
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
lis3mdl.c
00001 /** 00002 ****************************************************************************** 00003 * @file lis3mdl.c 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file provides a set of functions needed to manage the lis3mdl. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "lis3mdl.h" 00039 #include <math.h> 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup MEMS_SHIELD 00046 * @{ 00047 */ 00048 00049 /** @addtogroup LIS3MDL 00050 * @{ 00051 */ 00052 00053 /** @defgroup LIS3MDL_Private_TypesDefinitions 00054 * @{ 00055 */ 00056 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup LIS3MDL_Private_Defines 00062 * @{ 00063 */ 00064 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup LIS3MDL_Private_Macros 00070 * @{ 00071 */ 00072 00073 /** 00074 * @} 00075 */ 00076 00077 /** @defgroup LIS3MDL_Private_Variables 00078 * @{ 00079 */ 00080 00081 MAGNETO_DrvTypeDef LIS3MDLDrv = 00082 { 00083 LIS3MDL_Init, 00084 LIS3MDL_Read_M_ID, 00085 LIS3MDL_M_GetAxes 00086 }; 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup LIS3MDL_Private_FunctionPrototypes 00093 * @{ 00094 */ 00095 00096 void LIS3MDL_M_GetAxesRaw(int16_t *pData); 00097 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup LIS3MDL_Private_Functions 00103 * @{ 00104 */ 00105 00106 00107 /** 00108 * @brief Set LIS3MDL Initialization. 00109 * @param InitStruct: it contains the configuration setting for the LIS3MDL. 00110 * @retval None 00111 */ 00112 void LIS3MDL_Init(MAGNETO_InitTypeDef *LIS3MDL_Init) 00113 { 00114 uint8_t tmp1 = 0x00; 00115 00116 /* Configure the low level interface ---------------------------------------*/ 00117 MAGNETO_IO_Init(); 00118 00119 00120 /****** Magnetic sensor *******/ 00121 00122 MAGNETO_IO_Read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG3_M, 1); 00123 00124 /* Conversion mode selection */ 00125 tmp1 &= ~(LIS3MDL_M_MD_MASK); 00126 tmp1 |= LIS3MDL_Init->M_OperatingMode; 00127 00128 MAGNETO_IO_Write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG3_M, 1); 00129 00130 00131 MAGNETO_IO_Read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG1_M, 1); 00132 00133 /* Output data rate selection */ 00134 tmp1 &= ~(LIS3MDL_M_DO_MASK); 00135 tmp1 |= LIS3MDL_Init->M_OutputDataRate; 00136 00137 /* X and Y axes Operative mode selection */ 00138 tmp1 &= ~(LIS3MDL_M_OM_MASK); 00139 tmp1 |= LIS3MDL_Init->M_XYOperativeMode; 00140 00141 MAGNETO_IO_Write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG1_M, 1); 00142 00143 00144 MAGNETO_IO_Read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00145 00146 /* Full scale selection */ 00147 tmp1 &= ~(LIS3MDL_M_FS_MASK); 00148 tmp1 |= LIS3MDL_Init->M_FullScale; 00149 00150 MAGNETO_IO_Write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00151 00152 /******************************/ 00153 } 00154 00155 00156 /** 00157 * @brief Read ID of LIS3MDL Magnetic sensor 00158 * @param Device ID 00159 * @retval ID name 00160 */ 00161 uint8_t LIS3MDL_Read_M_ID(void) 00162 { 00163 uint8_t tmp = 0x00; 00164 00165 /* Read WHO I AM register */ 00166 MAGNETO_IO_Read(&tmp, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_WHO_AM_I_ADDR, 1); 00167 00168 /* Return the ID */ 00169 return (uint8_t)tmp; 00170 } 00171 00172 00173 /** 00174 * @brief Read raw data from LIS3MDL Magnetic sensor output register. 00175 * @param float *pfData 00176 * @retval None. 00177 */ 00178 void LIS3MDL_M_GetAxesRaw(int16_t *pData) 00179 { 00180 uint8_t tempReg[2] = {0,0}; 00181 00182 00183 MAGNETO_IO_Read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_X_L_M + 0x80, 2); 00184 00185 pData[0] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00186 00187 MAGNETO_IO_Read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_Y_L_M + 0x80, 2); 00188 00189 pData[1] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00190 00191 MAGNETO_IO_Read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_Z_L_M + 0x80, 2); 00192 00193 pData[2] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00194 } 00195 00196 00197 /** 00198 * @brief Read data from LIS3MDL Magnetic sensor and calculate Magnetic in mgauss. 00199 * @param float *pfData 00200 * @retval None. 00201 */ 00202 void LIS3MDL_M_GetAxes(int32_t *pData) 00203 { 00204 00205 uint8_t tempReg = 0x00; 00206 int16_t pDataRaw[3]; 00207 float sensitivity = 0; 00208 00209 LIS3MDL_M_GetAxesRaw(pDataRaw); 00210 00211 MAGNETO_IO_Read(&tempReg, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00212 00213 tempReg &= LIS3MDL_M_FS_MASK; 00214 00215 switch(tempReg) 00216 { 00217 case LIS3MDL_M_FS_4: 00218 sensitivity = 0.14; 00219 break; 00220 case LIS3MDL_M_FS_8: 00221 sensitivity = 0.29; 00222 break; 00223 case LIS3MDL_M_FS_12: 00224 sensitivity = 0.43; 00225 break; 00226 case LIS3MDL_M_FS_16: 00227 sensitivity = 0.58; 00228 break; 00229 } 00230 00231 pData[0] = (int32_t)(pDataRaw[0] * sensitivity); 00232 pData[1] = (int32_t)(pDataRaw[1] * sensitivity); 00233 pData[2] = (int32_t)(pDataRaw[2] * sensitivity); 00234 } 00235 00236 /** 00237 * @} 00238 */ 00239 00240 /** 00241 * @} 00242 */ 00243 00244 /** 00245 * @} 00246 */ 00247 00248 /** 00249 * @} 00250 */ 00251 00252 00253 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00254
Generated on Tue Jul 12 2022 21:42:00 by
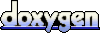