
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
lps25h.c
00001 /** 00002 ****************************************************************************** 00003 * @file LPS25H.c 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file provides a set of functions needed to manage the lps25h. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "lps25h.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32F439_SENSITRON 00045 * @{ 00046 */ 00047 00048 /** @addtogroup LPS25H 00049 * @{ 00050 */ 00051 00052 00053 /** @defgroup LPS25H_Private_TypesDefinitions 00054 * @{ 00055 */ 00056 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup LPS25H_Private_Defines 00062 * @{ 00063 */ 00064 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup LPS25H_Private_Macros 00070 * @{ 00071 */ 00072 00073 /** 00074 * @} 00075 */ 00076 00077 /** @defgroup LPS25H_Private_Variables 00078 * @{ 00079 */ 00080 00081 PRESSURE_DrvTypeDef LPS25HDrv = 00082 { 00083 LPS25H_Init, 00084 LPS25H_PowerOff, 00085 LPS25H_ReadID, 00086 LPS25H_RebootCmd, 00087 0, 00088 0, 00089 0, 00090 0, 00091 0, 00092 LPS25H_GetPressure, 00093 LPS25H_GetTemperature, 00094 LPS25H_SlaveAddrRemap 00095 }; 00096 00097 uint8_t LPS25H_SlaveAddress = LPS25H_ADDRESS_LOW; 00098 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup LPS25H_Private_FunctionPrototypes 00104 * @{ 00105 */ 00106 00107 /** 00108 * @brief Exit the shutdown mode for LPS25H. 00109 * @param None 00110 * @retval None 00111 */ 00112 void LPS25H_PowerOn(void); 00113 00114 void LPS25H_I2C_ReadRawPressure(uint32_t *raw_press); 00115 00116 void LPS25H_I2C_ReadRawTemperature(int16_t *raw_data); 00117 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup LPS25H_Private_Functions 00124 * @{ 00125 */ 00126 00127 00128 /** 00129 * @brief Set LPS25H Initialization. 00130 * @param InitStruct: it contains the configuration setting for the LPS25H. 00131 * @retval Error Code (PressureError_Enum) 00132 */ 00133 void LPS25H_Init(PRESSURE_InitTypeDef *LPS25H_Init) 00134 { 00135 uint8_t tmp1 = 0x00; 00136 00137 /* Configure the low level interface ---------------------------------------*/ 00138 PRESSURE_IO_Init(); 00139 00140 LPS25H_PowerOn(); 00141 00142 PRESSURE_IO_Read(&tmp1, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00143 00144 /* Output Data Rate selection */ 00145 tmp1 &= ~(LPS25H_ODR_MASK); 00146 tmp1 |= LPS25H_Init->OutputDataRate; 00147 00148 /* Interrupt circuit selection */ 00149 tmp1 &= ~(LPS25H_DIFF_EN_MASK); 00150 tmp1 |= LPS25H_Init->DiffEnable; 00151 00152 /* Block Data Update selection */ 00153 tmp1 &= ~(LPS25H_BDU_MASK); 00154 tmp1 |= LPS25H_Init->BlockDataUpdate; 00155 00156 /* Serial Interface Mode selection */ 00157 tmp1 &= ~(LPS25H_SPI_SIM_MASK); 00158 tmp1 |= LPS25H_Init->SPIMode; 00159 00160 PRESSURE_IO_Write(&tmp1, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00161 00162 PRESSURE_IO_Read(&tmp1, LPS25H_SlaveAddress, LPS25H_RES_CONF_ADDR, 1); 00163 00164 /* Serial Interface Mode selection */ 00165 tmp1 &= ~(LPS25H_P_RES_MASK); 00166 tmp1 |= LPS25H_Init->PressureResolution; 00167 00168 /* Serial Interface Mode selection */ 00169 tmp1 &= ~(LPS25H_T_RES_MASK); 00170 tmp1 |= LPS25H_Init->TemperatureResolution; 00171 00172 PRESSURE_IO_Write(&tmp1, LPS25H_SlaveAddress, LPS25H_RES_CONF_ADDR, 1); 00173 } 00174 00175 /** 00176 * @brief Read ID address of LPS25H 00177 * @param Device ID address 00178 * @retval ID name 00179 */ 00180 uint8_t LPS25H_ReadID(void) 00181 { 00182 uint8_t tmp; 00183 00184 /* Read WHO I AM register */ 00185 PRESSURE_IO_Read(&tmp, LPS25H_SlaveAddress, LPS25H_WHO_AM_I_ADDR, 1); 00186 00187 /* Return the ID */ 00188 return (uint8_t)tmp; 00189 } 00190 00191 /** 00192 * @brief Reboot memory content of LPS25H 00193 * @param None 00194 * @retval None 00195 */ 00196 void LPS25H_RebootCmd(void) 00197 { 00198 uint8_t tmpreg; 00199 00200 /* Read CTRL_REG5 register */ 00201 PRESSURE_IO_Read(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG2_ADDR, 1); 00202 00203 /* Enable or Disable the reboot memory */ 00204 tmpreg |= LPS25H_RESET_MEMORY; 00205 00206 /* Write value to MEMS CTRL_REG5 regsister */ 00207 PRESSURE_IO_Write(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG2_ADDR, 1); 00208 } 00209 00210 00211 /** 00212 * @brief Read LPS25H output register, and calculate the raw pressure. 00213 * @param uint32_t: raw_press. Pressure raw value. 00214 * @retval LPS25H_ERROR or LPS25H_OK. 00215 */ 00216 void LPS25H_I2C_ReadRawPressure(uint32_t *raw_press) 00217 { 00218 uint8_t buffer[3], i; 00219 uint32_t tempVal=0; 00220 00221 /* Read the register content */ 00222 00223 PRESSURE_IO_Read(buffer, LPS25H_SlaveAddress, LPS25H_PRESS_POUT_XL_ADDR+0x80, 3); 00224 // LPS25H_I2C_Read(LPS25H_PRESS_POUT_XL_ADDR+0x80, 3, buffer); 00225 00226 /* Build the raw data */ 00227 for (i = 0 ; i < 3 ; i++) 00228 tempVal |= (((uint32_t) buffer[i]) << (8 * i)); 00229 00230 /* convert the 2's complement 24 bit to 2's complement 32 bit */ 00231 if (tempVal & 0x00800000) 00232 tempVal |= 0xFF000000; 00233 00234 /* return the built value */ 00235 *raw_press = ((uint32_t) tempVal); 00236 } 00237 00238 /** 00239 * @brief Read LPS25H output register, and calculate the pressure in mbar. 00240 * @param float *pressure. Pressure value in mbar. 00241 * @retval LPS25H_ERROR or LPS25H_OK. 00242 */ 00243 void LPS25H_GetPressure(float* pfData) 00244 { 00245 uint32_t raw_press = 0; 00246 00247 LPS25H_I2C_ReadRawPressure(&raw_press); 00248 00249 /* return the built value */ 00250 //tempInt = raw_press / 4096; 00251 00252 *pfData = (float)raw_press /4096.0f; 00253 } 00254 00255 /** 00256 * @brief Read LPS25H output register, and calculate the raw temperature. 00257 * @param int16_t *raw_data: temperature raw value. 00258 * @retval LPS25H_ERROR or LPS25H_OK. 00259 */ 00260 void LPS25H_I2C_ReadRawTemperature(int16_t *raw_data) 00261 { 00262 uint8_t buffer[2]; 00263 uint16_t tempVal=0; 00264 00265 /* Read the register content */ 00266 PRESSURE_IO_Read(buffer, LPS25H_SlaveAddress, LPS25H_TEMP_OUT_L_ADDR+0x80, 2); 00267 // LPS25H_I2C_Read(LPS25H_TEMP_OUT_L_ADDR+0x80, 2, buffer); 00268 00269 /* Build the raw value */ 00270 tempVal = (((uint16_t)buffer[1]) << 8)+(uint16_t)buffer[0]; 00271 00272 /* Return it */ 00273 *raw_data = ((int16_t)tempVal); 00274 } 00275 00276 /** 00277 * @brief Read LPS25H output register, and calculate the temperature. 00278 * @param float *temperature : temperature value.. 00279 * @retval LPS25H_ERROR or LPS25H_OK. 00280 */ 00281 void LPS25H_GetTemperature(float* pfData) 00282 { 00283 int16_t raw_data; 00284 00285 LPS25H_I2C_ReadRawTemperature(&raw_data); 00286 00287 //*data_out = (int16_t)((((float)raw_data/480.0) + 42.5)*100); 00288 *pfData = (int16_t)((((float)raw_data/480.0) + 42.5)); 00289 } 00290 /** 00291 * @brief Exit the shutdown mode for LPS25H. 00292 * @param None 00293 * @retval LPS25H_ERROR or LPS25H_OK 00294 */ 00295 void LPS25H_PowerOn(void) 00296 { 00297 uint8_t tmpreg; 00298 00299 /* Read the register content */ 00300 // LPS25H_I2C_Read(LPS25H_CTRL_REG1_ADDR,1,&tmpReg); 00301 PRESSURE_IO_Read(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00302 00303 /* Set the power down bit */ 00304 tmpreg |= LPS25H_MODE_ACTIVE; 00305 00306 /* Write register */ 00307 // PRESSURE_IO_Write(LPS25H_CTRL_REG1_ADDR,1,&tmpReg); 00308 PRESSURE_IO_Write(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00309 00310 } 00311 00312 00313 /** 00314 * @brief Enter the shutdown mode for LPS25H. 00315 * @param None 00316 * @retval LPS25H_ERROR or LPS25H_OK 00317 */ 00318 void LPS25H_PowerOff(void) 00319 { 00320 uint8_t tmpreg; 00321 00322 /* Read the register content */ 00323 // PRESSURE_IO_Read( LPS25H_CTRL_REG1_ADDR,1,&tmpReg); 00324 PRESSURE_IO_Read(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00325 00326 /* Reset the power down bit */ 00327 tmpreg &= ~(LPS25H_MODE_ACTIVE); 00328 00329 /* Write register */ 00330 // PRESSURE_IO_Write( LPS25H_CTRL_REG1_ADDR,1,&tmpReg); 00331 PRESSURE_IO_Write(&tmpreg, LPS25H_SlaveAddress, LPS25H_CTRL_REG1_ADDR, 1); 00332 } 00333 00334 00335 /** 00336 * @brief Set the slave address according to SA0 bit. 00337 * @param SA0_Bit_Status: LPS25H_SA0_LOW or LPS25H_SA0_HIGH 00338 * @retval None 00339 */ 00340 void LPS25H_SlaveAddrRemap(uint8_t SA0_Bit_Status) 00341 { 00342 LPS25H_SlaveAddress = (SA0_Bit_Status==LPS25H_SA0_LOW?LPS25H_ADDRESS_LOW:LPS25H_ADDRESS_HIGH); 00343 } 00344 00345 /** 00346 * @} 00347 */ 00348 00349 /** 00350 * @} 00351 */ 00352 00353 /** 00354 * @} 00355 */ 00356 00357 /** 00358 * @} 00359 */ 00360 00361 00362 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00363
Generated on Tue Jul 12 2022 21:42:00 by
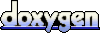