Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
lps22hb.c
00001 /** 00002 ****************************************************************************** 00003 * @file lps22hb.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the LPS22HB 00006 * pressure and temperature devices 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include "lps22hb.h" 00023 00024 /** @addtogroup BSP 00025 * @{ 00026 */ 00027 00028 /** @addtogroup Component 00029 * @{ 00030 */ 00031 00032 /** @defgroup LPS22HB LPS22HB 00033 * @{ 00034 */ 00035 00036 /** @defgroup LPS22HB_Private_FunctionsPrototypes LPS22HB Private Functions Prototypes 00037 * @{ 00038 */ 00039 static void LPS22HB_Init(uint16_t DeviceAddr); 00040 /** 00041 * @} 00042 */ 00043 00044 /** @defgroup LPS22HB_Private_Variables LPS22HB Private Variables 00045 * @{ 00046 */ 00047 /* Pressure Private Variables */ 00048 PSENSOR_DrvTypeDef LPS22HB_P_Drv = 00049 { 00050 LPS22HB_P_Init, 00051 LPS22HB_P_ReadID, 00052 LPS22HB_P_ReadPressure 00053 }; 00054 00055 /* Temperature Private Variables */ 00056 TSENSOR_DrvTypeDef LPS22HB_T_Drv = 00057 { 00058 LPS22HB_T_Init, 00059 0, 00060 0, 00061 LPS22HB_T_ReadTemp 00062 }; 00063 /** 00064 * @} 00065 */ 00066 00067 /** @defgroup LPS22HB_Pressure_Private_Functions LPS22HB Pressure Private Functions 00068 * @{ 00069 */ 00070 /** 00071 * @brief Set LPS22HB pressure sensor Initialization. 00072 */ 00073 void LPS22HB_P_Init(uint16_t DeviceAddr) 00074 { 00075 LPS22HB_Init(DeviceAddr); 00076 } 00077 00078 /** 00079 * @brief Read LPS22HB ID. 00080 * @retval ID 00081 */ 00082 uint8_t LPS22HB_P_ReadID(uint16_t DeviceAddr) 00083 { 00084 uint8_t ctrl = 0x00; 00085 00086 /* IO interface initialization */ 00087 SENSOR_IO_Init(); 00088 00089 /* Read value at Who am I register address */ 00090 ctrl = SENSOR_IO_Read(DeviceAddr, LPS22HB_WHO_AM_I_REG); 00091 00092 return ctrl; 00093 } 00094 00095 /** 00096 * @brief Read pressure value of LPS22HB 00097 * @retval pressure value 00098 */ 00099 float LPS22HB_P_ReadPressure(uint16_t DeviceAddr) 00100 { 00101 int32_t raw_press; 00102 uint8_t buffer[3]; 00103 uint32_t tmp = 0; 00104 uint8_t i; 00105 00106 for(i = 0; i < 3; i++) 00107 { 00108 buffer[i] = SENSOR_IO_Read(DeviceAddr, (LPS22HB_PRESS_OUT_XL_REG + i)); 00109 } 00110 00111 /* Build the raw data */ 00112 for(i = 0; i < 3; i++) 00113 tmp |= (((uint32_t)buffer[i]) << (8 * i)); 00114 00115 /* convert the 2's complement 24 bit to 2's complement 32 bit */ 00116 if(tmp & 0x00800000) 00117 tmp |= 0xFF000000; 00118 00119 raw_press = ((int32_t)tmp); 00120 00121 raw_press = (raw_press * 100) / 4096; 00122 00123 return (float)((float)raw_press / 100.0f); 00124 } 00125 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup LPS22HB_Temperature_Private_Functions LPS22HB Temperature Private Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Set LPS22HB temperature sensor Initialization. 00137 * @param DeviceAddr: I2C device address 00138 * @param InitStruct: pointer to a TSENSOR_InitTypeDef structure 00139 * that contains the configuration setting for the HTS221. 00140 * @retval None 00141 */ 00142 void LPS22HB_T_Init(uint16_t DeviceAddr, TSENSOR_InitTypeDef *pInitStruct) 00143 { 00144 LPS22HB_Init(DeviceAddr); 00145 } 00146 00147 /** 00148 * @brief Read temperature value of LPS22HB 00149 * @param DeviceAddr: I2C device address 00150 * @retval temperature value 00151 */ 00152 float LPS22HB_T_ReadTemp(uint16_t DeviceAddr) 00153 { 00154 int16_t raw_data; 00155 uint8_t buffer[2]; 00156 uint16_t tmp; 00157 uint8_t i; 00158 00159 for(i = 0; i < 2; i++) 00160 { 00161 buffer[i] = SENSOR_IO_Read(DeviceAddr, (LPS22HB_TEMP_OUT_L_REG + i)); 00162 } 00163 00164 /* Build the raw tmp */ 00165 tmp = (((uint16_t)buffer[1]) << 8) + (uint16_t)buffer[0]; 00166 00167 raw_data = (tmp * 10) / 100; 00168 00169 return ((float)(raw_data / 10.0f)); 00170 } 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** @addtogroup LPS22HB_Private_Functions LPS22HB Private functions 00177 * @{ 00178 */ 00179 00180 /** 00181 * @brief Set LPS22HB Initialization. 00182 * @param DeviceAddr: I2C device address 00183 * @retval None 00184 */ 00185 static void LPS22HB_Init(uint16_t DeviceAddr) 00186 { 00187 uint8_t tmp; 00188 00189 /* Set Power mode */ 00190 tmp = SENSOR_IO_Read(DeviceAddr, LPS22HB_RES_CONF_REG); 00191 00192 tmp &= ~LPS22HB_LCEN_MASK; 00193 tmp |= (uint8_t)0x01; /* Set low current mode */ 00194 00195 SENSOR_IO_Write(DeviceAddr, LPS22HB_RES_CONF_REG, tmp); 00196 00197 /* Read CTRL_REG1 */ 00198 tmp = SENSOR_IO_Read(DeviceAddr, LPS22HB_CTRL_REG1); 00199 00200 /* Set default ODR */ 00201 tmp &= ~LPS22HB_ODR_MASK; 00202 tmp |= (uint8_t)0x30; /* Set ODR to 25Hz */ 00203 00204 /* Enable BDU */ 00205 tmp &= ~LPS22HB_BDU_MASK; 00206 tmp |= ((uint8_t)0x02); 00207 00208 /* Apply settings to CTRL_REG1 */ 00209 SENSOR_IO_Write(DeviceAddr, LPS22HB_CTRL_REG1, tmp); 00210 } 00211 00212 /** 00213 * @} 00214 */ 00215 00216 /** 00217 * @} 00218 */ 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /** 00225 * @} 00226 */ 00227 00228 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
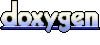