STM32746G-Discovery board drivers V1.0.0
Dependents: DISCO-F746NG_LCDTS_CC3000_NTP DISCO-F746NG_ROPE_WIFI F746_SpectralAnalysis_NoPhoto ecte433 ... more
stm32746g_discovery_camera.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery_camera.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Camera modules mounted on 00006 * STM32746G-Discovery board. 00007 @verbatim 00008 How to use this driver: 00009 ------------------------ 00010 - This driver is used to drive the camera. 00011 - The OV9655 component driver MUST be included with this driver. 00012 00013 Driver description: 00014 ------------------- 00015 + Initialization steps: 00016 o Initialize the camera using the BSP_CAMERA_Init() function. 00017 o Start the camera capture/snapshot using the CAMERA_Start() function. 00018 o Suspend, resume or stop the camera capture using the following functions: 00019 - BSP_CAMERA_Suspend() 00020 - BSP_CAMERA_Resume() 00021 - BSP_CAMERA_Stop() 00022 00023 + Options 00024 o Increase or decrease on the fly the brightness and/or contrast 00025 using the following function: 00026 - BSP_CAMERA_ContrastBrightnessConfig 00027 o Add a special effect on the fly using the following functions: 00028 - BSP_CAMERA_BlackWhiteConfig() 00029 - BSP_CAMERA_ColorEffectConfig() 00030 @endverbatim 00031 ****************************************************************************** 00032 * @attention 00033 * 00034 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00035 * 00036 * Redistribution and use in source and binary forms, with or without modification, 00037 * are permitted provided that the following conditions are met: 00038 * 1. Redistributions of source code must retain the above copyright notice, 00039 * this list of conditions and the following disclaimer. 00040 * 2. Redistributions in binary form must reproduce the above copyright notice, 00041 * this list of conditions and the following disclaimer in the documentation 00042 * and/or other materials provided with the distribution. 00043 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00044 * may be used to endorse or promote products derived from this software 00045 * without specific prior written permission. 00046 * 00047 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00048 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00049 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00050 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00051 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00052 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00053 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00054 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00055 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00056 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00057 * 00058 ****************************************************************************** 00059 */ 00060 00061 /* Dependencies 00062 - stm32746g_discovery.c 00063 - stm32f7xx_hal_dcmi.c 00064 - stm32f7xx_hal_dma.c 00065 - stm32f7xx_hal_gpio.c 00066 - stm32f7xx_hal_cortex.c 00067 - stm32f7xx_hal_rcc_ex.h 00068 - ov9655.c 00069 EndDependencies */ 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "stm32746g_discovery_camera.h" 00073 #include "stm32746g_discovery.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM32746G_DISCOVERY 00080 * @{ 00081 */ 00082 00083 /** @addtogroup STM32746G_DISCOVERY_CAMERA 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_TypesDefinitions STM32746G_DISCOVERY_CAMERA Private Types Definitions 00088 * @{ 00089 */ 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Defines STM32746G_DISCOVERY_CAMERA Private Defines 00095 * @{ 00096 */ 00097 #define CAMERA_VGA_RES_X 640 00098 #define CAMERA_VGA_RES_Y 480 00099 #define CAMERA_480x272_RES_X 480 00100 #define CAMERA_480x272_RES_Y 272 00101 #define CAMERA_QVGA_RES_X 320 00102 #define CAMERA_QVGA_RES_Y 240 00103 #define CAMERA_QQVGA_RES_X 160 00104 #define CAMERA_QQVGA_RES_Y 120 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Macros STM32746G_DISCOVERY_CAMERA Private Macros 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Variables STM32746G_DISCOVERY_CAMERA Private Variables 00117 * @{ 00118 */ 00119 DCMI_HandleTypeDef hDcmiHandler; 00120 CAMERA_DrvTypeDef *camera_drv; 00121 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00122 static uint32_t CameraCurrentResolution; 00123 00124 /* Camera module I2C HW address */ 00125 static uint32_t CameraHwAddress; 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_FunctionPrototypes STM32746G_DISCOVERY_CAMERA Private Function Prototypes 00131 * @{ 00132 */ 00133 static uint32_t GetSize(uint32_t resolution); 00134 /** 00135 * @} 00136 */ 00137 00138 /** @defgroup STM32746G_DISCOVERY_CAMERA_Exported_Functions STM32746G_DISCOVERY_CAMERA Exported Functions 00139 * @{ 00140 */ 00141 00142 /** 00143 * @brief Initializes the camera. 00144 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00145 * naming QQVGA, QVGA, VGA ... 00146 * @retval Camera status 00147 */ 00148 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00149 { 00150 DCMI_HandleTypeDef *phdcmi; 00151 uint8_t status = CAMERA_ERROR; 00152 00153 /* Get the DCMI handle structure */ 00154 phdcmi = &hDcmiHandler; 00155 00156 /*** Configures the DCMI to interface with the camera module ***/ 00157 /* DCMI configuration */ 00158 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00159 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_LOW; 00160 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00161 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00162 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00163 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00164 phdcmi->Instance = DCMI; 00165 00166 /* Power up camera */ 00167 BSP_CAMERA_PwrUp(); 00168 00169 /* Read ID of Camera module via I2C */ 00170 if(ov9655_ReadID(CAMERA_I2C_ADDRESS) == OV9655_ID) 00171 { 00172 /* Initialize the camera driver structure */ 00173 camera_drv = &ov9655_drv; 00174 CameraHwAddress = CAMERA_I2C_ADDRESS; 00175 00176 /* DCMI Initialization */ 00177 BSP_CAMERA_MspInit(&hDcmiHandler, NULL); 00178 HAL_DCMI_Init(phdcmi); 00179 00180 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00181 if (Resolution == CAMERA_R480x272) 00182 { /* For 480x272 resolution, the OV9655 sensor is set to VGA resolution 00183 * as OV9655 doesn't supports 480x272 resolution, 00184 * then DCMI is configured to output a 480x272 cropped window */ 00185 camera_drv->Init(CameraHwAddress, CAMERA_R640x480); 00186 HAL_DCMI_ConfigCROP(phdcmi, /* Crop in the middle of the VGA picture */ 00187 (CAMERA_VGA_RES_X - CAMERA_480x272_RES_X)/2, 00188 (CAMERA_VGA_RES_Y - CAMERA_480x272_RES_Y)/2, 00189 (CAMERA_480x272_RES_X * 2) - 1, 00190 CAMERA_480x272_RES_Y - 1); 00191 HAL_DCMI_EnableCROP(phdcmi); 00192 } 00193 else 00194 { 00195 camera_drv->Init(CameraHwAddress, Resolution); 00196 HAL_DCMI_DisableCROP(phdcmi); 00197 } 00198 00199 CameraCurrentResolution = Resolution; 00200 00201 /* Return CAMERA_OK status */ 00202 status = CAMERA_OK; 00203 } 00204 else 00205 { 00206 /* Return CAMERA_NOT_SUPPORTED status */ 00207 status = CAMERA_NOT_SUPPORTED; 00208 } 00209 00210 return status; 00211 } 00212 00213 /** 00214 * @brief DeInitializes the camera. 00215 * @retval Camera status 00216 */ 00217 uint8_t BSP_CAMERA_DeInit(void) 00218 { 00219 hDcmiHandler.Instance = DCMI; 00220 00221 HAL_DCMI_DeInit(&hDcmiHandler); 00222 BSP_CAMERA_MspDeInit(&hDcmiHandler, NULL); 00223 return CAMERA_OK; 00224 } 00225 00226 /** 00227 * @brief Starts the camera capture in continuous mode. 00228 * @param buff: pointer to the camera output buffer 00229 * @retval None 00230 */ 00231 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00232 { 00233 /* Start the camera capture */ 00234 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00235 } 00236 00237 /** 00238 * @brief Starts the camera capture in snapshot mode. 00239 * @param buff: pointer to the camera output buffer 00240 * @retval None 00241 */ 00242 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00243 { 00244 /* Start the camera capture */ 00245 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00246 } 00247 00248 /** 00249 * @brief Suspend the CAMERA capture 00250 * @retval None 00251 */ 00252 void BSP_CAMERA_Suspend(void) 00253 { 00254 /* Suspend the Camera Capture */ 00255 HAL_DCMI_Suspend(&hDcmiHandler); 00256 } 00257 00258 /** 00259 * @brief Resume the CAMERA capture 00260 * @retval None 00261 */ 00262 void BSP_CAMERA_Resume(void) 00263 { 00264 /* Start the Camera Capture */ 00265 HAL_DCMI_Resume(&hDcmiHandler); 00266 } 00267 00268 /** 00269 * @brief Stop the CAMERA capture 00270 * @retval Camera status 00271 */ 00272 uint8_t BSP_CAMERA_Stop(void) 00273 { 00274 uint8_t status = CAMERA_ERROR; 00275 00276 if(HAL_DCMI_Stop(&hDcmiHandler) == HAL_OK) 00277 { 00278 status = CAMERA_OK; 00279 } 00280 00281 /* Set Camera in Power Down */ 00282 BSP_CAMERA_PwrDown(); 00283 00284 return status; 00285 } 00286 00287 /** 00288 * @brief CANERA power up 00289 * @retval None 00290 */ 00291 void BSP_CAMERA_PwrUp(void) 00292 { 00293 GPIO_InitTypeDef gpio_init_structure; 00294 00295 /* Enable GPIO clock */ 00296 __HAL_RCC_GPIOH_CLK_ENABLE(); 00297 00298 /*** Configure the GPIO ***/ 00299 /* Configure DCMI GPIO as alternate function */ 00300 gpio_init_structure.Pin = GPIO_PIN_13; 00301 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00302 gpio_init_structure.Pull = GPIO_NOPULL; 00303 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00304 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00305 00306 /* De-assert the camera POWER_DOWN pin (active high) */ 00307 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_13, GPIO_PIN_RESET); 00308 00309 HAL_Delay(3); /* POWER_DOWN de-asserted during 3ms */ 00310 } 00311 00312 /** 00313 * @brief CAMERA power down 00314 * @retval None 00315 */ 00316 void BSP_CAMERA_PwrDown(void) 00317 { 00318 GPIO_InitTypeDef gpio_init_structure; 00319 00320 /* Enable GPIO clock */ 00321 __HAL_RCC_GPIOH_CLK_ENABLE(); 00322 00323 /*** Configure the GPIO ***/ 00324 /* Configure DCMI GPIO as alternate function */ 00325 gpio_init_structure.Pin = GPIO_PIN_13; 00326 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00327 gpio_init_structure.Pull = GPIO_NOPULL; 00328 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00329 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00330 00331 /* Assert the camera POWER_DOWN pin (active high) */ 00332 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_13, GPIO_PIN_SET); 00333 } 00334 00335 /** 00336 * @brief Configures the camera contrast and brightness. 00337 * @param contrast_level: Contrast level 00338 * This parameter can be one of the following values: 00339 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00340 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00341 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00342 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00343 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00344 * @param brightness_level: Contrast level 00345 * This parameter can be one of the following values: 00346 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00347 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00348 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00349 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00350 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00351 * @retval None 00352 */ 00353 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00354 { 00355 if(camera_drv->Config != NULL) 00356 { 00357 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00358 } 00359 } 00360 00361 /** 00362 * @brief Configures the camera white balance. 00363 * @param Mode: black_white mode 00364 * This parameter can be one of the following values: 00365 * @arg CAMERA_BLACK_WHITE_BW 00366 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00367 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00368 * @arg CAMERA_BLACK_WHITE_NORMAL 00369 * @retval None 00370 */ 00371 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00372 { 00373 if(camera_drv->Config != NULL) 00374 { 00375 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00376 } 00377 } 00378 00379 /** 00380 * @brief Configures the camera color effect. 00381 * @param Effect: Color effect 00382 * This parameter can be one of the following values: 00383 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00384 * @arg CAMERA_COLOR_EFFECT_BLUE 00385 * @arg CAMERA_COLOR_EFFECT_GREEN 00386 * @arg CAMERA_COLOR_EFFECT_RED 00387 * @retval None 00388 */ 00389 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00390 { 00391 if(camera_drv->Config != NULL) 00392 { 00393 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00394 } 00395 } 00396 00397 00398 /** 00399 * @brief Handles DCMI interrupt request. 00400 * @retval None 00401 */ 00402 void BSP_CAMERA_IRQHandler(void) 00403 { 00404 HAL_DCMI_IRQHandler(&hDcmiHandler); 00405 } 00406 00407 /** 00408 * @brief Handles DMA interrupt request. 00409 * @retval None 00410 */ 00411 void BSP_CAMERA_DMA_IRQHandler(void) 00412 { 00413 HAL_DMA_IRQHandler(hDcmiHandler.DMA_Handle); 00414 } 00415 00416 /** 00417 * @brief Get the capture size in pixels unit. 00418 * @param resolution: the current resolution. 00419 * @retval capture size in pixels unit. 00420 */ 00421 static uint32_t GetSize(uint32_t resolution) 00422 { 00423 uint32_t size = 0; 00424 00425 /* Get capture size */ 00426 switch (resolution) 00427 { 00428 case CAMERA_R160x120: 00429 { 00430 size = 0x2580; 00431 } 00432 break; 00433 case CAMERA_R320x240: 00434 { 00435 size = 0x9600; 00436 } 00437 break; 00438 case CAMERA_R480x272: 00439 { 00440 size = 0xFF00; 00441 } 00442 break; 00443 case CAMERA_R640x480: 00444 { 00445 size = 0x25800; 00446 } 00447 break; 00448 default: 00449 { 00450 break; 00451 } 00452 } 00453 00454 return size; 00455 } 00456 00457 /** 00458 * @brief Initializes the DCMI MSP. 00459 * @param hdcmi: HDMI handle 00460 * @param Params 00461 * @retval None 00462 */ 00463 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00464 { 00465 static DMA_HandleTypeDef hdma_handler; 00466 GPIO_InitTypeDef gpio_init_structure; 00467 00468 /*** Enable peripherals and GPIO clocks ***/ 00469 /* Enable DCMI clock */ 00470 __HAL_RCC_DCMI_CLK_ENABLE(); 00471 00472 /* Enable DMA2 clock */ 00473 __HAL_RCC_DMA2_CLK_ENABLE(); 00474 00475 /* Enable GPIO clocks */ 00476 __HAL_RCC_GPIOA_CLK_ENABLE(); 00477 __HAL_RCC_GPIOD_CLK_ENABLE(); 00478 __HAL_RCC_GPIOE_CLK_ENABLE(); 00479 __HAL_RCC_GPIOG_CLK_ENABLE(); 00480 __HAL_RCC_GPIOH_CLK_ENABLE(); 00481 00482 /*** Configure the GPIO ***/ 00483 /* Configure DCMI GPIO as alternate function */ 00484 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00485 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00486 gpio_init_structure.Pull = GPIO_PULLUP; 00487 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00488 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00489 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00490 00491 gpio_init_structure.Pin = GPIO_PIN_3; 00492 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00493 gpio_init_structure.Pull = GPIO_PULLUP; 00494 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00495 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00496 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00497 00498 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00499 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00500 gpio_init_structure.Pull = GPIO_PULLUP; 00501 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00502 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00503 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00504 00505 gpio_init_structure.Pin = GPIO_PIN_9; 00506 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00507 gpio_init_structure.Pull = GPIO_PULLUP; 00508 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00509 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00510 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00511 00512 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00513 GPIO_PIN_12 | GPIO_PIN_14; 00514 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00515 gpio_init_structure.Pull = GPIO_PULLUP; 00516 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00517 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00518 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00519 00520 /*** Configure the DMA ***/ 00521 /* Set the parameters to be configured */ 00522 hdma_handler.Init.Channel = DMA_CHANNEL_1; 00523 hdma_handler.Init.Direction = DMA_PERIPH_TO_MEMORY; 00524 hdma_handler.Init.PeriphInc = DMA_PINC_DISABLE; 00525 hdma_handler.Init.MemInc = DMA_MINC_ENABLE; 00526 hdma_handler.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00527 hdma_handler.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00528 hdma_handler.Init.Mode = DMA_CIRCULAR; 00529 hdma_handler.Init.Priority = DMA_PRIORITY_HIGH; 00530 hdma_handler.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00531 hdma_handler.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00532 hdma_handler.Init.MemBurst = DMA_MBURST_SINGLE; 00533 hdma_handler.Init.PeriphBurst = DMA_PBURST_SINGLE; 00534 00535 hdma_handler.Instance = DMA2_Stream1; 00536 00537 /* Associate the initialized DMA handle to the DCMI handle */ 00538 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_handler); 00539 00540 /*** Configure the NVIC for DCMI and DMA ***/ 00541 /* NVIC configuration for DCMI transfer complete interrupt */ 00542 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00543 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00544 00545 /* NVIC configuration for DMA2D transfer complete interrupt */ 00546 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 0x0F, 0); 00547 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00548 00549 /* Configure the DMA stream */ 00550 HAL_DMA_Init(hdcmi->DMA_Handle); 00551 } 00552 00553 00554 /** 00555 * @brief DeInitializes the DCMI MSP. 00556 * @param hdcmi: HDMI handle 00557 * @param Params 00558 * @retval None 00559 */ 00560 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00561 { 00562 /* Disable NVIC for DCMI transfer complete interrupt */ 00563 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00564 00565 /* Disable NVIC for DMA2 transfer complete interrupt */ 00566 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00567 00568 /* Configure the DMA stream */ 00569 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00570 00571 /* Disable DCMI clock */ 00572 __HAL_RCC_DCMI_CLK_DISABLE(); 00573 00574 /* GPIO pins clock and DMA clock can be shut down in the application 00575 by surcharging this __weak function */ 00576 } 00577 00578 /** 00579 * @brief Line event callback 00580 * @param hdcmi: pointer to the DCMI handle 00581 * @retval None 00582 */ 00583 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00584 { 00585 BSP_CAMERA_LineEventCallback(); 00586 } 00587 00588 /** 00589 * @brief Line Event callback. 00590 * @retval None 00591 */ 00592 __weak void BSP_CAMERA_LineEventCallback(void) 00593 { 00594 /* NOTE : This function Should not be modified, when the callback is needed, 00595 the HAL_DCMI_LineEventCallback could be implemented in the user file 00596 */ 00597 } 00598 00599 /** 00600 * @brief VSYNC event callback 00601 * @param hdcmi: pointer to the DCMI handle 00602 * @retval None 00603 */ 00604 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00605 { 00606 BSP_CAMERA_VsyncEventCallback(); 00607 } 00608 00609 /** 00610 * @brief VSYNC Event callback. 00611 * @retval None 00612 */ 00613 __weak void BSP_CAMERA_VsyncEventCallback(void) 00614 { 00615 /* NOTE : This function Should not be modified, when the callback is needed, 00616 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00617 */ 00618 } 00619 00620 /** 00621 * @brief Frame event callback 00622 * @param hdcmi: pointer to the DCMI handle 00623 * @retval None 00624 */ 00625 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00626 { 00627 BSP_CAMERA_FrameEventCallback(); 00628 } 00629 00630 /** 00631 * @brief Frame Event callback. 00632 * @retval None 00633 */ 00634 __weak void BSP_CAMERA_FrameEventCallback(void) 00635 { 00636 /* NOTE : This function Should not be modified, when the callback is needed, 00637 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00638 */ 00639 } 00640 00641 /** 00642 * @brief Error callback 00643 * @param hdcmi: pointer to the DCMI handle 00644 * @retval None 00645 */ 00646 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00647 { 00648 BSP_CAMERA_ErrorCallback(); 00649 } 00650 00651 /** 00652 * @brief Error callback. 00653 * @retval None 00654 */ 00655 __weak void BSP_CAMERA_ErrorCallback(void) 00656 { 00657 /* NOTE : This function Should not be modified, when the callback is needed, 00658 the HAL_DCMI_ErrorCallback could be implemented in the user file 00659 */ 00660 } 00661 00662 /** 00663 * @} 00664 */ 00665 00666 /** 00667 * @} 00668 */ 00669 00670 /** 00671 * @} 00672 */ 00673 00674 /** 00675 * @} 00676 */ 00677 00678 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:20:42 by
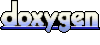