Class for BSP AUDIO for DISCO_F746NG
Dependents: DISCO-F746NG_Monttory_Base DISCO-F746NG_Monttory_Base DISCO-F746NG_AUDIO_demo DISCO-F746NG_AUDIO_demo_copy
AUDIO_DISCO_F746NG.h
00001 /* Copyright (c) 2010-2016 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __AUDIO_DISCO_F746NG_H 00020 #define __AUDIO_DISCO_F746NG_H 00021 00022 #ifdef TARGET_DISCO_F746NG 00023 00024 #include "mbed.h" 00025 #include "stm32746g_discovery_audio.h" 00026 00027 /* 00028 This class drives the uSD card driver mounted on DISCO_F746NG board. 00029 00030 Usage: 00031 00032 #include "mbed.h" 00033 #include "AUDIO_DISCO_F746NG.h" 00034 00035 AUDIO_DISCO_F746NG audio; 00036 00037 int main() 00038 { 00039 audio.IN_OUT_Init(INPUT_DEVICE_DIGITAL_MICROPHONE_2, OUTPUT_DEVICE_HEADPHONE, 90, DEFAULT_AUDIO_IN_FREQ): 00040 audio.IN_Record((uint16_t*)AUDIO_BUFFER_IN, AUDIO_BLOCK_SIZE); 00041 audio.OUT_SetAudioFrameSlot(CODEC_AUDIOFRAME_SLOT_02); 00042 audio.OUT_Play((uint16_t*)AUDIO_BUFFER_OUT, AUDIO_BLOCK_SIZE * 2); 00043 while 1 {} 00044 00045 } 00046 */ 00047 class AUDIO_DISCO_F746NG 00048 { 00049 00050 public: 00051 //! Constructor 00052 AUDIO_DISCO_F746NG(); 00053 00054 //! Destructor 00055 ~AUDIO_DISCO_F746NG(); 00056 00057 /** 00058 * @brief Configures the audio peripherals. 00059 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00060 * or OUTPUT_DEVICE_BOTH. 00061 * @param Volume: Initial volume level (from 0 (Mute); to 100 (Max)) 00062 * @param AudioFreq: Audio frequency used to play the audio stream. 00063 * @note The I2S PLL input clock must be done in the user application. 00064 * @retval AUDIO_OK if correct communication, else wrong communication 00065 */ 00066 uint8_t OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00067 00068 /** 00069 * @brief Starts playing audio stream from a data buffer for a determined size. 00070 * @param pBuffer: Pointer to the buffer 00071 * @param Size: Number of audio data in BYTES unit. 00072 * In memory, first element is for left channel, second element is for right channel 00073 * @retval AUDIO_OK if correct communication, else wrong communication 00074 */ 00075 uint8_t OUT_Play(uint16_t* pBuffer, uint32_t Size); 00076 00077 /** 00078 * @brief Sends n-Bytes on the SAI interface. 00079 * @param pData: pointer on data address 00080 * @param Size: number of data to be written 00081 * @retval None 00082 */ 00083 void OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00084 00085 /** 00086 * @brief This function Pauses the audio file stream. In case 00087 * of using DMA, the DMA Pause feature is used. 00088 * @note When calling OUT_Pause(); function for pause, only 00089 * OUT_Resume(); function should be called for resume (use of BSP_AUDIO_OUT_Play() 00090 * function for resume could lead to unexpected behaviour);. 00091 * @retval AUDIO_OK if correct communication, else wrong communication 00092 */ 00093 uint8_t OUT_Pause(void); 00094 00095 /** 00096 * @brief This function Resumes the audio file stream. 00097 * @note When calling OUT_Pause(); function for pause, only 00098 * OUT_Resume(); function should be called for resume (use of BSP_AUDIO_OUT_Play() 00099 * function for resume could lead to unexpected behaviour);. 00100 * @retval AUDIO_OK if correct communication, else wrong communication 00101 */ 00102 uint8_t OUT_Resume(void); 00103 00104 /** 00105 * @brief Stops audio playing and Power down the Audio Codec. 00106 * @param Option: could be one of the following parameters 00107 * - CODEC_PDWN_SW: for software power off (by writing registers);. 00108 * Then no need to reconfigure the Codec after power on. 00109 * - CODEC_PDWN_HW: completely shut down the codec (physically);. 00110 * Then need to reconfigure the Codec after power on. 00111 * @retval AUDIO_OK if correct communication, else wrong communication 00112 */ 00113 uint8_t OUT_Stop(uint32_t Option); 00114 00115 /** 00116 * @brief Controls the current audio volume level. 00117 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00118 * Mute and 100 for Max volume level);. 00119 * @retval AUDIO_OK if correct communication, else wrong communication 00120 */ 00121 uint8_t OUT_SetVolume(uint8_t Volume); 00122 00123 /** 00124 * @brief Enables or disables the MUTE mode by software 00125 * @param Cmd: Could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00126 * unmute the codec and restore previous volume level. 00127 * @retval AUDIO_OK if correct communication, else wrong communication 00128 */ 00129 uint8_t OUT_SetMute(uint32_t Cmd); 00130 00131 /** 00132 * @brief Switch dynamically (while audio file is played); the output target 00133 * (speaker or headphone);. 00134 * @param Output: The audio output target: OUTPUT_DEVICE_SPEAKER, 00135 * OUTPUT_DEVICE_HEADPHONE or OUTPUT_DEVICE_BOTH 00136 * @retval AUDIO_OK if correct communication, else wrong communication 00137 */ 00138 uint8_t OUT_SetOutputMode(uint8_t Output); 00139 00140 /** 00141 * @brief Updates the audio frequency. 00142 * @param AudioFreq: Audio frequency used to play the audio stream. 00143 * @note This API should be called after the OUT_Init(); to adjust the 00144 * audio frequency. 00145 * @retval None 00146 */ 00147 void OUT_SetFrequency(uint32_t AudioFreq); 00148 00149 /** 00150 * @brief Updates the Audio frame slot configuration. 00151 * @param AudioFrameSlot: specifies the audio Frame slot 00152 * This parameter can be one of the following values 00153 * @arg CODEC_AUDIOFRAME_SLOT_0123 00154 * @arg CODEC_AUDIOFRAME_SLOT_02 00155 * @arg CODEC_AUDIOFRAME_SLOT_13 00156 * @note This API should be called after the OUT_Init(); to adjust the 00157 * audio frame slot. 00158 * @retval None 00159 */ 00160 void OUT_SetAudioFrameSlot(uint32_t AudioFrameSlot); 00161 00162 /** 00163 * @brief Deinit the audio peripherals. 00164 * @retval None 00165 */ 00166 void OUT_DeInit(void); 00167 00168 /** 00169 * @brief Tx Transfer completed callbacks. 00170 * @param hsai: SAI handle 00171 * @retval None 00172 */ 00173 void HAL_SAI_TxCpltCallback(SAI_HandleTypeDef *hsai); 00174 00175 /** 00176 * @brief Tx Half Transfer completed callbacks. 00177 * @param hsai: SAI handle 00178 * @retval None 00179 */ 00180 void HAL_SAI_TxHalfCpltCallback(SAI_HandleTypeDef *hsai); 00181 00182 /** 00183 * @brief SAI error callbacks. 00184 * @param hsai: SAI handle 00185 * @retval None 00186 */ 00187 void HAL_SAI_ErrorCallback(SAI_HandleTypeDef *hsai); 00188 00189 /** 00190 * @brief Initializes the output Audio Codec audio interface (SAI);. 00191 * @param AudioFreq: Audio frequency to be configured for the SAI peripheral. 00192 * @note The default SlotActive configuration is set to CODEC_AUDIOFRAME_SLOT_0123 00193 * and user can update this configuration using 00194 * @retval None 00195 */ 00196 static void SAIx_Out_Init(uint32_t AudioFreq); 00197 00198 /** 00199 * @brief Deinitializes the output Audio Codec audio interface (SAI);. 00200 * @retval None 00201 */ 00202 static void SAIx_Out_DeInit(void); 00203 00204 /** 00205 * @brief Initializes wave recording. 00206 * @param InputDevice: INPUT_DEVICE_DIGITAL_MICROPHONE_2 or INPUT_DEVICE_INPUT_LINE_1 00207 * @param Volume: Initial volume level (in range 0(Mute);..80(+0dB)..100(+17.625dB)) 00208 * @param AudioFreq: Audio frequency to be configured for the SAI peripheral. 00209 * @retval AUDIO_OK if correct communication, else wrong communication 00210 */ 00211 uint8_t IN_Init(uint16_t InputDevice, uint8_t Volume, uint32_t AudioFreq); 00212 00213 /** 00214 * @brief Initializes wave recording and playback in parallel. 00215 * @param InputDevice: INPUT_DEVICE_DIGITAL_MICROPHONE_2 00216 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00217 * or OUTPUT_DEVICE_BOTH. 00218 * @param AudioFreq: Audio frequency to be configured for the SAI peripheral. 00219 * @param BitRes: Audio frequency to be configured. 00220 * @param ChnlNbr: Channel number. 00221 * @retval AUDIO_OK if correct communication, else wrong communication 00222 */ 00223 uint8_t IN_OUT_Init(uint16_t InputDevice, uint16_t OutputDevice, uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00224 00225 /** 00226 * @brief Starts audio recording. 00227 * @param pbuf: Main buffer pointer for the recorded data storing 00228 * @param size: size of the recorded buffer in number of elements (typically number of half-words); 00229 * Be careful that it is not the same unit than OUT_Play function 00230 * @retval AUDIO_OK if correct communication, else wrong communication 00231 */ 00232 uint8_t IN_Record(uint16_t* pbuf, uint32_t size); 00233 00234 /** 00235 * @brief Stops audio recording. 00236 * @param Option: could be one of the following parameters 00237 * - CODEC_PDWN_SW: for software power off (by writing registers);. 00238 * Then no need to reconfigure the Codec after power on. 00239 * - CODEC_PDWN_HW: completely shut down the codec (physically);. 00240 * Then need to reconfigure the Codec after power on. 00241 * @retval AUDIO_OK if correct communication, else wrong communication 00242 */ 00243 uint8_t IN_Stop(uint32_t Option); 00244 00245 /** 00246 * @brief Pauses the audio file stream. 00247 * @retval AUDIO_OK if correct communication, else wrong communication 00248 */ 00249 uint8_t IN_Pause(void); 00250 00251 /** 00252 * @brief Resumes the audio file stream. 00253 * @retval AUDIO_OK if correct communication, else wrong communication 00254 */ 00255 uint8_t IN_Resume(void); 00256 00257 /** 00258 * @brief Controls the audio in volume level. 00259 * @param Volume: Volume level in range 0(Mute);..80(+0dB)..100(+17.625dB) 00260 * @retval AUDIO_OK if correct communication, else wrong communication 00261 */ 00262 uint8_t IN_SetVolume(uint8_t Volume); 00263 00264 /** 00265 * @brief Deinit the audio IN peripherals. 00266 * @retval None 00267 */ 00268 void IN_DeInit(void); 00269 00270 /** 00271 * @brief Rx Transfer completed callbacks. 00272 * @param hsai: SAI handle 00273 * @retval None 00274 */ 00275 void HAL_SAI_RxCpltCallback(SAI_HandleTypeDef *hsai); 00276 00277 /** 00278 * @brief Rx Half Transfer completed callbacks. 00279 * @param hsai: SAI handle 00280 * @retval None 00281 */ 00282 void HAL_SAI_RxHalfCpltCallback(SAI_HandleTypeDef *hsai); 00283 00284 /** 00285 * @brief Initializes the input Audio Codec audio interface (SAI);. 00286 * @param SaiOutMode: SAI_MODEMASTER_TX (for record and playback in parallel); 00287 * or SAI_MODEMASTER_RX (for record only);. 00288 * @param SlotActive: CODEC_AUDIOFRAME_SLOT_02 or CODEC_AUDIOFRAME_SLOT_13 00289 * @param AudioFreq: Audio frequency to be configured for the SAI peripheral. 00290 * @retval None 00291 */ 00292 static void SAIx_In_Init(uint32_t SaiOutMode, uint32_t SlotActive, uint32_t AudioFreq); 00293 00294 /** 00295 * @brief Deinitializes the output Audio Codec audio interface (SAI);. 00296 * @retval None 00297 */ 00298 static void SAIx_In_DeInit(void); 00299 00300 private: 00301 00302 }; 00303 00304 #else 00305 #error "This class must be used with DISCO_F746NG board only." 00306 #endif // TARGET_DISCO_F746NG 00307 00308 #endif
Generated on Thu Jul 14 2022 09:28:47 by
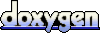