STM32Cube BSP FW for STM32F769I-Discovery
Dependents: mbed-os-example-blinky-5 DISCO-F769NI_TOUCHSCREEN_demo_custom_1 Datarecorder2 DISCO-F769NI_TOUCHSCREEN_demo ... more
ft6x06.h
00001 /** 00002 ****************************************************************************** 00003 * @file ft6x06.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * ft6x06.c IO expander driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __FT6X06_H 00039 #define __FT6X06_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Set Multi-touch as non supported */ 00046 #ifndef TS_MULTI_TOUCH_SUPPORTED 00047 #define TS_MULTI_TOUCH_SUPPORTED 0 00048 #endif 00049 00050 /* Set Auto-calibration as non supported */ 00051 #ifndef TS_AUTO_CALIBRATION_SUPPORTED 00052 #define TS_AUTO_CALIBRATION_SUPPORTED 0 00053 #endif 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "../Common/ts.h" 00057 00058 /* Macros --------------------------------------------------------------------*/ 00059 00060 /** @typedef ft6x06_handle_TypeDef 00061 * ft6x06 Handle definition. 00062 */ 00063 typedef struct 00064 { 00065 uint8_t i2cInitialized; 00066 00067 /* field holding the current number of simultaneous active touches */ 00068 uint8_t currActiveTouchNb; 00069 00070 /* field holding the touch index currently managed */ 00071 uint8_t currActiveTouchIdx; 00072 00073 } ft6x06_handle_TypeDef; 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup Component 00080 * @{ 00081 */ 00082 00083 /** @defgroup FT6X06 00084 * @{ 00085 */ 00086 00087 /* Exported types ------------------------------------------------------------*/ 00088 00089 /** @defgroup FT6X06_Exported_Types 00090 * @{ 00091 */ 00092 00093 /* Exported constants --------------------------------------------------------*/ 00094 00095 /** @defgroup FT6X06_Exported_Constants 00096 * @{ 00097 */ 00098 00099 /* Maximum border values of the touchscreen pad */ 00100 #define FT_6206_MAX_WIDTH ((uint16_t)800) /* Touchscreen pad max width */ 00101 #define FT_6206_MAX_HEIGHT ((uint16_t)480) /* Touchscreen pad max height */ 00102 00103 /* Touchscreen pad max width and height values for FT6x36 Touch*/ 00104 #define FT_6206_MAX_WIDTH_HEIGHT ((uint16_t)240) 00105 00106 /* Possible values of driver functions return status */ 00107 #define FT6206_STATUS_OK 0 00108 #define FT6206_STATUS_NOT_OK 1 00109 00110 /* Possible values of global variable 'TS_I2C_Initialized' */ 00111 #define FT6206_I2C_NOT_INITIALIZED 0 00112 #define FT6206_I2C_INITIALIZED 1 00113 00114 /* Max detectable simultaneous touches */ 00115 #define FT6206_MAX_DETECTABLE_TOUCH 2 00116 00117 /** 00118 * @brief : Definitions for FT6206 I2C register addresses on 8 bit 00119 **/ 00120 00121 /* Current mode register of the FT6206 (R/W) */ 00122 #define FT6206_DEV_MODE_REG 0x00 00123 00124 /* Possible values of FT6206_DEV_MODE_REG */ 00125 #define FT6206_DEV_MODE_WORKING 0x00 00126 #define FT6206_DEV_MODE_FACTORY 0x04 00127 00128 #define FT6206_DEV_MODE_MASK 0x7 00129 #define FT6206_DEV_MODE_SHIFT 4 00130 00131 /* Gesture ID register */ 00132 #define FT6206_GEST_ID_REG 0x01 00133 00134 /* Possible values of FT6206_GEST_ID_REG */ 00135 #define FT6206_GEST_ID_NO_GESTURE 0x00 00136 #define FT6206_GEST_ID_MOVE_UP 0x10 00137 #define FT6206_GEST_ID_MOVE_RIGHT 0x14 00138 #define FT6206_GEST_ID_MOVE_DOWN 0x18 00139 #define FT6206_GEST_ID_MOVE_LEFT 0x1C 00140 #define FT6206_GEST_ID_ZOOM_IN 0x48 00141 #define FT6206_GEST_ID_ZOOM_OUT 0x49 00142 00143 /* Touch Data Status register : gives number of active touch points (0..2) */ 00144 #define FT6206_TD_STAT_REG 0x02 00145 00146 /* Values related to FT6206_TD_STAT_REG */ 00147 #define FT6206_TD_STAT_MASK 0x0F 00148 #define FT6206_TD_STAT_SHIFT 0x00 00149 00150 /* Values Pn_XH and Pn_YH related */ 00151 #define FT6206_TOUCH_EVT_FLAG_PRESS_DOWN 0x00 00152 #define FT6206_TOUCH_EVT_FLAG_LIFT_UP 0x01 00153 #define FT6206_TOUCH_EVT_FLAG_CONTACT 0x02 00154 #define FT6206_TOUCH_EVT_FLAG_NO_EVENT 0x03 00155 00156 #define FT6206_TOUCH_EVT_FLAG_SHIFT 6 00157 #define FT6206_TOUCH_EVT_FLAG_MASK (3 << FT6206_TOUCH_EVT_FLAG_SHIFT) 00158 00159 #define FT6206_MSB_MASK 0x0F 00160 #define FT6206_MSB_SHIFT 0 00161 00162 /* Values Pn_XL and Pn_YL related */ 00163 #define FT6206_LSB_MASK 0xFF 00164 #define FT6206_LSB_SHIFT 0 00165 00166 #define FT6206_P1_XH_REG 0x03 00167 #define FT6206_P1_XL_REG 0x04 00168 #define FT6206_P1_YH_REG 0x05 00169 #define FT6206_P1_YL_REG 0x06 00170 00171 /* Touch Pressure register value (R) */ 00172 #define FT6206_P1_WEIGHT_REG 0x07 00173 00174 /* Values Pn_WEIGHT related */ 00175 #define FT6206_TOUCH_WEIGHT_MASK 0xFF 00176 #define FT6206_TOUCH_WEIGHT_SHIFT 0 00177 00178 /* Touch area register */ 00179 #define FT6206_P1_MISC_REG 0x08 00180 00181 /* Values related to FT6206_Pn_MISC_REG */ 00182 #define FT6206_TOUCH_AREA_MASK (0x04 << 4) 00183 #define FT6206_TOUCH_AREA_SHIFT 0x04 00184 00185 #define FT6206_P2_XH_REG 0x09 00186 #define FT6206_P2_XL_REG 0x0A 00187 #define FT6206_P2_YH_REG 0x0B 00188 #define FT6206_P2_YL_REG 0x0C 00189 #define FT6206_P2_WEIGHT_REG 0x0D 00190 #define FT6206_P2_MISC_REG 0x0E 00191 00192 /* Threshold for touch detection */ 00193 #define FT6206_TH_GROUP_REG 0x80 00194 00195 /* Values FT6206_TH_GROUP_REG : threshold related */ 00196 #define FT6206_THRESHOLD_MASK 0xFF 00197 #define FT6206_THRESHOLD_SHIFT 0 00198 00199 /* Filter function coefficients */ 00200 #define FT6206_TH_DIFF_REG 0x85 00201 00202 /* Control register */ 00203 #define FT6206_CTRL_REG 0x86 00204 00205 /* Values related to FT6206_CTRL_REG */ 00206 00207 /* Will keep the Active mode when there is no touching */ 00208 #define FT6206_CTRL_KEEP_ACTIVE_MODE 0x00 00209 00210 /* Switching from Active mode to Monitor mode automatically when there is no touching */ 00211 #define FT6206_CTRL_KEEP_AUTO_SWITCH_MONITOR_MODE 0x01 00212 00213 /* The time period of switching from Active mode to Monitor mode when there is no touching */ 00214 #define FT6206_TIMEENTERMONITOR_REG 0x87 00215 00216 /* Report rate in Active mode */ 00217 #define FT6206_PERIODACTIVE_REG 0x88 00218 00219 /* Report rate in Monitor mode */ 00220 #define FT6206_PERIODMONITOR_REG 0x89 00221 00222 /* The value of the minimum allowed angle while Rotating gesture mode */ 00223 #define FT6206_RADIAN_VALUE_REG 0x91 00224 00225 /* Maximum offset while Moving Left and Moving Right gesture */ 00226 #define FT6206_OFFSET_LEFT_RIGHT_REG 0x92 00227 00228 /* Maximum offset while Moving Up and Moving Down gesture */ 00229 #define FT6206_OFFSET_UP_DOWN_REG 0x93 00230 00231 /* Minimum distance while Moving Left and Moving Right gesture */ 00232 #define FT6206_DISTANCE_LEFT_RIGHT_REG 0x94 00233 00234 /* Minimum distance while Moving Up and Moving Down gesture */ 00235 #define FT6206_DISTANCE_UP_DOWN_REG 0x95 00236 00237 /* Maximum distance while Zoom In and Zoom Out gesture */ 00238 #define FT6206_DISTANCE_ZOOM_REG 0x96 00239 00240 /* High 8-bit of LIB Version info */ 00241 #define FT6206_LIB_VER_H_REG 0xA1 00242 00243 /* Low 8-bit of LIB Version info */ 00244 #define FT6206_LIB_VER_L_REG 0xA2 00245 00246 /* Chip Selecting */ 00247 #define FT6206_CIPHER_REG 0xA3 00248 00249 /* Interrupt mode register (used when in interrupt mode) */ 00250 #define FT6206_GMODE_REG 0xA4 00251 00252 #define FT6206_G_MODE_INTERRUPT_MASK 0x03 00253 #define FT6206_G_MODE_INTERRUPT_SHIFT 0x00 00254 00255 /* Possible values of FT6206_GMODE_REG */ 00256 #define FT6206_G_MODE_INTERRUPT_POLLING 0x00 00257 #define FT6206_G_MODE_INTERRUPT_TRIGGER 0x01 00258 00259 /* Current power mode the FT6206 system is in (R) */ 00260 #define FT6206_PWR_MODE_REG 0xA5 00261 00262 /* FT6206 firmware version */ 00263 #define FT6206_FIRMID_REG 0xA6 00264 00265 /* FT6206 Chip identification register */ 00266 #define FT6206_CHIP_ID_REG 0xA8 00267 00268 /* Possible values of FT6206_CHIP_ID_REG */ 00269 #define FT6206_ID_VALUE 0x11 00270 #define FT6x36_ID_VALUE 0xCD 00271 00272 /* Release code version */ 00273 #define FT6206_RELEASE_CODE_ID_REG 0xAF 00274 00275 /* Current operating mode the FT6206 system is in (R) */ 00276 #define FT6206_STATE_REG 0xBC 00277 00278 /** 00279 * @} 00280 */ 00281 00282 /* Exported macro ------------------------------------------------------------*/ 00283 00284 /** @defgroup ft6x06_Exported_Macros 00285 * @{ 00286 */ 00287 00288 /* Exported functions --------------------------------------------------------*/ 00289 00290 /** @defgroup ft6x06_Exported_Functions 00291 * @{ 00292 */ 00293 00294 /** 00295 * @brief ft6x06 Control functions 00296 */ 00297 00298 00299 /** 00300 * @brief Initialize the ft6x06 communication bus 00301 * from MCU to FT6206 : ie I2C channel initialization (if required). 00302 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00303 * @retval None 00304 */ 00305 void ft6x06_Init(uint16_t DeviceAddr); 00306 00307 /** 00308 * @brief Software Reset the ft6x06. 00309 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00310 * @retval None 00311 */ 00312 void ft6x06_Reset(uint16_t DeviceAddr); 00313 00314 /** 00315 * @brief Read the ft6x06 device ID, pre intitalize I2C in case of need to be 00316 * able to read the FT6206 device ID, and verify this is a FT6206. 00317 * @param DeviceAddr: I2C FT6x06 Slave address. 00318 * @retval The Device ID (two bytes). 00319 */ 00320 uint16_t ft6x06_ReadID(uint16_t DeviceAddr); 00321 00322 /** 00323 * @brief Configures the touch Screen IC device to start detecting touches 00324 * @param DeviceAddr: Device address on communication Bus (I2C slave address). 00325 * @retval None. 00326 */ 00327 void ft6x06_TS_Start(uint16_t DeviceAddr); 00328 00329 /** 00330 * @brief Return if there is touches detected or not. 00331 * Try to detect new touches and forget the old ones (reset internal global 00332 * variables). 00333 * @param DeviceAddr: Device address on communication Bus. 00334 * @retval : Number of active touches detected (can be 0, 1 or 2). 00335 */ 00336 uint8_t ft6x06_TS_DetectTouch(uint16_t DeviceAddr); 00337 00338 /** 00339 * @brief Get the touch screen X and Y positions values 00340 * Manage multi touch thanks to touch Index global 00341 * variable 'ft6x06_handle.currActiveTouchIdx'. 00342 * @param DeviceAddr: Device address on communication Bus. 00343 * @param X: Pointer to X position value 00344 * @param Y: Pointer to Y position value 00345 * @retval None. 00346 */ 00347 void ft6x06_TS_GetXY(uint16_t DeviceAddr, uint16_t *X, uint16_t *Y); 00348 00349 /** 00350 * @brief Configure the FT6206 device to generate IT on given INT pin 00351 * connected to MCU as EXTI. 00352 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT6206). 00353 * @retval None 00354 */ 00355 void ft6x06_TS_EnableIT(uint16_t DeviceAddr); 00356 00357 /** 00358 * @brief Configure the FT6206 device to stop generating IT on the given INT pin 00359 * connected to MCU as EXTI. 00360 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT6206). 00361 * @retval None 00362 */ 00363 void ft6x06_TS_DisableIT(uint16_t DeviceAddr); 00364 00365 /** 00366 * @brief Get IT status from FT6206 interrupt status registers 00367 * Should be called Following an EXTI coming to the MCU to know the detailed 00368 * reason of the interrupt. 00369 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00370 * @retval TS interrupts status 00371 */ 00372 uint8_t ft6x06_TS_ITStatus (uint16_t DeviceAddr); 00373 00374 /** 00375 * @brief Clear IT status in FT6206 interrupt status clear registers 00376 * Should be called Following an EXTI coming to the MCU. 00377 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00378 * @retval TS interrupts status 00379 */ 00380 void ft6x06_TS_ClearIT (uint16_t DeviceAddr); 00381 00382 /**** NEW FEATURES enabled when Multi-touch support is enabled ****/ 00383 00384 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00385 00386 /** 00387 * @brief Get the last touch gesture identification (zoom, move up/down...). 00388 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6x06). 00389 * @param pGestureId : Pointer to get last touch gesture Identification. 00390 * @retval None. 00391 */ 00392 void ft6x06_TS_GetGestureID(uint16_t DeviceAddr, uint32_t * pGestureId); 00393 00394 /** 00395 * @brief Get the touch detailed informations on touch number 'touchIdx' (0..1) 00396 * This touch detailed information contains : 00397 * - weight that was applied to this touch 00398 * - sub-area of the touch in the touch panel 00399 * - event of linked to the touch (press down, lift up, ...) 00400 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6x06). 00401 * @param touchIdx : Passed index of the touch (0..1) on which we want to get the 00402 * detailed information. 00403 * @param pWeight : Pointer to to get the weight information of 'touchIdx'. 00404 * @param pArea : Pointer to to get the sub-area information of 'touchIdx'. 00405 * @param pEvent : Pointer to to get the event information of 'touchIdx'. 00406 00407 * @retval None. 00408 */ 00409 void ft6x06_TS_GetTouchInfo(uint16_t DeviceAddr, 00410 uint32_t touchIdx, 00411 uint32_t * pWeight, 00412 uint32_t * pArea, 00413 uint32_t * pEvent); 00414 00415 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00416 00417 /* Imported TS IO functions --------------------------------------------------------*/ 00418 00419 /** @defgroup ft6x06_Imported_Functions 00420 * @{ 00421 */ 00422 00423 /* TouchScreen (TS) external IO functions */ 00424 extern void TS_IO_Init(void); 00425 extern void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00426 extern uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00427 extern uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00428 extern void TS_IO_Delay(uint32_t Delay); 00429 00430 /** 00431 * @} 00432 */ 00433 00434 /* Imported global variables --------------------------------------------------------*/ 00435 00436 /** @defgroup ft6x06_Imported_Globals 00437 * @{ 00438 */ 00439 00440 00441 /* Touch screen driver structure */ 00442 extern TS_DrvTypeDef ft6x06_ts_drv; 00443 00444 /** 00445 * @} 00446 */ 00447 00448 #ifdef __cplusplus 00449 } 00450 #endif 00451 #endif /* __FT6X06_H */ 00452 00453 00454 /** 00455 * @} 00456 */ 00457 00458 /** 00459 * @} 00460 */ 00461 00462 /** 00463 * @} 00464 */ 00465 00466 /** 00467 * @} 00468 */ 00469 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:55:04 by
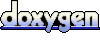