Contains the BSP driver for the DISCO_F413ZH board.
Dependents: DISCO_F413ZH-LCD-demo DISCO_F413ZH-touch-screen-demo DISCO_F413ZH-SD-demo DISCO_F413ZH-PSRAM-demo ... more
ft6x06.h
00001 /** 00002 ****************************************************************************** 00003 * @file ft6x06.h 00004 * @author MCD Application Team 00005 * @version V1.0.1 00006 * @date 03-May-2016 00007 * @brief This file contains all the functions prototypes for the 00008 * ft6x06.c IO expander driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __FT6X06_H 00041 #define __FT6X06_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Set Multi-touch as non supported */ 00048 #ifndef TS_MULTI_TOUCH_SUPPORTED 00049 #define TS_MULTI_TOUCH_SUPPORTED 0 00050 #endif 00051 00052 /* Set Auto-calibration as non supported */ 00053 #ifndef TS_AUTO_CALIBRATION_SUPPORTED 00054 #define TS_AUTO_CALIBRATION_SUPPORTED 0 00055 #endif 00056 00057 /* Includes ------------------------------------------------------------------*/ 00058 #include "../Common/ts.h" 00059 00060 /* Macros --------------------------------------------------------------------*/ 00061 00062 /** @typedef ft6x06_handle_TypeDef 00063 * ft6x06 Handle definition. 00064 */ 00065 typedef struct 00066 { 00067 uint8_t i2cInitialized; 00068 00069 /* field holding the current number of simultaneous active touches */ 00070 uint8_t currActiveTouchNb; 00071 00072 /* field holding the touch index currently managed */ 00073 uint8_t currActiveTouchIdx; 00074 00075 } ft6x06_handle_TypeDef; 00076 00077 /** @addtogroup BSP 00078 * @{ 00079 */ 00080 00081 /** @addtogroup Component 00082 * @{ 00083 */ 00084 00085 /** @defgroup FT6X06 00086 * @{ 00087 */ 00088 00089 /* Exported types ------------------------------------------------------------*/ 00090 00091 /** @defgroup FT6X06_Exported_Types 00092 * @{ 00093 */ 00094 00095 /* Exported constants --------------------------------------------------------*/ 00096 00097 /** @defgroup FT6X06_Exported_Constants 00098 * @{ 00099 */ 00100 00101 /* Maximum border values of the touchscreen pad */ 00102 #define FT_6206_MAX_WIDTH ((uint16_t)800) /* Touchscreen pad max width */ 00103 #define FT_6206_MAX_HEIGHT ((uint16_t)480) /* Touchscreen pad max height */ 00104 00105 /* Touchscreen pad max width and height values for FT6x36 Touch*/ 00106 #define FT_6206_MAX_WIDTH_HEIGHT ((uint16_t)240) 00107 00108 /* Possible values of driver functions return status */ 00109 #define FT6206_STATUS_OK 0 00110 #define FT6206_STATUS_NOT_OK 1 00111 00112 /* Possible values of global variable 'TS_I2C_Initialized' */ 00113 #define FT6206_I2C_NOT_INITIALIZED 0 00114 #define FT6206_I2C_INITIALIZED 1 00115 00116 /* Max detectable simultaneous touches */ 00117 #define FT6206_MAX_DETECTABLE_TOUCH 2 00118 00119 /** 00120 * @brief : Definitions for FT6206 I2C register addresses on 8 bit 00121 **/ 00122 00123 /* Current mode register of the FT6206 (R/W) */ 00124 #define FT6206_DEV_MODE_REG 0x00 00125 00126 /* Possible values of FT6206_DEV_MODE_REG */ 00127 #define FT6206_DEV_MODE_WORKING 0x00 00128 #define FT6206_DEV_MODE_FACTORY 0x04 00129 00130 #define FT6206_DEV_MODE_MASK 0x7 00131 #define FT6206_DEV_MODE_SHIFT 4 00132 00133 /* Gesture ID register */ 00134 #define FT6206_GEST_ID_REG 0x01 00135 00136 /* Possible values of FT6206_GEST_ID_REG */ 00137 #define FT6206_GEST_ID_NO_GESTURE 0x00 00138 #define FT6206_GEST_ID_MOVE_UP 0x10 00139 #define FT6206_GEST_ID_MOVE_RIGHT 0x14 00140 #define FT6206_GEST_ID_MOVE_DOWN 0x18 00141 #define FT6206_GEST_ID_MOVE_LEFT 0x1C 00142 #define FT6206_GEST_ID_ZOOM_IN 0x48 00143 #define FT6206_GEST_ID_ZOOM_OUT 0x49 00144 00145 /* Touch Data Status register : gives number of active touch points (0..2) */ 00146 #define FT6206_TD_STAT_REG 0x02 00147 00148 /* Values related to FT6206_TD_STAT_REG */ 00149 #define FT6206_TD_STAT_MASK 0x0F 00150 #define FT6206_TD_STAT_SHIFT 0x00 00151 00152 /* Values Pn_XH and Pn_YH related */ 00153 #define FT6206_TOUCH_EVT_FLAG_PRESS_DOWN 0x00 00154 #define FT6206_TOUCH_EVT_FLAG_LIFT_UP 0x01 00155 #define FT6206_TOUCH_EVT_FLAG_CONTACT 0x02 00156 #define FT6206_TOUCH_EVT_FLAG_NO_EVENT 0x03 00157 00158 #define FT6206_TOUCH_EVT_FLAG_SHIFT 6 00159 #define FT6206_TOUCH_EVT_FLAG_MASK (3 << FT6206_TOUCH_EVT_FLAG_SHIFT) 00160 00161 #define FT6206_MSB_MASK 0x0F 00162 #define FT6206_MSB_SHIFT 0 00163 00164 /* Values Pn_XL and Pn_YL related */ 00165 #define FT6206_LSB_MASK 0xFF 00166 #define FT6206_LSB_SHIFT 0 00167 00168 #define FT6206_P1_XH_REG 0x03 00169 #define FT6206_P1_XL_REG 0x04 00170 #define FT6206_P1_YH_REG 0x05 00171 #define FT6206_P1_YL_REG 0x06 00172 00173 /* Touch Pressure register value (R) */ 00174 #define FT6206_P1_WEIGHT_REG 0x07 00175 00176 /* Values Pn_WEIGHT related */ 00177 #define FT6206_TOUCH_WEIGHT_MASK 0xFF 00178 #define FT6206_TOUCH_WEIGHT_SHIFT 0 00179 00180 /* Touch area register */ 00181 #define FT6206_P1_MISC_REG 0x08 00182 00183 /* Values related to FT6206_Pn_MISC_REG */ 00184 #define FT6206_TOUCH_AREA_MASK (0x04 << 4) 00185 #define FT6206_TOUCH_AREA_SHIFT 0x04 00186 00187 #define FT6206_P2_XH_REG 0x09 00188 #define FT6206_P2_XL_REG 0x0A 00189 #define FT6206_P2_YH_REG 0x0B 00190 #define FT6206_P2_YL_REG 0x0C 00191 #define FT6206_P2_WEIGHT_REG 0x0D 00192 #define FT6206_P2_MISC_REG 0x0E 00193 00194 /* Threshold for touch detection */ 00195 #define FT6206_TH_GROUP_REG 0x80 00196 00197 /* Values FT6206_TH_GROUP_REG : threshold related */ 00198 #define FT6206_THRESHOLD_MASK 0xFF 00199 #define FT6206_THRESHOLD_SHIFT 0 00200 00201 /* Filter function coefficients */ 00202 #define FT6206_TH_DIFF_REG 0x85 00203 00204 /* Control register */ 00205 #define FT6206_CTRL_REG 0x86 00206 00207 /* Values related to FT6206_CTRL_REG */ 00208 00209 /* Will keep the Active mode when there is no touching */ 00210 #define FT6206_CTRL_KEEP_ACTIVE_MODE 0x00 00211 00212 /* Switching from Active mode to Monitor mode automatically when there is no touching */ 00213 #define FT6206_CTRL_KEEP_AUTO_SWITCH_MONITOR_MODE 0x01 00214 00215 /* The time period of switching from Active mode to Monitor mode when there is no touching */ 00216 #define FT6206_TIMEENTERMONITOR_REG 0x87 00217 00218 /* Report rate in Active mode */ 00219 #define FT6206_PERIODACTIVE_REG 0x88 00220 00221 /* Report rate in Monitor mode */ 00222 #define FT6206_PERIODMONITOR_REG 0x89 00223 00224 /* The value of the minimum allowed angle while Rotating gesture mode */ 00225 #define FT6206_RADIAN_VALUE_REG 0x91 00226 00227 /* Maximum offset while Moving Left and Moving Right gesture */ 00228 #define FT6206_OFFSET_LEFT_RIGHT_REG 0x92 00229 00230 /* Maximum offset while Moving Up and Moving Down gesture */ 00231 #define FT6206_OFFSET_UP_DOWN_REG 0x93 00232 00233 /* Minimum distance while Moving Left and Moving Right gesture */ 00234 #define FT6206_DISTANCE_LEFT_RIGHT_REG 0x94 00235 00236 /* Minimum distance while Moving Up and Moving Down gesture */ 00237 #define FT6206_DISTANCE_UP_DOWN_REG 0x95 00238 00239 /* Maximum distance while Zoom In and Zoom Out gesture */ 00240 #define FT6206_DISTANCE_ZOOM_REG 0x96 00241 00242 /* High 8-bit of LIB Version info */ 00243 #define FT6206_LIB_VER_H_REG 0xA1 00244 00245 /* Low 8-bit of LIB Version info */ 00246 #define FT6206_LIB_VER_L_REG 0xA2 00247 00248 /* Chip Selecting */ 00249 #define FT6206_CIPHER_REG 0xA3 00250 00251 /* Interrupt mode register (used when in interrupt mode) */ 00252 #define FT6206_GMODE_REG 0xA4 00253 00254 #define FT6206_G_MODE_INTERRUPT_MASK 0x03 00255 #define FT6206_G_MODE_INTERRUPT_SHIFT 0x00 00256 00257 /* Possible values of FT6206_GMODE_REG */ 00258 #define FT6206_G_MODE_INTERRUPT_POLLING 0x00 00259 #define FT6206_G_MODE_INTERRUPT_TRIGGER 0x01 00260 00261 /* Current power mode the FT6206 system is in (R) */ 00262 #define FT6206_PWR_MODE_REG 0xA5 00263 00264 /* FT6206 firmware version */ 00265 #define FT6206_FIRMID_REG 0xA6 00266 00267 /* FT6206 Chip identification register */ 00268 #define FT6206_CHIP_ID_REG 0xA8 00269 00270 /* Possible values of FT6206_CHIP_ID_REG */ 00271 #define FT6206_ID_VALUE 0x11 00272 #define FT6x36_ID_VALUE 0xCD 00273 00274 /* Release code version */ 00275 #define FT6206_RELEASE_CODE_ID_REG 0xAF 00276 00277 /* Current operating mode the FT6206 system is in (R) */ 00278 #define FT6206_STATE_REG 0xBC 00279 00280 /** 00281 * @} 00282 */ 00283 00284 /* Exported macro ------------------------------------------------------------*/ 00285 00286 /** @defgroup ft6x06_Exported_Macros 00287 * @{ 00288 */ 00289 00290 /* Exported functions --------------------------------------------------------*/ 00291 00292 /** @defgroup ft6x06_Exported_Functions 00293 * @{ 00294 */ 00295 00296 /** 00297 * @brief ft6x06 Control functions 00298 */ 00299 00300 00301 /** 00302 * @brief Initialize the ft6x06 communication bus 00303 * from MCU to FT6206 : ie I2C channel initialization (if required). 00304 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00305 * @retval None 00306 */ 00307 void ft6x06_Init(uint16_t DeviceAddr); 00308 00309 /** 00310 * @brief Software Reset the ft6x06. 00311 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00312 * @retval None 00313 */ 00314 void ft6x06_Reset(uint16_t DeviceAddr); 00315 00316 /** 00317 * @brief Read the ft6x06 device ID, pre intitalize I2C in case of need to be 00318 * able to read the FT6206 device ID, and verify this is a FT6206. 00319 * @param DeviceAddr: I2C FT6x06 Slave address. 00320 * @retval The Device ID (two bytes). 00321 */ 00322 uint16_t ft6x06_ReadID(uint16_t DeviceAddr); 00323 00324 /** 00325 * @brief Configures the touch Screen IC device to start detecting touches 00326 * @param DeviceAddr: Device address on communication Bus (I2C slave address). 00327 * @retval None. 00328 */ 00329 void ft6x06_TS_Start(uint16_t DeviceAddr); 00330 00331 /** 00332 * @brief Return if there is touches detected or not. 00333 * Try to detect new touches and forget the old ones (reset internal global 00334 * variables). 00335 * @param DeviceAddr: Device address on communication Bus. 00336 * @retval : Number of active touches detected (can be 0, 1 or 2). 00337 */ 00338 uint8_t ft6x06_TS_DetectTouch(uint16_t DeviceAddr); 00339 00340 /** 00341 * @brief Get the touch screen X and Y positions values 00342 * Manage multi touch thanks to touch Index global 00343 * variable 'ft6x06_handle.currActiveTouchIdx'. 00344 * @param DeviceAddr: Device address on communication Bus. 00345 * @param X: Pointer to X position value 00346 * @param Y: Pointer to Y position value 00347 * @retval None. 00348 */ 00349 void ft6x06_TS_GetXY(uint16_t DeviceAddr, uint16_t *X, uint16_t *Y); 00350 00351 /** 00352 * @brief Configure the FT6206 device to generate IT on given INT pin 00353 * connected to MCU as EXTI. 00354 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT6206). 00355 * @retval None 00356 */ 00357 void ft6x06_TS_EnableIT(uint16_t DeviceAddr); 00358 00359 /** 00360 * @brief Configure the FT6206 device to stop generating IT on the given INT pin 00361 * connected to MCU as EXTI. 00362 * @param DeviceAddr: Device address on communication Bus (Slave I2C address of FT6206). 00363 * @retval None 00364 */ 00365 void ft6x06_TS_DisableIT(uint16_t DeviceAddr); 00366 00367 /** 00368 * @brief Get IT status from FT6206 interrupt status registers 00369 * Should be called Following an EXTI coming to the MCU to know the detailed 00370 * reason of the interrupt. 00371 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00372 * @retval TS interrupts status 00373 */ 00374 uint8_t ft6x06_TS_ITStatus (uint16_t DeviceAddr); 00375 00376 /** 00377 * @brief Clear IT status in FT6206 interrupt status clear registers 00378 * Should be called Following an EXTI coming to the MCU. 00379 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6206). 00380 * @retval TS interrupts status 00381 */ 00382 void ft6x06_TS_ClearIT (uint16_t DeviceAddr); 00383 00384 /**** NEW FEATURES enabled when Multi-touch support is enabled ****/ 00385 00386 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00387 00388 /** 00389 * @brief Get the last touch gesture identification (zoom, move up/down...). 00390 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6x06). 00391 * @param pGestureId : Pointer to get last touch gesture Identification. 00392 * @retval None. 00393 */ 00394 void ft6x06_TS_GetGestureID(uint16_t DeviceAddr, uint32_t * pGestureId); 00395 00396 /** 00397 * @brief Get the touch detailed informations on touch number 'touchIdx' (0..1) 00398 * This touch detailed information contains : 00399 * - weight that was applied to this touch 00400 * - sub-area of the touch in the touch panel 00401 * - event of linked to the touch (press down, lift up, ...) 00402 * @param DeviceAddr: Device address on communication Bus (I2C slave address of FT6x06). 00403 * @param touchIdx : Passed index of the touch (0..1) on which we want to get the 00404 * detailed information. 00405 * @param pWeight : Pointer to to get the weight information of 'touchIdx'. 00406 * @param pArea : Pointer to to get the sub-area information of 'touchIdx'. 00407 * @param pEvent : Pointer to to get the event information of 'touchIdx'. 00408 00409 * @retval None. 00410 */ 00411 void ft6x06_TS_GetTouchInfo(uint16_t DeviceAddr, 00412 uint32_t touchIdx, 00413 uint32_t * pWeight, 00414 uint32_t * pArea, 00415 uint32_t * pEvent); 00416 00417 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00418 00419 /* Imported TS IO functions --------------------------------------------------------*/ 00420 00421 /** @defgroup ft6x06_Imported_Functions 00422 * @{ 00423 */ 00424 00425 /* TouchScreen (TS) external IO functions */ 00426 extern void TS_IO_Init(void); 00427 extern void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00428 extern uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00429 extern uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00430 extern void TS_IO_Delay(uint32_t Delay); 00431 00432 /** 00433 * @} 00434 */ 00435 00436 /* Imported global variables --------------------------------------------------------*/ 00437 00438 /** @defgroup ft6x06_Imported_Globals 00439 * @{ 00440 */ 00441 00442 00443 /* Touch screen driver structure */ 00444 extern TS_DrvTypeDef ft6x06_ts_drv; 00445 00446 /** 00447 * @} 00448 */ 00449 00450 #ifdef __cplusplus 00451 } 00452 #endif 00453 #endif /* __FT6X06_H */ 00454 00455 00456 /** 00457 * @} 00458 */ 00459 00460 /** 00461 * @} 00462 */ 00463 00464 /** 00465 * @} 00466 */ 00467 00468 /** 00469 * @} 00470 */ 00471 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:54:37 by
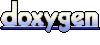