KX022 Accelerometer library
Dependents: LazuriteGraph_Hello KX022_Hello GR-PEACH_IoT_Platform_HTTP_sample
KX022.cpp
00001 /* Copyright (c) 2015 ARM Ltd., MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * 00019 * KX022 Accelerometer library 00020 * 00021 * @author Toyomasa Watarai 00022 * @version 1.0 00023 * @date 30-December-2015 00024 * 00025 * Library for "KX022 Accelerometer library" from Kionix a Rohm group 00026 * http://www.kionix.com/product/KX022-1020 00027 * 00028 */ 00029 00030 #include "mbed.h" 00031 #include "KX022.h" 00032 00033 KX022::KX022(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) 00034 { 00035 initialize(); 00036 } 00037 00038 KX022::KX022(I2C &i2c_obj, int addr) : m_i2c(i2c_obj), m_addr(addr) 00039 { 00040 initialize(); 00041 } 00042 00043 KX022::~KX022() 00044 { 00045 } 00046 00047 void KX022::initialize() 00048 { 00049 unsigned char buf; 00050 unsigned char reg[2]; 00051 00052 DEBUG_PRINT("KX022 init started\n\r"); 00053 readRegs(KX022_WHO_AM_I, &buf, sizeof(buf)); 00054 if (buf != KX022_WAI_VAL) { 00055 DEBUG_PRINT("KX022 initialization error. (WAI %d, not %d)\n\r", buf, KX022_WAI_VAL); 00056 DEBUG_PRINT("Trying to config anyway, in case there is some compatible sensor connected.\n\r"); 00057 } 00058 00059 reg[0] = KX022_CNTL1; 00060 reg[1] = 0x41; 00061 writeRegs(reg, 2); 00062 00063 reg[0] = KX022_ODCNTL; 00064 reg[1] = 0x02; 00065 writeRegs(reg, 2); 00066 00067 reg[0] = KX022_CNTL3; 00068 reg[1] = 0xD8; 00069 writeRegs(reg, 2); 00070 00071 reg[0] = KX022_TILT_TIMER; 00072 reg[1] = 0x01; 00073 writeRegs(reg, 2); 00074 00075 reg[0] = KX022_CNTL1; 00076 reg[1] = 0xC1; 00077 writeRegs(reg, 2); 00078 } 00079 00080 float KX022::getAccX() 00081 { 00082 return (float(getAccAxis(KX022_XOUT_L))/16384); 00083 } 00084 00085 float KX022::getAccY() 00086 { 00087 return (float(getAccAxis(KX022_YOUT_L))/16384); 00088 } 00089 00090 float KX022::getAccZ() 00091 { 00092 return (float(getAccAxis(KX022_ZOUT_L))/16384); 00093 } 00094 00095 void KX022::getAccAllAxis(float * res) 00096 { 00097 res[0] = getAccX(); 00098 res[1] = getAccY(); 00099 res[2] = getAccZ(); 00100 } 00101 00102 int16_t KX022::getAccAxis(uint8_t addr) 00103 { 00104 int16_t acc; 00105 uint8_t res[2]; 00106 00107 readRegs(addr, res, 2); 00108 acc = ((res[1] << 8) | res[0]); 00109 return acc; 00110 } 00111 00112 void KX022::readRegs(int addr, uint8_t * data, int len) 00113 { 00114 int read_nok; 00115 char t[1] = {addr}; 00116 00117 m_i2c.write(m_addr, t, 1, true); 00118 read_nok = m_i2c.read(m_addr, (char *)data, len); 00119 if (read_nok){ 00120 DEBUG_PRINT("Read fail\n\r"); 00121 } 00122 } 00123 00124 void KX022::writeRegs(uint8_t * data, int len) 00125 { 00126 m_i2c.write(m_addr, (char *)data, len); 00127 }
Generated on Fri Jul 15 2022 08:19:45 by
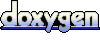