STM32Cube BSP FW for STM32F769I-Discovery
Dependents: mbed-os-example-blinky-5 DISCO-F769NI_TOUCHSCREEN_demo_custom_1 Datarecorder2 DISCO-F769NI_TOUCHSCREEN_demo ... more
stm32f769i_discovery_sdram.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery_sdram.c 00004 * @author MCD Application Team 00005 * @brief This file includes the SDRAM driver for the MT48LC4M32B2B5-6A memory 00006 * device mounted on STM32F769I-DISCOVERY boards. 00007 @verbatim 00008 How To use this driver: 00009 ----------------------- 00010 - This driver is used to drive the MT48LC4M32B2B5-6A SDRAM external memory mounted 00011 on STM32F769I-DISCOVERY board. 00012 - This driver does not need a specific component driver for the SDRAM device 00013 to be included with. 00014 00015 Driver description: 00016 ------------------ 00017 + Initialization steps: 00018 o Initialize the SDRAM external memory using the BSP_SDRAM_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 FMC controller configuration to interface with the external SDRAM memory. 00021 o It contains the SDRAM initialization sequence to program the SDRAM external 00022 device using the function BSP_SDRAM_Initialization_sequence(). Note that this 00023 sequence is standard for all SDRAM devices, but can include some differences 00024 from a device to another. If it is the case, the right sequence should be 00025 implemented separately. 00026 00027 + SDRAM read/write operations 00028 o SDRAM external memory can be accessed with read/write operations once it is 00029 initialized. 00030 Read/write operation can be performed with AHB access using the functions 00031 BSP_SDRAM_ReadData()/BSP_SDRAM_WriteData(), or by DMA transfer using the functions 00032 BSP_SDRAM_ReadData_DMA()/BSP_SDRAM_WriteData_DMA(). 00033 o The AHB access is performed with 32-bit width transaction, the DMA transfer 00034 configuration is fixed at single (no burst) word transfer (see the 00035 SDRAM_MspInit() static function). 00036 o User can implement his own functions for read/write access with his desired 00037 configurations. 00038 o If interrupt mode is used for DMA transfer, the function BSP_SDRAM_DMA_IRQHandler() 00039 is called in IRQ handler file, to serve the generated interrupt once the DMA 00040 transfer is complete. 00041 o You can send a command to the SDRAM device in runtime using the function 00042 BSP_SDRAM_Sendcmd(), and giving the desired command as parameter chosen between 00043 the predefined commands of the "FMC_SDRAM_CommandTypeDef" structure. 00044 @endverbatim 00045 ****************************************************************************** 00046 * @attention 00047 * 00048 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00049 * 00050 * Redistribution and use in source and binary forms, with or without modification, 00051 * are permitted provided that the following conditions are met: 00052 * 1. Redistributions of source code must retain the above copyright notice, 00053 * this list of conditions and the following disclaimer. 00054 * 2. Redistributions in binary form must reproduce the above copyright notice, 00055 * this list of conditions and the following disclaimer in the documentation 00056 * and/or other materials provided with the distribution. 00057 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00058 * may be used to endorse or promote products derived from this software 00059 * without specific prior written permission. 00060 * 00061 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00062 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00063 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00064 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00065 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00066 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00067 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00068 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00069 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00070 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00071 * 00072 ****************************************************************************** 00073 */ 00074 00075 /* Dependencies 00076 - stm32f7xx_hal_sdram.c 00077 - stm32f7xx_ll_fmc.c 00078 - stm32f7xx_hal_dma.c 00079 - stm32f7xx_hal_gpio.c 00080 - stm32f7xx_hal_cortex.c 00081 - stm32f7xx_hal_rcc_ex.h 00082 EndDependencies */ 00083 00084 /* Includes ------------------------------------------------------------------*/ 00085 #include "stm32f769i_discovery_sdram.h" 00086 00087 /** @addtogroup BSP 00088 * @{ 00089 */ 00090 00091 /** @addtogroup STM32F769I_DISCOVERY 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32F769I_DISCOVERY_SDRAM STM32F769I_DISCOVERY SDRAM 00096 * @{ 00097 */ 00098 00099 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Types_Definitions SDRAM Private Types Definitions 00100 * @{ 00101 */ 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Defines SDRAM Private Defines 00107 * @{ 00108 */ 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Macros SDRAM Private Macros 00114 * @{ 00115 */ 00116 /** 00117 * @} 00118 */ 00119 00120 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Variables SDRAM Private Variables 00121 * @{ 00122 */ 00123 SDRAM_HandleTypeDef sdramHandle; 00124 static FMC_SDRAM_TimingTypeDef Timing; 00125 static FMC_SDRAM_CommandTypeDef Command; 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Function_Prototypes SDRAM Private Function Prototypes 00131 * @{ 00132 */ 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32F769I_DISCOVERY_SDRAM_Private_Functions SDRAM Private Functions 00138 * @{ 00139 */ 00140 00141 /** 00142 * @brief Initializes the SDRAM device. 00143 * @retval SDRAM status 00144 */ 00145 uint8_t BSP_SDRAM_Init(void) 00146 { 00147 static uint8_t sdramstatus = SDRAM_ERROR; 00148 /* SDRAM device configuration */ 00149 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00150 00151 /* Timing configuration for 100Mhz as SDRAM clock frequency (System clock is up to 200Mhz) */ 00152 Timing.LoadToActiveDelay = 2; 00153 Timing.ExitSelfRefreshDelay = 7; 00154 Timing.SelfRefreshTime = 4; 00155 Timing.RowCycleDelay = 7; 00156 Timing.WriteRecoveryTime = 2; 00157 Timing.RPDelay = 2; 00158 Timing.RCDDelay = 2; 00159 00160 sdramHandle.Init.SDBank = FMC_SDRAM_BANK1; 00161 sdramHandle.Init.ColumnBitsNumber = FMC_SDRAM_COLUMN_BITS_NUM_8; 00162 sdramHandle.Init.RowBitsNumber = FMC_SDRAM_ROW_BITS_NUM_12; 00163 sdramHandle.Init.MemoryDataWidth = SDRAM_MEMORY_WIDTH; 00164 sdramHandle.Init.InternalBankNumber = FMC_SDRAM_INTERN_BANKS_NUM_4; 00165 sdramHandle.Init.CASLatency = FMC_SDRAM_CAS_LATENCY_3; 00166 sdramHandle.Init.WriteProtection = FMC_SDRAM_WRITE_PROTECTION_DISABLE; 00167 sdramHandle.Init.SDClockPeriod = SDCLOCK_PERIOD; 00168 sdramHandle.Init.ReadBurst = FMC_SDRAM_RBURST_ENABLE; 00169 sdramHandle.Init.ReadPipeDelay = FMC_SDRAM_RPIPE_DELAY_0; 00170 00171 /* SDRAM controller initialization */ 00172 00173 BSP_SDRAM_MspInit(&sdramHandle, NULL); /* __weak function can be rewritten by the application */ 00174 00175 if(HAL_SDRAM_Init(&sdramHandle, &Timing) != HAL_OK) 00176 { 00177 sdramstatus = SDRAM_ERROR; 00178 } 00179 else 00180 { 00181 sdramstatus = SDRAM_OK; 00182 } 00183 00184 /* SDRAM initialization sequence */ 00185 BSP_SDRAM_Initialization_sequence(REFRESH_COUNT); 00186 00187 return sdramstatus; 00188 } 00189 00190 /** 00191 * @brief DeInitializes the SDRAM device. 00192 * @retval SDRAM status 00193 */ 00194 uint8_t BSP_SDRAM_DeInit(void) 00195 { 00196 static uint8_t sdramstatus = SDRAM_ERROR; 00197 /* SDRAM device de-initialization */ 00198 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00199 00200 if(HAL_SDRAM_DeInit(&sdramHandle) != HAL_OK) 00201 { 00202 sdramstatus = SDRAM_ERROR; 00203 } 00204 else 00205 { 00206 sdramstatus = SDRAM_OK; 00207 } 00208 00209 /* SDRAM controller de-initialization */ 00210 BSP_SDRAM_MspDeInit(&sdramHandle, NULL); 00211 00212 return sdramstatus; 00213 } 00214 00215 /** 00216 * @brief Programs the SDRAM device. 00217 * @param RefreshCount: SDRAM refresh counter value 00218 * @retval None 00219 */ 00220 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount) 00221 { 00222 __IO uint32_t tmpmrd = 0; 00223 00224 /* Step 1: Configure a clock configuration enable command */ 00225 Command.CommandMode = FMC_SDRAM_CMD_CLK_ENABLE; 00226 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00227 Command.AutoRefreshNumber = 1; 00228 Command.ModeRegisterDefinition = 0; 00229 00230 /* Send the command */ 00231 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00232 00233 /* Step 2: Insert 100 us minimum delay */ 00234 /* Inserted delay is equal to 1 ms due to systick time base unit (ms) */ 00235 HAL_Delay(1); 00236 00237 /* Step 3: Configure a PALL (precharge all) command */ 00238 Command.CommandMode = FMC_SDRAM_CMD_PALL; 00239 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00240 Command.AutoRefreshNumber = 1; 00241 Command.ModeRegisterDefinition = 0; 00242 00243 /* Send the command */ 00244 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00245 00246 /* Step 4: Configure an Auto Refresh command */ 00247 Command.CommandMode = FMC_SDRAM_CMD_AUTOREFRESH_MODE; 00248 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00249 Command.AutoRefreshNumber = 8; 00250 Command.ModeRegisterDefinition = 0; 00251 00252 /* Send the command */ 00253 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00254 00255 /* Step 5: Program the external memory mode register */ 00256 tmpmrd = (uint32_t)SDRAM_MODEREG_BURST_LENGTH_1 |\ 00257 SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL |\ 00258 SDRAM_MODEREG_CAS_LATENCY_3 |\ 00259 SDRAM_MODEREG_OPERATING_MODE_STANDARD |\ 00260 SDRAM_MODEREG_WRITEBURST_MODE_SINGLE; 00261 00262 Command.CommandMode = FMC_SDRAM_CMD_LOAD_MODE; 00263 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00264 Command.AutoRefreshNumber = 1; 00265 Command.ModeRegisterDefinition = tmpmrd; 00266 00267 /* Send the command */ 00268 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00269 00270 /* Step 6: Set the refresh rate counter */ 00271 /* Set the device refresh rate */ 00272 HAL_SDRAM_ProgramRefreshRate(&sdramHandle, RefreshCount); 00273 } 00274 00275 /** 00276 * @brief Reads an amount of data from the SDRAM memory in polling mode. 00277 * @param uwStartAddress: Read start address 00278 * @param pData: Pointer to data to be read 00279 * @param uwDataSize: Size of read data from the memory 00280 * @retval SDRAM status 00281 */ 00282 uint8_t BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00283 { 00284 if(HAL_SDRAM_Read_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00285 { 00286 return SDRAM_ERROR; 00287 } 00288 else 00289 { 00290 return SDRAM_OK; 00291 } 00292 } 00293 00294 /** 00295 * @brief Reads an amount of data from the SDRAM memory in DMA mode. 00296 * @param uwStartAddress: Read start address 00297 * @param pData: Pointer to data to be read 00298 * @param uwDataSize: Size of read data from the memory 00299 * @retval SDRAM status 00300 */ 00301 uint8_t BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00302 { 00303 if(HAL_SDRAM_Read_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00304 { 00305 return SDRAM_ERROR; 00306 } 00307 else 00308 { 00309 return SDRAM_OK; 00310 } 00311 } 00312 00313 /** 00314 * @brief Writes an amount of data to the SDRAM memory in polling mode. 00315 * @param uwStartAddress: Write start address 00316 * @param pData: Pointer to data to be written 00317 * @param uwDataSize: Size of written data from the memory 00318 * @retval SDRAM status 00319 */ 00320 uint8_t BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00321 { 00322 if(HAL_SDRAM_Write_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00323 { 00324 return SDRAM_ERROR; 00325 } 00326 else 00327 { 00328 return SDRAM_OK; 00329 } 00330 } 00331 00332 /** 00333 * @brief Writes an amount of data to the SDRAM memory in DMA mode. 00334 * @param uwStartAddress: Write start address 00335 * @param pData: Pointer to data to be written 00336 * @param uwDataSize: Size of written data from the memory 00337 * @retval SDRAM status 00338 */ 00339 uint8_t BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00340 { 00341 if(HAL_SDRAM_Write_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00342 { 00343 return SDRAM_ERROR; 00344 } 00345 else 00346 { 00347 return SDRAM_OK; 00348 } 00349 } 00350 00351 /** 00352 * @brief Sends command to the SDRAM bank. 00353 * @param SdramCmd: Pointer to SDRAM command structure 00354 * @retval SDRAM status 00355 */ 00356 uint8_t BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd) 00357 { 00358 if(HAL_SDRAM_SendCommand(&sdramHandle, SdramCmd, SDRAM_TIMEOUT) != HAL_OK) 00359 { 00360 return SDRAM_ERROR; 00361 } 00362 else 00363 { 00364 return SDRAM_OK; 00365 } 00366 } 00367 00368 /** 00369 * @brief Initializes SDRAM MSP. 00370 * @param hsdram: SDRAM handle 00371 * @param Params 00372 * @retval None 00373 */ 00374 __weak void BSP_SDRAM_MspInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00375 { 00376 static DMA_HandleTypeDef dma_handle; 00377 GPIO_InitTypeDef gpio_init_structure; 00378 00379 /* Enable FMC clock */ 00380 __HAL_RCC_FMC_CLK_ENABLE(); 00381 00382 /* Enable chosen DMAx clock */ 00383 __DMAx_CLK_ENABLE(); 00384 00385 /* Enable GPIOs clock */ 00386 __HAL_RCC_GPIOD_CLK_ENABLE(); 00387 __HAL_RCC_GPIOE_CLK_ENABLE(); 00388 __HAL_RCC_GPIOF_CLK_ENABLE(); 00389 __HAL_RCC_GPIOG_CLK_ENABLE(); 00390 __HAL_RCC_GPIOH_CLK_ENABLE(); 00391 __HAL_RCC_GPIOI_CLK_ENABLE(); 00392 00393 /* Common GPIO configuration */ 00394 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00395 gpio_init_structure.Pull = GPIO_PULLUP; 00396 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00397 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00398 00399 /* GPIOD configuration */ 00400 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8| GPIO_PIN_9 | GPIO_PIN_10 |\ 00401 GPIO_PIN_14 | GPIO_PIN_15; 00402 00403 00404 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00405 00406 /* GPIOE configuration */ 00407 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7| GPIO_PIN_8 | GPIO_PIN_9 |\ 00408 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00409 GPIO_PIN_15; 00410 00411 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00412 00413 /* GPIOF configuration */ 00414 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00415 GPIO_PIN_5 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00416 GPIO_PIN_15; 00417 00418 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00419 00420 /* GPIOG configuration */ 00421 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_4|\ 00422 GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_15; 00423 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00424 00425 /* GPIOH configuration */ 00426 gpio_init_structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_9 |\ 00427 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00428 GPIO_PIN_15; 00429 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00430 00431 /* GPIOI configuration */ 00432 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00433 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10; 00434 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00435 00436 /* Configure common DMA parameters */ 00437 dma_handle.Init.Channel = SDRAM_DMAx_CHANNEL; 00438 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00439 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00440 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00441 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00442 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00443 dma_handle.Init.Mode = DMA_NORMAL; 00444 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00445 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00446 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00447 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00448 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00449 00450 dma_handle.Instance = SDRAM_DMAx_STREAM; 00451 00452 /* Associate the DMA handle */ 00453 __HAL_LINKDMA(hsdram, hdma, dma_handle); 00454 00455 /* Deinitialize the stream for new transfer */ 00456 HAL_DMA_DeInit(&dma_handle); 00457 00458 /* Configure the DMA stream */ 00459 HAL_DMA_Init(&dma_handle); 00460 00461 /* NVIC configuration for DMA transfer complete interrupt */ 00462 HAL_NVIC_SetPriority(SDRAM_DMAx_IRQn, 0x0F, 0); 00463 HAL_NVIC_EnableIRQ(SDRAM_DMAx_IRQn); 00464 } 00465 00466 /** 00467 * @brief DeInitializes SDRAM MSP. 00468 * @param hsdram: SDRAM handle 00469 * @param Params 00470 * @retval None 00471 */ 00472 __weak void BSP_SDRAM_MspDeInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00473 { 00474 static DMA_HandleTypeDef dma_handle; 00475 00476 /* Disable NVIC configuration for DMA interrupt */ 00477 HAL_NVIC_DisableIRQ(SDRAM_DMAx_IRQn); 00478 00479 /* Deinitialize the stream for new transfer */ 00480 dma_handle.Instance = SDRAM_DMAx_STREAM; 00481 HAL_DMA_DeInit(&dma_handle); 00482 00483 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00484 by surcharging this __weak function */ 00485 } 00486 00487 /** 00488 * @} 00489 */ 00490 00491 /** 00492 * @} 00493 */ 00494 00495 /** 00496 * @} 00497 */ 00498 00499 /** 00500 * @} 00501 */ 00502 00503 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:55:04 by
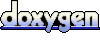