the LCD text font. | |
uint32_t | BSP_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads an LCD pixel. | |
void | BSP_LCD_Clear (uint32_t Color) |
Clears the whole currently active layer of LTDC. | |
void | BSP_LCD_ClearStringLine (uint32_t Line) |
Clears the selected line in currently active layer. | |
void | BSP_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character in currently active layer. | |
void | BSP_LCD_DisplayStringAt (uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) |
Displays characters in currently active layer. | |
void | BSP_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays a maximum of 60 characters on the LCD. | |
void | BSP_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws an horizontal line in currently active layer. | |
void | BSP_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws a vertical line in currently active layer. | |
void | BSP_LCD_DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) |
Draws an uni-line (between two points) in currently active layer. | |
void | BSP_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a rectangle in currently active layer. | |
void | BSP_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a circle in currently active layer. | |
void | BSP_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Draws an poly-line (between many points) in currently active layer. | |
void | BSP_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws an ellipse on LCD in currently active layer. | |
void | BSP_LCD_DrawBitmap (uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) |
Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer. | |
void | BSP_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a full rectangle in currently active layer. | |
void | BSP_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a full circle in currently active layer. | |
void | BSP_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Draws a full poly-line (between many points) in currently active layer. | |
void | BSP_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws a full ellipse in currently active layer. | |
void | BSP_LCD_DisplayOn (void) |
Switch back on the display if was switched off by previous call of BSP_LCD_DisplayOff(). | |
void | BSP_LCD_DisplayOff (void) |
Switch Off the display. | |
void | BSP_LCD_SetBrightness (uint8_t BrightnessValue) |
Set the brightness value. | |
void | DSI_IO_WriteCmd (uint32_t NbrParams, uint8_t *pParams) |
DCS or Generic short/long write command. | |
__weak void | BSP_LCD_MspDeInit (void) |
De-Initializes the BSP LCD Msp Application can surcharge if needed this function implementation. | |
__weak void | BSP_LCD_MspInit (void) |
Initialize the BSP LCD Msp. | |
void | BSP_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) |
Draws a pixel on LCD. | |
Variables | |
HDMI_FormatTypeDef | HDMI_Format [2] |
DSI timming used for different HDMI resolution (720x480 and 720x576) | |
HDMI_DSIPacketTypeDef | HDMI_DSIPacket [2] |
DSI packet size used for different HDMI resolution (720x480 and 720x576) | |
HDMI_PLLConfigTypeDef | HDMI_PLLConfig [4] |
LTDC PLL settings used for different HDMI resolution (720x480 and 720x576) | |
static uint32_t | ActiveLayer = LTDC_ACTIVE_LAYER_BACKGROUND |
Default Active LTDC Layer in which drawing is made is LTDC Layer Background. | |
static LCD_DrawPropTypeDef | DrawProp [LTDC_MAX_LAYER_NUMBER] |
Current Drawing Layer properties variable. |
Detailed Description
This file includes the driver for Liquid Crystal Display (LCD) module mounted on STM32H747I_DISCOVERY board.
- Attention:
© Copyright (c) 2019 STMicroelectronics. All rights reserved.
This software component is licensed by ST under BSD 3-Clause license, the "License"; You may not use this file except in compliance with the License. You may obtain a copy of the License at: opensource.org/licenses/BSD-3-Clause
Definition in file stm32h747i_discovery_lcd.c.
Generated on Tue Jul 12 2022 18:45:42 by
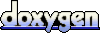