Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
stm32l475e_iot01_gyro.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01_gyro.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the gyroscope sensor 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00010 * All rights reserved.</center></h2> 00011 * 00012 * This software component is licensed by ST under BSD 3-Clause license, 00013 * the "License"; You may not use this file except in compliance with the 00014 * License. You may obtain a copy of the License at: 00015 * opensource.org/licenses/BSD-3-Clause 00016 * 00017 ****************************************************************************** 00018 */ 00019 00020 /* Includes ------------------------------------------------------------------*/ 00021 #include "stm32l475e_iot01_gyro.h" 00022 00023 /** @addtogroup BSP 00024 * @{ 00025 */ 00026 00027 /** @addtogroup STM32L475E_IOT01 00028 * @{ 00029 */ 00030 00031 /** @defgroup STM32L475E_IOT01_GYROSCOPE GYROSCOPE 00032 * @{ 00033 */ 00034 00035 /** @defgroup STM32L475E_IOT01_GYROSCOPE_Private_Variables GYROSCOPE Private Variables 00036 * @{ 00037 */ 00038 static GYRO_DrvTypeDef *GyroscopeDrv; 00039 00040 /** 00041 * @} 00042 */ 00043 00044 00045 /** @defgroup STM32L475E_IOT01_GYROSCOPE_Private_Functions GYROSCOPE Private Functions 00046 * @{ 00047 */ 00048 /** 00049 * @brief Initialize Gyroscope. 00050 * @retval GYRO_OK or GYRO_ERROR 00051 */ 00052 uint8_t BSP_GYRO_Init(void) 00053 { 00054 uint8_t ret = GYRO_ERROR; 00055 uint16_t ctrl = 0x0000; 00056 GYRO_InitTypeDef LSM6DSL_InitStructure; 00057 00058 if(Lsm6dslGyroDrv.ReadID() != LSM6DSL_ACC_GYRO_WHO_AM_I) 00059 { 00060 ret = GYRO_ERROR; 00061 } 00062 else 00063 { 00064 /* Initialize the gyroscope driver structure */ 00065 GyroscopeDrv = &Lsm6dslGyroDrv; 00066 00067 /* Configure Mems : data rate, power mode, full scale and axes */ 00068 LSM6DSL_InitStructure.Power_Mode = 0; 00069 LSM6DSL_InitStructure.Output_DataRate = LSM6DSL_ODR_52Hz; 00070 LSM6DSL_InitStructure.Axes_Enable = 0; 00071 LSM6DSL_InitStructure.Band_Width = 0; 00072 LSM6DSL_InitStructure.BlockData_Update = LSM6DSL_BDU_BLOCK_UPDATE; 00073 LSM6DSL_InitStructure.Endianness = 0; 00074 LSM6DSL_InitStructure.Full_Scale = LSM6DSL_GYRO_FS_2000; 00075 00076 /* Configure MEMS: data rate, full scale */ 00077 ctrl = (LSM6DSL_InitStructure.Full_Scale | LSM6DSL_InitStructure.Output_DataRate); 00078 00079 /* Configure MEMS: BDU and Auto-increment for multi read/write */ 00080 ctrl |= ((LSM6DSL_InitStructure.BlockData_Update | LSM6DSL_ACC_GYRO_IF_INC_ENABLED) << 8); 00081 00082 /* Initialize component */ 00083 GyroscopeDrv->Init(ctrl); 00084 00085 ret = GYRO_OK; 00086 } 00087 00088 return ret; 00089 } 00090 00091 00092 /** 00093 * @brief DeInitialize Gyroscope. 00094 */ 00095 void BSP_GYRO_DeInit(void) 00096 { 00097 /* DeInitialize the Gyroscope IO interfaces */ 00098 if(GyroscopeDrv != NULL) 00099 { 00100 if(GyroscopeDrv->DeInit!= NULL) 00101 { 00102 GyroscopeDrv->DeInit(); 00103 } 00104 } 00105 } 00106 00107 00108 /** 00109 * @brief Set/Unset Gyroscope in low power mode. 00110 * @param status 0 means disable Low Power Mode, otherwise Low Power Mode is enabled 00111 */ 00112 void BSP_GYRO_LowPower(uint16_t status) 00113 { 00114 /* Set/Unset component in low-power mode */ 00115 if(GyroscopeDrv != NULL) 00116 { 00117 if(GyroscopeDrv->LowPower!= NULL) 00118 { 00119 GyroscopeDrv->LowPower(status); 00120 } 00121 } 00122 } 00123 00124 /** 00125 * @brief Get XYZ angular acceleration from the Gyroscope. 00126 * @param pfData: pointer on floating array 00127 */ 00128 void BSP_GYRO_GetXYZ(float* pfData) 00129 { 00130 if(GyroscopeDrv != NULL) 00131 { 00132 if(GyroscopeDrv->GetXYZ!= NULL) 00133 { 00134 GyroscopeDrv->GetXYZ(pfData); 00135 } 00136 } 00137 } 00138 00139 /** 00140 * @} 00141 */ 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
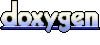