
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
x_nucleo_iks01a1_imu_6axes.c
00001 /** 00002 ****************************************************************************** 00003 * @file x_nucleo_iks01a1_imu_6axes.c 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file provides a set of functions needed to manage the lsm6ds0 sensor. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "x_nucleo_iks01a1_imu_6axes.h" 00039 #include "stdio.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup X_NUCLEO_IKS01A1 00046 * @{ 00047 */ 00048 00049 /** @addtogroup X_NUCLEO_IKS01A1_IMU_6AXES 00050 * @{ 00051 */ 00052 00053 00054 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_TypesDefinitions 00055 * @{ 00056 */ 00057 00058 /** 00059 * @} 00060 */ 00061 00062 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_Defines 00063 * @{ 00064 */ 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_Macros 00071 * @{ 00072 */ 00073 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_Variables 00079 * @{ 00080 */ 00081 static IMU_6AXES_DrvTypeDef *Imu6AxesDrv; 00082 static uint8_t Imu6AxesInitialized = 0; 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_FunctionPrototypes 00089 * @{ 00090 */ 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup X_NUCLEO_IKS01A1_IMU_6AXES_Private_Functions 00097 * @{ 00098 */ 00099 00100 /** 00101 * @brief Initialization 6 axes sensor. 00102 * @param None 00103 * @retval IMU_6AXES_OK if no problem during initialization 00104 */ 00105 IMU_6AXES_StatusTypeDef BSP_IMU_6AXES_Init(void) 00106 { 00107 IMU_6AXES_StatusTypeDef ret = IMU_6AXES_ERROR; 00108 IMU_6AXES_InitTypeDef LSM6DS0_InitStructure; 00109 00110 /* Initialize the six axes driver structure */ 00111 Imu6AxesDrv = &LSM6DS0Drv; 00112 00113 /* Configure sensor */ 00114 LSM6DS0_InitStructure.G_FullScale = LSM6DS0_G_FS_2000; 00115 LSM6DS0_InitStructure.G_OutputDataRate = LSM6DS0_G_ODR_119HZ; 00116 LSM6DS0_InitStructure.G_X_Axis = LSM6DS0_G_XEN_ENABLE; 00117 LSM6DS0_InitStructure.G_Y_Axis = LSM6DS0_G_YEN_ENABLE; 00118 LSM6DS0_InitStructure.G_Z_Axis = LSM6DS0_G_ZEN_ENABLE; 00119 00120 LSM6DS0_InitStructure.X_FullScale = LSM6DS0_XL_FS_2G; 00121 LSM6DS0_InitStructure.X_OutputDataRate = LSM6DS0_XL_ODR_119HZ; 00122 LSM6DS0_InitStructure.X_X_Axis = LSM6DS0_XL_XEN_ENABLE; 00123 LSM6DS0_InitStructure.X_Y_Axis = LSM6DS0_XL_YEN_ENABLE; 00124 LSM6DS0_InitStructure.X_Z_Axis = LSM6DS0_XL_ZEN_ENABLE; 00125 00126 /* six axes sensor init */ 00127 Imu6AxesDrv->Init(&LSM6DS0_InitStructure); 00128 00129 if(Imu6AxesDrv->Read_XG_ID() == I_AM_LSM6DS0_XG) 00130 { 00131 Imu6AxesInitialized = 1; 00132 ret = IMU_6AXES_OK; 00133 } 00134 00135 return ret; 00136 } 00137 00138 00139 uint8_t BSP_IMU_6AXES_isInitialized(void) 00140 { 00141 return Imu6AxesInitialized; 00142 } 00143 00144 00145 /** 00146 * @brief Read ID of LSM6DS0 Accelerometer and Gyroscope 00147 * @param None 00148 * @retval ID 00149 */ 00150 uint8_t BSP_IMU_6AXES_Read_XG_ID(void) 00151 { 00152 uint8_t id = 0x00; 00153 00154 if(Imu6AxesDrv->Read_XG_ID != NULL) 00155 { 00156 id = Imu6AxesDrv->Read_XG_ID(); 00157 } 00158 return id; 00159 } 00160 00161 00162 /** 00163 * @brief Check ID of LSM6DS0 Accelerometer and Gyroscope sensor 00164 * @param None 00165 * @retval Test status 00166 */ 00167 IMU_6AXES_StatusTypeDef BSP_IMU_6AXES_Check_XG_ID(void) { 00168 if (BSP_IMU_6AXES_Read_XG_ID() == I_AM_LSM6DS0_XG) { 00169 return IMU_6AXES_OK; 00170 } else { 00171 return IMU_6AXES_ERROR; 00172 } 00173 } 00174 00175 00176 /** 00177 * @brief Get Accelerometer raw axes 00178 * @param pData: pointer on AxesRaw_TypeDef data 00179 * @retval None 00180 */ 00181 void BSP_IMU_6AXES_X_GetAxesRaw(AxesRaw_TypeDef *pData) 00182 { 00183 if(Imu6AxesDrv->Get_X_Axes!= NULL) 00184 { 00185 Imu6AxesDrv->Get_X_Axes((int32_t *)pData); 00186 } 00187 } 00188 00189 00190 /** 00191 * @brief Get Gyroscope raw axes 00192 * @param pData: pointer on AxesRaw_TypeDef data 00193 * @retval None 00194 */ 00195 void BSP_IMU_6AXES_G_GetAxesRaw(AxesRaw_TypeDef *pData) 00196 { 00197 if(Imu6AxesDrv->Get_G_Axes!= NULL) 00198 { 00199 Imu6AxesDrv->Get_G_Axes((int32_t *)pData); 00200 } 00201 } 00202 00203 /** 00204 * @} 00205 */ 00206 00207 /** 00208 * @} 00209 */ 00210 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00220
Generated on Tue Jul 12 2022 21:42:00 by
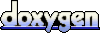