STM32746G-Discovery board drivers V1.0.0
Dependents: DISCO-F746NG_LCDTS_CC3000_NTP DISCO-F746NG_ROPE_WIFI F746_SpectralAnalysis_NoPhoto ecte433 ... more
stm32746g_discovery.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage LEDs, 00006 * push-buttons and COM ports available on STM32746G-Discovery 00007 * board(MB1191) from STMicroelectronics. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Dependencies 00039 - stm32f7xx_hal_cortex.c 00040 - stm32f7xx_hal_gpio.c 00041 - stm32f7xx_hal_uart.c 00042 - stm32f7xx_hal_i2c.c 00043 EndDependencies */ 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32746g_discovery.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32746G_DISCOVERY 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL STM32746G_DISCOVERY_LOW_LEVEL 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32746G_DISCOVERY_LOW_LEVEL Private Types Definitions 00061 * @{ 00062 */ 00063 /** 00064 * @} 00065 */ 00066 00067 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Defines STM32746G_DISCOVERY_LOW_LEVEL Private Defines 00068 * @{ 00069 */ 00070 /** 00071 * @brief STM32746G DISCOVERY BSP Driver version number V2.0.2 00072 */ 00073 #define __STM32746G_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00074 #define __STM32746G_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00075 #define __STM32746G_DISCO_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00076 #define __STM32746G_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00077 #define __STM32746G_DISCO_BSP_VERSION ((__STM32746G_DISCO_BSP_VERSION_MAIN << 24)\ 00078 |(__STM32746G_DISCO_BSP_VERSION_SUB1 << 16)\ 00079 |(__STM32746G_DISCO_BSP_VERSION_SUB2 << 8 )\ 00080 |(__STM32746G_DISCO_BSP_VERSION_RC)) 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Macros STM32746G_DISCOVERY_LOW_LEVEL Private Macros 00086 * @{ 00087 */ 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Variables STM32746G_DISCOVERY_LOW_LEVEL Private Variables 00093 * @{ 00094 */ 00095 00096 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN}; 00097 00098 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00099 TAMPER_BUTTON_GPIO_PORT, 00100 KEY_BUTTON_GPIO_PORT}; 00101 00102 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00103 TAMPER_BUTTON_PIN, 00104 KEY_BUTTON_PIN}; 00105 00106 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00107 TAMPER_BUTTON_EXTI_IRQn, 00108 KEY_BUTTON_EXTI_IRQn}; 00109 00110 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00111 00112 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00113 00114 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00115 00116 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00117 00118 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00119 00120 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00121 00122 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00123 00124 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00125 static I2C_HandleTypeDef hI2cExtHandler = {0}; 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32746G_DISCOVERY_LOW_LEVEL Private Function Prototypes 00132 * @{ 00133 */ 00134 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00135 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00136 00137 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00138 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00139 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00140 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00141 00142 /* AUDIO IO functions */ 00143 void AUDIO_IO_Init(void); 00144 void AUDIO_IO_DeInit(void); 00145 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00146 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00147 void AUDIO_IO_Delay(uint32_t Delay); 00148 00149 /* TOUCHSCREEN IO functions */ 00150 void TS_IO_Init(void); 00151 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00152 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00153 void TS_IO_Delay(uint32_t Delay); 00154 00155 /* CAMERA IO functions */ 00156 void CAMERA_IO_Init(void); 00157 void CAMERA_Delay(uint32_t Delay); 00158 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00159 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00160 00161 /* I2C EEPROM IO function */ 00162 void EEPROM_IO_Init(void); 00163 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00164 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00165 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Exported_Functions STM32746G_DISCOVERY_LOW_LEVELSTM32746G_DISCOVERY_LOW_LEVEL Exported Functions 00171 * @{ 00172 */ 00173 00174 /** 00175 * @brief This method returns the STM32746G DISCOVERY BSP Driver revision 00176 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00177 */ 00178 uint32_t BSP_GetVersion(void) 00179 { 00180 return __STM32746G_DISCO_BSP_VERSION; 00181 } 00182 00183 /** 00184 * @brief Configures LED on GPIO. 00185 * @param Led: LED to be configured. 00186 * This parameter can be one of the following values: 00187 * @arg DISCO_LED1 00188 * @retval None 00189 */ 00190 void BSP_LED_Init(Led_TypeDef Led) 00191 { 00192 GPIO_InitTypeDef gpio_init_structure; 00193 GPIO_TypeDef* gpio_led; 00194 00195 if (Led == DISCO_LED1) // MBED 00196 { 00197 gpio_led = LED1_GPIO_PORT; 00198 /* Enable the GPIO_LED clock */ 00199 LED1_GPIO_CLK_ENABLE(); 00200 00201 /* Configure the GPIO_LED pin */ 00202 gpio_init_structure.Pin = GPIO_PIN[Led]; 00203 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00204 gpio_init_structure.Pull = GPIO_PULLUP; 00205 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00206 00207 HAL_GPIO_Init(gpio_led, &gpio_init_structure); 00208 00209 /* By default, turn off LED */ 00210 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00211 } 00212 } 00213 00214 /** 00215 * @brief DeInit LEDs. 00216 * @param Led: LED to be configured. 00217 * This parameter can be one of the following values: 00218 * @arg DISCO_LED1 00219 * @note Led DeInit does not disable the GPIO clock 00220 * @retval None 00221 */ 00222 void BSP_LED_DeInit(Led_TypeDef Led) 00223 { 00224 GPIO_InitTypeDef gpio_init_structure; 00225 GPIO_TypeDef* gpio_led; 00226 00227 if (Led == DISCO_LED1) // MBED 00228 { 00229 gpio_led = LED1_GPIO_PORT; 00230 /* Turn off LED */ 00231 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00232 /* Configure the GPIO_LED pin */ 00233 gpio_init_structure.Pin = GPIO_PIN[Led]; 00234 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00235 } 00236 } 00237 00238 /** 00239 * @brief Turns selected LED On. 00240 * @param Led: LED to be set on 00241 * This parameter can be one of the following values: 00242 * @arg DISCO_LED1 00243 * @retval None 00244 */ 00245 void BSP_LED_On(Led_TypeDef Led) 00246 { 00247 GPIO_TypeDef* gpio_led; 00248 00249 if (Led == DISCO_LED1) /* Switch On LED connected to GPIO */ // MBED 00250 { 00251 gpio_led = LED1_GPIO_PORT; 00252 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00253 } 00254 } 00255 00256 /** 00257 * @brief Turns selected LED Off. 00258 * @param Led: LED to be set off 00259 * This parameter can be one of the following values: 00260 * @arg DISCO_LED1 00261 * @retval None 00262 */ 00263 void BSP_LED_Off(Led_TypeDef Led) 00264 { 00265 GPIO_TypeDef* gpio_led; 00266 00267 if (Led == DISCO_LED1) /* Switch Off LED connected to GPIO */ // MBED 00268 { 00269 gpio_led = LED1_GPIO_PORT; 00270 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00271 } 00272 } 00273 00274 /** 00275 * @brief Toggles the selected LED. 00276 * @param Led: LED to be toggled 00277 * This parameter can be one of the following values: 00278 * @arg DISCO_LED1 00279 * @retval None 00280 */ 00281 void BSP_LED_Toggle(Led_TypeDef Led) 00282 { 00283 GPIO_TypeDef* gpio_led; 00284 00285 if (Led == DISCO_LED1) /* Toggle LED connected to GPIO */ // MBED 00286 { 00287 gpio_led = LED1_GPIO_PORT; 00288 HAL_GPIO_TogglePin(gpio_led, GPIO_PIN[Led]); 00289 } 00290 } 00291 00292 /** 00293 * @brief Configures button GPIO and EXTI Line. 00294 * @param Button: Button to be configured 00295 * This parameter can be one of the following values: 00296 * @arg BUTTON_WAKEUP: Wakeup Push Button 00297 * @arg BUTTON_TAMPER: Tamper Push Button 00298 * @arg BUTTON_KEY: Key Push Button 00299 * @param ButtonMode: Button mode 00300 * This parameter can be one of the following values: 00301 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00302 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00303 * with interrupt generation capability 00304 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00305 * are mapped on the same push button named "User" 00306 * on the board serigraphy. 00307 * @retval None 00308 */ 00309 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00310 { 00311 GPIO_InitTypeDef gpio_init_structure; 00312 00313 /* Enable the BUTTON clock */ 00314 BUTTONx_GPIO_CLK_ENABLE(Button); 00315 00316 if(ButtonMode == BUTTON_MODE_GPIO) 00317 { 00318 /* Configure Button pin as input */ 00319 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00320 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00321 gpio_init_structure.Pull = GPIO_NOPULL; 00322 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00323 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00324 } 00325 00326 if(ButtonMode == BUTTON_MODE_EXTI) 00327 { 00328 /* Configure Button pin as input with External interrupt */ 00329 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00330 gpio_init_structure.Pull = GPIO_NOPULL; 00331 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00332 00333 if(Button != BUTTON_WAKEUP) 00334 { 00335 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00336 } 00337 else 00338 { 00339 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00340 } 00341 00342 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00343 00344 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00345 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00346 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00347 } 00348 } 00349 00350 /** 00351 * @brief Push Button DeInit. 00352 * @param Button: Button to be configured 00353 * This parameter can be one of the following values: 00354 * @arg BUTTON_WAKEUP: Wakeup Push Button 00355 * @arg BUTTON_TAMPER: Tamper Push Button 00356 * @arg BUTTON_KEY: Key Push Button 00357 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00358 * are mapped on the same push button named "User" 00359 * on the board serigraphy. 00360 * @note PB DeInit does not disable the GPIO clock 00361 * @retval None 00362 */ 00363 void BSP_PB_DeInit(Button_TypeDef Button) 00364 { 00365 GPIO_InitTypeDef gpio_init_structure; 00366 00367 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00368 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00369 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00370 } 00371 00372 00373 /** 00374 * @brief Returns the selected button state. 00375 * @param Button: Button to be checked 00376 * This parameter can be one of the following values: 00377 * @arg BUTTON_WAKEUP: Wakeup Push Button 00378 * @arg BUTTON_TAMPER: Tamper Push Button 00379 * @arg BUTTON_KEY: Key Push Button 00380 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00381 * are mapped on the same push button named "User" 00382 * on the board serigraphy. 00383 * @retval The Button GPIO pin value 00384 */ 00385 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00386 { 00387 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00388 } 00389 00390 /** 00391 * @brief Configures COM port. 00392 * @param COM: COM port to be configured. 00393 * This parameter can be one of the following values: 00394 * @arg COM1 00395 * @arg COM2 00396 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00397 * configuration information for the specified USART peripheral. 00398 * @retval None 00399 */ 00400 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00401 { 00402 GPIO_InitTypeDef gpio_init_structure; 00403 00404 /* Enable GPIO clock */ 00405 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00406 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00407 00408 /* Enable USART clock */ 00409 DISCOVERY_COMx_CLK_ENABLE(COM); 00410 00411 /* Configure USART Tx as alternate function */ 00412 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00413 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00414 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00415 gpio_init_structure.Pull = GPIO_PULLUP; 00416 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00417 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00418 00419 /* Configure USART Rx as alternate function */ 00420 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00421 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00422 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00423 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00424 00425 /* USART configuration */ 00426 huart->Instance = COM_USART[COM]; 00427 HAL_UART_Init(huart); 00428 } 00429 00430 /** 00431 * @brief DeInit COM port. 00432 * @param COM: COM port to be configured. 00433 * This parameter can be one of the following values: 00434 * @arg COM1 00435 * @arg COM2 00436 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00437 * configuration information for the specified USART peripheral. 00438 * @retval None 00439 */ 00440 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00441 { 00442 /* USART configuration */ 00443 huart->Instance = COM_USART[COM]; 00444 HAL_UART_DeInit(huart); 00445 00446 /* Enable USART clock */ 00447 DISCOVERY_COMx_CLK_DISABLE(COM); 00448 00449 /* DeInit GPIO pins can be done in the application 00450 (by surcharging this __weak function) */ 00451 00452 /* GPIO pins clock, DMA clock can be shut down in the application 00453 by surcharging this __weak function */ 00454 } 00455 00456 /******************************************************************************* 00457 BUS OPERATIONS 00458 *******************************************************************************/ 00459 00460 /******************************* I2C Routines *********************************/ 00461 /** 00462 * @brief Initializes I2C MSP. 00463 * @param i2c_handler : I2C handler 00464 * @retval None 00465 */ 00466 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00467 { 00468 GPIO_InitTypeDef gpio_init_structure; 00469 00470 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00471 { 00472 /* AUDIO and LCD I2C MSP init */ 00473 00474 /*** Configure the GPIOs ***/ 00475 /* Enable GPIO clock */ 00476 DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00477 00478 /* Configure I2C Tx as alternate function */ 00479 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00480 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00481 gpio_init_structure.Pull = GPIO_NOPULL; 00482 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00483 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00484 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00485 00486 /* Configure I2C Rx as alternate function */ 00487 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00488 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00489 00490 /*** Configure the I2C peripheral ***/ 00491 /* Enable I2C clock */ 00492 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00493 00494 /* Force the I2C peripheral clock reset */ 00495 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00496 00497 /* Release the I2C peripheral clock reset */ 00498 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00499 00500 /* Enable and set I2Cx Interrupt to a lower priority */ 00501 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00502 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00503 00504 /* Enable and set I2Cx Interrupt to a lower priority */ 00505 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00506 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00507 } 00508 else 00509 { 00510 /* External, camera and Arduino connector I2C MSP init */ 00511 00512 /*** Configure the GPIOs ***/ 00513 /* Enable GPIO clock */ 00514 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00515 00516 /* Configure I2C Tx as alternate function */ 00517 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00518 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00519 gpio_init_structure.Pull = GPIO_NOPULL; 00520 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00521 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00522 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00523 00524 /* Configure I2C Rx as alternate function */ 00525 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00526 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00527 00528 /*** Configure the I2C peripheral ***/ 00529 /* Enable I2C clock */ 00530 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00531 00532 /* Force the I2C peripheral clock reset */ 00533 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00534 00535 /* Release the I2C peripheral clock reset */ 00536 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00537 00538 /* Enable and set I2Cx Interrupt to a lower priority */ 00539 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00540 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00541 00542 /* Enable and set I2Cx Interrupt to a lower priority */ 00543 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00544 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00545 } 00546 } 00547 00548 /** 00549 * @brief Initializes I2C HAL. 00550 * @param i2c_handler : I2C handler 00551 * @retval None 00552 */ 00553 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00554 { 00555 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00556 { 00557 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00558 { 00559 /* Audio and LCD I2C configuration */ 00560 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00561 } 00562 else 00563 { 00564 /* External, camera and Arduino connector I2C configuration */ 00565 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00566 } 00567 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00568 i2c_handler->Init.OwnAddress1 = 0; 00569 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00570 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00571 i2c_handler->Init.OwnAddress2 = 0; 00572 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00573 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00574 00575 /* Init the I2C */ 00576 I2Cx_MspInit(i2c_handler); 00577 HAL_I2C_Init(i2c_handler); 00578 } 00579 } 00580 00581 /** 00582 * @brief Reads multiple data. 00583 * @param i2c_handler : I2C handler 00584 * @param Addr: I2C address 00585 * @param Reg: Reg address 00586 * @param MemAddress: Memory address 00587 * @param Buffer: Pointer to data buffer 00588 * @param Length: Length of the data 00589 * @retval Number of read data 00590 */ 00591 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, 00592 uint8_t Addr, 00593 uint16_t Reg, 00594 uint16_t MemAddress, 00595 uint8_t *Buffer, 00596 uint16_t Length) 00597 { 00598 HAL_StatusTypeDef status = HAL_OK; 00599 00600 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00601 00602 /* Check the communication status */ 00603 if(status != HAL_OK) 00604 { 00605 /* I2C error occurred */ 00606 I2Cx_Error(i2c_handler, Addr); 00607 } 00608 return status; 00609 } 00610 00611 /** 00612 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00613 * @param i2c_handler : I2C handler 00614 * @param Addr: Device address on BUS Bus. 00615 * @param Reg: The target register address to write 00616 * @param MemAddress: Memory address 00617 * @param Buffer: The target register value to be written 00618 * @param Length: buffer size to be written 00619 * @retval HAL status 00620 */ 00621 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, 00622 uint8_t Addr, 00623 uint16_t Reg, 00624 uint16_t MemAddress, 00625 uint8_t *Buffer, 00626 uint16_t Length) 00627 { 00628 HAL_StatusTypeDef status = HAL_OK; 00629 00630 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00631 00632 /* Check the communication status */ 00633 if(status != HAL_OK) 00634 { 00635 /* Re-Initiaize the I2C Bus */ 00636 I2Cx_Error(i2c_handler, Addr); 00637 } 00638 return status; 00639 } 00640 00641 /** 00642 * @brief Checks if target device is ready for communication. 00643 * @note This function is used with Memory devices 00644 * @param i2c_handler : I2C handler 00645 * @param DevAddress: Target device address 00646 * @param Trials: Number of trials 00647 * @retval HAL status 00648 */ 00649 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00650 { 00651 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00652 } 00653 00654 /** 00655 * @brief Manages error callback by re-initializing I2C. 00656 * @param i2c_handler : I2C handler 00657 * @param Addr: I2C Address 00658 * @retval None 00659 */ 00660 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00661 { 00662 /* De-initialize the I2C communication bus */ 00663 HAL_I2C_DeInit(i2c_handler); 00664 00665 /* Re-Initialize the I2C communication bus */ 00666 I2Cx_Init(i2c_handler); 00667 } 00668 00669 /******************************************************************************* 00670 LINK OPERATIONS 00671 *******************************************************************************/ 00672 00673 /********************************* LINK AUDIO *********************************/ 00674 00675 /** 00676 * @brief Initializes Audio low level. 00677 * @retval None 00678 */ 00679 void AUDIO_IO_Init(void) 00680 { 00681 I2Cx_Init(&hI2cAudioHandler); 00682 } 00683 00684 /** 00685 * @brief Deinitializes Audio low level. 00686 * @retval None 00687 */ 00688 void AUDIO_IO_DeInit(void) 00689 { 00690 } 00691 00692 /** 00693 * @brief Writes a single data. 00694 * @param Addr: I2C address 00695 * @param Reg: Reg address 00696 * @param Value: Data to be written 00697 * @retval None 00698 */ 00699 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00700 { 00701 uint16_t tmp = Value; 00702 00703 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00704 00705 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00706 00707 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00708 } 00709 00710 /** 00711 * @brief Reads a single data. 00712 * @param Addr: I2C address 00713 * @param Reg: Reg address 00714 * @retval Data to be read 00715 */ 00716 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00717 { 00718 uint16_t read_value = 0, tmp = 0; 00719 00720 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00721 00722 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00723 00724 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00725 00726 read_value = tmp; 00727 00728 return read_value; 00729 } 00730 00731 /** 00732 * @brief AUDIO Codec delay 00733 * @param Delay: Delay in ms 00734 * @retval None 00735 */ 00736 void AUDIO_IO_Delay(uint32_t Delay) 00737 { 00738 HAL_Delay(Delay); 00739 } 00740 00741 /********************************* LINK CAMERA ********************************/ 00742 00743 /** 00744 * @brief Initializes Camera low level. 00745 * @retval None 00746 */ 00747 void CAMERA_IO_Init(void) 00748 { 00749 I2Cx_Init(&hI2cExtHandler); 00750 } 00751 00752 /** 00753 * @brief Camera writes single data. 00754 * @param Addr: I2C address 00755 * @param Reg: Register address 00756 * @param Value: Data to be written 00757 * @retval None 00758 */ 00759 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00760 { 00761 I2Cx_WriteMultiple(&hI2cExtHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00762 } 00763 00764 /** 00765 * @brief Camera reads single data. 00766 * @param Addr: I2C address 00767 * @param Reg: Register address 00768 * @retval Read data 00769 */ 00770 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 00771 { 00772 uint8_t read_value = 0; 00773 00774 I2Cx_ReadMultiple(&hI2cExtHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00775 00776 return read_value; 00777 } 00778 00779 /** 00780 * @brief Camera delay 00781 * @param Delay: Delay in ms 00782 * @retval None 00783 */ 00784 void CAMERA_Delay(uint32_t Delay) 00785 { 00786 HAL_Delay(Delay); 00787 } 00788 00789 /******************************** LINK I2C EEPROM *****************************/ 00790 00791 /** 00792 * @brief Initializes peripherals used by the I2C EEPROM driver. 00793 * @retval None 00794 */ 00795 void EEPROM_IO_Init(void) 00796 { 00797 I2Cx_Init(&hI2cExtHandler); 00798 } 00799 00800 /** 00801 * @brief Write data to I2C EEPROM driver in using DMA channel. 00802 * @param DevAddress: Target device address 00803 * @param MemAddress: Internal memory address 00804 * @param pBuffer: Pointer to data buffer 00805 * @param BufferSize: Amount of data to be sent 00806 * @retval HAL status 00807 */ 00808 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00809 { 00810 return (I2Cx_WriteMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00811 } 00812 00813 /** 00814 * @brief Read data from I2C EEPROM driver in using DMA channel. 00815 * @param DevAddress: Target device address 00816 * @param MemAddress: Internal memory address 00817 * @param pBuffer: Pointer to data buffer 00818 * @param BufferSize: Amount of data to be read 00819 * @retval HAL status 00820 */ 00821 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00822 { 00823 return (I2Cx_ReadMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00824 } 00825 00826 /** 00827 * @brief Checks if target device is ready for communication. 00828 * @note This function is used with Memory devices 00829 * @param DevAddress: Target device address 00830 * @param Trials: Number of trials 00831 * @retval HAL status 00832 */ 00833 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00834 { 00835 return (I2Cx_IsDeviceReady(&hI2cExtHandler, DevAddress, Trials)); 00836 } 00837 00838 /********************************* LINK TOUCHSCREEN *********************************/ 00839 00840 /** 00841 * @brief Initializes Touchscreen low level. 00842 * @retval None 00843 */ 00844 void TS_IO_Init(void) 00845 { 00846 I2Cx_Init(&hI2cAudioHandler); 00847 } 00848 00849 /** 00850 * @brief Writes a single data. 00851 * @param Addr: I2C address 00852 * @param Reg: Reg address 00853 * @param Value: Data to be written 00854 * @retval None 00855 */ 00856 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00857 { 00858 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00859 } 00860 00861 /** 00862 * @brief Reads a single data. 00863 * @param Addr: I2C address 00864 * @param Reg: Reg address 00865 * @retval Data to be read 00866 */ 00867 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00868 { 00869 uint8_t read_value = 0; 00870 00871 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00872 00873 return read_value; 00874 } 00875 00876 /** 00877 * @brief TS delay 00878 * @param Delay: Delay in ms 00879 * @retval None 00880 */ 00881 void TS_IO_Delay(uint32_t Delay) 00882 { 00883 HAL_Delay(Delay); 00884 } 00885 00886 /** 00887 * @} 00888 */ 00889 00890 /** 00891 * @} 00892 */ 00893 00894 /** 00895 * @} 00896 */ 00897 00898 /** 00899 * @} 00900 */ 00901 00902 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:20:42 by
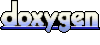