Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
stm32l475e_iot01_qspi.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01_qspi.c 00004 * @author MCD Application Team 00005 * @brief This file includes a standard driver for the MX25R6435F QSPI 00006 * memory mounted on STM32L475E IOT01 board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the MX25R6435F QSPI external 00013 memory mounted on STM32L475E IOT01 board. 00014 00015 (#) This driver need a specific component driver (MX25R6435F) to be included with. 00016 00017 (#) Initialization steps: 00018 (++) Initialize the QPSI external memory using the BSP_QSPI_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 QSPI interface with the external memory. The BSP_QSPI_DeInit() can be used 00021 to deactivate the QSPI interface. 00022 00023 (#) QSPI memory operations 00024 (++) QSPI memory can be accessed with read/write operations once it is 00025 initialized. 00026 Read/write operation can be performed with AHB access using the functions 00027 BSP_QSPI_Read()/BSP_QSPI_Write(). 00028 (++) The function to the QSPI memory in memory-mapped mode is possible after 00029 the call of the function BSP_QSPI_EnableMemoryMappedMode(). 00030 (++) The function BSP_QSPI_GetInfo() returns the configuration of the QSPI memory. 00031 (see the QSPI memory data sheet) 00032 (++) Perform erase block operation using the function BSP_QSPI_Erase_Block() and by 00033 specifying the block address. You can perform an erase operation of the whole 00034 chip by calling the function BSP_QSPI_Erase_Chip(). 00035 (++) The function BSP_QSPI_GetStatus() returns the current status of the QSPI memory. 00036 (see the QSPI memory data sheet) 00037 (++) Perform erase sector operation using the function BSP_QSPI_Erase_Sector() 00038 which is not blocking. So the function BSP_QSPI_GetStatus() should be used 00039 to check if the memory is busy, and the functions BSP_QSPI_SuspendErase()/ 00040 BSP_QSPI_ResumeErase() can be used to perform other operations during the 00041 sector erase. 00042 (++) Deep power down of the QSPI memory is managed with the call of the functions 00043 BSP_QSPI_EnterDeepPowerDown()/BSP_QSPI_LeaveDeepPowerDown() 00044 @endverbatim 00045 ****************************************************************************** 00046 * @attention 00047 * 00048 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00049 * All rights reserved.</center></h2> 00050 * 00051 * This software component is licensed by ST under BSD 3-Clause license, 00052 * the "License"; You may not use this file except in compliance with the 00053 * License. You may obtain a copy of the License at: 00054 * opensource.org/licenses/BSD-3-Clause 00055 * 00056 ****************************************************************************** 00057 */ 00058 00059 /* Includes ------------------------------------------------------------------*/ 00060 #include "stm32l475e_iot01_qspi.h" 00061 00062 /** @addtogroup BSP 00063 * @{ 00064 */ 00065 00066 /** @addtogroup STM32L475E_IOT01 00067 * @{ 00068 */ 00069 00070 /** @defgroup STM32L475E_IOT01_QSPI QSPI 00071 * @{ 00072 */ 00073 00074 /* Private constants --------------------------------------------------------*/ 00075 /** @defgroup STM32L475E_IOT01_QSPI_Private_Constants QSPI Private Constants 00076 * @{ 00077 */ 00078 #define QSPI_QUAD_DISABLE 0x0 00079 #define QSPI_QUAD_ENABLE 0x1 00080 00081 #define QSPI_HIGH_PERF_DISABLE 0x0 00082 #define QSPI_HIGH_PERF_ENABLE 0x1 00083 /** 00084 * @} 00085 */ 00086 /* Private variables ---------------------------------------------------------*/ 00087 00088 /** @defgroup STM32L475E_IOT01_QSPI_Private_Variables QSPI Private Variables 00089 * @{ 00090 */ 00091 QSPI_HandleTypeDef QSPIHandle; 00092 00093 /** 00094 * @} 00095 */ 00096 00097 00098 /* Private functions ---------------------------------------------------------*/ 00099 00100 /** @defgroup STM32L475E_IOT01_QSPI_Private_Functions QSPI Private Functions 00101 * @{ 00102 */ 00103 static uint8_t QSPI_ResetMemory (QSPI_HandleTypeDef *hqspi); 00104 static uint8_t QSPI_WriteEnable (QSPI_HandleTypeDef *hqspi); 00105 static uint8_t QSPI_AutoPollingMemReady(QSPI_HandleTypeDef *hqspi, uint32_t Timeout); 00106 static uint8_t QSPI_QuadMode (QSPI_HandleTypeDef *hqspi, uint8_t Operation); 00107 static uint8_t QSPI_HighPerfMode (QSPI_HandleTypeDef *hqspi, uint8_t Operation); 00108 00109 /** 00110 * @} 00111 */ 00112 00113 /* Exported functions ---------------------------------------------------------*/ 00114 00115 /** @addtogroup STM32L475E_IOT01_QSPI_Exported_Functions 00116 * @{ 00117 */ 00118 00119 /** 00120 * @brief Initializes the QSPI interface. 00121 * @retval QSPI memory status 00122 */ 00123 uint8_t BSP_QSPI_Init(void) 00124 { 00125 QSPIHandle.Instance = QUADSPI; 00126 00127 /* Call the DeInit function to reset the driver */ 00128 if (HAL_QSPI_DeInit(&QSPIHandle) != HAL_OK) 00129 { 00130 return QSPI_ERROR; 00131 } 00132 00133 /* System level initialization */ 00134 BSP_QSPI_MspInit(); 00135 00136 /* QSPI initialization */ 00137 QSPIHandle.Init.ClockPrescaler = 2; /* QSPI clock = 80MHz / (ClockPrescaler+1) = 26.67MHz */ 00138 QSPIHandle.Init.FifoThreshold = 4; 00139 QSPIHandle.Init.SampleShifting = QSPI_SAMPLE_SHIFTING_NONE; 00140 QSPIHandle.Init.FlashSize = POSITION_VAL(MX25R6435F_FLASH_SIZE) - 1; 00141 QSPIHandle.Init.ChipSelectHighTime = QSPI_CS_HIGH_TIME_1_CYCLE; 00142 QSPIHandle.Init.ClockMode = QSPI_CLOCK_MODE_0; 00143 00144 if (HAL_QSPI_Init(&QSPIHandle) != HAL_OK) 00145 { 00146 return QSPI_ERROR; 00147 } 00148 00149 /* QSPI memory reset */ 00150 if (QSPI_ResetMemory(&QSPIHandle) != QSPI_OK) 00151 { 00152 return QSPI_NOT_SUPPORTED; 00153 } 00154 00155 /* QSPI quad enable */ 00156 if (QSPI_QuadMode(&QSPIHandle, QSPI_QUAD_ENABLE) != QSPI_OK) 00157 { 00158 return QSPI_ERROR; 00159 } 00160 00161 /* High performance mode enable */ 00162 if (QSPI_HighPerfMode(&QSPIHandle, QSPI_HIGH_PERF_ENABLE) != QSPI_OK) 00163 { 00164 return QSPI_ERROR; 00165 } 00166 00167 /* Re-configure the clock for the high performance mode */ 00168 QSPIHandle.Init.ClockPrescaler = 1; /* QSPI clock = 80MHz / (ClockPrescaler+1) = 40MHz */ 00169 00170 if (HAL_QSPI_Init(&QSPIHandle) != HAL_OK) 00171 { 00172 return QSPI_ERROR; 00173 } 00174 00175 return QSPI_OK; 00176 } 00177 00178 /** 00179 * @brief De-Initializes the QSPI interface. 00180 * @retval QSPI memory status 00181 */ 00182 uint8_t BSP_QSPI_DeInit(void) 00183 { 00184 QSPIHandle.Instance = QUADSPI; 00185 00186 /* Call the DeInit function to reset the driver */ 00187 if (HAL_QSPI_DeInit(&QSPIHandle) != HAL_OK) 00188 { 00189 return QSPI_ERROR; 00190 } 00191 00192 /* System level De-initialization */ 00193 BSP_QSPI_MspDeInit(); 00194 00195 return QSPI_OK; 00196 } 00197 00198 /** 00199 * @brief Reads an amount of data from the QSPI memory. 00200 * @param pData : Pointer to data to be read 00201 * @param ReadAddr : Read start address 00202 * @param Size : Size of data to read 00203 * @retval QSPI memory status 00204 */ 00205 uint8_t BSP_QSPI_Read(uint8_t* pData, uint32_t ReadAddr, uint32_t Size) 00206 { 00207 QSPI_CommandTypeDef sCommand; 00208 00209 /* Initialize the read command */ 00210 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00211 sCommand.Instruction = QUAD_INOUT_READ_CMD; 00212 sCommand.AddressMode = QSPI_ADDRESS_4_LINES; 00213 sCommand.AddressSize = QSPI_ADDRESS_24_BITS; 00214 sCommand.Address = ReadAddr; 00215 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_4_LINES; 00216 sCommand.AlternateBytesSize = QSPI_ALTERNATE_BYTES_8_BITS; 00217 sCommand.AlternateBytes = MX25R6435F_ALT_BYTES_NO_PE_MODE; 00218 sCommand.DataMode = QSPI_DATA_4_LINES; 00219 sCommand.DummyCycles = MX25R6435F_DUMMY_CYCLES_READ_QUAD; 00220 sCommand.NbData = Size; 00221 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00222 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00223 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00224 00225 /* Configure the command */ 00226 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00227 { 00228 return QSPI_ERROR; 00229 } 00230 00231 /* Reception of the data */ 00232 if (HAL_QSPI_Receive(&QSPIHandle, pData, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00233 { 00234 return QSPI_ERROR; 00235 } 00236 00237 return QSPI_OK; 00238 } 00239 00240 /** 00241 * @brief Writes an amount of data to the QSPI memory. 00242 * @param pData : Pointer to data to be written 00243 * @param WriteAddr : Write start address 00244 * @param Size : Size of data to write 00245 * @retval QSPI memory status 00246 */ 00247 uint8_t BSP_QSPI_Write(uint8_t* pData, uint32_t WriteAddr, uint32_t Size) 00248 { 00249 QSPI_CommandTypeDef sCommand; 00250 uint32_t end_addr, current_size, current_addr; 00251 00252 /* Calculation of the size between the write address and the end of the page */ 00253 current_size = MX25R6435F_PAGE_SIZE - (WriteAddr % MX25R6435F_PAGE_SIZE); 00254 00255 /* Check if the size of the data is less than the remaining place in the page */ 00256 if (current_size > Size) 00257 { 00258 current_size = Size; 00259 } 00260 00261 /* Initialize the adress variables */ 00262 current_addr = WriteAddr; 00263 end_addr = WriteAddr + Size; 00264 00265 /* Initialize the program command */ 00266 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00267 sCommand.Instruction = QUAD_PAGE_PROG_CMD; 00268 sCommand.AddressMode = QSPI_ADDRESS_4_LINES; 00269 sCommand.AddressSize = QSPI_ADDRESS_24_BITS; 00270 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00271 sCommand.DataMode = QSPI_DATA_4_LINES; 00272 sCommand.DummyCycles = 0; 00273 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00274 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00275 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00276 00277 /* Perform the write page by page */ 00278 do 00279 { 00280 sCommand.Address = current_addr; 00281 sCommand.NbData = current_size; 00282 00283 /* Enable write operations */ 00284 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 00285 { 00286 return QSPI_ERROR; 00287 } 00288 00289 /* Configure the command */ 00290 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00291 { 00292 return QSPI_ERROR; 00293 } 00294 00295 /* Transmission of the data */ 00296 if (HAL_QSPI_Transmit(&QSPIHandle, pData, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00297 { 00298 return QSPI_ERROR; 00299 } 00300 00301 /* Configure automatic polling mode to wait for end of program */ 00302 if (QSPI_AutoPollingMemReady(&QSPIHandle, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != QSPI_OK) 00303 { 00304 return QSPI_ERROR; 00305 } 00306 00307 /* Update the address and size variables for next page programming */ 00308 current_addr += current_size; 00309 pData += current_size; 00310 current_size = ((current_addr + MX25R6435F_PAGE_SIZE) > end_addr) ? (end_addr - current_addr) : MX25R6435F_PAGE_SIZE; 00311 } while (current_addr < end_addr); 00312 00313 return QSPI_OK; 00314 } 00315 00316 /** 00317 * @brief Erases the specified block of the QSPI memory. 00318 * @param BlockAddress : Block address to erase 00319 * @retval QSPI memory status 00320 */ 00321 uint8_t BSP_QSPI_Erase_Block(uint32_t BlockAddress) 00322 { 00323 QSPI_CommandTypeDef sCommand; 00324 00325 /* Initialize the erase command */ 00326 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00327 sCommand.Instruction = BLOCK_ERASE_CMD; 00328 sCommand.AddressMode = QSPI_ADDRESS_1_LINE; 00329 sCommand.AddressSize = QSPI_ADDRESS_24_BITS; 00330 sCommand.Address = BlockAddress; 00331 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00332 sCommand.DataMode = QSPI_DATA_NONE; 00333 sCommand.DummyCycles = 0; 00334 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00335 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00336 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00337 00338 /* Enable write operations */ 00339 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 00340 { 00341 return QSPI_ERROR; 00342 } 00343 00344 /* Send the command */ 00345 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00346 { 00347 return QSPI_ERROR; 00348 } 00349 00350 /* Configure automatic polling mode to wait for end of erase */ 00351 if (QSPI_AutoPollingMemReady(&QSPIHandle, MX25R6435F_BLOCK_ERASE_MAX_TIME) != QSPI_OK) 00352 { 00353 return QSPI_ERROR; 00354 } 00355 00356 return QSPI_OK; 00357 } 00358 00359 /** 00360 * @brief Erases the specified sector of the QSPI memory. 00361 * @param Sector : Sector address to erase (0 to 255) 00362 * @retval QSPI memory status 00363 * @note This function is non blocking meaning that sector erase 00364 * operation is started but not completed when the function 00365 * returns. Application has to call BSP_QSPI_GetStatus() 00366 * to know when the device is available again (i.e. erase operation 00367 * completed). 00368 */ 00369 uint8_t BSP_QSPI_Erase_Sector(uint32_t Sector) 00370 { 00371 QSPI_CommandTypeDef sCommand; 00372 00373 if (Sector >= (uint32_t)(MX25R6435F_FLASH_SIZE/MX25R6435F_SECTOR_SIZE)) 00374 { 00375 return QSPI_ERROR; 00376 } 00377 00378 /* Initialize the erase command */ 00379 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00380 sCommand.Instruction = SECTOR_ERASE_CMD; 00381 sCommand.AddressMode = QSPI_ADDRESS_1_LINE; 00382 sCommand.AddressSize = QSPI_ADDRESS_24_BITS; 00383 sCommand.Address = (Sector * MX25R6435F_SECTOR_SIZE); 00384 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00385 sCommand.DataMode = QSPI_DATA_NONE; 00386 sCommand.DummyCycles = 0; 00387 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00388 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00389 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00390 00391 /* Enable write operations */ 00392 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 00393 { 00394 return QSPI_ERROR; 00395 } 00396 00397 /* Send the command */ 00398 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00399 { 00400 return QSPI_ERROR; 00401 } 00402 00403 return QSPI_OK; 00404 } 00405 00406 /** 00407 * @brief Erases the entire QSPI memory. 00408 * @retval QSPI memory status 00409 */ 00410 uint8_t BSP_QSPI_Erase_Chip(void) 00411 { 00412 QSPI_CommandTypeDef sCommand; 00413 00414 /* Initialize the erase command */ 00415 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00416 sCommand.Instruction = CHIP_ERASE_CMD; 00417 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00418 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00419 sCommand.DataMode = QSPI_DATA_NONE; 00420 sCommand.DummyCycles = 0; 00421 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00422 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00423 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00424 00425 /* Enable write operations */ 00426 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 00427 { 00428 return QSPI_ERROR; 00429 } 00430 00431 /* Send the command */ 00432 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00433 { 00434 return QSPI_ERROR; 00435 } 00436 00437 /* Configure automatic polling mode to wait for end of erase */ 00438 if (QSPI_AutoPollingMemReady(&QSPIHandle, MX25R6435F_CHIP_ERASE_MAX_TIME) != QSPI_OK) 00439 { 00440 return QSPI_ERROR; 00441 } 00442 00443 return QSPI_OK; 00444 } 00445 00446 /** 00447 * @brief Reads current status of the QSPI memory. 00448 * @retval QSPI memory status 00449 */ 00450 uint8_t BSP_QSPI_GetStatus(void) 00451 { 00452 QSPI_CommandTypeDef sCommand; 00453 uint8_t reg; 00454 00455 /* Initialize the read security register command */ 00456 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00457 sCommand.Instruction = READ_SEC_REG_CMD; 00458 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00459 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00460 sCommand.DataMode = QSPI_DATA_1_LINE; 00461 sCommand.DummyCycles = 0; 00462 sCommand.NbData = 1; 00463 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00464 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00465 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00466 00467 /* Configure the command */ 00468 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00469 { 00470 return QSPI_ERROR; 00471 } 00472 00473 /* Reception of the data */ 00474 if (HAL_QSPI_Receive(&QSPIHandle, ®, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00475 { 00476 return QSPI_ERROR; 00477 } 00478 00479 /* Check the value of the register */ 00480 if ((reg & (MX25R6435F_SECR_P_FAIL | MX25R6435F_SECR_E_FAIL)) != 0) 00481 { 00482 return QSPI_ERROR; 00483 } 00484 else if ((reg & (MX25R6435F_SECR_PSB | MX25R6435F_SECR_ESB)) != 0) 00485 { 00486 return QSPI_SUSPENDED; 00487 } 00488 00489 /* Initialize the read status register command */ 00490 sCommand.Instruction = READ_STATUS_REG_CMD; 00491 00492 /* Configure the command */ 00493 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00494 { 00495 return QSPI_ERROR; 00496 } 00497 00498 /* Reception of the data */ 00499 if (HAL_QSPI_Receive(&QSPIHandle, ®, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00500 { 00501 return QSPI_ERROR; 00502 } 00503 00504 /* Check the value of the register */ 00505 if ((reg & MX25R6435F_SR_WIP) != 0) 00506 { 00507 return QSPI_BUSY; 00508 } 00509 else 00510 { 00511 return QSPI_OK; 00512 } 00513 } 00514 00515 /** 00516 * @brief Return the configuration of the QSPI memory. 00517 * @param pInfo : pointer on the configuration structure 00518 * @retval QSPI memory status 00519 */ 00520 uint8_t BSP_QSPI_GetInfo(QSPI_Info* pInfo) 00521 { 00522 /* Configure the structure with the memory configuration */ 00523 pInfo->FlashSize = MX25R6435F_FLASH_SIZE; 00524 pInfo->EraseSectorSize = MX25R6435F_SECTOR_SIZE; 00525 pInfo->EraseSectorsNumber = (MX25R6435F_FLASH_SIZE/MX25R6435F_SECTOR_SIZE); 00526 pInfo->ProgPageSize = MX25R6435F_PAGE_SIZE; 00527 pInfo->ProgPagesNumber = (MX25R6435F_FLASH_SIZE/MX25R6435F_PAGE_SIZE); 00528 00529 return QSPI_OK; 00530 } 00531 00532 /** 00533 * @brief Configure the QSPI in memory-mapped mode 00534 * @retval QSPI memory status 00535 */ 00536 uint8_t BSP_QSPI_EnableMemoryMappedMode(void) 00537 { 00538 QSPI_CommandTypeDef sCommand; 00539 QSPI_MemoryMappedTypeDef sMemMappedCfg; 00540 00541 /* Configure the command for the read instruction */ 00542 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00543 sCommand.Instruction = QUAD_INOUT_READ_CMD; 00544 sCommand.AddressMode = QSPI_ADDRESS_4_LINES; 00545 sCommand.AddressSize = QSPI_ADDRESS_24_BITS; 00546 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_4_LINES; 00547 sCommand.AlternateBytesSize = QSPI_ALTERNATE_BYTES_8_BITS; 00548 sCommand.AlternateBytes = MX25R6435F_ALT_BYTES_NO_PE_MODE; 00549 sCommand.DataMode = QSPI_DATA_4_LINES; 00550 sCommand.DummyCycles = MX25R6435F_DUMMY_CYCLES_READ_QUAD; 00551 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00552 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00553 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00554 00555 /* Configure the memory mapped mode */ 00556 sMemMappedCfg.TimeOutActivation = QSPI_TIMEOUT_COUNTER_DISABLE; 00557 00558 if (HAL_QSPI_MemoryMapped(&QSPIHandle, &sCommand, &sMemMappedCfg) != HAL_OK) 00559 { 00560 return QSPI_ERROR; 00561 } 00562 00563 return QSPI_OK; 00564 } 00565 00566 /** 00567 * @brief This function suspends an ongoing erase command. 00568 * @retval QSPI memory status 00569 */ 00570 uint8_t BSP_QSPI_SuspendErase(void) 00571 { 00572 QSPI_CommandTypeDef sCommand; 00573 00574 /* Check whether the device is busy (erase operation is 00575 in progress). 00576 */ 00577 if (BSP_QSPI_GetStatus() == QSPI_BUSY) 00578 { 00579 /* Initialize the erase command */ 00580 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00581 sCommand.Instruction = PROG_ERASE_SUSPEND_CMD; 00582 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00583 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00584 sCommand.DataMode = QSPI_DATA_NONE; 00585 sCommand.DummyCycles = 0; 00586 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00587 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00588 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00589 00590 /* Send the command */ 00591 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00592 { 00593 return QSPI_ERROR; 00594 } 00595 00596 if (BSP_QSPI_GetStatus() == QSPI_SUSPENDED) 00597 { 00598 return QSPI_OK; 00599 } 00600 00601 return QSPI_ERROR; 00602 } 00603 00604 return QSPI_OK; 00605 } 00606 00607 /** 00608 * @brief This function resumes a paused erase command. 00609 * @retval QSPI memory status 00610 */ 00611 uint8_t BSP_QSPI_ResumeErase(void) 00612 { 00613 QSPI_CommandTypeDef sCommand; 00614 00615 /* Check whether the device is in suspended state */ 00616 if (BSP_QSPI_GetStatus() == QSPI_SUSPENDED) 00617 { 00618 /* Initialize the erase command */ 00619 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00620 sCommand.Instruction = PROG_ERASE_RESUME_CMD; 00621 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00622 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00623 sCommand.DataMode = QSPI_DATA_NONE; 00624 sCommand.DummyCycles = 0; 00625 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00626 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00627 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00628 00629 /* Send the command */ 00630 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00631 { 00632 return QSPI_ERROR; 00633 } 00634 00635 /* 00636 When this command is executed, the status register write in progress bit is set to 1, and 00637 the flag status register program erase controller bit is set to 0. This command is ignored 00638 if the device is not in a suspended state. 00639 */ 00640 00641 if (BSP_QSPI_GetStatus() == QSPI_BUSY) 00642 { 00643 return QSPI_OK; 00644 } 00645 00646 return QSPI_ERROR; 00647 } 00648 00649 return QSPI_OK; 00650 } 00651 00652 /** 00653 * @brief This function enter the QSPI memory in deep power down mode. 00654 * @retval QSPI memory status 00655 */ 00656 uint8_t BSP_QSPI_EnterDeepPowerDown(void) 00657 { 00658 QSPI_CommandTypeDef sCommand; 00659 00660 /* Initialize the deep power down command */ 00661 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00662 sCommand.Instruction = DEEP_POWER_DOWN_CMD; 00663 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00664 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00665 sCommand.DataMode = QSPI_DATA_NONE; 00666 sCommand.DummyCycles = 0; 00667 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00668 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00669 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00670 00671 /* Send the command */ 00672 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00673 { 00674 return QSPI_ERROR; 00675 } 00676 00677 /* --- Memory takes 10us max to enter deep power down --- */ 00678 /* --- At least 30us should be respected before leaving deep power down --- */ 00679 00680 return QSPI_OK; 00681 } 00682 00683 /** 00684 * @brief This function leave the QSPI memory from deep power down mode. 00685 * @retval QSPI memory status 00686 */ 00687 uint8_t BSP_QSPI_LeaveDeepPowerDown(void) 00688 { 00689 QSPI_CommandTypeDef sCommand; 00690 00691 /* Initialize the erase command */ 00692 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00693 sCommand.Instruction = NO_OPERATION_CMD; 00694 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00695 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00696 sCommand.DataMode = QSPI_DATA_NONE; 00697 sCommand.DummyCycles = 0; 00698 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00699 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00700 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00701 00702 /* Send the command */ 00703 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00704 { 00705 return QSPI_ERROR; 00706 } 00707 00708 /* --- A NOP command is sent to the memory, as the nCS should be low for at least 20 ns --- */ 00709 /* --- Memory takes 35us min to leave deep power down --- */ 00710 00711 return QSPI_OK; 00712 } 00713 00714 /** 00715 * @brief Initializes the QSPI MSP. 00716 * @retval None 00717 */ 00718 __weak void BSP_QSPI_MspInit(void) 00719 { 00720 GPIO_InitTypeDef GPIO_InitStruct; 00721 00722 /* Enable the QuadSPI memory interface clock */ 00723 __HAL_RCC_QSPI_CLK_ENABLE(); 00724 00725 /* Reset the QuadSPI memory interface */ 00726 __HAL_RCC_QSPI_FORCE_RESET(); 00727 __HAL_RCC_QSPI_RELEASE_RESET(); 00728 00729 /* Enable GPIO clocks */ 00730 __HAL_RCC_GPIOE_CLK_ENABLE(); 00731 00732 /* QSPI CLK, CS, D0, D1, D2 and D3 GPIO pins configuration */ 00733 GPIO_InitStruct.Pin = GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00734 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00735 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00736 GPIO_InitStruct.Pull = GPIO_NOPULL; 00737 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00738 GPIO_InitStruct.Alternate = GPIO_AF10_QUADSPI; 00739 HAL_GPIO_Init(GPIOE, &GPIO_InitStruct); 00740 } 00741 00742 /** 00743 * @brief De-Initializes the QSPI MSP. 00744 * @retval None 00745 */ 00746 __weak void BSP_QSPI_MspDeInit(void) 00747 { 00748 GPIO_InitTypeDef GPIO_InitStruct; 00749 00750 /* QSPI CLK, CS, D0-D3 GPIO pins de-configuration */ 00751 __HAL_RCC_GPIOE_CLK_ENABLE(); 00752 GPIO_InitStruct.Pin = GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00753 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00754 00755 HAL_GPIO_DeInit(GPIOE, GPIO_InitStruct.Pin); 00756 00757 /* Reset the QuadSPI memory interface */ 00758 __HAL_RCC_QSPI_FORCE_RESET(); 00759 __HAL_RCC_QSPI_RELEASE_RESET(); 00760 00761 /* Disable the QuadSPI memory interface clock */ 00762 __HAL_RCC_QSPI_CLK_DISABLE(); 00763 } 00764 00765 /** 00766 * @} 00767 */ 00768 00769 /** @addtogroup STM32L475E_IOT01_QSPI_Private_Functions 00770 * @{ 00771 */ 00772 00773 /** 00774 * @brief This function reset the QSPI memory. 00775 * @param hqspi : QSPI handle 00776 * @retval None 00777 */ 00778 static uint8_t QSPI_ResetMemory(QSPI_HandleTypeDef *hqspi) 00779 { 00780 QSPI_CommandTypeDef sCommand; 00781 00782 /* Initialize the reset enable command */ 00783 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00784 sCommand.Instruction = RESET_ENABLE_CMD; 00785 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00786 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00787 sCommand.DataMode = QSPI_DATA_NONE; 00788 sCommand.DummyCycles = 0; 00789 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00790 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00791 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00792 00793 /* Send the command */ 00794 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00795 { 00796 return QSPI_ERROR; 00797 } 00798 00799 /* Send the reset memory command */ 00800 sCommand.Instruction = RESET_MEMORY_CMD; 00801 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00802 { 00803 return QSPI_ERROR; 00804 } 00805 00806 /* Configure automatic polling mode to wait the memory is ready */ 00807 if (QSPI_AutoPollingMemReady(&QSPIHandle, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != QSPI_OK) 00808 { 00809 return QSPI_ERROR; 00810 } 00811 00812 return QSPI_OK; 00813 } 00814 00815 /** 00816 * @brief This function send a Write Enable and wait it is effective. 00817 * @param hqspi : QSPI handle 00818 * @retval None 00819 */ 00820 static uint8_t QSPI_WriteEnable(QSPI_HandleTypeDef *hqspi) 00821 { 00822 QSPI_CommandTypeDef sCommand; 00823 QSPI_AutoPollingTypeDef sConfig; 00824 00825 /* Enable write operations */ 00826 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00827 sCommand.Instruction = WRITE_ENABLE_CMD; 00828 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00829 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00830 sCommand.DataMode = QSPI_DATA_NONE; 00831 sCommand.DummyCycles = 0; 00832 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00833 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00834 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00835 00836 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00837 { 00838 return QSPI_ERROR; 00839 } 00840 00841 /* Configure automatic polling mode to wait for write enabling */ 00842 sConfig.Match = MX25R6435F_SR_WEL; 00843 sConfig.Mask = MX25R6435F_SR_WEL; 00844 sConfig.MatchMode = QSPI_MATCH_MODE_AND; 00845 sConfig.StatusBytesSize = 1; 00846 sConfig.Interval = 0x10; 00847 sConfig.AutomaticStop = QSPI_AUTOMATIC_STOP_ENABLE; 00848 00849 sCommand.Instruction = READ_STATUS_REG_CMD; 00850 sCommand.DataMode = QSPI_DATA_1_LINE; 00851 00852 if (HAL_QSPI_AutoPolling(&QSPIHandle, &sCommand, &sConfig, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00853 { 00854 return QSPI_ERROR; 00855 } 00856 00857 return QSPI_OK; 00858 } 00859 00860 /** 00861 * @brief This function read the SR of the memory and wait the EOP. 00862 * @param hqspi : QSPI handle 00863 * @param Timeout : Timeout for auto-polling 00864 * @retval None 00865 */ 00866 static uint8_t QSPI_AutoPollingMemReady(QSPI_HandleTypeDef *hqspi, uint32_t Timeout) 00867 { 00868 QSPI_CommandTypeDef sCommand; 00869 QSPI_AutoPollingTypeDef sConfig; 00870 00871 /* Configure automatic polling mode to wait for memory ready */ 00872 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00873 sCommand.Instruction = READ_STATUS_REG_CMD; 00874 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00875 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00876 sCommand.DataMode = QSPI_DATA_1_LINE; 00877 sCommand.DummyCycles = 0; 00878 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00879 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00880 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00881 00882 sConfig.Match = 0; 00883 sConfig.Mask = MX25R6435F_SR_WIP; 00884 sConfig.MatchMode = QSPI_MATCH_MODE_AND; 00885 sConfig.StatusBytesSize = 1; 00886 sConfig.Interval = 0x10; 00887 sConfig.AutomaticStop = QSPI_AUTOMATIC_STOP_ENABLE; 00888 00889 if (HAL_QSPI_AutoPolling(&QSPIHandle, &sCommand, &sConfig, Timeout) != HAL_OK) 00890 { 00891 return QSPI_ERROR; 00892 } 00893 00894 return QSPI_OK; 00895 } 00896 00897 /** 00898 * @brief This function enables/disables the Quad mode of the memory. 00899 * @param hqspi : QSPI handle 00900 * @param Operation : QSPI_QUAD_ENABLE or QSPI_QUAD_DISABLE mode 00901 * @retval None 00902 */ 00903 static uint8_t QSPI_QuadMode(QSPI_HandleTypeDef *hqspi, uint8_t Operation) 00904 { 00905 QSPI_CommandTypeDef sCommand; 00906 uint8_t reg; 00907 00908 /* Read status register */ 00909 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00910 sCommand.Instruction = READ_STATUS_REG_CMD; 00911 sCommand.AddressMode = QSPI_ADDRESS_NONE; 00912 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 00913 sCommand.DataMode = QSPI_DATA_1_LINE; 00914 sCommand.DummyCycles = 0; 00915 sCommand.NbData = 1; 00916 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 00917 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 00918 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 00919 00920 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00921 { 00922 return QSPI_ERROR; 00923 } 00924 00925 if (HAL_QSPI_Receive(&QSPIHandle, ®, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00926 { 00927 return QSPI_ERROR; 00928 } 00929 00930 /* Enable write operations */ 00931 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 00932 { 00933 return QSPI_ERROR; 00934 } 00935 00936 /* Activate/deactivate the Quad mode */ 00937 if (Operation == QSPI_QUAD_ENABLE) 00938 { 00939 SET_BIT(reg, MX25R6435F_SR_QE); 00940 } 00941 else 00942 { 00943 CLEAR_BIT(reg, MX25R6435F_SR_QE); 00944 } 00945 00946 sCommand.Instruction = WRITE_STATUS_CFG_REG_CMD; 00947 00948 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00949 { 00950 return QSPI_ERROR; 00951 } 00952 00953 if (HAL_QSPI_Transmit(&QSPIHandle, ®, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00954 { 00955 return QSPI_ERROR; 00956 } 00957 00958 /* Wait that memory is ready */ 00959 if (QSPI_AutoPollingMemReady(&QSPIHandle, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != QSPI_OK) 00960 { 00961 return QSPI_ERROR; 00962 } 00963 00964 /* Check the configuration has been correctly done */ 00965 sCommand.Instruction = READ_STATUS_REG_CMD; 00966 00967 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00968 { 00969 return QSPI_ERROR; 00970 } 00971 00972 if (HAL_QSPI_Receive(&QSPIHandle, ®, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 00973 { 00974 return QSPI_ERROR; 00975 } 00976 00977 if ((((reg & MX25R6435F_SR_QE) == 0) && (Operation == QSPI_QUAD_ENABLE)) || 00978 (((reg & MX25R6435F_SR_QE) != 0) && (Operation == QSPI_QUAD_DISABLE))) 00979 { 00980 return QSPI_ERROR; 00981 } 00982 00983 return QSPI_OK; 00984 } 00985 00986 /** 00987 * @brief This function enables/disables the high performance mode of the memory. 00988 * @param hqspi : QSPI handle 00989 * @param Operation : QSPI_HIGH_PERF_ENABLE or QSPI_HIGH_PERF_DISABLE high performance mode 00990 * @retval None 00991 */ 00992 static uint8_t QSPI_HighPerfMode(QSPI_HandleTypeDef *hqspi, uint8_t Operation) 00993 { 00994 QSPI_CommandTypeDef sCommand; 00995 uint8_t reg[3]; 00996 00997 /* Read status register */ 00998 sCommand.InstructionMode = QSPI_INSTRUCTION_1_LINE; 00999 sCommand.Instruction = READ_STATUS_REG_CMD; 01000 sCommand.AddressMode = QSPI_ADDRESS_NONE; 01001 sCommand.AlternateByteMode = QSPI_ALTERNATE_BYTES_NONE; 01002 sCommand.DataMode = QSPI_DATA_1_LINE; 01003 sCommand.DummyCycles = 0; 01004 sCommand.NbData = 1; 01005 sCommand.DdrMode = QSPI_DDR_MODE_DISABLE; 01006 sCommand.DdrHoldHalfCycle = QSPI_DDR_HHC_ANALOG_DELAY; 01007 sCommand.SIOOMode = QSPI_SIOO_INST_EVERY_CMD; 01008 01009 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01010 { 01011 return QSPI_ERROR; 01012 } 01013 01014 if (HAL_QSPI_Receive(&QSPIHandle, &(reg[0]), HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01015 { 01016 return QSPI_ERROR; 01017 } 01018 01019 /* Read configuration registers */ 01020 sCommand.Instruction = READ_CFG_REG_CMD; 01021 sCommand.NbData = 2; 01022 01023 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01024 { 01025 return QSPI_ERROR; 01026 } 01027 01028 if (HAL_QSPI_Receive(&QSPIHandle, &(reg[1]), HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01029 { 01030 return QSPI_ERROR; 01031 } 01032 01033 /* Enable write operations */ 01034 if (QSPI_WriteEnable(&QSPIHandle) != QSPI_OK) 01035 { 01036 return QSPI_ERROR; 01037 } 01038 01039 /* Activate/deactivate the Quad mode */ 01040 if (Operation == QSPI_HIGH_PERF_ENABLE) 01041 { 01042 SET_BIT(reg[2], MX25R6435F_CR2_LH_SWITCH); 01043 } 01044 else 01045 { 01046 CLEAR_BIT(reg[2], MX25R6435F_CR2_LH_SWITCH); 01047 } 01048 01049 sCommand.Instruction = WRITE_STATUS_CFG_REG_CMD; 01050 sCommand.NbData = 3; 01051 01052 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01053 { 01054 return QSPI_ERROR; 01055 } 01056 01057 if (HAL_QSPI_Transmit(&QSPIHandle, &(reg[0]), HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01058 { 01059 return QSPI_ERROR; 01060 } 01061 01062 /* Wait that memory is ready */ 01063 if (QSPI_AutoPollingMemReady(&QSPIHandle, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != QSPI_OK) 01064 { 01065 return QSPI_ERROR; 01066 } 01067 01068 /* Check the configuration has been correctly done */ 01069 sCommand.Instruction = READ_CFG_REG_CMD; 01070 sCommand.NbData = 2; 01071 01072 if (HAL_QSPI_Command(&QSPIHandle, &sCommand, HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01073 { 01074 return QSPI_ERROR; 01075 } 01076 01077 if (HAL_QSPI_Receive(&QSPIHandle, &(reg[0]), HAL_QPSI_TIMEOUT_DEFAULT_VALUE) != HAL_OK) 01078 { 01079 return QSPI_ERROR; 01080 } 01081 01082 if ((((reg[1] & MX25R6435F_CR2_LH_SWITCH) == 0) && (Operation == QSPI_HIGH_PERF_ENABLE)) || 01083 (((reg[1] & MX25R6435F_CR2_LH_SWITCH) != 0) && (Operation == QSPI_HIGH_PERF_DISABLE))) 01084 { 01085 return QSPI_ERROR; 01086 } 01087 01088 return QSPI_OK; 01089 } 01090 01091 /** 01092 * @} 01093 */ 01094 01095 /** 01096 * @} 01097 */ 01098 01099 /** 01100 * @} 01101 */ 01102 01103 /** 01104 * @} 01105 */ 01106 01107 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01108
Generated on Tue Jul 12 2022 13:55:42 by
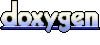