Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
lps22hb.h
00001 /** 00002 ****************************************************************************** 00003 * @file lps22hb.h 00004 * @author MCD Application Team 00005 * @brief LPS22HB header driver file 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00010 * All rights reserved.</center></h2> 00011 * 00012 * This software component is licensed by ST under BSD 3-Clause license, 00013 * the "License"; You may not use this file except in compliance with the 00014 * License. You may obtain a copy of the License at: 00015 * opensource.org/licenses/BSD-3-Clause 00016 * 00017 ****************************************************************************** 00018 */ 00019 00020 /* Define to prevent recursive inclusion -------------------------------------*/ 00021 #ifndef __LPS22HB__H 00022 #define __LPS22HB__H 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Includes ------------------------------------------------------------------*/ 00029 #include "../Common/psensor.h" 00030 #include "../Common/tsensor.h" 00031 00032 /** @addtogroup BSP 00033 * @{ 00034 */ 00035 00036 /** @addtogroup Component 00037 * @{ 00038 */ 00039 00040 /** @addtogroup LPS22HB 00041 * @{ 00042 */ 00043 00044 /** @defgroup LPS22HB_Exported_Macros LPS22HB Exported Macros 00045 * @{ 00046 */ 00047 00048 /** 00049 * @brief Bitfield positioning. 00050 */ 00051 #define LPS22HB_BIT(x) ((uint8_t)x) 00052 /** 00053 * @} 00054 */ 00055 00056 00057 /** @defgroup LPS22HB_Exported_Constants LPS22HB Exported Constants 00058 * @{ 00059 */ 00060 00061 /** 00062 * @brief Device Identification register. 00063 * Read 00064 * Default value: 0xB1 00065 * 7:0 This read-only register contains the device identifier that, for LPS22HB, is set to B1h. 00066 */ 00067 00068 #define LPS22HB_WHO_AM_I_REG (uint8_t)0x0F 00069 00070 /** 00071 * @brief Device Identification value. 00072 */ 00073 #define LPS22HB_WHO_AM_I_VAL (uint8_t)0xB1 00074 00075 /** 00076 * @brief Reference Pressure Register(LSB data) 00077 * Read/write 00078 * Default value: 0x00 00079 * 7:0 REFL7-0: Lower part of the reference pressure value that 00080 * is sum to the sensor output pressure. 00081 */ 00082 #define LPS22HB_REF_P_XL_REG (uint8_t)0x15 00083 00084 /** 00085 * @brief Reference Pressure Register (Middle data) 00086 * Read/write 00087 * Default value: 0x00 00088 * 7:0 REFL15-8: Middle part of the reference pressure value that 00089 * is sum to the sensor output pressure. 00090 */ 00091 #define LPS22HB_REF_P_L_REG (uint8_t)0x16 00092 00093 /** 00094 * @brief Reference Pressure Register (MSB data) 00095 * Read/write 00096 * Default value: 0x00 00097 * 7:0 REFL23-16 Higest part of the reference pressure value that 00098 * is sum to the sensor output pressure. 00099 */ 00100 #define LPS22HB_REF_P_H_REG (uint8_t)0x17 00101 00102 /** 00103 * @brief Pressure and temperature resolution mode Register 00104 * Read/write 00105 * Default value: 0x05 00106 * 7:2 These bits must be set to 0 for proper operation of the device 00107 * 1: Reserved 00108 * 0 LC_EN: Low Current Mode Enable. Default 0 00109 */ 00110 #define LPS22HB_RES_CONF_REG (uint8_t)0x1A 00111 #define LPS22HB_LCEN_MASK (uint8_t)0x01 00112 00113 /** 00114 * @brief Control Register 1 00115 * Read/write 00116 * Default value: 0x00 00117 * 7: This bit must be set to 0 for proper operation of the device 00118 * 6:4 ODR2, ODR1, ODR0: output data rate selection.Default 000 00119 * ODR2 | ODR1 | ODR0 | Pressure output data-rate(Hz) | Pressure output data-rate(Hz) 00120 * ---------------------------------------------------------------------------------- 00121 * 0 | 0 | 0 | one shot | one shot 00122 * 0 | 0 | 1 | 1 | 1 00123 * 0 | 1 | 0 | 10 | 10 00124 * 0 | 1 | 1 | 25 | 25 00125 * 1 | 0 | 0 | 50 | 50 00126 * 1 | 0 | 1 | 75 | 75 00127 * 1 | 1 | 0 | Reserved | Reserved 00128 * 1 | 1 | 1 | Reserved | Reserved 00129 * 00130 * 3 EN_LPFP: Enable Low Pass filter on Pressure data. Default value:0 00131 * 2:LPF_CFG Low-pass configuration register. (0: Filter cutoff is ODR/9; 1: filter cutoff is ODR/20) 00132 * 1 BDU: block data update. 0 - continuous update; 1 - output registers not updated until MSB and LSB reading. 00133 * 0 SIM: SPI Serial Interface Mode selection. 0 - SPI 4-wire; 1 - SPI 3-wire 00134 */ 00135 #define LPS22HB_CTRL_REG1 (uint8_t)0x10 00136 00137 #define LPS22HB_ODR_MASK (uint8_t)0x70 00138 #define LPS22HB_LPFP_MASK (uint8_t)0x08 00139 #define LPS22HB_LPFP_CUTOFF_MASK (uint8_t)0x04 00140 #define LPS22HB_BDU_MASK (uint8_t)0x02 00141 #define LPS22HB_SIM_MASK (uint8_t)0x01 00142 00143 #define LPS22HB_LPFP_BIT LPS22HB_BIT(3) 00144 00145 /** 00146 * @brief Control Register 2 00147 * Read/write 00148 * Default value: 0x10 00149 * 7 BOOT: Reboot memory content. 0: normal mode; 1: reboot memory content. Self-clearing upon completation 00150 * 6 FIFO_EN: FIFO Enable. 0: disable; 1: enable 00151 * 5 STOP_ON_FTH: Stop on FIFO Threshold FIFO Watermark level use. 0: disable; 1: enable 00152 * 4 IF_ADD_INC: Register address automatically incrementeed during a multiple byte access with a serial interface (I2C or SPI). 00153 * Default value 1.( 0: disable; 1: enable) 00154 * 3 I2C DIS: Disable I2C interface 0: I2C Enabled; 1: I2C disabled 00155 * 2 SWRESET: Software reset. 0: normal mode; 1: SW reset. Self-clearing upon completation 00156 * 1 AUTO_ZERO: Autozero enable. 0: normal mode; 1: autozero enable. 00157 * 0 ONE_SHOT: One shot enable. 0: waiting for start of conversion; 1: start for a new dataset 00158 */ 00159 #define LPS22HB_CTRL_REG2 (uint8_t)0x11 00160 00161 #define LPS22HB_BOOT_BIT LPS22HB_BIT(7) 00162 #define LPS22HB_FIFO_EN_BIT LPS22HB_BIT(6) 00163 #define LPS22HB_WTM_EN_BIT LPS22HB_BIT(5) 00164 #define LPS22HB_ADD_INC_BIT LPS22HB_BIT(4) 00165 #define LPS22HB_I2C_BIT LPS22HB_BIT(3) 00166 #define LPS22HB_SW_RESET_BIT LPS22HB_BIT(2) 00167 00168 #define LPS22HB_FIFO_EN_MASK (uint8_t)0x40 00169 #define LPS22HB_WTM_EN_MASK (uint8_t)0x20 00170 #define LPS22HB_ADD_INC_MASK (uint8_t)0x10 00171 #define LPS22HB_I2C_MASK (uint8_t)0x08 00172 #define LPS22HB_ONE_SHOT_MASK (uint8_t)0x01 00173 00174 00175 /** 00176 * @brief CTRL Reg3 Interrupt Control Register 00177 * Read/write 00178 * Default value: 0x00 00179 * 7 INT_H_L: Interrupt active high, low. 0:active high; 1: active low. 00180 * 6 PP_OD: Push-Pull/OpenDrain selection on interrupt pads. 0: Push-pull; 1: open drain. 00181 * 5 F_FSS5: FIFO full flag on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00182 * 4 F_FTH: FIFO threshold (watermark) status on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00183 * 3 F_OVR: FIFO overrun interrupt on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00184 * 2 DRDY: Data-ready signal on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00185 * 1:0 INT_S2, INT_S1: data signal on INT pad control bits. 00186 * INT_S2 | INT_S1 | INT pin 00187 * ------------------------------------------------------ 00188 * 0 | 0 | Data signal( in order of priority:PTH_DRDY or F_FTH or F_OVR_or F_FSS5 00189 * 0 | 1 | Pressure high (P_high) 00190 * 1 | 0 | Pressure low (P_low) 00191 * 1 | 1 | P_low OR P_high 00192 */ 00193 #define LPS22HB_CTRL_REG3 (uint8_t)0x12 00194 00195 #define LPS22HB_PP_OD_BIT LPS22HB_BIT(6) 00196 #define LPS22HB_FIFO_FULL_BIT LPS22HB_BIT(5) 00197 #define LPS22HB_FIFO_FTH_BIT LPS22HB_BIT(4) 00198 #define LPS22HB_FIFO_OVR_BIT LPS22HB_BIT(3) 00199 #define LPS22HB_DRDY_BIT LPS22HB_BIT(2) 00200 00201 00202 #define LPS22HB_INT_H_L_MASK (uint8_t)0x80 00203 #define LPS22HB_PP_OD_MASK (uint8_t)0x40 00204 #define LPS22HB_FIFO_FULL_MASK (uint8_t)0x20 00205 #define LPS22HB_FIFO_FTH_MASK (uint8_t)0x10 00206 #define LPS22HB_FIFO_OVR_MASK (uint8_t)0x08 00207 #define LPS22HB_DRDY_MASK (uint8_t)0x04 00208 #define LPS22HB_INT_S12_MASK (uint8_t)0x03 00209 00210 00211 /** 00212 * @brief Interrupt Differential configuration Register 00213 * Read/write 00214 * Default value: 0x00. 00215 * 7 AUTORIFP: AutoRifP Enable 00216 * 6 RESET_ARP: Reset AutoRifP function 00217 * 4 AUTOZERO: Autozero enabled 00218 * 5 RESET_AZ: Reset Autozero Function 00219 * 3 DIFF_EN: Interrupt generation enable 00220 * 2 LIR: Latch Interrupt request into INT_SOURCE register. 0 - interrupt request not latched 00221 * 1 - interrupt request latched 00222 * 1 PL_E: Enable interrupt generation on differential pressure low event. 0 - disable; 1 - enable 00223 * 0 PH_E: Enable interrupt generation on differential pressure high event. 0 - disable; 1 - enable 00224 */ 00225 #define LPS22HB_INTERRUPT_CFG_REG (uint8_t)0x0B 00226 00227 #define LPS22HB_DIFF_EN_BIT LPS22HB_BIT(3) 00228 #define LPS22HB_LIR_BIT LPS22HB_BIT(2) 00229 #define LPS22HB_PLE_BIT LPS22HB_BIT(1) 00230 #define LPS22HB_PHE_BIT LPS22HB_BIT(0) 00231 00232 #define LPS22HB_AUTORIFP_MASK (uint8_t)0x80 00233 #define LPS22HB_RESET_ARP_MASK (uint8_t)0x40 00234 #define LPS22HB_AUTOZERO_MASK (uint8_t)0x20 00235 #define LPS22HB_RESET_AZ_MASK (uint8_t)0x10 00236 #define LPS22HB_DIFF_EN_MASK (uint8_t)0x08 00237 #define LPS22HB_LIR_MASK (uint8_t)0x04 00238 #define LPS22HB_PLE_MASK (uint8_t)0x02 00239 #define LPS22HB_PHE_MASK (uint8_t)0x01 00240 00241 00242 00243 /** 00244 * @brief Interrupt source Register (It is cleared by reading it) 00245 * Read 00246 * 7 BOOT_STATUS: If 1 indicates that the Boot (Reboot) phase is running. 00247 * 6:3 Reserved: Keep these bits at 0 00248 * 2 IA: Interrupt Active.0: no interrupt has been generated 00249 * 1: one or more interrupt events have been generated. 00250 * 1 PL: Differential pressure Low. 0: no interrupt has been generated 00251 * 1: Low differential pressure event has occurred. 00252 * 0 PH: Differential pressure High. 0: no interrupt has been generated 00253 * 1: High differential pressure event has occurred. 00254 */ 00255 #define LPS22HB_INTERRUPT_SOURCE_REG (uint8_t)0x25 00256 00257 #define LPS22HB_BOOT_STATUS_BIT LPS22HB_BIT(7) 00258 #define LPS22HB_IA_BIT LPS22HB_BIT(2) 00259 #define LPS22HB_PL_BIT LPS22HB_BIT(1) 00260 #define LPS22HB_PH_BIT LPS22HB_BIT(0) 00261 00262 #define LPS22HB_BOOT_STATUS_MASK (uint8_t)0x80 00263 #define LPS22HB_IA_MASK (uint8_t)0x04 00264 #define LPS22HB_PL_MASK (uint8_t)0x02 00265 #define LPS22HB_PH_MASK (uint8_t)0x01 00266 00267 00268 /** 00269 * @brief Status Register 00270 * Read 00271 * 7:6 Reserved: 0 00272 * 5 T_OR: Temperature data overrun. 0: no overrun has occurred 00273 * 1: a new data for temperature has overwritten the previous one. 00274 * 4 P_OR: Pressure data overrun. 0: no overrun has occurred 00275 * 1: new data for pressure has overwritten the previous one. 00276 * 3:2 Reserved: 0 00277 * 1 T_DA: Temperature data available. 0: new data for temperature is not yet available 00278 * 1: new data for temperature is available. 00279 * 0 P_DA: Pressure data available. 0: new data for pressure is not yet available 00280 * 1: new data for pressure is available. 00281 */ 00282 #define LPS22HB_STATUS_REG (uint8_t)0x27 00283 00284 #define LPS22HB_TOR_BIT LPS22HB_BIT(5) 00285 #define LPS22HB_POR_BIT LPS22HB_BIT(4) 00286 #define LPS22HB_TDA_BIT LPS22HB_BIT(1) 00287 #define LPS22HB_PDA_BIT LPS22HB_BIT(0) 00288 00289 #define LPS22HB_TOR_MASK (uint8_t)0x20 00290 #define LPS22HB_POR_MASK (uint8_t)0x10 00291 #define LPS22HB_TDA_MASK (uint8_t)0x02 00292 #define LPS22HB_PDA_MASK (uint8_t)0x01 00293 00294 00295 /** 00296 * @brief Pressure data (LSB) register. 00297 * Read 00298 * Default value: 0x00.(To be verified) 00299 * POUT7 - POUT0: Pressure data LSB (2's complement). 00300 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00301 * PRESS_OUT_XL)[dec]/4096. 00302 */ 00303 #define LPS22HB_PRESS_OUT_XL_REG (uint8_t)0x28 00304 00305 /** 00306 * @brief Pressure data (Middle part) register. 00307 * Read 00308 * Default value: 0x80. 00309 * POUT15 - POUT8: Pressure data middle part (2's complement). 00310 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00311 * PRESS_OUT_XL)[dec]/4096. 00312 */ 00313 #define LPS22HB_PRESS_OUT_L_REG (uint8_t)0x29 00314 00315 /** 00316 * @brief Pressure data (MSB) register. 00317 * Read 00318 * Default value: 0x2F. 00319 * POUT23 - POUT16: Pressure data MSB (2's complement). 00320 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00321 * PRESS_OUT_XL)[dec]/4096. 00322 */ 00323 #define LPS22HB_PRESS_OUT_H_REG (uint8_t)0x2A 00324 00325 /** 00326 * @brief Temperature data (LSB) register. 00327 * Read 00328 * Default value: 0x00. 00329 * TOUT7 - TOUT0: temperature data LSB. 00330 * Tout(degC)=TEMP_OUT/100 00331 */ 00332 #define LPS22HB_TEMP_OUT_L_REG (uint8_t)0x2B 00333 00334 /** 00335 * @brief Temperature data (MSB) register. 00336 * Read 00337 * Default value: 0x00. 00338 * TOUT15 - TOUT8: temperature data MSB. 00339 * Tout(degC)=TEMP_OUT/100 00340 */ 00341 #define LPS22HBH_TEMP_OUT_H_REG (uint8_t)0x2C 00342 00343 /** 00344 * @brief Threshold pressure (LSB) register. 00345 * Read/write 00346 * Default value: 0x00. 00347 * 7:0 THS7-THS0: LSB Threshold pressure Low part of threshold value for pressure interrupt 00348 * generation. The complete threshold value is given by THS_P_H & THS_P_L and is 00349 * expressed as unsigned number. P_ths(hPA)=(THS_P_H & THS_P_L)[dec]/16. 00350 */ 00351 #define LPS22HB_THS_P_LOW_REG (uint8_t)0x0C 00352 00353 /** 00354 * @brief Threshold pressure (MSB) 00355 * Read/write 00356 * Default value: 0x00. 00357 * 7:0 THS15-THS8: MSB Threshold pressure. High part of threshold value for pressure interrupt 00358 * generation. The complete threshold value is given by THS_P_H & THS_P_L and is 00359 * expressed as unsigned number. P_ths(mbar)=(THS_P_H & THS_P_L)[dec]/16. 00360 */ 00361 #define LPS22HB_THS_P_HIGH_REG (uint8_t)0x0D 00362 00363 /** 00364 * @brief FIFO control register 00365 * Read/write 00366 * Default value: 0x00 00367 * 7:5 F_MODE2, F_MODE1, F_MODE0: FIFO mode selection. 00368 * FM2 | FM1 | FM0 | FIFO MODE 00369 * --------------------------------------------------- 00370 * 0 | 0 | 0 | BYPASS MODE 00371 * 0 | 0 | 1 | FIFO MODE. Stops collecting data when full 00372 * 0 | 1 | 0 | STREAM MODE: Keep the newest measurements in the FIFO 00373 * 0 | 1 | 1 | STREAM MODE until trigger deasserted, then change to FIFO MODE 00374 * 1 | 0 | 0 | BYPASS MODE until trigger deasserted, then STREAM MODE 00375 * 1 | 0 | 1 | Reserved for future use 00376 * 1 | 1 | 0 | Reserved 00377 * 1 | 1 | 1 | BYPASS mode until trigger deasserted, then FIFO MODE 00378 * 00379 * 4:0 WTM_POINT4-0 : FIFO Watermark level selection (0-31) 00380 */ 00381 #define LPS22HB_CTRL_FIFO_REG (uint8_t)0x14 00382 00383 #define LPS22HB_FIFO_MODE_MASK (uint8_t)0xE0 00384 #define LPS22HB_WTM_POINT_MASK (uint8_t)0x1F 00385 00386 00387 /** 00388 * @brief FIFO Status register 00389 * Read 00390 * 7 FTH_FIFO: FIFO threshold status. 0:FIFO filling is lower than FTH level 00391 * 1: FIFO is equal or higher than FTH level. 00392 * 6 OVR: Overrun bit status. 0 - FIFO not full 00393 * 1 - FIFO is full and at least one sample in the FIFO has been overwritten. 00394 * 5:0 FSS: FIFO Stored data level. 000000: FIFO empty, 100000: FIFO is full and has 32 unread samples. 00395 */ 00396 #define LPS22HB_STATUS_FIFO_REG (uint8_t)0x26 00397 00398 #define LPS22HB_FTH_FIFO_BIT LPS22HB_BIT(7) 00399 #define LPS22HB_OVR_FIFO_BIT LPS22HB_BIT(6) 00400 00401 #define LPS22HB_FTH_FIFO_MASK (uint8_t)0x80 00402 #define LPS22HB_OVR_FIFO_MASK (uint8_t)0x40 00403 #define LPS22HB_LEVEL_FIFO_MASK (uint8_t)0x3F 00404 #define LPS22HB_FIFO_EMPTY (uint8_t)0x00 00405 #define LPS22HB_FIFO_FULL (uint8_t)0x20 00406 00407 00408 00409 /** 00410 * @brief Pressure offset register (LSB) 00411 * Read/write 00412 * Default value: 0x00 00413 * 7:0 RPDS7-0:Pressure Offset for 1 point calibration (OPC) after soldering. 00414 * This register contains the low part of the pressure offset value after soldering,for 00415 * differential pressure computing. The complete value is given by RPDS_L & RPDS_H 00416 * and is expressed as signed 2 complement value. 00417 */ 00418 #define LPS22HB_RPDS_L_REG (uint8_t)0x18 00419 00420 /** 00421 * @brief Pressure offset register (MSB) 00422 * Read/write 00423 * Default value: 0x00 00424 * 7:0 RPDS15-8:Pressure Offset for 1 point calibration (OPC) after soldering. 00425 * This register contains the high part of the pressure offset value after soldering (see description RPDS_L) 00426 */ 00427 #define LPS22HB_RPDS_H_REG (uint8_t)0x19 00428 00429 00430 /** 00431 * @brief Clock Tree Configuration register 00432 * Read/write 00433 * Default value: 0x00 00434 * 7:6 Reserved. 00435 * 5: CTE: Clock Tree Enhancement 00436 */ 00437 #define LPS22HB_CLOCK_TREE_CONFIGURATION (uint8_t)0x43 00438 #define LPS22HB_CTE_MASK (uint8_t)0x20 00439 00440 /** 00441 * @} 00442 */ 00443 00444 00445 /** @defgroup LPS22HB_Pressure_Exported_Functions LPS22HB Pressure Exported Functions 00446 * @{ 00447 */ 00448 /* PRESSURE functions */ 00449 void LPS22HB_P_Init(uint16_t DeviceAddr); 00450 uint8_t LPS22HB_P_ReadID(uint16_t DeviceAddr); 00451 float LPS22HB_P_ReadPressure(uint16_t DeviceAddr); 00452 /** 00453 * @} 00454 */ 00455 00456 /** @defgroup HTS221_PressImported_Globals PRESSURE Imported Globals 00457 * @{ 00458 */ 00459 /* PRESSURE driver structure */ 00460 extern PSENSOR_DrvTypeDef LPS22HB_P_Drv; 00461 /** 00462 * @} 00463 */ 00464 00465 /** @defgroup LPS22HB_Temperature_Exported_Functions LPS22HB Temperature Exported Functions 00466 * @{ 00467 */ 00468 /* TEMPERATURE functions */ 00469 void LPS22HB_T_Init(uint16_t DeviceAddr, TSENSOR_InitTypeDef *pInitStruct); 00470 float LPS22HB_T_ReadTemp(uint16_t DeviceAddr); 00471 /** 00472 * @} 00473 */ 00474 00475 /** @defgroup HTS221_TempImported_Globals Temperature Imported Globals 00476 * @{ 00477 */ 00478 /* Temperature driver structure */ 00479 extern TSENSOR_DrvTypeDef LPS22HB_T_Drv; 00480 /** 00481 * @} 00482 */ 00483 00484 /** @defgroup LPS22HB_Imported_Functions LPS22HB Imported Functions 00485 * @{ 00486 */ 00487 /* IO functions */ 00488 extern void SENSOR_IO_Init(void); 00489 extern void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00490 extern uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg); 00491 extern uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00492 extern void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00493 00494 /** 00495 * @} 00496 */ 00497 00498 #ifdef __cplusplus 00499 } 00500 #endif 00501 00502 #endif /* __LPS22HB__H */ 00503 00504 /** 00505 * @} 00506 */ 00507 00508 /** 00509 * @} 00510 */ 00511 00512 /** 00513 * @} 00514 */ 00515 00516 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
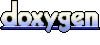