
Heart Rate monitor example for the Nucleo board and the Bluetooth Low Energy Nucleo board.
Dependencies: Nucleo_BLE_API Nucleo_BLE_BlueNRG mbed
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLEDevice.h" 00019 #include "HeartRateService.h" 00020 #include "BatteryService.h" 00021 #include "DeviceInformationService.h" 00022 #include "Utils.h" 00023 00024 BLEDevice ble; 00025 DigitalOut led1(LED1); 00026 00027 const static char DEVICE_NAME[] = "BlueNRG_HRM"; 00028 static const uint16_t uuid16_list[] = {GattService::UUID_HEART_RATE_SERVICE, 00029 GattService::UUID_BATTERY_SERVICE, 00030 GattService::UUID_DEVICE_INFORMATION_SERVICE}; 00031 static volatile bool triggerSensorPolling = false; 00032 00033 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00034 { 00035 DEBUG("Disconnected!\n\r"); 00036 DEBUG("Restarting the advertising process\n\r"); 00037 ble.startAdvertising(); // restart advertising 00038 } 00039 00040 void connectionCallback(Gap::Handle_t handle, const Gap::ConnectionParams_t *reason) 00041 { 00042 DEBUG("Connected\r\n"); 00043 } 00044 00045 void periodicCallback(void) 00046 { 00047 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00048 00049 /* Note that the periodicCallback() executes in interrupt context, so it is safer to do 00050 * heavy-weight sensor polling from the main thread. */ 00051 triggerSensorPolling = true; 00052 } 00053 00054 int main(void) 00055 { 00056 led1 = 1; 00057 Ticker ticker; 00058 ticker.attach(periodicCallback, 1); 00059 00060 DEBUG("Initialising \n\r"); 00061 ble.init(); 00062 ble.onDisconnection(disconnectionCallback); 00063 ble.onConnection(connectionCallback); 00064 00065 /* Setup primary service. */ 00066 uint8_t hrmCounter = 100; 00067 HeartRateService hrService(ble, hrmCounter, HeartRateService::LOCATION_FINGER); 00068 00069 /* Setup auxiliary services. */ 00070 BatteryService battery(ble); 00071 DeviceInformationService deviceInfo(ble, "ST", "Nucleo", "SN1", "hw-rev1", "fw-rev1", "soft-rev1"); 00072 00073 /* Setup advertising. */ 00074 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00075 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00076 ble.accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_HEART_RATE_SENSOR); 00077 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00078 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00079 ble.setAdvertisingInterval(1600); /* 1000ms; in multiples of 0.625ms. */ 00080 ble.startAdvertising(); 00081 00082 while (true) 00083 { 00084 if (triggerSensorPolling) 00085 { 00086 triggerSensorPolling = false; 00087 00088 /* Do blocking calls or whatever is necessary for sensor polling. */ 00089 /* In our case, we simply update the dummy HRM measurement. */ 00090 hrmCounter++; 00091 00092 if (hrmCounter == 175) 00093 { 00094 hrmCounter = 100; 00095 } 00096 00097 hrService.updateHeartRate(hrmCounter); 00098 } 00099 else 00100 { 00101 ble.waitForEvent(); 00102 } 00103 } 00104 }
Generated on Fri Jul 15 2022 09:10:11 by
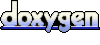