Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
idd.h
00001 /** 00002 ****************************************************************************** 00003 * @file idd.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the IDD driver. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00010 * All rights reserved.</center></h2> 00011 * 00012 * This software component is licensed by ST under BSD 3-Clause license, 00013 * the "License"; You may not use this file except in compliance with the 00014 * License. You may obtain a copy of the License at: 00015 * opensource.org/licenses/BSD-3-Clause 00016 * 00017 ****************************************************************************** 00018 */ 00019 00020 /* Define to prevent recursive inclusion -------------------------------------*/ 00021 #ifndef __IDD_H 00022 #define __IDD_H 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Includes ------------------------------------------------------------------*/ 00029 #include <stdint.h> 00030 00031 /** @addtogroup BSP 00032 * @{ 00033 */ 00034 00035 /** @addtogroup Components 00036 * @{ 00037 */ 00038 00039 /** @addtogroup IDD 00040 * @{ 00041 */ 00042 00043 /** @defgroup IDD_Exported_Types IDD Exported Types 00044 * @{ 00045 */ 00046 00047 /** @defgroup IDD_Config_structure IDD Configuration structure 00048 * @{ 00049 */ 00050 typedef struct 00051 { 00052 uint16_t AmpliGain; /*!< Specifies ampli gain value 00053 */ 00054 uint16_t VddMin; /*!< Specifies minimum MCU VDD can reach to protect MCU from reset 00055 */ 00056 uint16_t Shunt0Value; /*!< Specifies value of Shunt 0 if existing 00057 */ 00058 uint16_t Shunt1Value; /*!< Specifies value of Shunt 1 if existing 00059 */ 00060 uint16_t Shunt2Value; /*!< Specifies value of Shunt 2 if existing 00061 */ 00062 uint16_t Shunt3Value; /*!< Specifies value of Shunt 3 if existing 00063 */ 00064 uint16_t Shunt4Value; /*!< Specifies value of Shunt 4 if existing 00065 */ 00066 uint16_t Shunt0StabDelay; /*!< Specifies delay of Shunt 0 stabilization if existing 00067 */ 00068 uint16_t Shunt1StabDelay; /*!< Specifies delay of Shunt 1 stabilization if existing 00069 */ 00070 uint16_t Shunt2StabDelay; /*!< Specifies delay of Shunt 2 stabilization if existing 00071 */ 00072 uint16_t Shunt3StabDelay; /*!< Specifies delay of Shunt 3 stabilization if existing 00073 */ 00074 uint16_t Shunt4StabDelay; /*!< Specifies delay of Shunt 4 stabilization if existing 00075 */ 00076 uint8_t ShuntNbOnBoard; /*!< Specifies number of shunts that are present on board 00077 This parameter can be a value of @ref IDD_shunt_number */ 00078 uint8_t ShuntNbUsed; /*!< Specifies number of shunts used for measurement 00079 This parameter can be a value of @ref IDD_shunt_number */ 00080 uint8_t VrefMeasurement; /*!< Specifies if Vref is automatically measured before each Idd measurement 00081 This parameter can be a value of @ref IDD_Vref_Measurement */ 00082 uint8_t Calibration; /*!< Specifies if calibration is done before each Idd measurement 00083 */ 00084 uint8_t PreDelayUnit; /*!< Specifies Pre delay unit 00085 This parameter can be a value of @ref IDD_PreDelay */ 00086 uint8_t PreDelayValue; /*!< Specifies Pre delay value in selected unit 00087 */ 00088 uint8_t MeasureNb; /*!< Specifies number of Measure to be performed 00089 This parameter can be a value between 1 and 256 */ 00090 uint8_t DeltaDelayUnit; /*!< Specifies Delta delay unit 00091 This parameter can be a value of @ref IDD_DeltaDelay */ 00092 uint8_t DeltaDelayValue; /*!< Specifies Delta delay between 2 measures 00093 value can be between 1 and 128 */ 00094 }IDD_ConfigTypeDef; 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup IDD_Driver_structure IDD Driver structure 00100 * @{ 00101 */ 00102 typedef struct 00103 { 00104 void (*Init)(uint16_t); 00105 void (*DeInit)(uint16_t); 00106 uint16_t (*ReadID)(uint16_t); 00107 void (*Reset)(uint16_t); 00108 void (*LowPower)(uint16_t); 00109 void (*WakeUp)(uint16_t); 00110 void (*Start)(uint16_t); 00111 void (*Config)(uint16_t,IDD_ConfigTypeDef); 00112 void (*GetValue)(uint16_t, uint32_t *); 00113 void (*EnableIT)(uint16_t); 00114 void (*ClearIT)(uint16_t); 00115 uint8_t (*GetITStatus)(uint16_t); 00116 void (*DisableIT)(uint16_t); 00117 void (*ErrorEnableIT)(uint16_t); 00118 void (*ErrorClearIT)(uint16_t); 00119 uint8_t (*ErrorGetITStatus)(uint16_t); 00120 void (*ErrorDisableIT)(uint16_t); 00121 uint8_t (*ErrorGetSrc)(uint16_t); 00122 uint8_t (*ErrorGetCode)(uint16_t); 00123 }IDD_DrvTypeDef; 00124 /** 00125 * @} 00126 */ 00127 00128 /** 00129 * @} 00130 */ 00131 00132 /** 00133 * @} 00134 */ 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** 00141 * @} 00142 */ 00143 00144 #ifdef __cplusplus 00145 } 00146 #endif 00147 00148 #endif /* __IDD_H */ 00149 00150 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
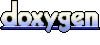