Contains the BSP driver for the B-L475E-IOT01 board.
Dependents: mbed-os-example-ble-Thermometer DISCO_L475VG_IOT01-Telegram-BOT DISCO_L475VG_IOT01-sche_cheveux DISCO_L475VG_IOT01-QSPI_FLASH_FILE_SYSTEM ... more
hts221.h
00001 /** 00002 ****************************************************************************** 00003 * @file hts221.h 00004 * @author MCD Application Team 00005 * @brief HTS221 header driver file 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© Copyright (c) 2017 STMicroelectronics. 00010 * All rights reserved.</center></h2> 00011 * 00012 * This software component is licensed by ST under BSD 3-Clause license, 00013 * the "License"; You may not use this file except in compliance with the 00014 * License. You may obtain a copy of the License at: 00015 * opensource.org/licenses/BSD-3-Clause 00016 * 00017 ****************************************************************************** 00018 */ 00019 00020 /* Define to prevent recursive inclusion -------------------------------------*/ 00021 #ifndef __HTS221__H 00022 #define __HTS221__H 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Includes ------------------------------------------------------------------*/ 00029 #include "../Common/hsensor.h" 00030 #include "../Common/tsensor.h" 00031 00032 /** @addtogroup BSP 00033 * @{ 00034 */ 00035 00036 /** @addtogroup Component 00037 * @{ 00038 */ 00039 00040 /** @addtogroup HTS221 00041 * @{ 00042 */ 00043 00044 /** @defgroup HTS221_Exported_Constants HTS221 Exported Constants 00045 * @{ 00046 */ 00047 00048 /** 00049 * @brief Bitfield positioning. 00050 */ 00051 #define HTS221_BIT(x) ((uint8_t)x) 00052 00053 00054 /** 00055 * @brief Device Identification register. 00056 * Read 00057 * Default value: 0xBC 00058 * 7:0 This read-only register contains the device identifier for HTS221. 00059 */ 00060 #define HTS221_WHO_AM_I_REG (uint8_t)0x0F 00061 00062 /** 00063 * @brief Device Identification value. 00064 */ 00065 #define HTS221_WHO_AM_I_VAL (uint8_t)0xBC 00066 00067 00068 /** 00069 * @brief Humidity and temperature average mode register. 00070 * Read/write 00071 * Default value: 0x1B 00072 * 7:6 Reserved. 00073 * 5:3 AVGT2-AVGT1-AVGT0: Select the temperature internal average. 00074 * 00075 * AVGT2 | AVGT1 | AVGT0 | Nr. Internal Average 00076 * ---------------------------------------------------- 00077 * 0 | 0 | 0 | 2 00078 * 0 | 0 | 1 | 4 00079 * 0 | 1 | 0 | 8 00080 * 0 | 1 | 1 | 16 00081 * 1 | 0 | 0 | 32 00082 * 1 | 0 | 1 | 64 00083 * 1 | 1 | 0 | 128 00084 * 1 | 1 | 1 | 256 00085 * 00086 * 2:0 AVGH2-AVGH1-AVGH0: Select humidity internal average. 00087 * AVGH2 | AVGH1 | AVGH0 | Nr. Internal Average 00088 * ------------------------------------------------------ 00089 * 0 | 0 | 0 | 4 00090 * 0 | 0 | 1 | 8 00091 * 0 | 1 | 0 | 16 00092 * 0 | 1 | 1 | 32 00093 * 1 | 0 | 0 | 64 00094 * 1 | 0 | 1 | 128 00095 * 1 | 1 | 0 | 256 00096 * 1 | 1 | 1 | 512 00097 * 00098 */ 00099 #define HTS221_AV_CONF_REG (uint8_t)0x10 00100 00101 #define HTS221_AVGT_BIT HTS221_BIT(3) 00102 #define HTS221_AVGH_BIT HTS221_BIT(0) 00103 00104 #define HTS221_AVGH_MASK (uint8_t)0x07 00105 #define HTS221_AVGT_MASK (uint8_t)0x38 00106 00107 /** 00108 * @brief Control register 1. 00109 * Read/write 00110 * Default value: 0x00 00111 * 7 PD: power down control. 0 - power down mode; 1 - active mode. 00112 * 6:3 Reserved. 00113 * 2 BDU: block data update. 0 - continuous update 00114 * 1 - output registers not updated until MSB and LSB reading. 00115 * 1:0 ODR1, ODR0: output data rate selection. 00116 * 00117 * ODR1 | ODR0 | Humidity output data-rate(Hz) | Pressure output data-rate(Hz) 00118 * ---------------------------------------------------------------------------------- 00119 * 0 | 0 | one shot | one shot 00120 * 0 | 1 | 1 | 1 00121 * 1 | 0 | 7 | 7 00122 * 1 | 1 | 12.5 | 12.5 00123 * 00124 */ 00125 #define HTS221_CTRL_REG1 (uint8_t)0x20 00126 00127 #define HTS221_PD_BIT HTS221_BIT(7) 00128 #define HTS221_BDU_BIT HTS221_BIT(2) 00129 #define HTS221_ODR_BIT HTS221_BIT(0) 00130 00131 #define HTS221_PD_MASK (uint8_t)0x80 00132 #define HTS221_BDU_MASK (uint8_t)0x04 00133 #define HTS221_ODR_MASK (uint8_t)0x03 00134 00135 /** 00136 * @brief Control register 2. 00137 * Read/write 00138 * Default value: 0x00 00139 * 7 BOOT: Reboot memory content. 0: normal mode 00140 * 1: reboot memory content. Self-cleared upon completation. 00141 * 6:2 Reserved. 00142 * 1 HEATHER: 0: heater enable; 1: heater disable. 00143 * 0 ONE_SHOT: 0: waiting for start of conversion 00144 * 1: start for a new dataset. Self-cleared upon completation. 00145 */ 00146 #define HTS221_CTRL_REG2 (uint8_t)0x21 00147 00148 #define HTS221_BOOT_BIT HTS221_BIT(7) 00149 #define HTS221_HEATHER_BIT HTS221_BIT(1) 00150 #define HTS221_ONESHOT_BIT HTS221_BIT(0) 00151 00152 #define HTS221_BOOT_MASK (uint8_t)0x80 00153 #define HTS221_HEATHER_MASK (uint8_t)0x02 00154 #define HTS221_ONE_SHOT_MASK (uint8_t)0x01 00155 00156 /** 00157 * @brief Control register 3. 00158 * Read/write 00159 * Default value: 0x00 00160 * 7 DRDY_H_L: Interrupt edge. 0: active high, 1: active low. 00161 * 6 PP_OD: Push-Pull/OpenDrain selection on interrupt pads. 0: push-pull 00162 * 1: open drain. 00163 * 5:3 Reserved. 00164 * 2 DRDY: interrupt config. 0: disable, 1: enable. 00165 */ 00166 #define HTS221_CTRL_REG3 (uint8_t)0x22 00167 00168 #define HTS221_DRDY_H_L_BIT HTS221_BIT(7) 00169 #define HTS221_PP_OD_BIT HTS221_BIT(6) 00170 #define HTS221_DRDY_BIT HTS221_BIT(2) 00171 00172 #define HTS221_DRDY_H_L_MASK (uint8_t)0x80 00173 #define HTS221_PP_OD_MASK (uint8_t)0x40 00174 #define HTS221_DRDY_MASK (uint8_t)0x04 00175 00176 /** 00177 * @brief Status register. 00178 * Read 00179 * Default value: 0x00 00180 * 7:2 Reserved. 00181 * 1 H_DA: Humidity data available. 0: new data for humidity is not yet available 00182 * 1: new data for humidity is available. 00183 * 0 T_DA: Temperature data available. 0: new data for temperature is not yet available 00184 * 1: new data for temperature is available. 00185 */ 00186 #define HTS221_STATUS_REG (uint8_t)0x27 00187 00188 #define HTS221_H_DA_BIT HTS221_BIT(1) 00189 #define HTS221_T_DA_BIT HTS221_BIT(0) 00190 00191 #define HTS221_HDA_MASK (uint8_t)0x02 00192 #define HTS221_TDA_MASK (uint8_t)0x01 00193 00194 /** 00195 * @brief Humidity data (LSB). 00196 * Read 00197 * Default value: 0x00. 00198 * HOUT7 - HOUT0: Humidity data LSB (2's complement). 00199 */ 00200 #define HTS221_HR_OUT_L_REG (uint8_t)0x28 00201 00202 /** 00203 * @brief Humidity data (MSB). 00204 * Read 00205 * Default value: 0x00. 00206 * HOUT15 - HOUT8: Humidity data MSB (2's complement). 00207 */ 00208 #define HTS221_HR_OUT_H_REG (uint8_t)0x29 00209 00210 /** 00211 * @brief Temperature data (LSB). 00212 * Read 00213 * Default value: 0x00. 00214 * TOUT7 - TOUT0: temperature data LSB. 00215 */ 00216 #define HTS221_TEMP_OUT_L_REG (uint8_t)0x2A 00217 00218 /** 00219 * @brief Temperature data (MSB). 00220 * Read 00221 * Default value: 0x00. 00222 * TOUT15 - TOUT8: temperature data MSB. 00223 */ 00224 #define HTS221_TEMP_OUT_H_REG (uint8_t)0x2B 00225 00226 /** 00227 * @brief Calibration registers. 00228 * Read 00229 */ 00230 #define HTS221_H0_RH_X2 (uint8_t)0x30 00231 #define HTS221_H1_RH_X2 (uint8_t)0x31 00232 #define HTS221_T0_DEGC_X8 (uint8_t)0x32 00233 #define HTS221_T1_DEGC_X8 (uint8_t)0x33 00234 #define HTS221_T0_T1_DEGC_H2 (uint8_t)0x35 00235 #define HTS221_H0_T0_OUT_L (uint8_t)0x36 00236 #define HTS221_H0_T0_OUT_H (uint8_t)0x37 00237 #define HTS221_H1_T0_OUT_L (uint8_t)0x3A 00238 #define HTS221_H1_T0_OUT_H (uint8_t)0x3B 00239 #define HTS221_T0_OUT_L (uint8_t)0x3C 00240 #define HTS221_T0_OUT_H (uint8_t)0x3D 00241 #define HTS221_T1_OUT_L (uint8_t)0x3E 00242 #define HTS221_T1_OUT_H (uint8_t)0x3F 00243 00244 /** 00245 * @} 00246 */ 00247 00248 00249 /** @defgroup HTS221_Humidity_Exported_Functions HTS221 Humidity Exported Functions 00250 * @{ 00251 */ 00252 /* HUMIDITY functions */ 00253 void HTS221_H_Init(uint16_t DeviceAddr); 00254 uint8_t HTS221_H_ReadID(uint16_t DeviceAddr); 00255 float HTS221_H_ReadHumidity(uint16_t DeviceAddr); 00256 /** 00257 * @} 00258 */ 00259 00260 /** @defgroup HTS221_HumImported_Globals Humidity Imported Globals 00261 * @{ 00262 */ 00263 /* Humidity driver structure */ 00264 extern HSENSOR_DrvTypeDef HTS221_H_Drv; 00265 /** 00266 * @} 00267 */ 00268 00269 /** @defgroup HTS221_Temperature_Exported_Functions HTS221 Temperature Exported Functions 00270 * @{ 00271 */ 00272 /* TEMPERATURE functions */ 00273 void HTS221_T_Init(uint16_t DeviceAddr, TSENSOR_InitTypeDef *pInitStruct); 00274 float HTS221_T_ReadTemp(uint16_t DeviceAddr); 00275 /** 00276 * @} 00277 */ 00278 00279 /** @defgroup HTS221_TempImported_Globals Temperature Imported Globals 00280 * @{ 00281 */ 00282 /* Temperature driver structure */ 00283 extern TSENSOR_DrvTypeDef HTS221_T_Drv; 00284 00285 /** 00286 * @} 00287 */ 00288 00289 /** @defgroup HTS221_Imported_Functions HTS221 Imported Functions 00290 * @{ 00291 */ 00292 /* IO functions */ 00293 extern void SENSOR_IO_Init(void); 00294 extern void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00295 extern uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg); 00296 extern uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00297 extern void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00298 /** 00299 * @} 00300 */ 00301 00302 #ifdef __cplusplus 00303 } 00304 #endif 00305 00306 #endif /* __HTS221__H */ 00307 00308 /** 00309 * @} 00310 */ 00311 00312 /** 00313 * @} 00314 */ 00315 00316 /** 00317 * @} 00318 */ 00319 00320 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 13:55:42 by
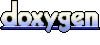