Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
stm32l475e_iot01.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01.c 00004 * @author MCD Application Team 00005 * @brief STM32L475E-IOT01 board support package 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00010 * All rights reserved.</center></h2> 00011 * 00012 * This software component is licensed by ST under BSD 3-Clause license, 00013 * the "License"; You may not use this file except in compliance with the 00014 * License. You may obtain a copy of the License at: 00015 * opensource.org/licenses/BSD-3-Clause 00016 * 00017 ****************************************************************************** 00018 */ 00019 00020 /* Includes ------------------------------------------------------------------*/ 00021 #include "stm32l475e_iot01.h" 00022 00023 /** @defgroup BSP BSP 00024 * @{ 00025 */ 00026 00027 /** @defgroup STM32L475E_IOT01 STM32L475E_IOT01 00028 * @{ 00029 */ 00030 00031 /** @defgroup STM32L475E_IOT01_LOW_LEVEL LOW LEVEL 00032 * @{ 00033 */ 00034 00035 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Defines LOW LEVEL Private Def 00036 * @{ 00037 */ 00038 /** 00039 * @brief STM32L475E IOT01 BSP Driver version number 00040 */ 00041 #define __STM32L475E_IOT01_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00042 #define __STM32L475E_IOT01_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00043 #define __STM32L475E_IOT01_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00044 #define __STM32L475E_IOT01_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00045 #define __STM32L475E_IOT01_BSP_VERSION ((__STM32L475E_IOT01_BSP_VERSION_MAIN << 24)\ 00046 |(__STM32L475E_IOT01_BSP_VERSION_SUB1 << 16)\ 00047 |(__STM32L475E_IOT01_BSP_VERSION_SUB2 << 8 )\ 00048 |(__STM32L475E_IOT01_BSP_VERSION_RC)) 00049 /** 00050 * @} 00051 */ 00052 00053 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Variables LOW LEVEL Variables 00054 * @{ 00055 */ 00056 00057 const uint32_t GPIO_PIN[LEDn] = {LED2_PIN}; 00058 00059 00060 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED2_GPIO_PORT}; 00061 00062 00063 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00064 00065 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00066 00067 const uint16_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00068 00069 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00070 00071 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00072 00073 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00074 00075 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00076 00077 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00078 00079 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00080 00081 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00082 00083 I2C_HandleTypeDef hI2cHandler; 00084 UART_HandleTypeDef hDiscoUart; 00085 00086 /** 00087 * @} 00088 */ 00089 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_FunctionPrototypes LOW LEVEL Private Function Prototypes 00090 * @{ 00091 */ 00092 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00093 static void I2Cx_MspDeInit(I2C_HandleTypeDef *i2c_handler); 00094 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00095 static void I2Cx_DeInit(I2C_HandleTypeDef *i2c_handler); 00096 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00097 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00098 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00099 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00100 00101 /* Sensors IO functions */ 00102 void SENSOR_IO_Init(void); 00103 void SENSOR_IO_DeInit(void); 00104 void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00105 uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg); 00106 uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00107 void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00108 HAL_StatusTypeDef SENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00109 void SENSOR_IO_Delay(uint32_t Delay); 00110 00111 void NFC_IO_Init(uint8_t GpoIrqEnable); 00112 void NFC_IO_DeInit(void); 00113 uint16_t NFC_IO_ReadMultiple (uint8_t Addr, uint8_t *pBuffer, uint16_t Length ); 00114 uint16_t NFC_IO_WriteMultiple (uint8_t Addr, uint8_t *pBuffer, uint16_t Length); 00115 uint16_t NFC_IO_IsDeviceReady (uint8_t Addr, uint32_t Trials); 00116 void NFC_IO_ReadState(uint8_t * pPinState); 00117 void NFC_IO_RfDisable(uint8_t PinState); 00118 void NFC_IO_Delay(uint32_t Delay); 00119 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Functions LOW LEVEL Private Functions 00125 * @{ 00126 */ 00127 00128 /** 00129 * @brief This method returns the STM32L475E IOT01 BSP Driver revision 00130 * @retval version 0xXYZR (8bits for each decimal, R for RC) 00131 */ 00132 uint32_t BSP_GetVersion(void) 00133 { 00134 return __STM32L475E_IOT01_BSP_VERSION; 00135 } 00136 00137 /** 00138 * @brief Initializes LED GPIO. 00139 * @param Led LED to be initialized. 00140 * This parameter can be one of the following values: 00141 * @arg DISCO_LED2 00142 */ 00143 void BSP_LED_Init(Led_TypeDef Led) 00144 { 00145 GPIO_InitTypeDef gpio_init_structure; 00146 00147 LEDx_GPIO_CLK_ENABLE(Led); 00148 /* Configure the GPIO_LED pin */ 00149 gpio_init_structure.Pin = GPIO_PIN[Led]; 00150 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00151 gpio_init_structure.Pull = GPIO_NOPULL; 00152 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00153 00154 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00155 } 00156 00157 /** 00158 * @brief DeInitializes LED GPIO. 00159 * @param Led LED to be deinitialized. 00160 * This parameter can be one of the following values: 00161 * @arg DISCO_LED2 00162 */ 00163 void BSP_LED_DeInit(Led_TypeDef Led) 00164 { 00165 GPIO_InitTypeDef gpio_init_structure; 00166 00167 /* DeInit the GPIO_LED pin */ 00168 gpio_init_structure.Pin = GPIO_PIN[Led]; 00169 00170 /* Turn off LED */ 00171 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00172 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00173 } 00174 00175 /** 00176 * @brief Turns the selected LED On. 00177 * @param Led LED to be set on 00178 * This parameter can be one of the following values: 00179 * @arg DISCO_LED2 00180 */ 00181 void BSP_LED_On(Led_TypeDef Led) 00182 { 00183 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00184 } 00185 00186 /** 00187 * @brief Turns the selected LED Off. 00188 * @param Led LED to be set off 00189 * This parameter can be one of the following values: 00190 * @arg DISCO_LED2 00191 */ 00192 void BSP_LED_Off(Led_TypeDef Led) 00193 { 00194 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00195 } 00196 00197 /** 00198 * @brief Toggles the selected LED. 00199 * @param Led LED to be toggled 00200 * This parameter can be one of the following values: 00201 * @arg DISCO_LED2 00202 */ 00203 void BSP_LED_Toggle(Led_TypeDef Led) 00204 { 00205 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00206 } 00207 00208 /** 00209 * @brief Initializes push button GPIO and EXTI Line. 00210 * @param Button Button to be configured 00211 * This parameter can be one of the following values: 00212 * @arg BUTTON_USER User Push Button 00213 * @param ButtonMode Button mode 00214 * This parameter can be one of the following values: 00215 * @arg BUTTON_MODE_GPIO Button will be used as simple IO 00216 * @arg BUTTON_MODE_EXTI Button will be connected to EXTI line 00217 * with interrupt generation capability 00218 */ 00219 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00220 { 00221 GPIO_InitTypeDef gpio_init_structure; 00222 00223 /* Enable the BUTTON clock */ 00224 USER_BUTTON_GPIO_CLK_ENABLE(); 00225 00226 if(ButtonMode == BUTTON_MODE_GPIO) 00227 { 00228 /* Configure Button pin as input */ 00229 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00230 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00231 gpio_init_structure.Pull = GPIO_PULLUP; 00232 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00233 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00234 } 00235 00236 if(ButtonMode == BUTTON_MODE_EXTI) 00237 { 00238 /* Configure Button pin as input with External interrupt */ 00239 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00240 gpio_init_structure.Pull = GPIO_PULLUP; 00241 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00242 00243 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00244 00245 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00246 00247 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00248 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00249 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00250 } 00251 } 00252 00253 /** 00254 * @brief DeInitializes push button. 00255 * @param Button Button to be configured 00256 * This parameter can be one of the following values: 00257 * @arg BUTTON_USER User Push Button 00258 * @note PB DeInit does not disable the GPIO clock 00259 */ 00260 void BSP_PB_DeInit(Button_TypeDef Button) 00261 { 00262 GPIO_InitTypeDef gpio_init_structure; 00263 00264 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00265 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00266 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00267 } 00268 00269 00270 /** 00271 * @brief Returns the selected button state. 00272 * @param Button Button to be checked 00273 * This parameter can be one of the following values: 00274 * @arg BUTTON_USER User Push Button 00275 * @retval The Button GPIO pin value (GPIO_PIN_RESET = button pressed) 00276 */ 00277 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00278 { 00279 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00280 } 00281 00282 /** 00283 * @brief Configures COM port. 00284 * @param COM COM port to be initialized. 00285 * This parameter can be one of the following values: 00286 * @arg COM1 00287 * @param huart Pointer to a UART_HandleTypeDef structure that contains the 00288 * configuration information for the specified USART peripheral. 00289 */ 00290 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00291 { 00292 GPIO_InitTypeDef gpio_init_structure; 00293 00294 /* Enable GPIO clock */ 00295 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00296 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00297 00298 /* Enable USART clock */ 00299 DISCOVERY_COMx_CLK_ENABLE(COM); 00300 00301 /* Configure USART Tx as alternate function */ 00302 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00303 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00304 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00305 gpio_init_structure.Pull = GPIO_NOPULL; 00306 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00307 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00308 00309 /* Configure USART Rx as alternate function */ 00310 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00311 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00312 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00313 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00314 00315 /* USART configuration */ 00316 huart->Instance = COM_USART[COM]; 00317 HAL_UART_Init(huart); 00318 } 00319 00320 /** 00321 * @brief DeInitializes COM port. 00322 * @param COM COM port to be deinitialized. 00323 * This parameter can be one of the following values: 00324 * @arg COM1 00325 * @param huart Pointer to a UART_HandleTypeDef structure that contains the 00326 * configuration information for the specified USART peripheral. 00327 */ 00328 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00329 { 00330 /* USART configuration */ 00331 huart->Instance = COM_USART[COM]; 00332 HAL_UART_DeInit(huart); 00333 00334 /* Enable USART clock */ 00335 DISCOVERY_COMx_CLK_DISABLE(COM); 00336 00337 /* DeInit GPIO pins can be done in the application 00338 (by surcharging this __weak function) */ 00339 00340 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00341 by surcharging this __weak function */ 00342 } 00343 00344 00345 /******************************************************************************* 00346 BUS OPERATIONS 00347 *******************************************************************************/ 00348 00349 /******************************* I2C Routines *********************************/ 00350 /** 00351 * @brief Initializes I2C MSP. 00352 * @param i2c_handler I2C handler 00353 * @retval None 00354 */ 00355 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00356 { 00357 GPIO_InitTypeDef gpio_init_structure; 00358 00359 /*** Configure the GPIOs ***/ 00360 /* Enable GPIO clock */ 00361 DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00362 00363 /* Configure I2C Tx, Rx as alternate function */ 00364 gpio_init_structure.Pin = DISCOVERY_I2Cx_SCL_PIN | DISCOVERY_I2Cx_SDA_PIN; 00365 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00366 gpio_init_structure.Pull = GPIO_PULLUP; 00367 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00368 gpio_init_structure.Alternate = DISCOVERY_I2Cx_SCL_SDA_AF; 00369 HAL_GPIO_Init(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00370 00371 HAL_GPIO_Init(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00372 00373 /*** Configure the I2C peripheral ***/ 00374 /* Enable I2C clock */ 00375 DISCOVERY_I2Cx_CLK_ENABLE(); 00376 00377 /* Force the I2C peripheral clock reset */ 00378 DISCOVERY_I2Cx_FORCE_RESET(); 00379 00380 /* Release the I2C peripheral clock reset */ 00381 DISCOVERY_I2Cx_RELEASE_RESET(); 00382 00383 /* Enable and set I2Cx Interrupt to a lower priority */ 00384 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_EV_IRQn, 0x0F, 0); 00385 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_EV_IRQn); 00386 00387 /* Enable and set I2Cx Interrupt to a lower priority */ 00388 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_ER_IRQn, 0x0F, 0); 00389 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_ER_IRQn); 00390 } 00391 00392 /** 00393 * @brief DeInitializes I2C MSP. 00394 * @param i2c_handler I2C handler 00395 * @retval None 00396 */ 00397 static void I2Cx_MspDeInit(I2C_HandleTypeDef *i2c_handler) 00398 { 00399 GPIO_InitTypeDef gpio_init_structure; 00400 00401 /* Configure I2C Tx, Rx as alternate function */ 00402 gpio_init_structure.Pin = DISCOVERY_I2Cx_SCL_PIN | DISCOVERY_I2Cx_SDA_PIN; 00403 HAL_GPIO_DeInit(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, gpio_init_structure.Pin); 00404 /* Disable GPIO clock */ 00405 DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_DISABLE(); 00406 00407 /* Disable I2C clock */ 00408 DISCOVERY_I2Cx_CLK_DISABLE(); 00409 } 00410 00411 /** 00412 * @brief Initializes I2C HAL. 00413 * @param i2c_handler I2C handler 00414 * @retval None 00415 */ 00416 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00417 { 00418 /* I2C configuration */ 00419 i2c_handler->Instance = DISCOVERY_I2Cx; 00420 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00421 i2c_handler->Init.OwnAddress1 = 0; 00422 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00423 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00424 i2c_handler->Init.OwnAddress2 = 0; 00425 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00426 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00427 00428 /* Init the I2C */ 00429 I2Cx_MspInit(i2c_handler); 00430 HAL_I2C_Init(i2c_handler); 00431 00432 /**Configure Analogue filter */ 00433 HAL_I2CEx_ConfigAnalogFilter(i2c_handler, I2C_ANALOGFILTER_ENABLE); 00434 } 00435 00436 /** 00437 * @brief DeInitializes I2C HAL. 00438 * @param i2c_handler I2C handler 00439 * @retval None 00440 */ 00441 static void I2Cx_DeInit(I2C_HandleTypeDef *i2c_handler) 00442 { /* DeInit the I2C */ 00443 I2Cx_MspDeInit(i2c_handler); 00444 HAL_I2C_DeInit(i2c_handler); 00445 } 00446 00447 /** 00448 * @brief Reads multiple data. 00449 * @param i2c_handler I2C handler 00450 * @param Addr I2C address 00451 * @param Reg Reg address 00452 * @param MemAddress memory address 00453 * @param Buffer Pointer to data buffer 00454 * @param Length Length of the data 00455 * @retval HAL status 00456 */ 00457 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00458 { 00459 HAL_StatusTypeDef status = HAL_OK; 00460 00461 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00462 00463 /* Check the communication status */ 00464 if(status != HAL_OK) 00465 { 00466 /* I2C error occured */ 00467 I2Cx_Error(i2c_handler, Addr); 00468 } 00469 return status; 00470 } 00471 00472 00473 /** 00474 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00475 * @param i2c_handler I2C handler 00476 * @param Addr Device address on BUS Bus. 00477 * @param Reg The target register address to write 00478 * @param MemAddress memory address 00479 * @param Buffer The target register value to be written 00480 * @param Length buffer size to be written 00481 * @retval HAL status 00482 */ 00483 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00484 { 00485 HAL_StatusTypeDef status = HAL_OK; 00486 00487 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00488 00489 /* Check the communication status */ 00490 if(status != HAL_OK) 00491 { 00492 /* Re-Initiaize the I2C Bus */ 00493 I2Cx_Error(i2c_handler, Addr); 00494 } 00495 return status; 00496 } 00497 00498 /** 00499 * @brief Checks if target device is ready for communication. 00500 * @note This function is used with Memory devices 00501 * @param i2c_handler I2C handler 00502 * @param DevAddress Target device address 00503 * @param Trials Number of trials 00504 * @retval HAL status 00505 */ 00506 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00507 { 00508 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00509 } 00510 00511 /** 00512 * @brief Manages error callback by re-initializing I2C. 00513 * @param i2c_handler I2C handler 00514 * @param Addr I2C Address 00515 * @retval None 00516 */ 00517 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00518 { 00519 /* De-initialize the I2C communication bus */ 00520 HAL_I2C_DeInit(i2c_handler); 00521 00522 /* Re-Initialize the I2C communication bus */ 00523 I2Cx_Init(i2c_handler); 00524 } 00525 00526 /** 00527 * @} 00528 */ 00529 00530 /******************************************************************************* 00531 LINK OPERATIONS 00532 *******************************************************************************/ 00533 /******************************** LINK Sensors ********************************/ 00534 00535 /** 00536 * @brief Initializes Sensors low level. 00537 * @retval None 00538 */ 00539 void SENSOR_IO_Init(void) 00540 { 00541 I2Cx_Init(&hI2cHandler); 00542 } 00543 00544 /** 00545 * @brief DeInitializes Sensors low level. 00546 * @retval None 00547 */ 00548 void SENSOR_IO_DeInit(void) 00549 { 00550 I2Cx_DeInit(&hI2cHandler); 00551 } 00552 00553 /** 00554 * @brief Writes a single data. 00555 * @param Addr I2C address 00556 * @param Reg Reg address 00557 * @param Value Data to be written 00558 * @retval None 00559 */ 00560 void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00561 { 00562 I2Cx_WriteMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00563 } 00564 00565 /** 00566 * @brief Reads a single data. 00567 * @param Addr I2C address 00568 * @param Reg Reg address 00569 * @retval Data to be read 00570 */ 00571 uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg) 00572 { 00573 uint8_t read_value = 0; 00574 00575 I2Cx_ReadMultiple(&hI2cHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00576 00577 return read_value; 00578 } 00579 00580 /** 00581 * @brief Reads multiple data with I2C communication 00582 * channel from TouchScreen. 00583 * @param Addr I2C address 00584 * @param Reg Register address 00585 * @param Buffer Pointer to data buffer 00586 * @param Length Length of the data 00587 * @retval HAL status 00588 */ 00589 uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00590 { 00591 return I2Cx_ReadMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00592 } 00593 00594 /** 00595 * @brief Writes multiple data with I2C communication 00596 * channel from MCU to TouchScreen. 00597 * @param Addr I2C address 00598 * @param Reg Register address 00599 * @param Buffer Pointer to data buffer 00600 * @param Length Length of the data 00601 * @retval None 00602 */ 00603 void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00604 { 00605 I2Cx_WriteMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00606 } 00607 00608 /** 00609 * @brief Checks if target device is ready for communication. 00610 * @note This function is used with Memory devices 00611 * @param DevAddress Target device address 00612 * @param Trials Number of trials 00613 * @retval HAL status 00614 */ 00615 HAL_StatusTypeDef SENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00616 { 00617 return (I2Cx_IsDeviceReady(&hI2cHandler, DevAddress, Trials)); 00618 } 00619 00620 /** 00621 * @brief Delay function used in Sensor low level driver. 00622 * @param Delay Delay in ms 00623 * @retval None 00624 */ 00625 void SENSOR_IO_Delay(uint32_t Delay) 00626 { 00627 HAL_Delay(Delay); 00628 } 00629 00630 /******************************** LINK NFC ********************************/ 00631 00632 /** 00633 * @brief Initializes Sensors low level. 00634 * @param GpoIrqEnable 0x0 is disable, otherwise enabled 00635 * @retval None 00636 */ 00637 void NFC_IO_Init(uint8_t GpoIrqEnable) 00638 { 00639 GPIO_InitTypeDef GPIO_InitStruct; 00640 00641 /* I2C init */ 00642 I2Cx_Init(&hI2cHandler); 00643 00644 /* GPIO Ports Clock Enable */ 00645 NFC_GPIO_CLK_ENABLE(); 00646 00647 /* Configure GPIO pins for GPO (PE4) */ 00648 if(GpoIrqEnable == 0) 00649 { 00650 GPIO_InitStruct.Pin = NFC_GPIO_GPO_PIN; 00651 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00652 GPIO_InitStruct.Pull = GPIO_PULLUP; 00653 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00654 HAL_GPIO_Init(NFC_GPIO_GPO_PIN_PORT, &GPIO_InitStruct); 00655 } 00656 else 00657 { 00658 GPIO_InitStruct.Pin = NFC_GPIO_GPO_PIN; 00659 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00660 GPIO_InitStruct.Pull = GPIO_PULLUP; 00661 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00662 HAL_GPIO_Init(NFC_GPIO_GPO_PIN_PORT, &GPIO_InitStruct); 00663 /* Enable and set EXTI4_IRQn Interrupt to the lowest priority */ 00664 HAL_NVIC_SetPriority(EXTI4_IRQn, 3, 0); 00665 HAL_NVIC_EnableIRQ(EXTI4_IRQn); 00666 } 00667 00668 /* Configure GPIO pins for DISABLE (PE2)*/ 00669 GPIO_InitStruct.Pin = NFC_GPIO_RFDISABLE_PIN; 00670 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00671 GPIO_InitStruct.Pull = GPIO_NOPULL; 00672 HAL_GPIO_Init(NFC_GPIO_RFDISABLE_PIN_PORT, &GPIO_InitStruct); 00673 } 00674 00675 /** 00676 * @brief DeInitializes Sensors low level. 00677 * @retval None 00678 */ 00679 void NFC_IO_DeInit(void) 00680 { 00681 I2Cx_DeInit(&hI2cHandler); 00682 } 00683 00684 /** 00685 * @brief This functions reads a response of the M24SR device 00686 * @param Addr: M24SR I2C address (do we really need to add??) 00687 * @param pBuffer Pointer on the buffer to retrieve M24SR response 00688 * @param Length Length of the data 00689 * @retval Status Success or Timeout 00690 */ 00691 uint16_t NFC_IO_ReadMultiple (uint8_t Addr, uint8_t *pBuffer, uint16_t Length ) 00692 { 00693 uint16_t status ; 00694 00695 /* Before calling this function M24SR must be ready: check to detect potential issues */ 00696 status = NFC_IO_IsDeviceReady(Addr, NFC_I2C_TRIALS); 00697 if (status != NFC_I2C_STATUS_SUCCESS) 00698 { 00699 return NFC_I2C_ERROR_TIMEOUT; 00700 } 00701 00702 if( HAL_I2C_Master_Receive(&hI2cHandler, Addr, (uint8_t*)pBuffer, Length, NFC_I2C_TIMEOUT_STD) != HAL_OK) 00703 { 00704 return NFC_I2C_ERROR_TIMEOUT; 00705 } 00706 00707 return NFC_I2C_STATUS_SUCCESS; 00708 } 00709 00710 /** 00711 * @brief This functions sends the command buffer 00712 * @param Addr: M24SR I2C address (do we really need to add??) 00713 * @param pBuffer pointer to the buffer to send to the M24SR 00714 * @param Length Length of the data 00715 * @retval Status Success or Timeout 00716 */ 00717 uint16_t NFC_IO_WriteMultiple (uint8_t Addr, uint8_t *pBuffer, uint16_t Length) 00718 { 00719 uint16_t status ; 00720 00721 /* Before calling this function M24SR must be ready: check to detect potential issues */ 00722 status = NFC_IO_IsDeviceReady(Addr, NFC_I2C_TRIALS); 00723 if (status != NFC_I2C_STATUS_SUCCESS) 00724 { 00725 return NFC_I2C_ERROR_TIMEOUT; 00726 } 00727 00728 if( HAL_I2C_Master_Transmit(&hI2cHandler, Addr, (uint8_t*)pBuffer, Length, NFC_I2C_TIMEOUT_STD) != HAL_OK) 00729 { 00730 return NFC_I2C_ERROR_TIMEOUT; 00731 } 00732 00733 return NFC_I2C_STATUS_SUCCESS; 00734 } 00735 00736 /** 00737 * @brief Checks if target device is ready for communication. 00738 * @param Addr: M24SR I2C address (do we really need to add??) 00739 * @param Trials Number of trials (currently not present in M24sr) 00740 * @retval Status Success or Timeout 00741 */ 00742 uint16_t NFC_IO_IsDeviceReady (uint8_t Addr, uint32_t Trials) 00743 { 00744 HAL_StatusTypeDef status; 00745 uint32_t tickstart = 0; 00746 uint32_t currenttick = 0; 00747 00748 /* Get tick */ 00749 tickstart = HAL_GetTick(); 00750 00751 /* Wait until M24SR is ready or timeout occurs */ 00752 do 00753 { 00754 status = HAL_I2C_IsDeviceReady(&hI2cHandler, Addr, Trials, NFC_I2C_TIMEOUT_STD); 00755 currenttick = HAL_GetTick(); 00756 } while( ( (currenttick - tickstart) < NFC_I2C_TIMEOUT_MAX) && (status != HAL_OK) ); 00757 00758 if (status != HAL_OK) 00759 { 00760 return NFC_I2C_ERROR_TIMEOUT; 00761 } 00762 00763 return NFC_I2C_STATUS_SUCCESS; 00764 } 00765 00766 /** 00767 * @brief This function read the state of the M24SR GPO 00768 * @retval GPIO_PinState state of the M24SR GPO 00769 */ 00770 void NFC_IO_ReadState(uint8_t * pPinState) 00771 { 00772 *pPinState = (uint8_t)HAL_GPIO_ReadPin(NFC_GPIO_GPO_PIN_PORT,NFC_GPIO_GPO_PIN); 00773 } 00774 00775 /** 00776 * @brief This function sets the state of the M24SR RF disable pin 00777 * @param PinState put RF disable pin of M24SR in PinState (1 or 0) 00778 */ 00779 void NFC_IO_RfDisable(uint8_t PinState) 00780 { 00781 HAL_GPIO_WritePin(NFC_GPIO_RFDISABLE_PIN_PORT,NFC_GPIO_RFDISABLE_PIN,(GPIO_PinState)PinState); 00782 } 00783 00784 /** 00785 * @brief Delay function used in M24SR low level driver. 00786 * @param Delay Delay in ms 00787 * @retval None 00788 */ 00789 void NFC_IO_Delay(uint32_t Delay) 00790 { 00791 HAL_Delay(Delay); 00792 } 00793 00794 /** 00795 * @} 00796 */ 00797 00798 /** 00799 * @} 00800 */ 00801 00802 /** 00803 * @} 00804 */ 00805 00806 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
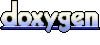