Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
lis3mdl.c
00001 /** 00002 ****************************************************************************** 00003 * @file lis3mdl.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the LIS3MDL 00006 * magnetometer devices 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include "lis3mdl.h" 00023 00024 /** @addtogroup BSP 00025 * @{ 00026 */ 00027 00028 /** @addtogroup Component 00029 * @{ 00030 */ 00031 00032 /** @defgroup LIS3MDL LIS3MDL 00033 * @{ 00034 */ 00035 00036 /** @defgroup LIS3MDL_Mag_Private_Variables LIS3MDL Mag Private Variables 00037 * @{ 00038 */ 00039 MAGNETO_DrvTypeDef Lis3mdlMagDrv = 00040 { 00041 LIS3MDL_MagInit, 00042 LIS3MDL_MagDeInit, 00043 LIS3MDL_MagReadID, 00044 0, 00045 LIS3MDL_MagLowPower, 00046 0, 00047 0, 00048 0, 00049 0, 00050 0, 00051 0, 00052 0, 00053 LIS3MDL_MagReadXYZ 00054 }; 00055 /** 00056 * @} 00057 */ 00058 00059 00060 /** @defgroup LIS3MDL_Mag_Private_Functions LIS3MDL Mag Private Functions 00061 * @{ 00062 */ 00063 /** 00064 * @brief Set LIS3MDL Magnetometer Initialization. 00065 * @param LIS3MDL_InitStruct: pointer to a LIS3MDL_MagInitTypeDef structure 00066 * that contains the configuration setting for the LIS3MDL. 00067 */ 00068 void LIS3MDL_MagInit(MAGNETO_InitTypeDef LIS3MDL_InitStruct) 00069 { 00070 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG1, LIS3MDL_InitStruct.Register1); 00071 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG2, LIS3MDL_InitStruct.Register2); 00072 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG3, LIS3MDL_InitStruct.Register3); 00073 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG4, LIS3MDL_InitStruct.Register4); 00074 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG5, LIS3MDL_InitStruct.Register5); 00075 } 00076 00077 /** 00078 * @brief LIS3MDL Magnetometer De-initialization. 00079 */ 00080 void LIS3MDL_MagDeInit(void) 00081 { 00082 uint8_t ctrl = 0x00; 00083 00084 /* Read control register 1 value */ 00085 ctrl = SENSOR_IO_Read(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG3); 00086 00087 /* Clear Selection Mode bits */ 00088 ctrl &= ~(LIS3MDL_MAG_SELECTION_MODE); 00089 00090 /* Set Power down */ 00091 ctrl |= LIS3MDL_MAG_POWERDOWN2_MODE; 00092 00093 /* write back control register */ 00094 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG3, ctrl); 00095 } 00096 00097 /** 00098 * @brief Read LIS3MDL ID. 00099 * @retval ID 00100 */ 00101 uint8_t LIS3MDL_MagReadID(void) 00102 { 00103 /* IO interface initialization */ 00104 SENSOR_IO_Init(); 00105 /* Read value at Who am I register address */ 00106 return (SENSOR_IO_Read(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_WHO_AM_I_REG)); 00107 } 00108 00109 /** 00110 * @brief Set/Unset Magnetometer in low power mode. 00111 * @param status 0 means disable Low Power Mode, otherwise Low Power Mode is enabled 00112 */ 00113 void LIS3MDL_MagLowPower(uint16_t status) 00114 { 00115 uint8_t ctrl = 0; 00116 00117 /* Read control register 1 value */ 00118 ctrl = SENSOR_IO_Read(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG3); 00119 00120 /* Clear Low Power Mode bit */ 00121 ctrl &= ~(0x20); 00122 00123 /* Set Low Power Mode */ 00124 if(status) 00125 { 00126 ctrl |= LIS3MDL_MAG_CONFIG_LOWPOWER_MODE; 00127 }else 00128 { 00129 ctrl |= LIS3MDL_MAG_CONFIG_NORMAL_MODE; 00130 } 00131 00132 /* write back control register */ 00133 SENSOR_IO_Write(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG3, ctrl); 00134 } 00135 00136 /** 00137 * @brief Read X, Y & Z Magnetometer values 00138 * @param pData: Data out pointer 00139 */ 00140 void LIS3MDL_MagReadXYZ(int16_t* pData) 00141 { 00142 int16_t pnRawData[3]; 00143 uint8_t ctrlm= 0; 00144 uint8_t buffer[6]; 00145 uint8_t i = 0; 00146 float sensitivity = 0; 00147 00148 /* Read the magnetometer control register content */ 00149 ctrlm = SENSOR_IO_Read(LIS3MDL_MAG_I2C_ADDRESS_HIGH, LIS3MDL_MAG_CTRL_REG2); 00150 00151 /* Read output register X, Y & Z acceleration */ 00152 SENSOR_IO_ReadMultiple(LIS3MDL_MAG_I2C_ADDRESS_HIGH, (LIS3MDL_MAG_OUTX_L | 0x80), buffer, 6); 00153 00154 for(i=0; i<3; i++) 00155 { 00156 pnRawData[i]=((((uint16_t)buffer[2*i+1]) << 8) + (uint16_t)buffer[2*i]); 00157 } 00158 00159 /* Normal mode */ 00160 /* Switch the sensitivity value set in the CRTL_REG2 */ 00161 switch(ctrlm & 0x60) 00162 { 00163 case LIS3MDL_MAG_FS_4_GA: 00164 sensitivity = LIS3MDL_MAG_SENSITIVITY_FOR_FS_4GA; 00165 break; 00166 case LIS3MDL_MAG_FS_8_GA: 00167 sensitivity = LIS3MDL_MAG_SENSITIVITY_FOR_FS_8GA; 00168 break; 00169 case LIS3MDL_MAG_FS_12_GA: 00170 sensitivity = LIS3MDL_MAG_SENSITIVITY_FOR_FS_12GA; 00171 break; 00172 case LIS3MDL_MAG_FS_16_GA: 00173 sensitivity = LIS3MDL_MAG_SENSITIVITY_FOR_FS_16GA; 00174 break; 00175 } 00176 00177 /* Obtain the mGauss value for the three axis */ 00178 for(i=0; i<3; i++) 00179 { 00180 pData[i]=( int16_t )(pnRawData[i] * sensitivity); 00181 } 00182 } 00183 00184 00185 /** 00186 * @} 00187 */ 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /** 00194 * @} 00195 */ 00196 00197 /** 00198 * @} 00199 */ 00200 00201 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00202
Generated on Tue Jul 12 2022 13:55:42 by
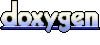