This is GR_PEACH_WlanBP3595 class library. The base library is EthernetInterface.
Dependents: GR-PEACH_WlanBP3595AP GR-PEACH_WlanBP3595STA
GR_PEACH_WlanBP3595.cpp
00001 /* GR_PEACH_WlanBP3595.cpp */ 00002 /* Copyright (C) 2016 Grape Systems, Inc. */ 00003 /* The base file is EthernetInterface.cpp. */ 00004 00005 /* EthernetInterface.cpp */ 00006 /* Copyright (C) 2012 mbed.org, MIT License 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00009 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00010 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00011 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in all copies or 00015 * substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00018 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00020 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00022 */ 00023 #include "GR_PEACH_WlanBP3595.h" 00024 #include "GR_PEACH_WlanBP3595_BssType.h" 00025 #include "WlanBP3595.h" 00026 00027 #include "lwip/inet.h" 00028 #include "lwip/netif.h" 00029 #include "netif/etharp.h" 00030 #include "lwip/dhcp.h" 00031 #include "wifi_arch.h" 00032 #include "lwip/tcpip.h" 00033 00034 #include "mbed.h" 00035 00036 /* TCP/IP and Network Interface Initialisation */ 00037 static struct netif netif; 00038 00039 static char mac_addr[19]; 00040 static char ip_addr[17] = "\0"; 00041 static char gateway[17] = "\0"; 00042 static char networkmask[17] = "\0"; 00043 static bool use_dhcp = false; 00044 00045 static Semaphore tcpip_inited(0); 00046 static Semaphore netif_linked(0); 00047 static Semaphore netif_up(0); 00048 static void (*_wlan_callback_func)(uint8_t ucType, uint16_t usWid, uint16_t usSize, uint8_t *pucData) = NULL; 00049 00050 static void tcpip_init_done(void *arg) { 00051 tcpip_inited.release(); 00052 } 00053 00054 static void netif_link_callback(struct netif *netif) { 00055 if (netif_is_link_up(netif)) { 00056 netif_linked.release(); 00057 } 00058 } 00059 00060 static void netif_status_callback(struct netif *netif) { 00061 if (netif_is_up(netif)) { 00062 strcpy(ip_addr, inet_ntoa(netif->ip_addr)); 00063 strcpy(gateway, inet_ntoa(netif->gw)); 00064 strcpy(networkmask, inet_ntoa(netif->netmask)); 00065 netif_up.release(); 00066 } 00067 } 00068 00069 static void init_netif(ip_addr_t *ipaddr, ip_addr_t *netmask, ip_addr_t *gw) { 00070 tcpip_init(tcpip_init_done, NULL); 00071 tcpip_inited.wait(); 00072 00073 memset((void*) &netif, 0, sizeof(netif)); 00074 netif_add(&netif, ipaddr, netmask, gw, NULL, wifi_arch_enetif_init, tcpip_input); 00075 netif_set_default(&netif); 00076 00077 netif_set_link_callback (&netif, netif_link_callback); 00078 netif_set_status_callback(&netif, netif_status_callback); 00079 } 00080 00081 static void set_mac_address(void) { 00082 snprintf(mac_addr, 19, "%02x:%02x:%02x:%02x:%02x:%02x", netif.hwaddr[0], netif.hwaddr[1], 00083 netif.hwaddr[2], netif.hwaddr[3], netif.hwaddr[4], netif.hwaddr[5]); 00084 } 00085 00086 static void _wlan_inf_callback(uint8_t ucType, uint16_t usWid, uint16_t usSize, uint8_t *pucData) { 00087 if ((ucType == 'I') && (usWid == 0x0005)) { 00088 if (pucData[0] == 0x01) { // CONNECTED 00089 /* Notify the EthernetInterface driver that WLAN was connected */ 00090 WlanBP3595_Connected(); 00091 } else { 00092 /* Notify the EthernetInterface driver that WLAN was disconnected */ 00093 WlanBP3595_Disconnected(); 00094 } 00095 } 00096 if (_wlan_callback_func != NULL) { 00097 _wlan_callback_func(ucType, usWid, usSize, pucData); 00098 } 00099 } 00100 00101 static int _wlan_init() { 00102 uint32_t status; 00103 00104 /* Initialize WlanBP3595 */ 00105 if (WlanBP3595_Init(&_wlan_inf_callback) != 0) { 00106 return -1; 00107 } 00108 00109 /* Wait until WLAN_BP3595_START timeout 60s */ 00110 while (1) { 00111 Thread::wait(200); 00112 status = WlanBP3595_GetWlanSts(); 00113 if (status == WLAN_BP3595_START) { 00114 break; 00115 } 00116 } 00117 00118 return 0; 00119 } 00120 00121 static int _wlan_setting(const char *ssid, const char *pass, nsapi_security_t security) 00122 { 00123 int ret; 00124 grp_u8 ucWidData8; // 8bit wid data 00125 grp_wld_byte_array tBAWidData; // byte array wid data 00126 00127 // Set BSS type 00128 ucWidData8 = BSS_TYPE; 00129 ret = WlanBP3595_Ioctl(GRP_WLD_IOCTL_SET_BSS_TYPE, &ucWidData8); 00130 if (ret != 0) { 00131 return -1; 00132 } 00133 00134 // Set SSID 00135 tBAWidData.pucData = (grp_u8 *)ssid; 00136 tBAWidData.ulSize = strlen((char *)tBAWidData.pucData); 00137 ret = WlanBP3595_Ioctl(GRP_WLD_IOCTL_SET_SSID, &tBAWidData); 00138 if (ret != 0) { 00139 return -1; 00140 } 00141 00142 if ((security == NSAPI_SECURITY_WPA) || (security == NSAPI_SECURITY_WPA2)) { 00143 // Set PSK 00144 tBAWidData.pucData = (grp_u8 *)pass; 00145 tBAWidData.ulSize = strlen((char *)tBAWidData.pucData); 00146 ret = WlanBP3595_Ioctl(GRP_WLD_IOCTL_SET_11I_PSK, &tBAWidData); 00147 if (ret != 0) { 00148 return -1; 00149 } 00150 } 00151 00152 // Set 11i mode 00153 switch (security) { 00154 case NSAPI_SECURITY_WEP: 00155 ret = strlen(pass); 00156 if (ret == 5) { 00157 ucWidData8 = 0x03; // WEP64 00158 } else if (ret == 13) { 00159 ucWidData8 = 0x07; // WEP128 00160 } else { 00161 return -1; 00162 } 00163 break; 00164 case NSAPI_SECURITY_WPA: 00165 case NSAPI_SECURITY_WPA2: 00166 ucWidData8 = 0x79; // WPA/WPA2 Mixed 00167 break; 00168 case NSAPI_SECURITY_NONE: 00169 default: 00170 ucWidData8 = 0x00; 00171 break; 00172 } 00173 ret = WlanBP3595_Ioctl(GRP_WLD_IOCTL_SET_11I_MODE, &ucWidData8); 00174 if (ret != 0) { 00175 return -1; 00176 } 00177 00178 if (security == NSAPI_SECURITY_WEP) { 00179 // Set WEP KEY 00180 tBAWidData.pucData = (grp_u8 *)pass; 00181 tBAWidData.ulSize = strlen((char *)tBAWidData.pucData); 00182 ret = WlanBP3595_Ioctl(GRP_WLD_IOCTL_SET_WEP_KEY, &tBAWidData); 00183 if (ret != 0) { 00184 return -1; 00185 } 00186 } 00187 00188 return 0; 00189 } 00190 00191 int GR_PEACH_WlanBP3595::init() { 00192 _wlan_init(); 00193 use_dhcp = true; 00194 init_netif(NULL, NULL, NULL); 00195 set_mac_address(); 00196 return 0; 00197 } 00198 00199 int GR_PEACH_WlanBP3595::init(const char* ip, const char* mask, const char* gateway) { 00200 _wlan_init(); 00201 use_dhcp = false; 00202 00203 strcpy(ip_addr, ip); 00204 00205 ip_addr_t ip_n, mask_n, gateway_n; 00206 inet_aton(ip, &ip_n); 00207 inet_aton(mask, &mask_n); 00208 inet_aton(gateway, &gateway_n); 00209 init_netif(&ip_n, &mask_n, &gateway_n); 00210 set_mac_address(); 00211 00212 return 0; 00213 } 00214 00215 int GR_PEACH_WlanBP3595::connect(const char *ssid, 00216 const char *pass, 00217 nsapi_security_t security, 00218 unsigned int timeout_ms) 00219 { 00220 _wlan_setting(ssid, pass, security); 00221 wifi_arch_enable_interrupts(); 00222 00223 int inited; 00224 if (use_dhcp) { 00225 dhcp_start(&netif); 00226 00227 // Wait for an IP Address 00228 // -1: error, 0: timeout 00229 inited = netif_up.wait(timeout_ms); 00230 } else { 00231 netif_set_up(&netif); 00232 00233 // Wait for the link up 00234 inited = netif_linked.wait(timeout_ms); 00235 } 00236 00237 return (inited > 0) ? (0) : (-1); 00238 } 00239 00240 int GR_PEACH_WlanBP3595::disconnect() { 00241 if (use_dhcp) { 00242 dhcp_release(&netif); 00243 dhcp_stop(&netif); 00244 } else { 00245 netif_set_down(&netif); 00246 } 00247 00248 wifi_arch_disable_interrupts(); 00249 00250 return 0; 00251 } 00252 00253 char* GR_PEACH_WlanBP3595::getMACAddress() { 00254 return mac_addr; 00255 } 00256 00257 char* GR_PEACH_WlanBP3595::getIPAddress() { 00258 return ip_addr; 00259 } 00260 00261 char* GR_PEACH_WlanBP3595::getGateway() { 00262 return gateway; 00263 } 00264 00265 char* GR_PEACH_WlanBP3595::getNetworkMask() { 00266 return networkmask; 00267 } 00268 00269 int GR_PEACH_WlanBP3595::WlanIoctl(uint32_t ulFunc, void *pvParam) { 00270 return WlanBP3595_Ioctl(ulFunc, pvParam); 00271 } 00272 00273 void GR_PEACH_WlanBP3595::setWlanCbFunction(void(*fptr)(uint8_t ucType, uint16_t usWid, uint16_t usSize, uint8_t *pucData)) { 00274 _wlan_callback_func = fptr; 00275 }
Generated on Tue Jul 12 2022 20:35:41 by
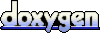