
Code petit robot
Dependencies: X_NUCLEO_COMMON X_NUCLEO_IHM01A1 mbed
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @author Julien Tiron, FIP Télécom Bretagne 00005 * @version V1.0.0 00006 * @date March 23th, 2016 00007 * @brief DoorCloser robot main code 00008 ****************************************************************************** 00009 **/ 00010 00011 /* Includes ------------------------------------------------------------------*/ 00012 00013 #include "mbed.h" 00014 #include "DevSPI.h" 00015 #include "l6474_class.h" 00016 #include "DevI2C.h" 00017 #include "vl6180x_class.h" 00018 00019 /* Definitions ---------------------------------------------------------------*/ 00020 00021 #define VL6180X_ADDRESS 0x29 00022 00023 /* Variables -----------------------------------------------------------------*/ 00024 00025 /* Start and Stop Component */ 00026 InterruptIn startup(PC_1); 00027 Ticker game_length; 00028 volatile bool start = 1; 00029 volatile bool end = 1; 00030 00031 /* Motor Control Component */ 00032 L6474 *motor1; 00033 L6474 *motor2; 00034 00035 /* Distance Sensors Component */ 00036 DevI2C *i2c =new DevI2C(D14, D15); 00037 VL6180X sensor1(i2c); 00038 VL6180X sensor2(i2c); 00039 VL6180X sensor3(i2c); 00040 00041 /* Functions -----------------------------------------------------------------*/ 00042 00043 void go() 00044 { 00045 start = 0; 00046 } 00047 00048 void stop() 00049 { 00050 end = 0; 00051 } 00052 00053 void init_sensor(){ 00054 00055 } 00056 00057 void switch_sensor(int number){ 00058 00059 } 00060 00061 /* Main ----------------------------------------------------------------------*/ 00062 00063 int main() 00064 { 00065 /*----- Initialization. -----*/ 00066 00067 /* Initializing SPI bus. */ 00068 DevSPI dev_spi(D11, D12, D13); 00069 00070 /* Initializing Motor Control Components. */ 00071 motor1 = new L6474(D2, D8, D7, D9, D10, dev_spi); 00072 motor2 = new L6474(D2, D8, D4, D3, D10, dev_spi); 00073 if (motor1->Init() != COMPONENT_OK) 00074 exit(EXIT_FAILURE); 00075 if (motor2->Init() != COMPONENT_OK) 00076 exit(EXIT_FAILURE); 00077 00078 /* Interrupt to start the robot */ 00079 startup.fall(&go); 00080 00081 /* Interrupt to stop the robot */ 00082 game_length.attach(&stop, 90); //1 minutes 30 secondes pour la Coupe 00083 00084 while(start) { 00085 /* Waiting code */ 00086 } 00087 00088 while(end) { 00089 /* In-game code */ 00090 00091 /* Requesting to run backward. */ 00092 motor1->Run(StepperMotor::BWD); 00093 motor2->Run(StepperMotor::FWD); 00094 00095 /* Waiting until delay has expired. */ 00096 wait_ms(3000); 00097 00098 motor1->HardStop(); 00099 motor2->HardStop(); 00100 00101 motor1->WaitWhileActive(); 00102 motor2->WaitWhileActive(); 00103 00104 /* Requesting to run backward. */ 00105 motor1->Run(StepperMotor::FWD); 00106 motor2->Run(StepperMotor::BWD); 00107 00108 /* Waiting until delay has expired. */ 00109 wait_ms(3000); 00110 00111 motor1->HardStop(); 00112 motor2->HardStop(); 00113 00114 motor1->WaitWhileActive(); 00115 motor2->WaitWhileActive(); 00116 } 00117 00118 motor1->HardStop(); 00119 motor2->HardStop(); 00120 00121 motor1->WaitWhileActive(); 00122 motor2->WaitWhileActive(); 00123 }
Generated on Thu Jul 14 2022 09:04:19 by
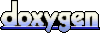