NUCLEO-NFC01A1 board drivers.
Dependents: Nucleo_NFC_Example I2C_NFC_Master Print_Entire_Nucleo_NFC01A1_Memory
Fork of X-NUCLEO-NFC01A1 by
drv_I2C_M24SR_mbed.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file drv_I2C_M24SR_mbed.cpp 00004 * @author MMY Application Team 00005 * @version V1.0.0 00006 * @date 19-March-2014 00007 * @brief This file provides a set of functions needed to manage the I2C of 00008 * the M24SR device. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT 2014 STMicroelectronics</center></h2> 00013 * 00014 * Licensed under MMY-ST Liberty SW License Agreement V2, (the "License"); 00015 * You may not use this file except in compliance with the License. 00016 * You may obtain a copy of the License at: 00017 * 00018 * http://www.st.com/software_license_agreement_liberty_v2 00019 * 00020 * Unless required by applicable law or agreed to in writing, software 00021 * distributed under the License is distributed on an "AS IS" BASIS, 00022 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00023 * See the License for the specific language governing permissions and 00024 * limitations under the License. 00025 * 00026 ****************************************************************************** 00027 */ 00028 00029 /* Includes ------------------------------------------------------------------*/ 00030 #include "drv_I2C_M24SR.h" 00031 #include "mbed.h" 00032 00033 #define M24SR_SDA_PIN D14 00034 #define M24SR_SCL_PIN D15 00035 #define M24SR_GPO_PIN D12 00036 #define M24SR_RFDIS_PIN D11 00037 00038 #if defined __MBED__ /* F4 */ 00039 #define M24SR_I2C_SPEED_10 10000 00040 #define M24SR_I2C_SPEED_100 100000 00041 #define M24SR_I2C_SPEED_400 400000 00042 #define M24SR_I2C_SPEED_1000 M24SR_I2C_SPEED_400 // 1MHz I2C not supported by mbed 00043 #else 00044 #error "Use only for mbed" 00045 #endif 00046 00047 #define M24SR_I2C_SPEED M24SR_I2C_SPEED_400 00048 00049 /* MBED objects */ 00050 I2C i2c(I2C_SDA, I2C_SCL); 00051 DigitalOut pRFD(M24SR_RFDIS_PIN); 00052 DigitalIn pGPO(M24SR_GPO_PIN); 00053 00054 static uint8_t uSynchroMode = M24SR_WAITINGTIME_POLLING; 00055 00056 /** 00057 * @brief This function initializes the M24SR_I2C interface 00058 * @retval None 00059 */ 00060 void M24SR_I2CInit(void) 00061 { 00062 i2c.frequency(M24SR_I2C_SPEED); 00063 pRFD.write(0); 00064 pGPO.mode(PullNone); 00065 } 00066 00067 /** 00068 * @brief this functions configure I2C synchronization mode 00069 * @param mode : 00070 * @retval None 00071 */ 00072 void M24SR_SetI2CSynchroMode(uint8_t mode) 00073 { 00074 #if defined (I2C_GPO_SYNCHRO_ALLOWED) 00075 uSynchroMode = mode; 00076 #else 00077 if(mode == M24SR_WAITINGTIME_GPO || mode == M24SR_INTERRUPT_GPO) 00078 uSynchroMode = M24SR_WAITINGTIME_POLLING; 00079 else 00080 uSynchroMode = mode; 00081 #endif /* I2C_GPO_SYNCHRO_ALLOWED */ 00082 } 00083 00084 /** 00085 * @brief This functions polls the I2C interface 00086 * @retval M24SR_STATUS_SUCCESS : the function is succesful 00087 * @retval M24SR_ERROR_I2CTIMEOUT : The I2C timeout occured. 00088 */ 00089 int8_t M24SR_PollI2C(void) 00090 { 00091 int status = 1; 00092 uint32_t nbTry = 0; 00093 char buffer; 00094 // 00095 wait_ms(M24SR_POLL_DELAY); 00096 while (status != 0 && nbTry < M24SR_I2C_POLLING) 00097 { 00098 status = i2c.read(M24SR_ADDR, &buffer, 1, false); 00099 nbTry++; 00100 } 00101 if (status == 0) 00102 return M24SR_STATUS_SUCCESS; 00103 else 00104 { 00105 return M24SR_ERROR_I2CTIMEOUT; 00106 } 00107 } 00108 00109 /** 00110 * @brief This functions reads a response of the M24SR device 00111 * @param NbByte : Number of byte to read (shall be >= 5) 00112 * @param pBuffer : Pointer to the buffer to send to the M24SR 00113 * @retval M24SR_STATUS_SUCCESS : The function is succesful 00114 * @retval M24SR_ERROR_I2CTIMEOUT : The I2C timeout occured. 00115 */ 00116 int8_t M24SR_ReceiveI2Cresponse(uint8_t NbByte, uint8_t *pBuffer) 00117 { 00118 if (i2c.read(M24SR_ADDR, (char*)pBuffer, NbByte, false) == 0) 00119 return M24SR_STATUS_SUCCESS; 00120 else 00121 return M24SR_ERROR_I2CTIMEOUT; 00122 } 00123 00124 /** 00125 * @brief This functions sends the command buffer 00126 * @param NbByte : Number of byte to send 00127 * @param pBuffer : pointer to the buffer to send to the M24SR 00128 * @retval M24SR_STATUS_SUCCESS : the function is succesful 00129 * @retval M24SR_ERROR_I2CTIMEOUT : The I2C timeout occured. 00130 */ 00131 int8_t M24SR_SendI2Ccommand(uint8_t NbByte ,uint8_t *pBuffer) 00132 { 00133 if (i2c.write(M24SR_ADDR, (char*)pBuffer, NbByte, false) == 0) 00134 { 00135 return M24SR_STATUS_SUCCESS; 00136 } 00137 else 00138 { 00139 return M24SR_ERROR_I2CTIMEOUT; 00140 } 00141 } 00142 00143 /** 00144 * @brief This functions returns M24SR_STATUS_SUCCESS when a response is ready 00145 * @retval M24SR_STATUS_SUCCESS : a response of the M24LR is ready 00146 * @retval M24SR_ERROR_DEFAULT : the response of the M24LR is not ready 00147 */ 00148 int8_t M24SR_IsAnswerReady(void) 00149 { 00150 uint16_t status ; 00151 uint32_t retry = 0xFFFFF; 00152 uint8_t stable = 0; 00153 00154 switch (uSynchroMode) 00155 { 00156 case M24SR_WAITINGTIME_POLLING : 00157 errchk(M24SR_PollI2C ( )); 00158 return M24SR_STATUS_SUCCESS; 00159 00160 case M24SR_WAITINGTIME_TIMEOUT : 00161 // M24SR FWI=5 => (256*16/fc)*2^5=9.6ms but M24SR ask for extended time to program up to 246Bytes. 00162 // can be improved by 00163 wait_ms(80); 00164 return M24SR_STATUS_SUCCESS; 00165 00166 case M24SR_WAITINGTIME_GPO : 00167 do 00168 { 00169 if(pGPO.read() == 0) 00170 { 00171 stable ++; 00172 } 00173 retry --; 00174 } 00175 while(stable <5 && retry>0); 00176 if(!retry) 00177 goto Error; 00178 return M24SR_STATUS_SUCCESS; 00179 00180 default : 00181 return M24SR_ERROR_DEFAULT; 00182 } 00183 00184 Error : 00185 return M24SR_ERROR_DEFAULT; 00186 } 00187 00188 /** 00189 * @brief This function enable or disable RF communication 00190 * @param OnOffChoice: GPO configuration to set 00191 */ 00192 void M24SR_RFConfig_Hard(uint8_t OnOffChoice) 00193 { 00194 /* Disable RF */ 00195 if ( OnOffChoice != 0 ) 00196 { 00197 pRFD = 0; 00198 } 00199 else 00200 { 00201 pRFD = 1; 00202 } 00203 } 00204
Generated on Sat Jul 16 2022 11:22:31 by
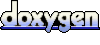