BSP files for STM32H747I-Discovery Copy from ST Cube delivery
Dependents: DISCO_H747I_LCD_demo DISCO_H747I_AUDIO_demo
otm8009a.h
00001 /** 00002 ****************************************************************************** 00003 * @file otm8009a.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 27-January-2017 00007 * @brief This file contains all the constants parameters for the OTM8009A 00008 * which is the LCD Driver for KoD KM-040TMP-02-0621 (WVGA) 00009 * DSI LCD Display. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __OTM8009A_H 00042 #define __OTM8009A_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include <stdint.h> 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup Components 00055 * @{ 00056 */ 00057 00058 /** @addtogroup otm8009a 00059 * @{ 00060 */ 00061 00062 /** @addtogroup OTM8009A_Exported_Variables 00063 * @{ 00064 */ 00065 00066 #if defined ( __GNUC__ ) 00067 #ifndef __weak 00068 #define __weak __attribute__((weak)) 00069 #endif /* __weak */ 00070 #endif /* __GNUC__ */ 00071 00072 /** 00073 * @brief LCD_OrientationTypeDef 00074 * Possible values of Display Orientation 00075 */ 00076 #define OTM8009A_ORIENTATION_PORTRAIT ((uint32_t)0x00) /* Portrait orientation choice of LCD screen */ 00077 #define OTM8009A_ORIENTATION_LANDSCAPE ((uint32_t)0x01) /* Landscape orientation choice of LCD screen */ 00078 00079 /** 00080 * @brief Possible values of 00081 * pixel data format (ie color coding) transmitted on DSI Data lane in DSI packets 00082 */ 00083 #define OTM8009A_FORMAT_RGB888 ((uint32_t)0x00) /* Pixel format chosen is RGB888 : 24 bpp */ 00084 #define OTM8009A_FORMAT_RBG565 ((uint32_t)0x02) /* Pixel format chosen is RGB565 : 16 bpp */ 00085 00086 /** 00087 * @brief otm8009a_480x800 Size 00088 */ 00089 00090 /* Width and Height in Portrait mode */ 00091 #define OTM8009A_480X800_WIDTH ((uint16_t)480) /* LCD PIXEL WIDTH */ 00092 #define OTM8009A_480X800_HEIGHT ((uint16_t)800) /* LCD PIXEL HEIGHT */ 00093 00094 /* Width and Height in Landscape mode */ 00095 #define OTM8009A_800X480_WIDTH ((uint16_t)800) /* LCD PIXEL WIDTH */ 00096 #define OTM8009A_800X480_HEIGHT ((uint16_t)480) /* LCD PIXEL HEIGHT */ 00097 00098 /** 00099 * @brief OTM8009A_480X800 Timing parameters for Portrait orientation mode 00100 */ 00101 #define OTM8009A_480X800_HSYNC ((uint16_t)2) /* Horizontal synchronization */ 00102 #define OTM8009A_480X800_HBP ((uint16_t)34) /* Horizontal back porch */ 00103 #define OTM8009A_480X800_HFP ((uint16_t)34) /* Horizontal front porch */ 00104 #define OTM8009A_480X800_VSYNC ((uint16_t)1) /* Vertical synchronization */ 00105 #define OTM8009A_480X800_VBP ((uint16_t)15) /* Vertical back porch */ 00106 #define OTM8009A_480X800_VFP ((uint16_t)16) /* Vertical front porch */ 00107 00108 /** 00109 * @brief OTM8009A_800X480 Timing parameters for Landscape orientation mode 00110 * Same values as for Portrait mode in fact. 00111 */ 00112 #define OTM8009A_800X480_HSYNC OTM8009A_480X800_VSYNC /* Horizontal synchronization */ 00113 #define OTM8009A_800X480_HBP OTM8009A_480X800_VBP /* Horizontal back porch */ 00114 #define OTM8009A_800X480_HFP OTM8009A_480X800_VFP /* Horizontal front porch */ 00115 #define OTM8009A_800X480_VSYNC OTM8009A_480X800_HSYNC /* Vertical synchronization */ 00116 #define OTM8009A_800X480_VBP OTM8009A_480X800_HBP /* Vertical back porch */ 00117 #define OTM8009A_800X480_VFP OTM8009A_480X800_HFP /* Vertical front porch */ 00118 00119 00120 /* List of OTM8009A used commands */ 00121 /* Detailed in OTM8009A Data Sheet 'DATA_SHEET_OTM8009A_V0 92.pdf' */ 00122 /* Version of 14 June 2012 */ 00123 #define OTM8009A_CMD_NOP 0x00 /* NOP command */ 00124 #define OTM8009A_CMD_SWRESET 0x01 /* Sw reset command */ 00125 #define OTM8009A_CMD_RDDMADCTL 0x0B /* Read Display MADCTR command : read memory display access ctrl */ 00126 #define OTM8009A_CMD_RDDCOLMOD 0x0C /* Read Display pixel format */ 00127 #define OTM8009A_CMD_SLPIN 0x10 /* Sleep In command */ 00128 #define OTM8009A_CMD_SLPOUT 0x11 /* Sleep Out command */ 00129 #define OTM8009A_CMD_PTLON 0x12 /* Partial mode On command */ 00130 00131 #define OTM8009A_CMD_DISPOFF 0x28 /* Display Off command */ 00132 #define OTM8009A_CMD_DISPON 0x29 /* Display On command */ 00133 00134 #define OTM8009A_CMD_CASET 0x2A /* Column address set command */ 00135 #define OTM8009A_CMD_PASET 0x2B /* Page address set command */ 00136 00137 #define OTM8009A_CMD_RAMWR 0x2C /* Memory (GRAM) write command */ 00138 #define OTM8009A_CMD_RAMRD 0x2E /* Memory (GRAM) read command */ 00139 00140 #define OTM8009A_CMD_PLTAR 0x30 /* Partial area command (4 parameters) */ 00141 00142 #define OTM8009A_CMD_TEOFF 0x34 /* Tearing Effect Line Off command : command with no parameter */ 00143 00144 #define OTM8009A_CMD_TEEON 0x35 /* Tearing Effect Line On command : command with 1 parameter 'TELOM' */ 00145 00146 /* Parameter TELOM : Tearing Effect Line Output Mode : possible values */ 00147 #define OTM8009A_TEEON_TELOM_VBLANKING_INFO_ONLY 0x00 00148 #define OTM8009A_TEEON_TELOM_VBLANKING_AND_HBLANKING_INFO 0x01 00149 00150 #define OTM8009A_CMD_MADCTR 0x36 /* Memory Access write control command */ 00151 00152 /* Possible used values of MADCTR */ 00153 #define OTM8009A_MADCTR_MODE_PORTRAIT 0x00 00154 #define OTM8009A_MADCTR_MODE_LANDSCAPE 0x60 /* MY = 0, MX = 1, MV = 1, ML = 0, RGB = 0 */ 00155 00156 #define OTM8009A_CMD_IDMOFF 0x38 /* Idle mode Off command */ 00157 #define OTM8009A_CMD_IDMON 0x39 /* Idle mode On command */ 00158 00159 #define OTM8009A_CMD_COLMOD 0x3A /* Interface Pixel format command */ 00160 00161 /* Possible values of COLMOD parameter corresponding to used pixel formats */ 00162 #define OTM8009A_COLMOD_RGB565 0x55 00163 #define OTM8009A_COLMOD_RGB888 0x77 00164 00165 #define OTM8009A_CMD_RAMWRC 0x3C /* Memory write continue command */ 00166 #define OTM8009A_CMD_RAMRDC 0x3E /* Memory read continue command */ 00167 00168 #define OTM8009A_CMD_WRTESCN 0x44 /* Write Tearing Effect Scan line command */ 00169 #define OTM8009A_CMD_RDSCNL 0x45 /* Read Tearing Effect Scan line command */ 00170 00171 /* CABC Management : ie : Content Adaptive Back light Control in IC OTM8009a */ 00172 #define OTM8009A_CMD_WRDISBV 0x51 /* Write Display Brightness command */ 00173 #define OTM8009A_CMD_WRCTRLD 0x53 /* Write CTRL Display command */ 00174 #define OTM8009A_CMD_WRCABC 0x55 /* Write Content Adaptive Brightness command */ 00175 #define OTM8009A_CMD_WRCABCMB 0x5E /* Write CABC Minimum Brightness command */ 00176 00177 /** 00178 * @brief OTM8009A_480X800 frequency divider 00179 */ 00180 #define OTM8009A_480X800_FREQUENCY_DIVIDER 2 /* LCD Frequency divider */ 00181 00182 /** 00183 * @} 00184 */ 00185 00186 /* Exported macro ------------------------------------------------------------*/ 00187 00188 /** @defgroup OTM8009A_Exported_Macros OTM8009A Exported Macros 00189 * @{ 00190 */ 00191 00192 /** 00193 * @} 00194 */ 00195 00196 /* Exported functions --------------------------------------------------------*/ 00197 00198 /** @addtogroup OTM8009A_Exported_Functions 00199 * @{ 00200 */ 00201 void DSI_IO_WriteCmd(uint32_t NbrParams, uint8_t *pParams); 00202 uint8_t OTM8009A_Init(uint32_t ColorCoding, uint32_t orientation); 00203 void OTM8009A_IO_Delay(uint32_t Delay); 00204 /** 00205 * @} 00206 */ 00207 #ifdef __cplusplus 00208 } 00209 #endif 00210 00211 #endif /* __OTM8009A_480X800_H */ 00212 /** 00213 * @} 00214 */ 00215 00216 /** 00217 * @} 00218 */ 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 18:45:41 by
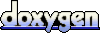