STM32746G-Discovery board drivers V1.0.0
Dependents: DISCO-F746NG_LCDTS_CC3000_NTP DISCO-F746NG_ROPE_WIFI F746_SpectralAnalysis_NoPhoto ecte433 ... more
ft5336.h
00001 /** 00002 ****************************************************************************** 00003 * @file ft5336.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * ft5336.c Touch screen driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __FT5336_H 00039 #define __FT5336_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Set Multi-touch as supported */ 00046 #if !defined(TS_MONO_TOUCH_SUPPORTED) 00047 #define TS_MULTI_TOUCH_SUPPORTED 1 00048 #endif /* TS_MONO_TOUCH_SUPPORTED */ 00049 00050 /* Includes ------------------------------------------------------------------*/ 00051 #include "../Common/ts.h" 00052 00053 /* Macros --------------------------------------------------------------------*/ 00054 00055 #if defined(FT5336_ENABLE_ASSERT) 00056 /* Assert activated */ 00057 #define FT5336_ASSERT(__condition__) do { if(__condition__) \ 00058 { \ 00059 while(1); \ 00060 } \ 00061 }while(0) 00062 #else 00063 /* Assert not activated : macro has no effect */ 00064 #define FT5336_ASSERT(__condition__) do { if(__condition__) \ 00065 { \ 00066 ; \ 00067 } \ 00068 }while(0) 00069 #endif /* FT5336_ENABLE_ASSERT == 1 */ 00070 00071 /** @typedef ft5336_handle_TypeDef 00072 * ft5336 Handle definition. 00073 */ 00074 typedef struct 00075 { 00076 uint8_t i2cInitialized; 00077 00078 /* field holding the current number of simultaneous active touches */ 00079 uint8_t currActiveTouchNb; 00080 00081 /* field holding the touch index currently managed */ 00082 uint8_t currActiveTouchIdx; 00083 00084 } ft5336_handle_TypeDef; 00085 00086 /** @addtogroup BSP 00087 * @{ 00088 */ 00089 00090 /** @addtogroup Component 00091 * @{ 00092 */ 00093 00094 /** @defgroup FT5336 00095 * @{ 00096 */ 00097 00098 /* Exported types ------------------------------------------------------------*/ 00099 00100 /** @defgroup FT5336_Exported_Types 00101 * @{ 00102 */ 00103 00104 /* Exported constants --------------------------------------------------------*/ 00105 00106 /** @defgroup FT5336_Exported_Constants 00107 * @{ 00108 */ 00109 00110 /* I2C Slave address of touchscreen FocalTech FT5336 */ 00111 #define FT5336_I2C_SLAVE_ADDRESS ((uint8_t)0x70) 00112 00113 /* Maximum border values of the touchscreen pad */ 00114 #define FT5336_MAX_WIDTH ((uint16_t)480) /* Touchscreen pad max width */ 00115 #define FT5336_MAX_HEIGHT ((uint16_t)272) /* Touchscreen pad max height */ 00116 00117 /* Possible values of driver functions return status */ 00118 #define FT5336_STATUS_OK ((uint8_t)0x00) 00119 #define FT5336_STATUS_NOT_OK ((uint8_t)0x01) 00120 00121 /* Possible values of global variable 'TS_I2C_Initialized' */ 00122 #define FT5336_I2C_NOT_INITIALIZED ((uint8_t)0x00) 00123 #define FT5336_I2C_INITIALIZED ((uint8_t)0x01) 00124 00125 /* Max detectable simultaneous touches */ 00126 #define FT5336_MAX_DETECTABLE_TOUCH ((uint8_t)0x05) 00127 00128 /** 00129 * @brief : Definitions for FT5336 I2C register addresses on 8 bit 00130 **/ 00131 00132 /* Current mode register of the FT5336 (R/W) */ 00133 #define FT5336_DEV_MODE_REG ((uint8_t)0x00) 00134 00135 /* Possible values of FT5336_DEV_MODE_REG */ 00136 #define FT5336_DEV_MODE_WORKING ((uint8_t)0x00) 00137 #define FT5336_DEV_MODE_FACTORY ((uint8_t)0x04) 00138 00139 #define FT5336_DEV_MODE_MASK ((uint8_t)0x07) 00140 #define FT5336_DEV_MODE_SHIFT ((uint8_t)0x04) 00141 00142 /* Gesture ID register */ 00143 #define FT5336_GEST_ID_REG ((uint8_t)0x01) 00144 00145 /* Possible values of FT5336_GEST_ID_REG */ 00146 #define FT5336_GEST_ID_NO_GESTURE ((uint8_t)0x00) 00147 #define FT5336_GEST_ID_MOVE_UP ((uint8_t)0x10) 00148 #define FT5336_GEST_ID_MOVE_RIGHT ((uint8_t)0x14) 00149 #define FT5336_GEST_ID_MOVE_DOWN ((uint8_t)0x18) 00150 #define FT5336_GEST_ID_MOVE_LEFT ((uint8_t)0x1C) 00151 #define FT5336_GEST_ID_SINGLE_CLICK ((uint8_t)0x20) 00152 #define FT5336_GEST_ID_DOUBLE_CLICK ((uint8_t)0x22) 00153 #define FT5336_GEST_ID_ROTATE_CLOCKWISE ((uint8_t)0x28) 00154 #define FT5336_GEST_ID_ROTATE_C_CLOCKWISE ((uint8_t)0x29) 00155 #define FT5336_GEST_ID_ZOOM_IN ((uint8_t)0x40) 00156 #define FT5336_GEST_ID_ZOOM_OUT ((uint8_t)0x49) 00157 00158 /* Touch Data Status register : gives number of active touch points (0..5) */ 00159 #define FT5336_TD_STAT_REG ((uint8_t)0x02) 00160 00161 /* Values related to FT5336_TD_STAT_REG */ 00162 #define FT5336_TD_STAT_MASK ((uint8_t)0x0F) 00163 #define FT5336_TD_STAT_SHIFT ((uint8_t)0x00) 00164 00165 /* Values Pn_XH and Pn_YH related */ 00166 #define FT5336_TOUCH_EVT_FLAG_PRESS_DOWN ((uint8_t)0x00) 00167 #define FT5336_TOUCH_EVT_FLAG_LIFT_UP ((uint8_t)0x01) 00168 #define FT5336_TOUCH_EVT_FLAG_CONTACT ((uint8_t)0x02) 00169 #define FT5336_TOUCH_EVT_FLAG_NO_EVENT ((uint8_t)0x03) 00170 00171 #define FT5336_TOUCH_EVT_FLAG_SHIFT ((uint8_t)0x06) 00172 #define FT5336_TOUCH_EVT_FLAG_MASK ((uint8_t)(3 << FT5336_TOUCH_EVT_FLAG_SHIFT)) 00173 00174 #define FT5336_TOUCH_POS_MSB_MASK ((uint8_t)0x0F) 00175 #define FT5336_TOUCH_POS_MSB_SHIFT ((uint8_t)0x00) 00176 00177 /* Values Pn_XL and Pn_YL related */ 00178 #define FT5336_TOUCH_POS_LSB_MASK ((uint8_t)0xFF) 00179 #define FT5336_TOUCH_POS_LSB_SHIFT ((uint8_t)0x00) 00180 00181 #define FT5336_P1_XH_REG ((uint8_t)0x03) 00182 #define FT5336_P1_XL_REG ((uint8_t)0x04) 00183 #define FT5336_P1_YH_REG ((uint8_t)0x05) 00184 #define FT5336_P1_YL_REG ((uint8_t)0x06) 00185 00186 /* Touch Pressure register value (R) */ 00187 #define FT5336_P1_WEIGHT_REG ((uint8_t)0x07) 00188 00189 /* Values Pn_WEIGHT related */ 00190 #define FT5336_TOUCH_WEIGHT_MASK ((uint8_t)0xFF) 00191 #define FT5336_TOUCH_WEIGHT_SHIFT ((uint8_t)0x00) 00192 00193 /* Touch area register */ 00194 #define FT5336_P1_MISC_REG ((uint8_t)0x08) 00195 00196 /* Values related to FT5336_Pn_MISC_REG */ 00197 #define FT5336_TOUCH_AREA_MASK ((uint8_t)(0x04 << 4)) 00198 #define FT5336_TOUCH_AREA_SHIFT ((uint8_t)0x04) 00199 00200 #define FT5336_P2_XH_REG ((uint8_t)0x09) 00201 #define FT5336_P2_XL_REG ((uint8_t)0x0A) 00202 #define FT5336_P2_YH_REG ((uint8_t)0x0B) 00203 #define FT5336_P2_YL_REG ((uint8_t)0x0C) 00204 #define FT5336_P2_WEIGHT_REG ((uint8_t)0x0D) 00205 #define FT5336_P2_MISC_REG ((uint8_t)0x0E) 00206 00207 #define FT5336_P3_XH_REG ((uint8_t)0x0F) 00208 #define FT5336_P3_XL_REG ((uint8_t)0x10) 00209 #define FT5336_P3_YH_REG ((uint8_t)0x11) 00210 #define FT5336_P3_YL_REG ((uint8_t)0x12) 00211 #define FT5336_P3_WEIGHT_REG ((uint8_t)0x13) 00212 #define FT5336_P3_MISC_REG ((uint8_t)0x14) 00213 00214 #define FT5336_P4_XH_REG ((uint8_t)0x15) 00215 #define FT5336_P4_XL_REG ((uint8_t)0x16) 00216 #define FT5336_P4_YH_REG ((uint8_t)0x17) 00217 #define FT5336_P4_YL_REG ((uint8_t)0x18) 00218 #define FT5336_P4_WEIGHT_REG ((uint8_t)0x19) 00219 #define FT5336_P4_MISC_REG ((uint8_t)0x1A) 00220 00221 #define FT5336_P5_XH_REG ((uint8_t)0x1B) 00222 #define FT5336_P5_XL_REG ((uint8_t)0x1C) 00223 #define FT5336_P5_YH_REG ((uint8_t)0x1D) 00224 #define FT5336_P5_YL_REG ((uint8_t)0x1E) 00225 #define FT5336_P5_WEIGHT_REG ((uint8_t)0x1F) 00226 #define FT5336_P5_MISC_REG ((uint8_t)0x20) 00227 00228 #define FT5336_P6_XH_REG ((uint8_t)0x21) 00229 #define FT5336_P6_XL_REG ((uint8_t)0x22) 00230 #define FT5336_P6_YH_REG ((uint8_t)0x23) 00231 #define FT5336_P6_YL_REG ((uint8_t)0x24) 00232 #define FT5336_P6_WEIGHT_REG ((uint8_t)0x25) 00233 #define FT5336_P6_MISC_REG ((uint8_t)0x26) 00234 00235 #define FT5336_P7_XH_REG ((uint8_t)0x27) 00236 #define FT5336_P7_XL_REG ((uint8_t)0x28) 00237 #define FT5336_P7_YH_REG ((uint8_t)0x29) 00238 #define FT5336_P7_YL_REG ((uint8_t)0x2A) 00239 #define FT5336_P7_WEIGHT_REG ((uint8_t)0x2B) 00240 #define FT5336_P7_MISC_REG ((uint8_t)0x2C) 00241 00242 #define FT5336_P8_XH_REG ((uint8_t)0x2D) 00243 #define FT5336_P8_XL_REG ((uint8_t)0x2E) 00244 #define FT5336_P8_YH_REG ((uint8_t)0x2F) 00245 #define FT5336_P8_YL_REG ((uint8_t)0x30) 00246 #define FT5336_P8_WEIGHT_REG ((uint8_t)0x31) 00247 #define FT5336_P8_MISC_REG ((uint8_t)0x32) 00248 00249 #define FT5336_P9_XH_REG ((uint8_t)0x33) 00250 #define FT5336_P9_XL_REG ((uint8_t)0x34) 00251 #define FT5336_P9_YH_REG ((uint8_t)0x35) 00252 #define FT5336_P9_YL_REG ((uint8_t)0x36) 00253 #define FT5336_P9_WEIGHT_REG ((uint8_t)0x37) 00254 #define FT5336_P9_MISC_REG ((uint8_t)0x38) 00255 00256 #define FT5336_P10_XH_REG ((uint8_t)0x39) 00257 #define FT5336_P10_XL_REG ((uint8_t)0x3A) 00258 #define FT5336_P10_YH_REG ((uint8_t)0x3B) 00259 #define FT5336_P10_YL_REG ((uint8_t)0x3C) 00260 #define FT5336_P10_WEIGHT_REG ((uint8_t)0x3D) 00261 #define FT5336_P10_MISC_REG ((uint8_t)0x3E) 00262 00263 /* Threshold for touch detection */ 00264 #define FT5336_TH_GROUP_REG ((uint8_t)0x80) 00265 00266 /* Values FT5336_TH_GROUP_REG : threshold related */ 00267 #define FT5336_THRESHOLD_MASK ((uint8_t)0xFF) 00268 #define FT5336_THRESHOLD_SHIFT ((uint8_t)0x00) 00269 00270 /* Filter function coefficients */ 00271 #define FT5336_TH_DIFF_REG ((uint8_t)0x85) 00272 00273 /* Control register */ 00274 #define FT5336_CTRL_REG ((uint8_t)0x86) 00275 00276 /* Values related to FT5336_CTRL_REG */ 00277 00278 /* Will keep the Active mode when there is no touching */ 00279 #define FT5336_CTRL_KEEP_ACTIVE_MODE ((uint8_t)0x00) 00280 00281 /* Switching from Active mode to Monitor mode automatically when there is no touching */ 00282 #define FT5336_CTRL_KEEP_AUTO_SWITCH_MONITOR_MODE ((uint8_t)0x01 00283 00284 /* The time period of switching from Active mode to Monitor mode when there is no touching */ 00285 #define FT5336_TIMEENTERMONITOR_REG ((uint8_t)0x87) 00286 00287 /* Report rate in Active mode */ 00288 #define FT5336_PERIODACTIVE_REG ((uint8_t)0x88) 00289 00290 /* Report rate in Monitor mode */ 00291 #define FT5336_PERIODMONITOR_REG ((uint8_t)0x89) 00292 00293 /* The value of the minimum allowed angle while Rotating gesture mode */ 00294 #define FT5336_RADIAN_VALUE_REG ((uint8_t)0x91) 00295 00296 /* Maximum offset while Moving Left and Moving Right gesture */ 00297 #define FT5336_OFFSET_LEFT_RIGHT_REG ((uint8_t)0x92) 00298 00299 /* Maximum offset while Moving Up and Moving Down gesture */ 00300 #define FT5336_OFFSET_UP_DOWN_REG ((uint8_t)0x93) 00301 00302 /* Minimum distance while Moving Left and Moving Right gesture */ 00303 #define FT5336_DISTANCE_LEFT_RIGHT_REG ((uint8_t)0x94) 00304 00305 /* Minimum distance while Moving Up and Moving Down gesture */ 00306 #define FT5336_DISTANCE_UP_DOWN_REG ((uint8_t)0x95) 00307 00308 /* Maximum distance while Zoom In and Zoom Out gesture */ 00309 #define FT5336_DISTANCE_ZOOM_REG ((uint8_t)0x96) 00310 00311 /* High 8-bit of LIB Version info */ 00312 #define FT5336_LIB_VER_H_REG ((uint8_t)0xA1) 00313 00314 /* Low 8-bit of LIB Version info */ 00315 #define FT5336_LIB_VER_L_REG ((uint8_t)0xA2) 00316 00317 /* Chip Selecting */ 00318 #define FT5336_CIPHER_REG ((uint8_t)0xA3) 00319 00320 /* Interrupt mode register (used when in interrupt mode) */ 00321 #define FT5336_GMODE_REG ((uint8_t)0xA4) 00322 00323 #define FT5336_G_MODE_INTERRUPT_MASK ((uint8_t)0x03) 00324 #define FT5336_G_MODE_INTERRUPT_SHIFT ((uint8_t)0x00) 00325 00326 /* Possible values of FT5336_GMODE_REG */ 00327 #define FT5336_G_MODE_INTERRUPT_POLLING ((uint8_t)0x00) 00328 #define FT5336_G_MODE_INTERRUPT_TRIGGER ((uint8_t)0x01) 00329 00330 /* Current power mode the FT5336 system is in (R) */ 00331 #define FT5336_PWR_MODE_REG ((uint8_t)0xA5) 00332 00333 /* FT5336 firmware version */ 00334 #define FT5336_FIRMID_REG ((uint8_t)0xA6) 00335 00336 /* FT5336 Chip identification register */ 00337 #define FT5336_CHIP_ID_REG ((uint8_t)0xA8) 00338 00339 /* Possible values of FT5336_CHIP_ID_REG */ 00340 #define FT5336_ID_VALUE ((uint8_t)0x51) 00341 00342 /* Release code version */ 00343 #define FT5336_RELEASE_CODE_ID_REG ((uint8_t)0xAF) 00344 00345 /* Current operating mode the FT5336 system is in (R) */ 00346 #define FT5336_STATE_REG ((uint8_t)0xBC) 00347 00348 /** 00349 * @} 00350 */ 00351 00352 /* Exported macro ------------------------------------------------------------*/ 00353 00354 /** @defgroup ft5336_Exported_Macros 00355 * @{ 00356 */ 00357 00358 /* Exported functions --------------------------------------------------------*/ 00359 00360 /** @defgroup ft5336_Exported_Functions 00361 * @{ 00362 */ 00363 00364 /** 00365 * @brief ft5336 Control functions 00366 */ 00367 00368 00369 /** 00370 * @brief Initialize the ft5336 communication bus 00371 * from MCU to FT5336 : ie I2C channel initialization (if required). 00372 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00373 * @retval None 00374 */ 00375 void ft5336_Init(uint16_t DeviceAddr); 00376 00377 /** 00378 * @brief Software Reset the ft5336. 00379 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00380 * @retval None 00381 */ 00382 void ft5336_Reset(uint16_t DeviceAddr); 00383 00384 /** 00385 * @brief Read the ft5336 device ID, pre initialize I2C in case of need to be 00386 * able to read the FT5336 device ID, and verify this is a FT5336. 00387 * @param DeviceAddr: I2C FT5336 Slave address. 00388 * @retval The Device ID (two bytes). 00389 */ 00390 uint16_t ft5336_ReadID(uint16_t DeviceAddr); 00391 00392 /** 00393 * @brief Configures the touch Screen IC device to start detecting touches 00394 * @param DeviceAddr: Device address on communication Bus (I2C slave address). 00395 * @retval None. 00396 */ 00397 void ft5336_TS_Start(uint16_t DeviceAddr); 00398 00399 /** 00400 * @brief Return if there is touches detected or not. 00401 * Try to detect new touches and forget the old ones (reset internal global 00402 * variables). 00403 * @param DeviceAddr: Device address on communication Bus. 00404 * @retval : Number of active touches detected (can be 0, 1 or 2). 00405 */ 00406 uint8_t ft5336_TS_DetectTouch(uint16_t DeviceAddr); 00407 00408 /** 00409 * @brief Get the touch screen X and Y positions values 00410 * Manage multi touch thanks to touch Index global 00411 * variable 'ft5336_handle.currActiveTouchIdx'. 00412 * @param DeviceAddr: Device address on communication Bus. 00413 * @param X: Pointer to X position value 00414 * @param Y: Pointer to Y position value 00415 * @retval None. 00416 */ 00417 void ft5336_TS_GetXY(uint16_t DeviceAddr, uint16_t *X, uint16_t *Y); 00418 00419 /** 00420 * @brief Configure the FT5336 device to generate IT on given INT pin 00421 * connected to MCU as EXTI. 00422 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT5336). 00423 * @retval None 00424 */ 00425 void ft5336_TS_EnableIT(uint16_t DeviceAddr); 00426 00427 /** 00428 * @brief Configure the FT5336 device to stop generating IT on the given INT pin 00429 * connected to MCU as EXTI. 00430 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT5336). 00431 * @retval None 00432 */ 00433 void ft5336_TS_DisableIT(uint16_t DeviceAddr); 00434 00435 /** 00436 * @brief Get IT status from FT5336 interrupt status registers 00437 * Should be called Following an EXTI coming to the MCU to know the detailed 00438 * reason of the interrupt. 00439 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00440 * @retval TS interrupts status 00441 */ 00442 uint8_t ft5336_TS_ITStatus (uint16_t DeviceAddr); 00443 00444 /** 00445 * @brief Clear IT status in FT5336 interrupt status clear registers 00446 * Should be called Following an EXTI coming to the MCU. 00447 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00448 * @retval TS interrupts status 00449 */ 00450 void ft5336_TS_ClearIT (uint16_t DeviceAddr); 00451 00452 /**** NEW FEATURES enabled when Multi-touch support is enabled ****/ 00453 00454 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00455 00456 /** 00457 * @brief Get the last touch gesture identification (zoom, move up/down...). 00458 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00459 * @param pGestureId : Pointer to get last touch gesture Identification. 00460 * @retval None. 00461 */ 00462 void ft5336_TS_GetGestureID(uint16_t DeviceAddr, uint32_t * pGestureId); 00463 00464 /** 00465 * @brief Get the touch detailed informations on touch number 'touchIdx' (0..1) 00466 * This touch detailed information contains : 00467 * - weight that was applied to this touch 00468 * - sub-area of the touch in the touch panel 00469 * - event of linked to the touch (press down, lift up, ...) 00470 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT5336). 00471 * @param touchIdx : Passed index of the touch (0..1) on which we want to get the 00472 * detailed information. 00473 * @param pWeight : Pointer to to get the weight information of 'touchIdx'. 00474 * @param pArea : Pointer to to get the sub-area information of 'touchIdx'. 00475 * @param pEvent : Pointer to to get the event information of 'touchIdx'. 00476 00477 * @retval None. 00478 */ 00479 void ft5336_TS_GetTouchInfo(uint16_t DeviceAddr, 00480 uint32_t touchIdx, 00481 uint32_t * pWeight, 00482 uint32_t * pArea, 00483 uint32_t * pEvent); 00484 00485 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00486 00487 /* Imported TS IO functions --------------------------------------------------------*/ 00488 00489 /** @defgroup ft5336_Imported_Functions 00490 * @{ 00491 */ 00492 00493 /* TouchScreen (TS) external IO functions */ 00494 extern void TS_IO_Init(void); 00495 extern void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00496 extern uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00497 extern void TS_IO_Delay(uint32_t Delay); 00498 00499 /** 00500 * @} 00501 */ 00502 00503 /* Imported global variables --------------------------------------------------------*/ 00504 00505 /** @defgroup ft5336_Imported_Globals 00506 * @{ 00507 */ 00508 00509 00510 /* Touch screen driver structure */ 00511 extern TS_DrvTypeDef ft5336_ts_drv; 00512 00513 /** 00514 * @} 00515 */ 00516 00517 #ifdef __cplusplus 00518 } 00519 #endif 00520 #endif /* __FT5336_H */ 00521 00522 00523 /** 00524 * @} 00525 */ 00526 00527 /** 00528 * @} 00529 */ 00530 00531 /** 00532 * @} 00533 */ 00534 00535 /** 00536 * @} 00537 */ 00538 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:20:42 by
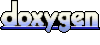