
Sample program showing how to connect GR-PEACH into Watson IoT through mbed Connector and Watson's Connector Bridge
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
OptionsBuilder.cpp
00001 /** 00002 * @file OptionsBuilder.cpp 00003 * @brief mbed CoAP OptionsBuilder class implementation 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // Class support 00024 #include "mbed-connector-interface/OptionsBuilder.h" 00025 00026 // ResourceObserver support 00027 #include "mbed-connector-interface/ThreadedResourceObserver.h" 00028 #include "mbed-connector-interface/TickerResourceObserver.h" 00029 #include "mbed-connector-interface/MinarResourceObserver.h" 00030 00031 // Connector namespace 00032 namespace Connector { 00033 00034 // Constructor 00035 OptionsBuilder::OptionsBuilder() 00036 { 00037 this->m_endpoint = NULL; 00038 this->m_domain = DEFAULT_DOMAIN; 00039 this->m_endpoint_type = DEFAULT_ENDPOINT_TYPE; 00040 this->m_node_name = NODE_NAME; 00041 this->m_lifetime = REG_LIFETIME_SEC; 00042 this->m_connector_url = string(CONNECTOR_URL); 00043 this->m_server_cert = NULL; 00044 this->m_server_cert_length = 0; 00045 this->m_client_cert = NULL; 00046 this->m_client_cert_length = 0; 00047 this->m_client_key = NULL; 00048 this->m_client_key_length = 0; 00049 this->m_device_resources_object = NULL; 00050 this->m_firmware_resources_object = NULL; 00051 this->m_static_resources.clear(); 00052 this->m_dynamic_resources.clear(); 00053 this->m_resource_observers.clear(); 00054 } 00055 00056 // Copy Constructor 00057 OptionsBuilder::OptionsBuilder(const OptionsBuilder &ob) : Options(ob) 00058 { 00059 this->m_endpoint = ob.m_endpoint; 00060 this->m_domain = ob.m_domain; 00061 this->m_endpoint_type = ob.m_endpoint_type; 00062 this->m_node_name = ob.m_node_name; 00063 this->m_lifetime = ob.m_lifetime; 00064 this->m_connector_url = ob.m_connector_url; 00065 this->m_server_cert = ob.m_server_cert; 00066 this->m_server_cert_length = ob.m_server_cert_length; 00067 this->m_client_cert = ob.m_client_cert; 00068 this->m_client_cert_length = ob.m_client_cert_length; 00069 this->m_client_key = ob.m_client_key; 00070 this->m_client_key_length= ob.m_client_key_length; 00071 this->m_device_resources_object = ob.m_device_resources_object; 00072 this->m_firmware_resources_object = ob.m_firmware_resources_object; 00073 this->m_static_resources = ob.m_static_resources; 00074 this->m_dynamic_resources = ob.m_dynamic_resources; 00075 this->m_resource_observers = ob.m_resource_observers; 00076 this->m_wifi_ssid = ob.m_wifi_ssid; 00077 this->m_wifi_auth_key = ob.m_wifi_auth_key; 00078 this->m_wifi_auth_type = ob.m_wifi_auth_type; 00079 this->m_coap_connection_type = ob.m_coap_connection_type; 00080 this->m_ip_address_type = ob.m_ip_address_type; 00081 this->m_enable_immediate_observation = ob.m_enable_immediate_observation; 00082 this->m_enable_get_obs_control = ob.m_enable_get_obs_control; 00083 this->m_endpoint = ob.m_endpoint; 00084 } 00085 00086 // Destructor 00087 OptionsBuilder::~OptionsBuilder() 00088 { 00089 this->m_device_resources_object = NULL; 00090 this->m_firmware_resources_object = NULL; 00091 this->m_static_resources.clear(); 00092 this->m_dynamic_resources.clear(); 00093 this->m_resource_observers.clear(); 00094 } 00095 00096 // set lifetime 00097 OptionsBuilder &OptionsBuilder::setLifetime(int lifetime) 00098 { 00099 this->m_lifetime = lifetime; 00100 return *this; 00101 } 00102 00103 // set domain 00104 OptionsBuilder &OptionsBuilder::setDomain(const char *domain) 00105 { 00106 this->m_domain = string(domain); 00107 return *this; 00108 } 00109 00110 // set endpoint nodename 00111 OptionsBuilder &OptionsBuilder::setEndpointNodename(const char *node_name) 00112 { 00113 this->m_node_name = string(node_name); 00114 return *this; 00115 } 00116 00117 // set lifetime 00118 OptionsBuilder &OptionsBuilder::setEndpointType(const char *endpoint_type) 00119 { 00120 this->m_endpoint_type = string(endpoint_type); 00121 return *this; 00122 } 00123 00124 // set Connector URL 00125 OptionsBuilder &OptionsBuilder::setConnectorURL(const char *connector_url) 00126 { 00127 if (connector_url != NULL) { 00128 this->m_connector_url = string(connector_url); 00129 } 00130 return *this; 00131 } 00132 00133 // add the device resources object 00134 OptionsBuilder &OptionsBuilder::setDeviceResourcesObject(const void *device_resources_object) 00135 { 00136 if (device_resources_object != NULL) { 00137 this->m_device_resources_object = (void *)device_resources_object; 00138 } 00139 return *this; 00140 } 00141 00142 // add the firmware resources object 00143 OptionsBuilder &OptionsBuilder::setFirmwareResourcesObject(const void *firmware_resources_object) 00144 { 00145 if (firmware_resources_object != NULL) { 00146 this->m_firmware_resources_object = (void *)firmware_resources_object; 00147 } 00148 return *this; 00149 } 00150 00151 // add static resource 00152 OptionsBuilder &OptionsBuilder::addResource(const StaticResource *resource) 00153 { 00154 if (resource != NULL) { 00155 ((StaticResource *)resource)->setOptions(this); 00156 this->m_static_resources.push_back((StaticResource *)resource); 00157 } 00158 return *this; 00159 } 00160 00161 // add dynamic resource 00162 OptionsBuilder &OptionsBuilder::addResource(const DynamicResource *resource) 00163 { 00164 // ensure that the boolean isn't mistaken by the compiler for the obs period... 00165 return this->addResource(resource,DEFAULT_OBS_PERIOD,!(((DynamicResource *)resource)->implementsObservation())); 00166 } 00167 00168 // add dynamic resource 00169 OptionsBuilder &OptionsBuilder::addResource(const DynamicResource *resource,const int sleep_time) 00170 { 00171 // ensure that the boolean isn't mistaken by the compiler for the obs period... 00172 return this->addResource(resource,sleep_time,!(((DynamicResource *)resource)->implementsObservation())); 00173 } 00174 00175 // add dynamic resource 00176 OptionsBuilder &OptionsBuilder::addResource(const DynamicResource *resource,const bool use_observer) 00177 { 00178 // ensure that the boolean isn't mistaken by the compiler for the obs period... 00179 return this->addResource(resource,DEFAULT_OBS_PERIOD,use_observer); 00180 } 00181 00182 // add dynamic resource 00183 OptionsBuilder &OptionsBuilder::addResource(const DynamicResource *resource,const int sleep_time,const bool use_observer) 00184 { 00185 if (resource != NULL) { 00186 this->m_dynamic_resources.push_back((DynamicResource *)resource); 00187 ((DynamicResource *)resource)->setOptions(this); 00188 ((DynamicResource *)resource)->setEndpoint((const void *)this->getEndpoint()); 00189 if (((DynamicResource *)resource)->isObservable() == true && use_observer == true) { 00190 // Establish the appropriate ResourceObserver 00191 #if defined (MCI_MINAR_SCHEDULER) 00192 // Minar-based Scheduler ResourceObserver 00193 MinarResourceObserver *observer = new MinarResourceObserver((DynamicResource *)resource,(int)sleep_time); 00194 #else 00195 #ifdef CONNECTOR_USING_THREADS 00196 // mbedOS RTOS Thread ResourceObserver 00197 ThreadedResourceObserver *observer = new ThreadedResourceObserver((DynamicResource *)resource,(int)sleep_time); 00198 #endif 00199 #ifdef CONNECTOR_USING_TICKER 00200 // mbed Ticker ResourceObserver 00201 TickerResourceObserver *observer = new TickerResourceObserver((DynamicResource *)resource,(int)sleep_time); 00202 #endif 00203 #endif 00204 // If no observer type is set in mbed-connector-interface/configuration.h (EndpointNetwork lib), then "observer" will be unresolved 00205 this->m_resource_observers.push_back(observer); 00206 00207 // immedate observation enablement option 00208 if (this->immedateObservationEnabled()) { 00209 observer->beginObservation(); 00210 } 00211 } 00212 } 00213 return *this; 00214 } 00215 00216 // set WiFi SSID 00217 OptionsBuilder &OptionsBuilder::setWiFiSSID(char *ssid) 00218 { 00219 this->m_wifi_ssid = string(ssid); 00220 return *this; 00221 } 00222 00223 // set WiFi AuthType 00224 OptionsBuilder &OptionsBuilder::setWiFiAuthType(WiFiAuthTypes auth_type) 00225 { 00226 this->m_wifi_auth_type = auth_type; 00227 return *this; 00228 } 00229 00230 // set WiFi AuthKey 00231 OptionsBuilder &OptionsBuilder::setWiFiAuthKey(char *auth_key) 00232 { 00233 this->m_wifi_auth_key = string(auth_key); 00234 return *this; 00235 } 00236 00237 // set the CoAP Connection Type 00238 OptionsBuilder &OptionsBuilder::setCoAPConnectionType(CoAPConnectionTypes coap_connection_type) 00239 { 00240 this->m_coap_connection_type = coap_connection_type; 00241 return *this; 00242 } 00243 00244 // set the IP Address Type 00245 OptionsBuilder &OptionsBuilder::setIPAddressType(IPAddressTypes ip_address_type) 00246 { 00247 this->m_ip_address_type = ip_address_type; 00248 return *this; 00249 } 00250 00251 // build out our immutable self 00252 Options *OptionsBuilder::build() 00253 { 00254 return (Options *)this; 00255 } 00256 00257 // Enable/Disable immediate observationing 00258 OptionsBuilder &OptionsBuilder::setImmedateObservationEnabled(bool enable) { 00259 this->m_enable_immediate_observation = enable; 00260 return *this; 00261 } 00262 00263 // Enable/Disable GET-based control of observations 00264 OptionsBuilder &OptionsBuilder::setEnableGETObservationControl(bool enable) { 00265 this->m_enable_get_obs_control = enable; 00266 return *this; 00267 } 00268 00269 // set the server certificate 00270 OptionsBuilder &OptionsBuilder::setServerCertificate(uint8_t *cert,int cert_size) { 00271 this->m_server_cert = cert; 00272 this->m_server_cert_length = cert_size; 00273 return *this; 00274 } 00275 00276 // set the client certificate 00277 OptionsBuilder &OptionsBuilder::setClientCertificate(uint8_t *cert,int cert_size) { 00278 this->m_client_cert = cert; 00279 this->m_client_cert_length = cert_size; 00280 return *this; 00281 } 00282 00283 // set the client key 00284 OptionsBuilder &OptionsBuilder::setClientKey(uint8_t *key,int key_size) { 00285 this->m_client_key = key; 00286 this->m_client_key_length = key_size; 00287 return *this; 00288 } 00289 00290 // set our endpoint 00291 void OptionsBuilder::setEndpoint(void *endpoint) { 00292 this->m_endpoint = endpoint; 00293 } 00294 00295 } // namespace Connector
Generated on Thu Jul 14 2022 21:25:54 by
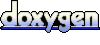