BM1383GLV pressure sensor library
Dependents: BM1383GLV_Hello LazuriteGraph_Hello GR-PEACH_IoT_Platform_HTTP_sample
BM1383GLV.h
00001 /* Copyright (c) 2015 ARM Ltd., MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * 00019 * BM1383GLV Pressure sensor library 00020 * 00021 * @author Toyomasa Watarai 00022 * @version 1.0 00023 * @date 30-December-2015 00024 * 00025 * Library for "BM1383GLV Pressure sensor library" 00026 * http://www.rohm.com/web/eu/news-detail?news-title=2014-12-11_ad_pressure&defaultGroupId=false 00027 * 00028 */ 00029 00030 #ifndef BM1383GLV_H 00031 #define BM1383GLV_H 00032 00033 #include "mbed.h" 00034 00035 #define BM1383GLV_DEFAULT_SLAVE_ADDRESS (0x5D << 1) 00036 #define BM1383GLV_ID_VAL (0x31) 00037 #define BM1383GLV_ID (0x10) 00038 #define BM1383GLV_POWER_DOWN (0x12) 00039 #define BM1383GLV_SLEEP (0x13) 00040 #define BM1383GLV_MODE_CONTROL (0x14) 00041 #define BM1383GLV_TEMPERATURE_MSB (0x1A) 00042 #define BM1383GLV_PRESSURE_MSB (0x1C) 00043 00044 #ifdef _DEBUG 00045 extern Serial pc; 00046 #define DEBUG_PRINT(...) pc.printf(__VA_ARGS__) 00047 #else 00048 #define DEBUG_PRINT(...) 00049 #endif 00050 00051 /** 00052 * BM1383GLV pressure sensor example 00053 * 00054 * @code 00055 * BM1383GLV sensor(I2C_SDA, I2C_SCL); 00056 * Serial pc(USBTX, USBRX); 00057 * 00058 * int main() { 00059 * pc.printf("\nBM1383GLV Pressure sensor library test program.\n"); 00060 * 00061 * while(1) { 00062 * pc.printf("pressure=%7.2f, temperature=%5.3f\n", sensor.getPressure(), sensor.getTemperature()); 00063 * wait(0.5); 00064 * } 00065 * } 00066 * @endcode 00067 */ 00068 class BM1383GLV 00069 { 00070 public: 00071 /** 00072 * BM1383GLV constructor 00073 * 00074 * @param sda SDA pin 00075 * @param sdl SCL pin 00076 * @param addr slave address of the I2C peripheral (default: 0xBA) 00077 */ 00078 BM1383GLV(PinName sda, PinName scl, int addr = BM1383GLV_DEFAULT_SLAVE_ADDRESS); 00079 00080 /** 00081 * Create a BM1383GLV instance which is connected to specified I2C pins 00082 * with specified address 00083 * 00084 * @param i2c_obj I2C object (instance) 00085 * @param addr slave address of the I2C-bus peripheral (default: 0xBA) 00086 */ 00087 BM1383GLV(I2C &i2c_obj, int addr = BM1383GLV_DEFAULT_SLAVE_ADDRESS); 00088 00089 /** 00090 * BM1383GLV destructor 00091 */ 00092 ~BM1383GLV(); 00093 00094 /** Initializa BM1383GLV sensor 00095 * 00096 * Configure sensor setting 00097 * 00098 */ 00099 void initialize(void); 00100 00101 /** 00102 * Get pressure 00103 * 00104 * @returns pressure 00105 */ 00106 float getPressure(); 00107 00108 /** 00109 * Get temerature 00110 * 00111 * @returns temperature 00112 */ 00113 float getTemperature(); 00114 00115 private: 00116 I2C m_i2c; 00117 int m_addr; 00118 uint8_t m_buf[3]; 00119 void readRegs(int addr, uint8_t * data, int len); 00120 void writeRegs(uint8_t * data, int len); 00121 00122 }; 00123 00124 #endif
Generated on Wed Jul 13 2022 11:03:57 by
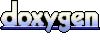