
Uses 2 HC-SR04 ultrasonic modules to steer the RenBuggy away from obstacles. Renishaw Team page fork.
Dependencies: mbed
Fork of RenBuggy_Ultrasonic by
ultrasonic_buggy.h
00001 /********************************************************* 00002 *buggy_functions.h * 00003 *Author: Elijah Orr & Dan Argust * 00004 * * 00005 *A library of functions that can be used to control the * 00006 *RenBuggy. * 00007 *********************************************************/ 00008 00009 /* include guards are used to prevent problems caused by 00010 multiple definitions */ 00011 #ifndef BUGGY_FUNCTIONS_H 00012 #define BUGGY_FUNCTIONS_H 00013 00014 /* mbed.h must be included in this file also */ 00015 #include "mbed.h" 00016 00017 /* #define LeftMotorPin p5 tells the preprocessor to replace 00018 any mention of LeftMotorPin with p5 etc. */ 00019 #define LEFT_MOTOR_PIN P0_9 00020 #define RIGHT_MOTOR_PIN P0_8 00021 00022 /* define pins to be used to operate the ultrasonic */ 00023 #define TRIGGER_PIN P0_11 00024 #define LEFT_ECHO_PIN P1_20 00025 #define RIGHT_ECHO_PIN P0_12 00026 00027 #define SPEED_OF_SOUND 340 //speed of sound =~340m/s at standard conditions 00028 00029 /* these are function prototypes that declare all the functions 00030 in the library.*/ 00031 extern void forward(float); //Move the buggy forward for (float) seconds 00032 extern void left(float); //Turn left for (float) seconds 00033 extern void right(float); //Turn right for (float) seconds 00034 extern void hold(float); //Hold the buggy in an idle state for (float) seconds 00035 extern void stop(); //Stop all motors 00036 00037 extern float getDistance_l(void); //get a distance reading in metres from the left sensor 00038 extern float getDistance_r(void); //get a distance reading in metres from the right sensor 00039 void resetSR04(void); //reset both ultrasonic modules 00040 00041 #endif // BUGGY_FUNCTIONS_H
Generated on Wed Jul 20 2022 03:45:32 by
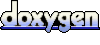