BM1383GLV pressure sensor library
Dependents: BM1383GLV_Hello LazuriteGraph_Hello GR-PEACH_IoT_Platform_HTTP_sample
BM1383GLV.cpp
00001 /* Copyright (c) 2015 ARM Ltd., MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * 00019 * BM1383GLV Pressure sensor library 00020 * 00021 * @author Toyomasa Watarai 00022 * @version 1.0 00023 * @date 30-December-2015 00024 * 00025 * Library for "BM1383GLV Pressure sensor library" 00026 * http://www.rohm.com/web/eu/news-detail?news-title=2014-12-11_ad_pressure&defaultGroupId=false 00027 * 00028 */ 00029 00030 #include "mbed.h" 00031 #include "BM1383GLV.h" 00032 00033 BM1383GLV::BM1383GLV(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) 00034 { 00035 initialize(); 00036 } 00037 00038 BM1383GLV::BM1383GLV(I2C &i2c_obj, int addr) : m_i2c(i2c_obj), m_addr(addr) 00039 { 00040 initialize(); 00041 } 00042 00043 BM1383GLV::~BM1383GLV() 00044 { 00045 } 00046 00047 void BM1383GLV::initialize() 00048 { 00049 unsigned char buf; 00050 unsigned char reg[2]; 00051 00052 readRegs(BM1383GLV_ID, &buf, sizeof(buf)); 00053 if (buf != BM1383GLV_ID_VAL) { 00054 DEBUG_PRINT("BM1383GLV initialization error. (%d)\n", buf); 00055 return; 00056 } 00057 00058 reg[0] = BM1383GLV_POWER_DOWN; 00059 reg[1] = 0x01; 00060 writeRegs(reg, 2); 00061 00062 wait(1); 00063 00064 reg[0] = BM1383GLV_SLEEP; 00065 reg[1] = 0x01; 00066 writeRegs(reg, 2); 00067 00068 reg[0] = BM1383GLV_MODE_CONTROL; 00069 reg[1] = 0xC4; 00070 writeRegs(reg, 2); 00071 } 00072 00073 float BM1383GLV::getPressure() 00074 { 00075 uint32_t rawPressure; 00076 00077 readRegs(BM1383GLV_PRESSURE_MSB, m_buf, 3); 00078 rawPressure = (((uint32_t)m_buf[0] << 16) | ((uint32_t)m_buf[1] << 8) | m_buf[2]&0xFC) >> 2; 00079 return (float)rawPressure / (2048); 00080 } 00081 00082 float BM1383GLV::getTemperature() 00083 { 00084 int32_t rawTemerature; 00085 00086 readRegs(BM1383GLV_TEMPERATURE_MSB, m_buf, 2); 00087 rawTemerature = ((int32_t)m_buf[0] << 8) | (m_buf[1]); 00088 return (float)rawTemerature / 32; 00089 } 00090 00091 void BM1383GLV::readRegs(int addr, uint8_t * data, int len) 00092 { 00093 char t[1] = {addr}; 00094 m_i2c.write(m_addr, t, 1, true); 00095 m_i2c.read(m_addr, (char *)data, len); 00096 } 00097 00098 void BM1383GLV::writeRegs(uint8_t * data, int len) 00099 { 00100 m_i2c.write(m_addr, (char *)data, len); 00101 }
Generated on Wed Jul 13 2022 11:03:57 by
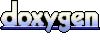