
This sample will play a ".wav" file of the USB root folder. Only RIFF format, 48kHz, 16bit, 2ch.
Dependencies: USBHostDac USBHost_custom_Addiso
Fork of USBHostDac_example by
main.cpp
00001 #include "mbed.h" 00002 #include "USBHostDac.h" 00003 #include "FATFileSystem.h" 00004 #include "USBHostMSD.h" 00005 #include "dec_wav.h" 00006 00007 #if defined(TARGET_RZ_A1H) 00008 #include "usb_host_setting.h" 00009 #else 00010 #define USB_HOST_CH 0 00011 #endif 00012 00013 #if (USB_HOST_CH == 1) //Audio Shield USB1 00014 DigitalOut usb1en(P3_8); 00015 #endif 00016 DigitalIn button(USER_BUTTON0); 00017 00018 #define AUDIO_WRITE_BUFF_SIZE (4096) 00019 #define FILE_NAME_LEN (64) 00020 #define TEXT_SIZE (64 + 1) //null-terminated 00021 #define FLD_PATH "/usb/" 00022 00023 static uint8_t audio_write_buff[AUDIO_WRITE_BUFF_SIZE]; 00024 //Tag buffer 00025 static uint8_t title_buf[TEXT_SIZE]; 00026 static uint8_t artist_buf[TEXT_SIZE]; 00027 static uint8_t album_buf[TEXT_SIZE]; 00028 00029 int main() { 00030 FILE * fp = NULL; 00031 DIR * d = NULL; 00032 char file_path[sizeof(FLD_PATH) + FILE_NAME_LEN]; 00033 size_t audio_data_size; 00034 dec_wav wav_file; 00035 00036 #if (USB_HOST_CH == 1) //Audio Shield USB1 00037 //Audio Shield USB1 enable 00038 usb1en = 1; //Outputs high level 00039 Thread::wait(5); 00040 usb1en = 0; //Outputs low level 00041 #endif 00042 00043 FATFileSystem fs("usb"); 00044 USBHostMSD msd; 00045 USBHostDac usbdac; 00046 00047 while(1) { 00048 // try to connect a MSD device 00049 while(!msd.connect()) { 00050 Thread::wait(500); 00051 } 00052 fs.mount(&msd); 00053 00054 // in a loop, append a file 00055 // if the device is disconnected, we try to connect it again 00056 while(1) { 00057 // if device disconnected, try to connect again 00058 if (!msd.connected()) { 00059 break; 00060 } 00061 if (fp == NULL) { 00062 // file search 00063 if (d == NULL) { 00064 d = opendir(FLD_PATH); 00065 } 00066 struct dirent * p; 00067 while ((p = readdir(d)) != NULL) { 00068 size_t len = strlen(p->d_name); 00069 if ((len > 4) && (len < FILE_NAME_LEN) 00070 && (memcmp(&p->d_name[len - 4], ".wav", 4) == 0)) { 00071 strcpy(file_path, FLD_PATH); 00072 strcat(file_path, p->d_name); 00073 fp = fopen(file_path, "r"); 00074 if (wav_file.AnalyzeHeder(title_buf, artist_buf, album_buf, 00075 TEXT_SIZE, fp) == false) { 00076 fclose(fp); 00077 fp = NULL; 00078 } else if ((wav_file.GetChannel() != 2) 00079 || (wav_file.GetBlockSize() != 16) 00080 || (wav_file.GetSamplingRate() != 48000)) { 00081 fclose(fp); 00082 fp = NULL; 00083 } else { 00084 printf("File :%s\n", p->d_name); 00085 printf("Title :%s\n", title_buf); 00086 printf("Artist:%s\n", artist_buf); 00087 printf("Album :%s\n", album_buf); 00088 printf("\n"); 00089 break; 00090 } 00091 } 00092 } 00093 if (p == NULL) { 00094 closedir(d); 00095 d = NULL; 00096 } 00097 } else { 00098 // file read 00099 uint8_t * p_buf = audio_write_buff; 00100 00101 audio_data_size = wav_file.GetNextData(p_buf, AUDIO_WRITE_BUFF_SIZE); 00102 if (audio_data_size > 0) { 00103 bool flush = false; 00104 00105 // try to connect a usbdac device 00106 while(!usbdac.connect()) { 00107 Thread::wait(500); 00108 } 00109 if (audio_data_size < AUDIO_WRITE_BUFF_SIZE) { 00110 flush = true; 00111 } 00112 usbdac.send(p_buf, audio_data_size, flush); 00113 } 00114 00115 // file close 00116 if ((audio_data_size < AUDIO_WRITE_BUFF_SIZE) || (button == 0)) { 00117 fclose(fp); 00118 fp = NULL; 00119 Thread::wait(500); 00120 } 00121 } 00122 } 00123 00124 // close check 00125 if (fp != NULL) { 00126 fclose(fp); 00127 fp = NULL; 00128 } 00129 if (d != NULL) { 00130 closedir(d); 00131 d = NULL; 00132 } 00133 } 00134 }
Generated on Wed Jul 13 2022 02:40:52 by
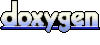