
Hello World for RGA(Renesas Graphics Architecture). RGA is the Graphics Library of RZ/A1. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependencies: GR-PEACH_video GraphicsFramework R_BSP LCD_shield_config
rga_func.h
00001 /* 00002 Permission is hereby granted, free of charge, to any person obtaining a copy 00003 of this software and associated documentation files (the "Software"), to deal 00004 in the Software without restriction, including without limitation the rights 00005 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00006 copies of the Software, and to permit persons to whom the Software is 00007 furnished to do so, subject to the following conditions: 00008 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00013 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00014 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00015 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00016 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00018 THE SOFTWARE. 00019 */ 00020 00021 #ifndef RGA_FUNC_H 00022 #define RGA_FUNC_H 00023 00024 #include "RGA.h" 00025 00026 typedef struct { 00027 char * style; 00028 int x; 00029 int y; 00030 int w; 00031 int h; 00032 } draw_rectangle_pos_t; 00033 00034 #define IMG_DRAW_WIDTH (241) 00035 #define IMG_DRAW_HEIGHT (180) 00036 #define IMAGE_WIDTH_SCROLL_FUNC (352) // Image width of Scroll function 00037 #define IMAGE_HEIGHT_SCROLL_FUNC (233) // Image height of Scroll function 00038 #define IMAGE_WIDTH_ZOOM_FUNC (352) // Image width of Zoom function 00039 #define IMAGE_HEIGHT_ZOOM_FUNC (233) // Image height of Zoom function 00040 00041 #define DISSOLVE_MAX_NUM (256) 00042 #define SCROLL_MAX_NUM (IMAGE_WIDTH_SCROLL_FUNC - IMG_DRAW_WIDTH) 00043 #define ZOOM_MAX_NUM (IMAGE_HEIGHT_ZOOM_FUNC / 2) 00044 #define ROTATION_MAX_NUM (360) 00045 #define ACCELERATE_MAX_NUM (256) 00046 00047 #define ANIMATION_TIMING_EASE (0) 00048 #define ANIMATION_TIMING_LINEAR (1) 00049 #define ANIMATION_TIMING_EASE_IN (2) 00050 #define ANIMATION_TIMING_EASE_OUT (3) 00051 #define ANIMATION_TIMING_EASE_IN_OUT (4) 00052 00053 extern void Set_RGAObject(frame_buffer_t* frmbuf_info); 00054 extern void RGA_Func_DrawTopScreen(frame_buffer_t* frmbuf_info); 00055 extern void RGA_Func_DrawRectangle(frame_buffer_t* frmbuf_info, draw_rectangle_pos_t * pos, int pos_num); 00056 extern void RGA_Func_DrawImage(frame_buffer_t* frmbuf_info, int x, int y); 00057 extern void RGA_Func_Dissolve(frame_buffer_t* frmbuf_info, float32_t global_alpha); 00058 extern void RGA_Func_Scroll(frame_buffer_t* frmbuf_info, int src_width_pos); 00059 extern void RGA_Func_Zoom(frame_buffer_t* frmbuf_info, int src_height_pos); 00060 extern void RGA_Func_Rotation(frame_buffer_t* frmbuf_info, graphics_matrix_float_t image_angle); 00061 extern void RGA_Func_Accelerate(frame_buffer_t* frmbuf_info, int animation_timing, float32_t relative_pos); 00062 00063 #endif
Generated on Sat Jul 16 2022 00:32:54 by
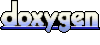