
Example application for the STMicroelectronics X-NUCLEO-NFC05A1
Dependencies: RFAL ST25R3911 BSP05
logger.cpp
00001 /****************************************************************************** 00002 * @attention 00003 * 00004 * <h2><center>© COPYRIGHT 2016 STMicroelectronics</center></h2> 00005 * 00006 * Licensed under ST MYLIBERTY SOFTWARE LICENSE AGREEMENT (the "License"); 00007 * You may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at: 00009 * 00010 * http://www.st.com/myliberty 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied, 00015 * AND SPECIFICALLY DISCLAIMING THE IMPLIED WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE, AND NON-INFRINGEMENT. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 * 00020 ******************************************************************************/ 00021 /* 00022 * PROJECT: 00023 * $Revision: $ 00024 * LANGUAGE: ANSI C 00025 */ 00026 00027 /*! \file 00028 * 00029 * \author 00030 * 00031 * \brief Debug log output utility implementation. 00032 * 00033 */ 00034 00035 /* 00036 ****************************************************************************** 00037 * INCLUDES 00038 ****************************************************************************** 00039 */ 00040 //#include "usart.h" 00041 #include "logger.h" 00042 #include "st_errno.h" 00043 #include <string.h> 00044 #include <stdarg.h> 00045 00046 /* 00047 ****************************************************************************** 00048 * LOCAL DEFINES 00049 ****************************************************************************** 00050 */ 00051 00052 00053 #if (USE_LOGGER == LOGGER_ON) 00054 00055 #define MAX_HEX_STR 4 00056 #define MAX_HEX_STR_LENGTH 128 00057 char hexStr[MAX_HEX_STR][MAX_HEX_STR_LENGTH]; 00058 uint8_t hexStrIdx = 0; 00059 #endif /* #if USE_LOGGER == LOGGER_ON */ 00060 00061 #define USART_TIMEOUT 1000 00062 00063 UART_HandleTypeDef *pLogUsart = 0; 00064 uint8_t logUsartTx(uint8_t *data, uint16_t dataLen); 00065 00066 /** 00067 * @brief This function initalize the UART handle. 00068 * @param husart : already initalized handle to USART HW 00069 * @retval none : 00070 */ 00071 void logUsartInit(UART_HandleTypeDef *husart) 00072 { 00073 pLogUsart = husart; 00074 } 00075 00076 /** 00077 * @brief This function Transmit data via USART 00078 * @param data : data to be transmitted 00079 * @param dataLen : length of data to be transmitted 00080 * @retval ERR_INVALID_HANDLE : in case the SPI HW is not initalized yet 00081 * @retval others : HAL status 00082 */ 00083 uint8_t logUsartTx(uint8_t *data, uint16_t dataLen) 00084 { 00085 if(pLogUsart == 0) 00086 return ERR_INVALID_HANDLE; 00087 00088 return HAL_UART_Transmit(pLogUsart, data, dataLen, USART_TIMEOUT); 00089 } 00090 00091 int logUsart(const char* format, ...) 00092 { 00093 #if (USE_LOGGER == LOGGER_ON) 00094 { 00095 #define LOG_BUFFER_SIZE 256 00096 char buf[LOG_BUFFER_SIZE]; 00097 va_list argptr; 00098 va_start(argptr, format); 00099 int cnt = vsnprintf(buf, LOG_BUFFER_SIZE, format, argptr); 00100 va_end(argptr); 00101 00102 /* */ 00103 logUsartTx((uint8_t*)buf, strlen(buf)); 00104 return cnt; 00105 } 00106 #else 00107 { 00108 return 0; 00109 } 00110 #endif /* #if USE_LOGGER == LOGGER_ON */ 00111 } 00112 00113 /* */ 00114 00115 char* hex2Str(unsigned char * data, size_t dataLen) 00116 { 00117 #if (USE_LOGGER == LOGGER_ON) 00118 { 00119 unsigned char * pin = data; 00120 const char * hex = "0123456789ABCDEF"; 00121 char * pout = hexStr[hexStrIdx]; 00122 uint8_t i = 0; 00123 uint8_t idx = hexStrIdx; 00124 if(dataLen == 0) 00125 { 00126 pout[0] = 0; 00127 } 00128 else 00129 { 00130 for(; i < dataLen - 1; ++i) 00131 { 00132 *pout++ = hex[(*pin>>4)&0xF]; 00133 *pout++ = hex[(*pin++)&0xF]; 00134 } 00135 *pout++ = hex[(*pin>>4)&0xF]; 00136 *pout++ = hex[(*pin)&0xF]; 00137 *pout = 0; 00138 } 00139 00140 hexStrIdx++; 00141 hexStrIdx %= MAX_HEX_STR; 00142 00143 return hexStr[idx]; 00144 } 00145 #else 00146 { 00147 return NULL; 00148 } 00149 #endif /* #if USE_LOGGER == LOGGER_ON */ 00150 }
Generated on Thu Jul 21 2022 02:04:36 by
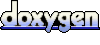