BSP files for STM32H747I-Discovery Copy from ST Cube delivery
Dependents: DISCO_H747I_LCD_demo DISCO_H747I_AUDIO_demo
stm32h747i_discovery_camera.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h747i_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Camera modules mounted on 00006 * STM32H747I-DISCOVERY board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2019 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* File Info: ------------------------------------------------------------------ 00022 User NOTES 00023 1. How to use this driver: 00024 -------------------------- 00025 - This driver is used to drive the camera. 00026 - The OV9655 component driver MUST be included with this driver. 00027 00028 2. Driver description: 00029 --------------------- 00030 + Initialization steps: 00031 o Initialize the camera using the BSP_CAMERA_Init() function. 00032 o Start the camera capture/snapshot using the CAMERA_Start() function. 00033 o Suspend, resume or stop the camera capture using the following functions: 00034 - BSP_CAMERA_Suspend() 00035 - BSP_CAMERA_Resume() 00036 - BSP_CAMERA_Stop() 00037 00038 + Options 00039 o Increase or decrease on the fly the brightness and/or contrast 00040 using the following function: 00041 - BSP_CAMERA_ContrastBrightnessConfig 00042 o Add a special effect on the fly using the following functions: 00043 - BSP_CAMERA_BlackWhiteConfig() 00044 - BSP_CAMERA_ColorEffectConfig() 00045 00046 ------------------------------------------------------------------------------*/ 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32h747i_discovery_camera.h" 00050 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32H747I_DISCOVERY 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32H747I_DISCOVERY_CAMERA STM32H747I_DISCOVERY_CAMERA 00061 * @{ 00062 */ 00063 00064 /** @defgroup STM32H747I_DISCOVERY_CAMERA_Exported_Variables Exported Variables 00065 * @{ 00066 */ 00067 DCMI_HandleTypeDef hdcmi_discovery; 00068 CAMERA_DrvTypeDef *camera_drv; 00069 /** 00070 * @} 00071 */ 00072 00073 /** @defgroup STM32H747I_DISCOVERY_CAMERA_Private_Variables Private Variables 00074 * @{ 00075 */ 00076 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00077 static uint32_t CameraCurrentResolution; 00078 00079 /* Camera image rotation on LCD Displayed frame buffer */ 00080 uint32_t CameraRotation = CAMERA_ROTATION_INVALID; 00081 00082 /* Camera module I2C HW address */ 00083 static uint32_t CameraHwAddress; 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32H747I_DISCOVERY_CAMERA_Private_FunctionPrototypes Private FunctionPrototypes 00089 * @{ 00090 */ 00091 static uint32_t GetSize(uint32_t Resolution); 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32H747I_DISCOVERY_CAMERA_Exported_Functions Exported Functions 00097 * @{ 00098 */ 00099 00100 /** 00101 * @brief Set Camera image rotation on LCD Displayed frame buffer. 00102 * @param rotation : uint32_t rotation of camera image in preview buffer sent to LCD 00103 * need to be of type Camera_ImageRotationTypeDef 00104 * @retval Camera status 00105 */ 00106 uint8_t BSP_CAMERA_SetRotation(uint32_t rotation) 00107 { 00108 uint8_t status = CAMERA_ERROR; 00109 00110 if(rotation < CAMERA_ROTATION_INVALID) 00111 { 00112 /* Set Camera image rotation on LCD Displayed frame buffer */ 00113 CameraRotation = rotation; 00114 status = CAMERA_OK; 00115 } 00116 00117 return status; 00118 } 00119 00120 /** 00121 * @brief Get Camera image rotation on LCD Displayed frame buffer. 00122 * @retval rotation : uint32_t value of type Camera_ImageRotationTypeDef 00123 */ 00124 uint32_t BSP_CAMERA_GetRotation(void) 00125 { 00126 return(CameraRotation); 00127 } 00128 00129 /** 00130 * @brief Initializes the camera. 00131 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00132 * naming QQVGA, QVGA, VGA ... 00133 * @retval Camera status 00134 */ 00135 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00136 { 00137 DCMI_HandleTypeDef *phdcmi; 00138 uint8_t status = CAMERA_ERROR; 00139 00140 /* Get the DCMI handle structure */ 00141 phdcmi = &hdcmi_discovery; 00142 00143 /*** Configures the DCMI to interface with the camera module ***/ 00144 /* DCMI configuration */ 00145 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00146 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_LOW; 00147 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00148 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00149 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00150 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00151 phdcmi->Instance = DCMI; 00152 00153 /* Power up camera */ 00154 BSP_CAMERA_PwrUp(); 00155 00156 /* Read ID of Camera module via I2C */ 00157 if(ov9655_ReadID(CAMERA_I2C_ADDRESS) == OV9655_ID) 00158 { 00159 /* Initialize the camera driver structure */ 00160 camera_drv = &ov9655_drv; 00161 CameraHwAddress = CAMERA_I2C_ADDRESS; 00162 00163 /* DCMI Initialization */ 00164 BSP_CAMERA_MspInit(&hdcmi_discovery, NULL); 00165 HAL_DCMI_Init(phdcmi); 00166 00167 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00168 if (Resolution == CAMERA_R480x272) 00169 { /* For 480x272 resolution, the OV9655 sensor is set to VGA resolution 00170 * as OV9655 doesn't supports 480x272 resolution, 00171 * then DCMI is configured to output a 480x272 cropped window */ 00172 camera_drv->Init(CameraHwAddress, CAMERA_R640x480); 00173 HAL_DCMI_ConfigCROP(phdcmi, /* Crop in the middle of the VGA picture */ 00174 (CAMERA_VGA_RES_X - CAMERA_480x272_RES_X)/2, 00175 (CAMERA_VGA_RES_Y - CAMERA_480x272_RES_Y)/2, 00176 (CAMERA_480x272_RES_X * 2) - 1, 00177 CAMERA_480x272_RES_Y - 1); 00178 HAL_DCMI_EnableCROP(phdcmi); 00179 } 00180 else 00181 { 00182 camera_drv->Init(CameraHwAddress, Resolution); 00183 HAL_DCMI_DisableCROP(phdcmi); 00184 } 00185 00186 CameraCurrentResolution = Resolution; 00187 00188 /* Return CAMERA_OK status */ 00189 status = CAMERA_OK; 00190 } 00191 else 00192 { 00193 /* Return CAMERA_NOT_SUPPORTED status */ 00194 status = CAMERA_NOT_SUPPORTED; 00195 } 00196 00197 return status; 00198 } 00199 00200 00201 /** 00202 * @brief DeInitializes the camera. 00203 * @retval Camera status 00204 */ 00205 uint8_t BSP_CAMERA_DeInit(void) 00206 { 00207 hdcmi_discovery.Instance = DCMI; 00208 00209 HAL_DCMI_DeInit(&hdcmi_discovery); 00210 BSP_CAMERA_MspDeInit(&hdcmi_discovery, NULL); 00211 return CAMERA_OK; 00212 } 00213 00214 /** 00215 * @brief Starts the camera capture in continuous mode. 00216 * @param buff: pointer to the camera output buffer 00217 * @retval None 00218 */ 00219 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00220 { 00221 /* Start the camera capture */ 00222 HAL_DCMI_Start_DMA(&hdcmi_discovery, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00223 } 00224 00225 /** 00226 * @brief Starts the camera capture in snapshot mode. 00227 * @param buff: pointer to the camera output buffer 00228 * @retval None 00229 */ 00230 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00231 { 00232 /* Start the camera capture */ 00233 HAL_DCMI_Start_DMA(&hdcmi_discovery, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00234 } 00235 00236 /** 00237 * @brief Suspend the CAMERA capture 00238 * @retval None 00239 */ 00240 void BSP_CAMERA_Suspend(void) 00241 { 00242 /* Suspend the Camera Capture */ 00243 HAL_DCMI_Suspend(&hdcmi_discovery); 00244 } 00245 00246 /** 00247 * @brief Resume the CAMERA capture 00248 * @retval None 00249 */ 00250 void BSP_CAMERA_Resume(void) 00251 { 00252 /* Start the Camera Capture */ 00253 HAL_DCMI_Resume(&hdcmi_discovery); 00254 } 00255 00256 /** 00257 * @brief Stop the CAMERA capture 00258 * @retval Camera status 00259 */ 00260 uint8_t BSP_CAMERA_Stop(void) 00261 { 00262 uint8_t status = CAMERA_ERROR; 00263 00264 if(HAL_DCMI_Stop(&hdcmi_discovery) == HAL_OK) 00265 { 00266 status = CAMERA_OK; 00267 } 00268 00269 /* Set Camera in Power Down */ 00270 BSP_CAMERA_PwrDown(); 00271 00272 return status; 00273 } 00274 00275 /** 00276 * @brief CANERA power up 00277 * @retval None 00278 */ 00279 void BSP_CAMERA_PwrUp(void) 00280 { 00281 GPIO_InitTypeDef gpio_init_structure; 00282 00283 /* Enable GPIO clock */ 00284 __HAL_RCC_GPIOJ_CLK_ENABLE(); 00285 00286 /*** Configure the GPIO ***/ 00287 /* Configure DCMI GPIO as alternate function */ 00288 gpio_init_structure.Pin = GPIO_PIN_14; 00289 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00290 gpio_init_structure.Pull = GPIO_NOPULL; 00291 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00292 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 00293 00294 /* De-assert the camera POWER_DOWN pin (active high) */ 00295 HAL_GPIO_WritePin(GPIOJ, GPIO_PIN_14, GPIO_PIN_RESET); 00296 00297 HAL_Delay(3); /* POWER_DOWN de-asserted during 3ms */ 00298 } 00299 00300 /** 00301 * @brief CAMERA power down 00302 * @retval None 00303 */ 00304 void BSP_CAMERA_PwrDown(void) 00305 { 00306 GPIO_InitTypeDef gpio_init_structure; 00307 00308 /* Enable GPIO clock */ 00309 __HAL_RCC_GPIOJ_CLK_ENABLE(); 00310 00311 /*** Configure the GPIO ***/ 00312 /* Configure DCMI GPIO as alternate function */ 00313 gpio_init_structure.Pin = GPIO_PIN_14; 00314 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00315 gpio_init_structure.Pull = GPIO_NOPULL; 00316 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00317 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 00318 00319 /* Assert the camera POWER_DOWN pin (active high) */ 00320 HAL_GPIO_WritePin(GPIOJ, GPIO_PIN_14, GPIO_PIN_SET); 00321 } 00322 00323 /** 00324 * @brief Configures the camera contrast and brightness. 00325 * @param contrast_level: Contrast level 00326 * This parameter can be one of the following values: 00327 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00328 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00329 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00330 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00331 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00332 * @param brightness_level: Contrast level 00333 * This parameter can be one of the following values: 00334 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00335 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00336 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00337 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00338 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00339 */ 00340 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00341 { 00342 if(camera_drv->Config != NULL) 00343 { 00344 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00345 } 00346 } 00347 00348 /** 00349 * @brief Configures the camera white balance. 00350 * @param Mode: black_white mode 00351 * This parameter can be one of the following values: 00352 * @arg CAMERA_BLACK_WHITE_BW 00353 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00354 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00355 * @arg CAMERA_BLACK_WHITE_NORMAL 00356 */ 00357 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00358 { 00359 if(camera_drv->Config != NULL) 00360 { 00361 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00362 } 00363 } 00364 00365 /** 00366 * @brief Configures the camera color effect. 00367 * @param Effect: Color effect 00368 * This parameter can be one of the following values: 00369 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00370 * @arg CAMERA_COLOR_EFFECT_BLUE 00371 * @arg CAMERA_COLOR_EFFECT_GREEN 00372 * @arg CAMERA_COLOR_EFFECT_RED 00373 * @retval None 00374 */ 00375 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00376 { 00377 if(camera_drv->Config != NULL) 00378 { 00379 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00380 } 00381 } 00382 00383 /** 00384 * @brief Get the capture size in pixels unit. 00385 * @param Resolution: the current resolution. 00386 * @retval capture size in pixels unit. 00387 */ 00388 static uint32_t GetSize(uint32_t Resolution) 00389 { 00390 uint32_t size = 0; 00391 00392 /* Get capture size */ 00393 switch (Resolution) 00394 { 00395 case CAMERA_R160x120: 00396 { 00397 size = 0x2580; 00398 } 00399 break; 00400 case CAMERA_R320x240: 00401 { 00402 size = 0x9600; 00403 } 00404 break; 00405 case CAMERA_R480x272: 00406 { 00407 size = 0xFF00; 00408 } 00409 break; 00410 case CAMERA_R640x480: 00411 { 00412 size = 0x25800; 00413 } 00414 break; 00415 default: 00416 { 00417 break; 00418 } 00419 } 00420 00421 return size; 00422 } 00423 00424 /** 00425 * @brief Initializes the DCMI MSP. 00426 * @param hdcmi: HDMI handle 00427 * @param Params : pointer on additional configuration parameters, can be NULL. 00428 * @retval None 00429 */ 00430 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00431 { 00432 static DMA_HandleTypeDef hdma_handler; 00433 GPIO_InitTypeDef gpio_init_structure; 00434 00435 /*** Enable peripherals and GPIO clocks ***/ 00436 /* Enable DCMI clock */ 00437 __HAL_RCC_DCMI_CLK_ENABLE(); 00438 00439 /* Enable DMA clock */ 00440 CAMERA_DCMI_DMAx_CLK_ENABLE(); 00441 00442 /* Enable GPIO clocks */ 00443 __HAL_RCC_GPIOA_CLK_ENABLE(); 00444 __HAL_RCC_GPIOB_CLK_ENABLE(); 00445 __HAL_RCC_GPIOC_CLK_ENABLE(); 00446 __HAL_RCC_GPIOD_CLK_ENABLE(); 00447 __HAL_RCC_GPIOG_CLK_ENABLE(); 00448 00449 /*** Configure the GPIO ***/ 00450 /* Configure DCMI GPIO as alternate function */ 00451 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00452 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00453 gpio_init_structure.Pull = GPIO_PULLUP; 00454 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00455 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00456 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00457 00458 gpio_init_structure.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9; 00459 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00460 gpio_init_structure.Pull = GPIO_PULLUP; 00461 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00462 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00463 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00464 00465 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_11; 00466 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00467 gpio_init_structure.Pull = GPIO_PULLUP; 00468 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00469 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00470 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00471 00472 gpio_init_structure.Pin = GPIO_PIN_3; 00473 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00474 gpio_init_structure.Pull = GPIO_PULLUP; 00475 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00476 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00477 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00478 00479 gpio_init_structure.Pin = GPIO_PIN_10; 00480 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00481 gpio_init_structure.Pull = GPIO_PULLUP; 00482 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00483 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00484 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00485 00486 /*** Configure the DMA ***/ 00487 /* Set the parameters to be configured */ 00488 hdma_handler.Init.Request = DMA_REQUEST_DCMI; 00489 hdma_handler.Init.Direction = DMA_PERIPH_TO_MEMORY; 00490 hdma_handler.Init.PeriphInc = DMA_PINC_DISABLE; 00491 hdma_handler.Init.MemInc = DMA_MINC_ENABLE; 00492 hdma_handler.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00493 hdma_handler.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00494 hdma_handler.Init.Mode = DMA_CIRCULAR; 00495 hdma_handler.Init.Priority = DMA_PRIORITY_HIGH; 00496 hdma_handler.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00497 hdma_handler.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00498 hdma_handler.Init.MemBurst = DMA_MBURST_SINGLE; 00499 hdma_handler.Init.PeriphBurst = DMA_PBURST_SINGLE; 00500 00501 hdma_handler.Instance = CAMERA_DCMI_DMAx_STREAM; 00502 00503 /* Associate the initialized DMA handle to the DCMI handle */ 00504 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_handler); 00505 00506 /*** Configure the NVIC for DCMI and DMA ***/ 00507 /* NVIC configuration for DCMI transfer complete interrupt */ 00508 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00509 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00510 00511 /* NVIC configuration for DMA transfer complete interrupt */ 00512 HAL_NVIC_SetPriority(CAMERA_DCMI_DMAx_IRQ, 0x0F, 0); 00513 HAL_NVIC_EnableIRQ(CAMERA_DCMI_DMAx_IRQ); 00514 00515 /* Configure the DMA stream */ 00516 HAL_DMA_Init(hdcmi->DMA_Handle); 00517 } 00518 00519 /** 00520 * @brief DeInitializes the DCMI MSP. 00521 * @param hdcmi: HDMI handle 00522 * @param Params : pointer on additional configuration parameters, can be NULL. 00523 * @retval None 00524 */ 00525 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00526 { 00527 /* Disable NVIC for DCMI transfer complete interrupt */ 00528 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00529 00530 /* Disable NVIC for DMA2 transfer complete interrupt */ 00531 HAL_NVIC_DisableIRQ(CAMERA_DCMI_DMAx_IRQ); 00532 00533 /* Configure the DMA stream */ 00534 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00535 00536 /* Disable DCMI clock */ 00537 __HAL_RCC_DCMI_CLK_DISABLE(); 00538 00539 /* GPIO pins clock and DMA clock can be shut down in the application 00540 by surcharging this __weak function */ 00541 } 00542 00543 /** 00544 * @brief Line event callback 00545 * @param hdcmi: pointer to the DCMI handle 00546 * @retval None 00547 */ 00548 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00549 { 00550 BSP_CAMERA_LineEventCallback(); 00551 } 00552 00553 /** 00554 * @brief Line Event callback. 00555 * @retval None 00556 */ 00557 __weak void BSP_CAMERA_LineEventCallback(void) 00558 { 00559 /* NOTE : This function Should not be modified, when the callback is needed, 00560 the HAL_DCMI_LineEventCallback could be implemented in the user file 00561 */ 00562 } 00563 00564 /** 00565 * @brief VSYNC event callback 00566 * @param hdcmi: pointer to the DCMI handle 00567 * @retval None 00568 */ 00569 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00570 { 00571 BSP_CAMERA_VsyncEventCallback(); 00572 } 00573 00574 /** 00575 * @brief VSYNC Event callback. 00576 * @retval None 00577 */ 00578 __weak void BSP_CAMERA_VsyncEventCallback(void) 00579 { 00580 /* NOTE : This function Should not be modified, when the callback is needed, 00581 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00582 */ 00583 } 00584 00585 /** 00586 * @brief Frame event callback 00587 * @param hdcmi: pointer to the DCMI handle 00588 * @retval None 00589 */ 00590 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00591 { 00592 BSP_CAMERA_FrameEventCallback(); 00593 } 00594 00595 /** 00596 * @brief Frame Event callback. 00597 * @retval None 00598 */ 00599 __weak void BSP_CAMERA_FrameEventCallback(void) 00600 { 00601 /* NOTE : This function Should not be modified, when the callback is needed, 00602 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00603 */ 00604 } 00605 00606 /** 00607 * @brief Error callback 00608 * @param hdcmi: pointer to the DCMI handle 00609 * @retval None 00610 */ 00611 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00612 { 00613 BSP_CAMERA_ErrorCallback(); 00614 } 00615 00616 /** 00617 * @brief Error callback. 00618 * @retval None 00619 */ 00620 __weak void BSP_CAMERA_ErrorCallback(void) 00621 { 00622 /* NOTE : This function Should not be modified, when the callback is needed, 00623 the HAL_DCMI_ErrorCallback could be implemented in the user file 00624 */ 00625 } 00626 00627 /** 00628 * @} 00629 */ 00630 00631 /** 00632 * @} 00633 */ 00634 00635 /** 00636 * @} 00637 */ 00638 00639 /** 00640 * @} 00641 */ 00642 00643 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 18:45:41 by
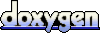